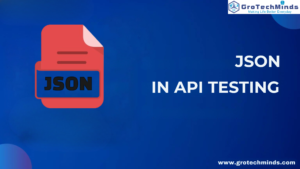

Playwright's Auto-Wait and Web-First Assertions in Python
Introduction
In Automation testing of software applications, waiting is one of the most important functionality. It is necessary to properly handle the waits for effective execution of tests. Playwright is one of the most popular tools that efficiently handles the wait mechanisms which helps in managing the dynamic web elements.
What is Playwright wait?
Playwright wait refers to the process which stops the execution of tests for a particular period of time until some conditions are satisfied like visibility of elements, presence of alerts and completion of network responses etc. The waits in playwright are important for the synchronization of tests for their effective execution especially during testing of dynamic web applications.
Why Wait is important in Playwright?
The waits in Playwright are important because of the following reasons:
- Playwright wait ensure that all the activities in the website like animations,UI changes etc. are completed fully before test execution thus reducing the chances of errors.
- If test scripts are executed before the elements are fully loaded then the test scripts will have flakiness and will give run time exception. The waits in playwright automatically waits for elements to get fully loaded which helps in effective execution of tests thus preventing flakiness in tests.
- Before we perform any activities on a web page like clicking on buttons, links, giving inputs to textbox it is necessary to apply wait until the element becomes visible in the web page. This prevents the occurrence of errors while trying to perform actions on hidden elements.
- Playwright ensures better customization of tests through wait commands like waitForSelector, waitForNavigation etc. as per the testing requirements.
- Playwright wait increases accuracy and reliability of tests.
When to use Wait?
We should use Playwright wait in the following scenarios:
- Whenever we need to stop the execution of test script
- We should use wait until the DOM structure of the application is completely loaded.
- Wait is used until a particular element of a web page is fully visible
- Wait is used until the navigation of a specific page of a website is done.
AutoWait in Playwright
Playwright has an inbuild autowait functionality which results in automatic waiting of the specified element or elements to get fully ready before performing actions. This is mostly used for performing actions like clicking a link, giving inputs to textbox etc. This reduces the need to use give wait manually, simplifies our codes and increases the effectiveness of tests.
For example for performing action on click component playwright waits for the below conditions to be satisfied:
- The element should be displayed
- The element should be enabled
- The element should have proper stability
- The locator should identity only one element at a time.
- The element should be able to receive events.
Types of Wait in Playwright
Automation testing is a software testing technique that uses specialised tools and scripts to run predefined test cases, compare the results to what was expected, and report the results.
The fundamental purpose of automated testing is to improve the efficiency, effectiveness, and coverage of the testing process by automating repetitive and time-consuming processes that would otherwise be completed manually
Below are the different types of wait commands used in Playwright
1.page.waitForTimeout(): This wait command is used to stop the execution of test script for a particular interval of time before the component is fully loaded. This method is mostly not preferred to be used as it provides delays and slowers the test execution. Below is the syntax for waitForTimeout command.
2.page.waitForSelector(): This command is used to wait for the element having similar selector to get fully displayed. This is the most preferred wait command as it ensures that the test execution will continue only if the element is fully ready. Below is the syntax for waitForSelector command to wait until the element is not visible.
Syntax: await page.waitForSelector(‘#search-button’);
The above syntax shows that playwright waits for ‘search button’ element to get fully displayed before proceeding for test execution.Below is another syntax for using waitForSelector command until the element disappears from web page
Syntax: await page.waitForSelector(‘#search-button’, ‘hidden’);
The above syntax shows that playwright waits until search button disappears from the web page so that test execution can proceed further.
3.page.waitForNavigation(): This command is used to pause the test execution until the page navigation is done. It is mostly used while testing movement from one page to another webpage, redirection features or any other action that causes navigation. Below is the syntax for waitForNavigation command.
Syntax: await page.waitForNavigation();
The above syntax shows that playwright waits until the navigation process from one web page to another gets completed.
4.page.waitForRequest(): This command is used to stop the tests until a specified request is sent. Suppose we are placing an order over an e commerce applicationsearching for a particular thing like “Playwright” over google then some tasks will be done before the search request is sent to backend. Below is the syntax for waitForRequest command.
Syntax: await page.waitForRequest(request => request.url()=== ‘https://www.google.com/search?q=Playwright’);
The above syntax shows that playwright pauses the tests until the search request is sent.
5.page.waitForResponse(): This command is used to pause the test execution until the specified response is received. It is mostly used for waiting on API responses as well as for other server interactions. It can be used in two ways which are as follows:
Wait for a matched response from a network request with a predicate:Below is the syntax for above condition
Syntax: await page.waitForResponse(response => response.url().includes(‘search?q=Playwright’)&& response.status==200 && response.request().method()===’GET’);
Wait for a complete URL to have a response:Below is the syntax for above condition
Syntax: page.waitForResponse(‘https://www.google.com/search?q=Playwright’);
6.page.waitForLoadState(): This command waits for a web page to reach a particular load stage like “load”,”networkidle” etc. This command ensures that the web page is fully loaded before any action is performed on the elements present in that page.Below is the syntax for the above wait command.
Syntax: await page.waitForLoadState(‘load’);
The above syntax shows playwright waits until the web page reaches the ‘load’ stage.
What are Assertions in Playwright?
Assertions in Playwright are in built methods that are used to check the behavior and state of the application that is under test. Using Assertions we can check whether some specific conditions are satisfied like checking the presence of element, presence of some texts in an element and checking the specified state of the element. Assertion is essential to validate that the application performs as per expectation during the end to end testing process. Playwright provides various assertion types which are as follows:
- State of Elements: It refers to testing the visibility and availablity of elements as well as validating interaction activity of elements having user interface.
- Validation of Contents: It refers to making sure that the element is displaying accurate texts, values and matching of particular patterns.
- Page Properties: It refers to the confirmation of various details of a web page like URL, title and presence of cookies.
- Interaction of Networks: It is used for validation of results of network requests and their respective responses thus resulting in proper loading of datas and effective submission of forms.
Different Types of Playwright Assertions
Playwright Assertions are divided into following categories
1.Auto-retrying Assertions- Auto-retrying Assertions are used for validation of assertions again and again until it is passed or the desired timeout is achieved. This assertion is very important in playwright as it enhances the effectiveness and reliability of test scripts. It is mostly useful in scenarios where the web elements do not immediately provide the expected results due to network issues, loading of dynamic contents and scripts activities from client side. Some of the important Auto-retrying Assertions are mentioned below:
Assertion Name | Description |
await expect(locator).toBeAttached() | It validates that the desired element is attached. |
await expect(locator).toBeChecked() | It ensures that the checkbox functionality is checked. |
await expect(locator).toBeDisabled() | It ensures that the element is disabled |
await expect(locator).toBeEditable() | It ensures that the element is editable |
await expect(locator).toBeEmpty() | It ensures that a particular web element or a container is empty |
await expect(locator).toBeEnabled() | It ensures that the given element is enabled. |
await expect(locator).toBeFocused() | It used for ensuring the element to be focused. |
await expect(locator).toBeHidden() | It ensures that the element is not visible. |
await expect(locator).toBeInViewport() | It ensures that the element is able to intersect viewport |
await expect(locator).toBeVisible() | It ensures the visibility of element. |
await expect(locator).toContainText() | It ensures that the element has texts in it. |
await expect(locator).toHaveAttribute() | It ensures that the element has a proper DOM attribute. |
await expect(locator).toHaveClass() | It ensures that the element has a class property. |
await expect(locator).toHaveCount() | It ensures that the given List has exact number of children. |
await expect(locator).toHaveCSS() | It ensures that the element has CSS characteristics |
await expect(locator).toHaveId() | It ensures that the element has ID |
await expect(locator).toHaveJSProperty() | It ensures that the element has javascript property |
await expect(locator).toHaveScreenshot() | It ensures that the element has screenshot |
await expect(locator).toHaveText() | It ensures that the element matches the given text. |
await expect(locator).toHaveValue() | It ensures that the input has a value |
await expect(locator).toHaveValues() | It ensures that the all the options of Select are selected. |
await expect(page).toHaveScreenshot() | It ensures that the page has a screenshot |
await expect(page).toHaveTitle() | It ensures that the page has a title |
await expect(page).toHaveURL() | It ensures that the page has a URL |
await expect(response).toBeOK() | It ensures that the status of Response is OK |
2.Non-retrying Assertions- Non- retrying Assertions are only used when the datas loaded by web pages are not synchronized. Assertion will fail without using retry and timeout. Some of the important Non- retrying Assertions are mentioned below.
Assertion Name | Description |
expect(value).toBe() | It means that the value is equal. |
expect(value).toBeCloseTo() | It means that the number is approximately equal. |
expect(value).toBeDefined() | It means that the value is not defined |
expect(value).toBeFalsy() | It means the given value is falsy i.e false, null, 0 |
expect(value).toBeGreaterThan() | It means that the given number is more than another number ‘s value |
expect(value).toBeGreaterThanOrEqual() | It means given number has value more than or equal to another number’s value. |
expect(value).toBeInstanceOf() | It means the Object or entity is an instance of a class. |
expect(value).toBeLessThan() | It means the given number is less than another number. |
expect(value).toBeLessThanOrEqual() | It means the given number is less than or equal to another number. |
expect(value).toBeNaN() | It means the value is NaN |
expect(value).toBeNull() | It means the value is Null |
expect(value).toBeTruthy() | It means the value is truthy. For example: not false,0,null |
expect(value).toBeUndefined() | It means value is undefined. |
expect(value).toContain(){String} | It means the given String has substring present in it. |
expect(value).toContain() | It means an Array or a set contains an element. |
expect(value).toContainEqual() | It means ans Array or a set has similar element. |
expect(value).toEqual() | It means that the value is equal. |
expect(value).toHaveLength() | It means the given array or string has length. |
expect(value).toHaveProperty() | It means Object has a property. |
expect(value).toMatch() | It means the given String matches with the regular expression. |
expect(value).toMatchObject() | It means the Object contains specified properties. |
expect(value).toStrictEqual() | It means the value as well as property types are similar. |
expect(value).toThrow() | It means the given function is giving an error. |
expect(value).any() | It means matching with any object of a class |
expect(value).anything() | It means matching with anything |
expect(value).arrayContaining() | It means the array has specific elements present in it. |
expect(value).closeTo() | It means the number is approximately equal. |
expect(value).objectContaining() | It means the object has specified properties. |
expect(value).stringContaining() | It means that the string contains a substring. |
expect(value).stringMatching() | It means the string matches with the regular expression. |
3.Negating Matchers- Negating Matchers are used in cases where we want to validate that a particular condition is false. In other words Negating Matchers checks the condition which is exactly opposite to what we are checking like absence of an element or a condition. Negating Matchers ensures that the web page or the application is free from defects, improper state and from unwanted elements.
4.Soft Assertions- Whenever there is difference between expected results and actual results, assertion will automatically stop the test execution. In many scenarios we have to verify numerous assertions and error is thrown after completion of tests. Soft Assertion is used whenever we want to validate large number of test cases and fail the tests towards the end of test execution. Without the usage of soft assertions the tests will fail after first assertion and will not proceed further.
Best Practices of Autowait and Assertions
To make the best use of Autowait and Assertions in playwright following best practices are needed to be followed
- Avoid using arbitrary waits: We should not use wait command waitForTimeout() since it result in arbitrary waits. Instead we should use the autowait commands like waitForSelector(), which only waits as per the requirements thus speeding up the test execution and enhances the reliability and efficiency of tests.
- Assertions should be used to achieve stability: Assertions act like a proper check to ensure that the element is in desired state thus resulting in its stability. Combining Assertion with autowait make our tests more faster, effective and reliable.
- Use Assertions after performing actions:In many scenarios actions like click() and fill() may result in improper updates in the software. Using assertions after completion of actions ensures that the application is in correct stage before proceeding for further actions.
- Always wait for navigation: After performing various navigation activities like clicking on a link component, clicking on submit button of a form and login page we should wait for the navigation action to complete. In such scenario we can use either waitForNavigation() command or the autowait feature of playwright for actions like click().
- Use Page and Locator waits: For the elements of a web page that needs to be loaded dynamically we should use page.waitForSelector() command with relevant wait conditions like (state: ‘attached’ , state: ‘visible’) in order to wait for those element to get displayed.
Conclusion
By combining Playwright’s autowait functionalities with assertions we can significantly increase the efficiency, reliability and robustness of our test scripts. This decreases the need of using arbitrary waits and make the tests run effectively thus ensuring that the actions are performed only if the elements meet the expected results. Remember to practise, stay updated with the latest trends in Python with Playwright Course,and maintain a positive attitude throughout your interview process. The main aim is to focus on element interactions that automatically wait for expected conditions to be satisfied before performing actions and to use to assertion to check the status of the web page or the status of the element of that web page at various stages of tests.
Also read:
Consult Us