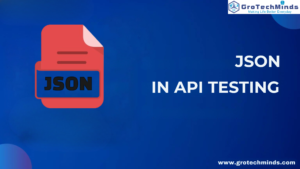
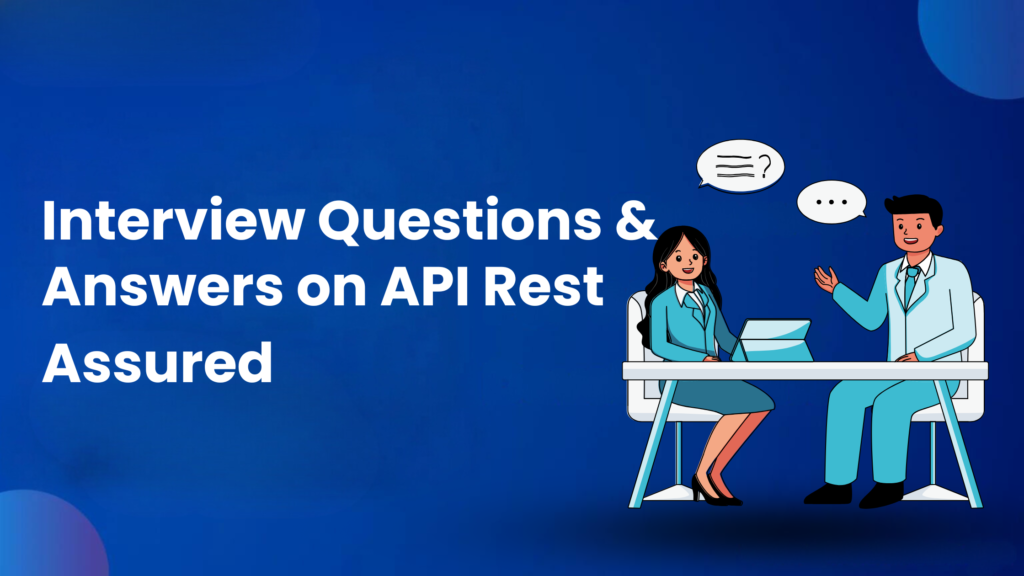
Interview Questions and Answers on API Rest Assured
Q1) What is POJO in Rest Assured?
Ans1) POJO stands for Plain Old Java Object. POJO is just like a regular object of Java. The task of POJO is to increase the reusability of the code. It is also used to improve the readability of the code.
Q2) What is the Serialization of POJO in Rest Assured?
Ans2) The serialization of POJO is converting a Java object (POJO) into JSON or XML format to send in an API request.
Q3) What is the Deserialization of POJO in Rest Assured?
Ans3) The deserialization of POJO converts a JSON or XML response into a Java object (POJO) for easy manipulation of the code
Q4) What is the ObjectMapper class in Rest Assured?
Ans4) The ObjectMapper class belongs to the Jackson data-bind package. It provides functionality for reading and writing JSON, either to and from basic POJOs (Plain Old Java Objects). We always use the ObjectMapper class to convert Java objects into JSON.
Q5) What is the syntax of the ObjectMapper class?
ObjectMapper obj=new ObjectMapper();
String empJson=obj.writerWithDefaultPrettyPrinter().writeValueAsString(variable of POJO class);
Q6) What are Cucumber Shells in Cucumber?
Ans6) Cucumber Shells concrete code is created at the backend then these unimplemented steps are added to the Step Definition package.
Q7) What is PicoContainer?
Ans7) PicoContainer is a dependency injection container that shares the context between multiple feature files.
Q8) What are Hooks in Cucumber?
Ans8) 1)@Before Hooks- Whatever is written in the @Before hooks tag will be executed first before each of the scenarios in the feature in the file.
2)@AfterHooks- Whatever is written in the @Affore hooks tag that will execute after each of the scenarios in the feature in the file.
3)@BeforeTaggedHooks-We can tag scenarios so whichever tagged scenario is called in BeforeHooks will execute after @Beforehooks.
4)@AfterTaggedHooks-We can tag scenarios so whichever tagged scenario is called in @AfterHooks will execute before tagged @After hooks.
Q9) What is the Background in Cucumber?
Ans9) When a set of features is repeated for multiple scenarios we can move that set of statements in put in the keyword called Background. In Background from the middle of the feature file, we cannot remove a set of statements it can only be removed from the start of the feature file and also we cannot parametrize a set of statements in Background. It will be executed for the scenarios that we have created under the feature file.
Q10) What is the order of execution in Cucumber?
Ans10) @Before Hooks
@Tagged Before Hooks
Background
Scenario Statements
@Tagged After Hooks
@After Hooks
Q11) What Is Test Runner in Cucumber?
Ans11) To control the flow of an execution we create a TestRunner class in the StepDefintion package. We have to add Cucumber JUnit dependency in pom.xml.
Q12) How do we handle SSL certificates in Rest Assured tests?
Ans12) SSL is a secured socket layer we need an SSL certificate so that any random request or any intruder cannot access the application. When we hit the private API HTTPS protocol request we have to bypass the SSL Layer(Secured Socket Layer) then only we will be able to access the API. We can bypass this with the help of the concept called relaxedHTTPSValidation() we are going to pass in the given() section. This means we are trusting all the hosts regardless if the SSL certificate is valid or not.
Q13) How do we fetch data from an Excel sheet in Rest Assured?
Ans13) We fetch the data from the Excel sheet and pass it in the payload. We need to add two APachePOI dependencies in pom.xml.Whenever we integrate Excelsheet, we have to consider it a workbook consisting of a number of sheets, and we also have to mention Rows and Columns.
The process to follow:
-Open the workbook, then we will go to the individual sheet and then we will select specific rows and columns.
-Create Object of XSSFWorkbook-it is a workspace where we are going to work
-Pass the sheet we need
-We need to pass data from rows and columns then we create an Object of DataFormatter.This is a Class which will help to fetch data from the Cell(from specific row and column)
File f=new File(“location of the file”);
XSSFWorkbook workbook=new XSSFWorkbook(f);
XSSFSheet sheet=workbook.getSheetAt(0);
DataFormatter formatter=new DataFormatter();
Objectvalue=formatter.formatCellValue(sheet.getRow(1).getCell(0));
String val=value.toString();
System.out.println(val);
Q14) What is the SessionFilter in Rest Assured?
Ans14) We receive a session ID each time we log into a session. We can work on the same session ID for extended periods without having to log out and back in. As long as we are using the application, the session ID will remain active. If we wish to execute a CRUD activity in RestAssured using the same session ID, we will use the SessionFilter concept, which will provide us with the session ID. Then, we will access the application using the same ID. With the help of the Java class SessionFilter, we can create an object, pass the same session ID across requests, and supply it to the specified given() Rest Assured section.
Q15) What is JSON in Rest Assured?
Ans15) Since it is lightweight, JSON(Java String Object Notation) is the most commonly used data exchange format between server and client. It is independent of any programming language. Data is stored in the structured format in a key-value pair. Keys will always be a String with double quotes( “ “) and values can be of any data type.
Q16) How do we pass three employee details in the request body?
Ans16) Firstly, we will use Map to add all the employee details
Secondly, we will merge all the details using List.
Thirdly, in the request body, we will send the List.
Q17) How do you handle file uploads in Rest Assured?
Ans17) We handle file upload methods by using the multipart() method in Rest Assured.
Firstly, we will create an Object of File class and add the location of the file.
Secondly, we can also create one more Object of the File class and add the location of the file.
Important:
Thirdly, in headers(“Content-Type”, “multipart/form-data”).
.multiPart(“file”,f)
.multiPart(“file”,f1)
Q18) What are static packages in Rest Assured?
Ans18) Static packages directly help us locate the specific class inside a package without creating a reference to it, and they also help provide better memory management. In Rest Assured there are different kinds of static packages that we have to import manually
Import static io.restassured.RestAssured.*;
- given()- in this API method we pass all the input in the request body like request headers(key-value pair), body, and query parameter(key-value pair).
- when()-in this API method we send which HTTP method and endpoint.
- then()-in this API method we pass all the validation like status code, time, and response headers.
Q19)What is the difference between Get and Post?
Get | Post |
It is used to retrieve the data | It is used to create the data |
There are no headers and body. | Headers and body are mandatory. |
The response code is 200. | The response code is 201. |
Q20)What is Hamcrest Matchers in Rest Assured?
Ans20) To validate only one response in the body, we need to import the static package known as Hamcrest Matchers manually. It has multiple methods; we usually use the equalTo() method.
Import static org.hamcrest.Matchers.*;
Q21) How do we validate the response in the Rest Assured?
Ans21) A specific class validates the response in the RestAssured library called JsonPath. It helps to traverse through JSON response and extract specific value from there.
Q22) How do we pass static payload in Rest Assured?
Ans22) We will pass static payload in the request body of the given() section
body(new String(Files.readAllBytes(Paths.get(“Location of the file”)))).
Conclusion
A crucial component of software quality assurance is API testing, which verifies the security, performance, dependability, and usefulness of APIs. By following this tutorial and gaining hands-on experience, you are equipping yourself with valuable knowledge that can propel your career in Software Testing Cucumber to new heights.A candidate’s technical proficiency, problem-solving abilities, and comprehension of real-world situations are demonstrated by their mastery of frequently asked interview questions and responses related to API testing.
Also read :
Consult Us