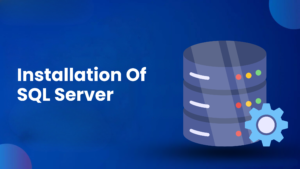
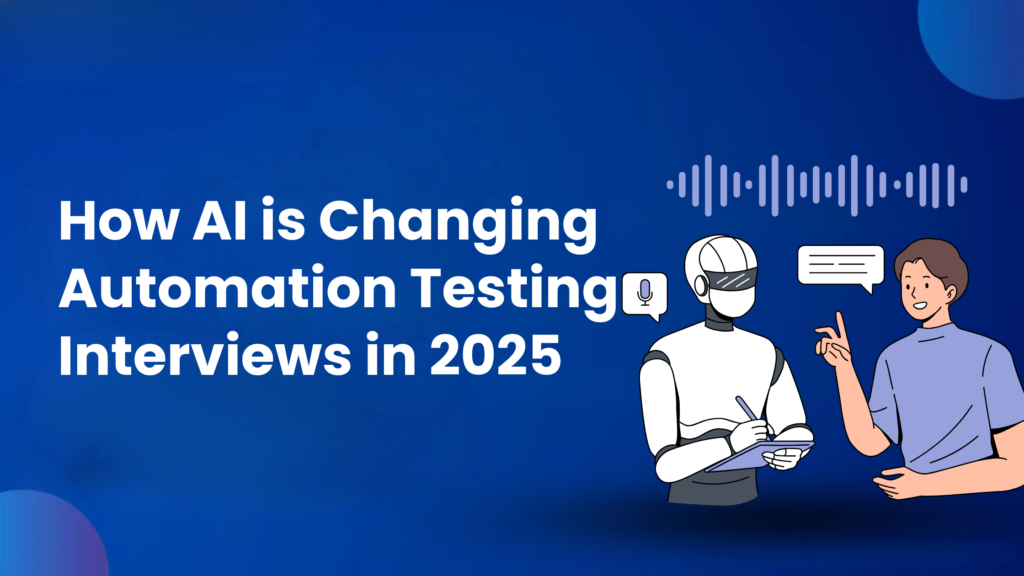
How AI is Changing Automation Testing Interviews in 2025 | Top 80+ Questions
Q1.) What is Selenium?
Selenium is an open-source automation testing tool used for testing web applications across various browsers and platforms. Its components include:Â Â
- Selenium WebDriver: Core for automating browser interactions. Â
- Selenium Grid: For running tests in parallel across different environments. Â
- Selenium IDE: A record-and-playback tool for beginners.
Q2.) What are the limitations of Selenium?Â
- Cannot test desktop or mobile applications directly. Â
- Limited support for image-based testing. Â
- No built-in reporting; relies on tools like TestNG or Extent Reports. Â
- Requires programming knowledge. Â
- Cannot handle CAPTCHA or OTP natively.
Q3.) What are the different types of waits in Selenium?
 Implicit Wait: Waits for elements globally. Example:Â
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
Explicit Wait: Waits for a specific condition. Example:
 WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
 wait.until(ExpectedConditions.visibilityOf(element))
Fluent Wait: Configures polling frequency and timeout.
Q4.) What is the difference between driver.get() and driver.navigate().to()?Â
- driver.get(): Waits until the page is fully loaded before proceeding. Â
- driver.navigate().to(): Does not wait for the full page load, allowing quicker navigation.Â
Q5.) How do you locate elements in Selenium?
Selenium locators include:
By.id: Locate elements by ID. Â
By.name: Locate elements by name. Â
By.className: Locate elements by class. Â
By.xpath: Locate elements using XPath. Example:  WebElement element = driver.findElement(By.xpath("//input[@id='username']"));   Â
By.cssSelector: Locate elements using CSS selectors.
Q6.) How do you handle pop-ups or alerts in Selenium?Â
 Switch to the alert:   Alert alert = driver.switchTo().alert();
 Accept alert:  alert.accept();
 Dismiss alert:  alert.dismiss();
 Retrieve text:   String alertText = alert.getText();
Q7.) How do you select a value from a dropdown?Â
Use the Select class:Â Â
Select dropdown = new Select(driver.findElement(By.id("dropdown")));
dropdown.selectByVisibleText("Option 1");Â // Select by text
dropdown.selectByValue("option1");Â Â Â Â // Select by value
dropdown.selectByIndex(0);Â Â Â Â Â Â Â Â // Select by index
Q8.) What is the difference between findElement() and findElements()?Â
- findElement(): Returns a single WebElement. Throws NoSuchElementException if not found. Â
- findElements(): Returns a list of WebElements. Returns an empty list if no elements are found.
Q9.) What are WebDriver commands you commonly use?Â
Navigation Commands:Â Â
driver.get(“URL”);Â Â
driver.navigate().refresh();
driver.navigate().back();
driver.navigate().forward();
Element Interaction Commands:Â Â
- element.click();Â Â
- element.sendKeys(“text”);
Browser Commands:Â Â
- driver.manage().window().maximize();Â Â
- driver.quit();
Q10.) How do you maximize a browser window in Selenium?Â
driver.manage().window().maximize();
This ensures that the browser is fully visible for interaction and testing.
Q11.) How do you perform mouse hover using Selenium?Â
Use the Actions class to perform mouse hover:Â Â
Actions actions = new Actions(driver);
WebElement element = driver.findElement(By.id("hoverElement"));
actions.moveToElement(element).perform();
This simulates moving the mouse pointer over the specified element.
Q12.) How do you handle multiple windows or tabs in Selenium?Â
Selenium uses getWindowHandles() to manage multiple windows:Â
String mainWindow = driver.getWindowHandle(); // Get the main window
Set allWindows = driver.getWindowHandles();
for (String window : allWindows) {
if (!window.equals(mainWindow)) {
driver.switchTo().window(window); // Switch to the new window
}
}
// Perform actions on the new window
driver.switchTo().window(mainWindow); // Switch back to the main window
Q13.) How do you switch to an iframe in Selenium?
Switch using index, name, or WebElement:
1. By Index:Â
driver.switchTo().frame(0);
2. By Name/ID:Â
driver.switchTo().frame("iframeName");
3. By WebElement:Â
WebElement iframe = driver.findElement(By.tagName("iframe"));
driver.switchTo().frame(iframe);
To exit the iframe:Â
driver.switchTo().defaultContent();
Q14.) What is the difference between isDisplayed(), isEnabled(), and isSelected()?Â
isDisplayed(): Checks if the element is visible on the page. Â
isEnabled(): Checks if the element is interactable (e.g., a button is clickable). Â
isSelected(): Checks if an option (e.g., checkbox or radio button) is selected. Â
Example:Â Â
boolean visible = element.isDisplayed();
boolean enabled = element.isEnabled();
boolean selected = checkbox.isSelected();
Q15.) How do you take a screenshot in Selenium?Â
Use TakesScreenshot interface:
TakesScreenshot screenshot = (TakesScreenshot) driver;
File srcFile = screenshot.getScreenshotAs(OutputType.FILE);
File destFile = new File("./screenshot/page.png");
FileUtils.copyFile(srcFile, destFile); // Requires Apache Commons IO
Or
FileHandler.copy(srcFile, destFile);
Q16.) What are the 3 top common exceptions in Selenium?Â
Common exceptions include:Â Â
- NoSuchElementException: Element not found. Â
- TimeoutException: Action took too long. Â
- StaleElementReferenceException: Element is no longer attached to the DOM.
Q17.) How do you scroll a webpage using Selenium?Â
1. Scroll to a specific position:
scriptExecutor js = (scriptExecutor) driver;
js.executeScript("window.scrollBy(0,500)"); // Scroll down 500 pixels
2. Scroll to an element:Â
js.executeScript("arguments[0].scrollIntoView(true);", element);
 Q18.) Explain how you perform drag-and-drop in Selenium.Â
Use the Actions class:Â
Actions actions = new Actions(driver);
WebElement source = driver.findElement(By.id("source"));
WebElement target = driver.findElement(By.id("target"));
actions.dragAndDrop(source, target).perform();
Alternatively, use click-and-hold:Â
actions.clickAndHold(source).moveToElement(target).release().perform();
 Q19.) How do you integrate Selenium with TestNG?Â
1. Add TestNG dependencies to your project.
2. Annotate your test methods with TestNG annotations like @Test and use assertions:Â
import org.testng.annotations.Test;
import org.testng.Assert;
public class SeleniumTest {
@Test
public void testExample() {
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
String title = driver.getTitle();
Assert.assertEquals(title, "Expected Title");
driver.quit();
}
}
Q20.) How do you validate tooltips in Selenium?Â
Tooltips are usually present in the title attribute of an element:Â
WebElement tooltipElement = driver.findElement(By.id("example"));
String tooltipText = tooltipElement.getAttribute("title");
System.out.println("Tooltip text: " + tooltipText);
 Q21.) How do you handle file uploads in Selenium?Â
For standard file upload dialogs, use sendKeys() to input the file path:
WebElement uploadButton = driver.findElement(By.id("fileUpload"));
uploadButton.sendKeys("C:\\path\\to\\file.txt");
 Q22.) How do you verify an element is present in Selenium?Â
Use findElements() to check for the element:Â Â
List elements = driver.findElements(By.id("example"));
if (elements.size() > 0) {
System.out.println("Element is present");
} else {
System.out.println("Element is not present");
}
 Q23.) What is the difference between hard and soft assertions in Selenium?Â
Hard Assertions (Assert): Stop the test immediately if the assertion fails.
Assert.assertEquals(actual, expected);
Soft Assertions (SoftAssert): Continue the test even if the assertion fails, allowing multiple validations.
SoftAssert softAssert = new SoftAssert();
softAssert.assertEquals(actual, expected);
softAssert.assertAll(); // Consolidates results at the end
Automation testing is a software testing technique that uses specialised tools and scripts to run predefined test cases, compare the results to what was expected, and report the results.
The fundamental purpose of automated testing is to improve the efficiency, effectiveness, and coverage of the testing process by automating repetitive and time-consuming processes that would otherwise be completed manually
Q24.) How do you perform parallel testing in Selenium?Â
Use TestNG with configuration in the testng.xml file:Â Â
 Q25.) What is a Page Object Model (POM) in Selenium?Â
POM is a design pattern that creates an object repository for storing WebElements. Â
– It separates test logic from UI interactions.Â
Example: Â
public class LoginPage {
WebDriver driver;
@FindBy(id = "username")
WebElement usernameField;
@FindBy(id = "password")
WebElement passwordField;
@FindBy(id = "loginButton")
WebElement loginButton;
public LoginPage(WebDriver driver) {
PageFactory.initElements(driver, this);
}
public void login(String username, String password) {
usernameField.sendKeys(username);
passwordField.sendKeys(password);
loginButton.click();
}
}
 Q26.) How do you execute a test on a remote machine using Selenium Grid?Â
- Set up Selenium Grid with a Hub and Nodes. Â
- Define the desired capabilities and initialize the remote WebDriver:Â
DesiredCapabilities capabilities = DesiredCapabilities.chrome();
URL gridURL = new URL("http://remote_host:4444/wd/hub");
WebDriver driver = new RemoteWebDriver(gridURL, capabilities);
 Q27.) How do you capture browser logs in Selenium?Â
Enable logging with ChromeOptions or DesiredCapabilities:Â
LogEntries logs = driver.manage().logs().get(LogType.BROWSER);
for (LogEntry log : logs) {
System.out.println(log.getMessage());
}
Automation testing is a software testing technique that uses specialised tools and scripts to run predefined test cases, compare the results to what was expected, and report the results.
The fundamental purpose of automated testing is to improve the efficiency, effectiveness, and coverage of the testing process by automating repetitive and time-consuming processes that would otherwise be completed manually
Q28.) What is the difference between absolute and relative XPath?
Absolute XPath: Specifies the complete path from the root node. Example:Â
/html/body/div[1]/header/a
Relative XPath: Starts from anywhere in the DOM. Example:Â Â
xpath
//div[@class='example']
 Q29.) How do you handle HTTPS certificates in Selenium?Â
Use ChromeOptions or DesiredCapabilities:
ChromeOptions options = new ChromeOptions();
options.setAcceptInsecureCerts(true);
WebDriver driver = new ChromeDriver(options);
 Q30.) How do you validate text on a webpage?
Use getText() to retrieve and compare text:
WebElement element = driver.findElement(By.id("textElement"));
String actualText = element.getText();
Assert.assertEquals(actualText, "Expected Text");
 Q31.) How do you minimize the browser window in Selenium?Â
console.log( 'Code is Poetry' );
 Q32.) What is the purpose of setProperty in Selenium?Â
setProperty specifies the path of the WebDriver executable for Selenium to control the browser:Â
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver.exe");
WebDriver driver = new ChromeDriver();
//this is no longer a concept in selenium in the latest versions
 Q33.) How do you manage browser cookies in Selenium?
Add a cookie:
Set cookies = driver.manage().getCookies();
Get all cookies:
Cookie cookie = new Cookie("key", "value");
driver.manage().addCookie(cookie);
Delete a cookie:
driver.manage().deleteCookie(cookie);
Q 34.) How do you handle file download in Selenium?Â
1. Set preferences for downloading files:Â
ChromeOptions options = new ChromeOptions();
options.addArguments("download.default_directory=/path/to/download");
WebDriver driver = new ChromeDriver(options);
2. Manually handle file downloads by checking the download folder for the downloaded file.
Q 35.) What is Fluent Wait in Selenium?
Fluent Wait defines the maximum time to wait for a condition and checks the condition at regular intervals:Â Â
Wait wait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(2))
.ignoring(NoSuchElementException.class);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("example")));
 Q36.) What is WebDriverWait and its usage?Â
WebDriverWait is an explicit wait that waits until a condition is met:Â
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("button")));
element.click();
 Q37.) How do you handle alerts in Selenium?Â
Use Alert interface:Â
1. Accept alert:Â
Alert alert = driver.switchTo().alert();
alert.accept();
2. Dismiss alert:Â
alert.dismiss();
3. Get alert text:
String alertText = alert.getText();
4. Send input to prompt alerts:Â
alert.sendKeys("Input text");
alert.accept();
 Q38.) What is the difference between driver.close() and driver.quit()?Â
- driver.close(): Closes the current browser tab. Â
- driver.quit(): Closes all browser tabs and ends the WebDriver session.Â
Q 39.) How do you perform right-click (context click) in Selenium?Â
Use the Actions class:Â
Actions actions = new Actions(driver);
WebElement element = driver.findElement(By.id("contextClickElement"));
actions.contextClick(element).perform();
Q 40.) What are the different types of waits in Selenium?Â
1. Implicit Wait: Sets a global wait time for finding elements.
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
2. Explicit Wait: Waits for a specific condition to be met.Â
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("example")));
3. Fluent Wait: Customizes the wait behavior (polling interval, exceptions to ignore).Â
Wait wait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(2))
.ignoring(NoSuchElementException.class);
 Q41.) How do you handle dropdowns in Selenium?
Use the Select class to interact with dropdowns:Â
1. Select by visible text:
Select dropdown = new Select(driver.findElement(By.id("dropdown")));
dropdown.selectByVisibleText("Option1");
2. Select by index:
dropdown.selectByIndex(2);
3. Select by value:
dropdown.selectByValue("optionValue");
Q 42.) How do you scroll a webpage in Selenium?
Use script Executor:Â
1. Scroll to a specific element:
scriptExecutor js = (scriptExecutor) driver;
js.executeScript("arguments[0].scrollIntoView(true);", element);
2. Scroll by coordinates:
js.executeScript("window.scrollBy(0,500)");
3. Scroll to the bottom of the page:
js.executeScript("window.scrollTo(0, document.body.scrollHeight)");
Q 43.) How do you handle frames and iframes in Selenium?Â
Switch to the frame before interacting with its elements:
1. Switch by index:
driver.switchTo().frame(0);
2. Switch by name or ID:
driver.switchTo().frame("frameName");
3. Switch by WebElement:
WebElement iframe = driver.findElement(By.tagName("iframe"));
driver.switchTo().frame(iframe);
4. To exit the frame:
driver.switchTo().defaultContent();
Q44.) How do you handle stale element exceptions in Selenium?Â
1. Re-locate the element:
WebElement element = driver.findElement(By.id("example"));
element.click(); // Re-locate if a StaleElementReferenceException occurs
2. Use explicit waits to wait for the element to reload:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.refreshed(ExpectedConditions.elementToBeClickable(By.id("example"))));
Q46.) How do you perform drag and drop in Selenium?Â
Use the Actions class:Â
Actions actions = new Actions(driver);
WebElement source = driver.findElement(By.id("sourceElement"));
WebElement target = driver.findElement(By.id("targetElement"));
actions.dragAndDrop(source, target).perform();
Q47.) What are Selenium Grid and its advantages?Â
Selenium Grid is used for distributed execution of tests across multiple environments:Â Â
- Allows parallel testing on multiple machines and browsers. Â
- Reduces execution time. Â
- Supports cross-platform and cross-browser testing.
Q 48.) How do you verify the title of a webpage in Selenium?Â
Use getTitle() to fetch and compare the title:
String actualTitle = driver.getTitle();
Assert.assertEquals(actualTitle, "Expected Page Title");
Q 49.) How do you check if a radio button is selected in Selenium?Â
Use the isSelected() method:
WebElement radioButton = driver.findElement(By.id("radioButtonID"));
if (radioButton.isSelected()==true) {
System.out.println("Radio button is selected");
} else {
System.out.println("Radio button is not selected");
}
Q 50.) How do you get the current URL of a webpage in Selenium?
Use the getCurrentUrl() method:Â
String currentUrl = driver.getCurrentUrl();
System.out.println("Current URL: " + currentUrl);
Q 51.) How do you get the page source of a webpage in Selenium?
Use the getPageSource() method:
String pageSource = driver.getPageSource();
System.out.println("Page Source: " + pageSource);
Q52.) What is the difference between findElement() and findElements()?
findElement(): Returns the first matching element or throws a NoSuchElementException if no element is found.
WebElement element = driver.findElement(By.id("elementID"));
findElements(): Returns a list of all matching elements. If no elements are found, it returns an empty list.
List elements = driver.findElements(By.className("className"));
Q53.) How do you perform double-click in Selenium?Â
Use the Actions class:
Actions actions = new Actions(driver);
WebElement element = driver.findElement(By.id("doubleClickElement"));
actions.doubleClick(element).perform();
Q54.) How do you close all browser windows in Selenium?Â
Use the quit() method:Â
driver.quit();
Q55.) What are the advantages of using Selenium for automation testing?
- Open-source and free to use. Â
- Supports multiple browsers (Chrome, Firefox, Safari, etc.). Â
- Provides cross-platform support (Windows, Mac, Linux). Â
- Integrates with test frameworks (JUnit, TestNG). Â
- Supports multiple programming languages (, Python, C#, etc.). Â
- Enables parallel testing with Selenium Grid.Â
Q 56.) How do you switch between multiple tabs in Selenium?Â
Automation testing is a software testing technique that uses specialised tools and scripts to run predefined test cases, compare the results to what was expected, and report the results.
The fundamental purpose of automated testing is to improve the efficiency, effectiveness, and coverage of the testing process by automating repetitive and time-consuming processes that would otherwise be completed manually
Use getWindowHandles() and switchTo().window():
Set allWindows = driver.getWindowHandles();
for (String window : allWindows) {
driver.switchTo().window(window);
// Perform actions on each tab if needed
}
Q57.) How do you verify if an element is enabled on a webpage in Selenium?Â
Use the isEnabled() method:
WebElement element = driver.findElement(By.id("elementID"));
if (element.isEnabled()==true) {
System.out.println("Element is enabled");
} else {
System.out.println("Element is disabled");
}
Q58.) How do you clear the contents of a text box in Selenium?Â
Use the clear() method:
WebElement textBox = driver.findElement(By.id("textBoxID"));
textBox.clear();
Q59.) How do you retrieve the tag name of an element in Selenium?
Use the getTagName() method:Â
WebElement element = driver.findElement(By.id("elementID"));
String tagName = element.getTagName();
System.out.println("Tag name: " + tagName);
Q60.) How do you perform keyboard operations in Selenium?Â
Use the Actions class:Â
1. Send keys combination:
Actions actions = new Actions(driver);
actions.sendKeys(Keys.CONTROL, "a").perform(); // Ctrl + A
2. Press and release keys:
actions.keyDown(Keys.SHIFT).sendKeys("text").keyUp(Keys.SHIFT).perform();
Q61.) How do you handle dynamic elements in Selenium?
1. Use dynamic locators like XPath with conditions:
WebElement element = driver.findElement(By.xpath("//button[contains(text(),'Submit')]"));
2. Use explicit waits to wait for the element to load:Â
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamicElement")));
Q62.) Explain the differences between Selenium Grid and Selenium Standalone Server.
- Selenium Standalone Server: Used for running Selenium tests on a single machine.
- Selenium Grid: Allows running tests in parallel on multiple machines (nodes), enabling distributed test execution and cross-browser testing.
Q63.) What are the best practices for designing a test automation framework?
- Use a modular design (Page Object Model or Screenplay Pattern).
- Incorporate a data-driven or keyword-driven approach for scalability.
- Centralize configuration files (e.g., test data, browser settings).
- Implement logging, reporting, and exception handling mechanisms.
- Integrate with CI/CD tools like Jenkins for continuous testing.
- Optimize tests to reduce execution time (e.g., parallel execution).
Q64.) How do you implement parallel testing in Selenium?
Parallel testing can be implemented using:
TestNG: Configure parallel attribute in testng.xml.
xml
...
...
Selenium Grid: Set up a hub and multiple nodes to execute tests on different environments concurrently.
Q65.) What are some key differences between Selenium and Cypress?
Feature | Selenium | Cypress |
Language Support | Multiple (, Python, etc.) | Script only |
Browser Support | All major browsers | Primarily Chromium-based |
Execution Speed | Relatively slower | Faster, runs in same browser |
Debugging | Requires external tools | Built-in time travel and debugging |
Community Support | Larger, older community | Rapidly growing community |
Q66.) How would you handle CAPTCHA or reCAPTCHA in Selenium?
Selenium cannot handle CAPTCHA directly. Possible solutions include:
- Bypassing CAPTCHA: Use test environments where CAPTCHA is disabled.
- Third-party APIs: Use services like Anti-Captcha or 2Captcha to solve CAPTCHAs.
- Manual Intervention: Use breaks in the test for manual CAPTCHA entry.
Q67.) What are TestNG Listeners, and how are they used?
TestNG Listeners are interfaces that allow customizing test execution by listening to events (e.g., test start, test success, test failure). Commonly used listeners include:
- ITestListener: For tracking test lifecycle events.
- ISuiteListener: For suite-level events.
Example:
public class TestListener implements ITestListener {
@Override
public void onTestFailure(ITestResult result) {
System.out.println("Test Failed: " + result.getName());
}
}
Q68.) Explain how to validate an API response in an automation test.
Using RestAssured in :
import io.restassured.RestAssured;
import io.restassured.response.Response;
Response response = RestAssured.get("https://api.example.com/users/1");
Assert.assertEquals(response.getStatusCode(), 200);
Assert.assertTrue(response.getBody().asString().contains("username"));
Here, you validate:
- Status Code
- Response Body
- Headers
Q69.) How do you handle browser notifications in Selenium?
Chrome: Use ChromeOptions to disable notifications.
ChromeOptions options = new ChromeOptions();
options.addArguments("--disable-notifications");
WebDriver driver = new ChromeDriver(options);
Firefox: Similar setup with FirefoxOptions.
Q70.) How do you test web applications with basic authentication pop-ups in Selenium?
Pass credentials in the URL:
driver.get("https://username:password@example.com");
Automation testing is a software testing technique that uses specialised tools and scripts to run predefined test cases, compare the results to what was expected, and report the results.
The fundamental purpose of automated testing is to improve the efficiency, effectiveness, and coverage of the testing process by automating repetitive and time-consuming processes that would otherwise be completed manually
Q71.) What is the purpose of the scriptExecutor interface, and when should you use it?
The scriptExecutor interface is used to execute Script in the browser.
Common uses:
Scrolling:
((scriptExecutor) driver).executeScript("window.scrollTo(0, document.body.scrollHeight);");
Interacting with hidden elements:
((scriptExecutor) driver).executeScript("arguments[0].click();", hiddenElement);
Fetching page title or inner HTML:
console.log( 'Code is Poetry' );
Q72.) How do you handle a situation where the page load takes too long in Selenium?
Set a page load timeout:Â
driver.manage().timeouts().pageLoadTimeout(Duration.ofSeconds(30));
You can also configure an implicit or explicit wait to handle delayed elements.
Q 73.) How do you retrieve the CSS value of an element in Selenium?
Use the getCssValue() method:
WebElement element = driver.findElement(By.id("elementID"));
String color = element.getCssValue("color");
System.out.println("CSS Color Value: " + color);
Q 74.) How do you handle AJAX calls in Selenium?Â
Use explicit waits to handle elements that are loaded asynchronously:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("ajaxElement")));
Q 75.) How do you work with browser cookies in Selenium?
1. Add a cookie:Â
Cookie cookie = new Cookie("name", "value");
driver.manage().addCookie(cookie);
2. Retrieve all cookies:
Set cookies = driver.manage().getCookies();
3. Delete a specific cookie:
driver.manage().deleteCookieNamed("cookieName");
Q 76.) How do you perform mouse hover in Selenium?Â
Use the Actions class:Â
Actions actions = new Actions(driver);
WebElement element = driver.findElement(By.id("hoverElement"));
actions.moveToElement(element).perform();
Q 77.) How do you handle a situation where an element is not clickable?
1. Use explicit wait to wait for clickability:
Automation testing is a software testing technique that uses specialised tools and scripts to run predefined test cases, compare the results to what was expected, and report the results.
The fundamental purpose of automated testing is to improve the efficiency, effectiveness, and coverage of the testing process by automating repetitive and time-consuming processes that would otherwise be completed manually
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("clickableElement")));
element.click();
2. Use Script click:
scriptExecutor js = (scriptExecutor) driver;
js.executeScript("arguments[0].click();", element);
Q78.) How do you retrieve an attribute value of a web element in Selenium?
Use the getAttribute() method:Â
WebElement element = driver.findElement(By.id("elementID"));
String attributeValue = element.getAttribute("placeholder");
System.out.println("Attribute Value: " + attributeValue);
Q 79.) How do you retrieve the position (x, y coordinates) of a web element in Selenium?
Use the getLocation() method:
WebElement element = driver.findElement(By.id("elementID"));
Point location = element.getLocation();
System.out.println("X Coordinate: " + location.getX());
System.out.println("Y Coordinate: " + location.getY());
Q80.) How do you check if a drop-down is multi-select in Selenium?Â
Use the isMultiple() method in the Select class:Â
WebElement dropdown = driver.findElement(By.id("dropdownID"));
Select select = new Select(dropdown);
if (select.isMultiple()==true) {
System.out.println("The dropdown is multi-select");
} else {
System.out.println("The dropdown is single-select");
}
Q81.) How do you get the size (height and width) of a web element in Selenium? Â
Use the getSize() method:
WebElement element = driver.findElement(By.id("elementID"));
Dimension size = element.getSize();
System.out.println("Width: " + size.getWidth());
System.out.println("Height: " + size.getHeight());
Q82.) How do you handle elements with dynamic IDs in Selenium?
Use dynamic XPath or CSS selectors:Â
- XPath with contains():
WebElement element = driver.findElement(By.xpath("//*[contains(@id, 'dynamicPart')]"));
- CSS selector with attribute starts-with:Â Â
WebElement element = driver.findElement(By.cssSelector("[id^='dynamicPrefix']"));
Q83.) How do you handle loading spinners or progress bars in Selenium?
Use explicit waits to wait until the spinner disappears:Â
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.invisibilityOfElementLocated(By.id("spinnerID")));
Conclusion:
In conclusion, by familiarizing yourself with these Interview Questions and Answers, you are better prepared to showcase your knowledge and skills to potential employers. Remember to practice, stay updated with the latest trends in Java with Selenium, and maintain a positive attitude throughout your interview process. With dedication and perseverance, you can ace any automation testing interview and secure your dream job.Â
Also read :
Consult Us