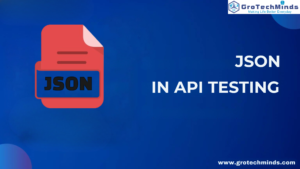
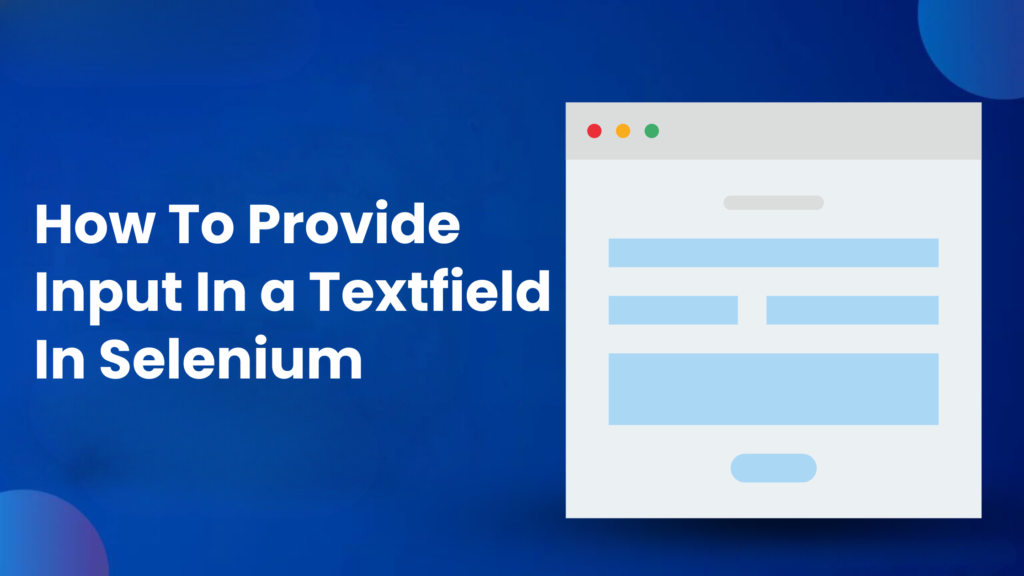
Different ways to provide input in a textfield in Selenium
In Automation testing of web applications one of the most commonly used functionality is providing input to text field.This feature is found in login page, registration page, product search page of e commerce website and in google search page. Selenium WebDriver is a key component of Selenium which facilitates programmatic user inputs to the text field ensuring that the application handles the user inputs efficiently. In this blog we will discuss about the different ways of providing input to text field.
Three different Ways of providing input to a text field
There are three ways of providing input to a text field in a web application which are as follows:
1.By using sendkeys method()
The steps involved in providing input to textfields using sendkeys() method are as follows:
- Launch the ChromeBrowser using ChromeDriver class by importing the class from “org.openqa.selenium.chrome” package.
- Launch the google.com webpage using get() method.
- Use find element method and name locator to locate the search text field
- Using sendkeys method type something in the search text field which is to be searched.
- Use virtually keys functionality keys.enter to execute the search actions.
Code Snippet:
package alternateWaysOfSendKey package alternateWaysOfSendKeys;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.chrome.ChromeDriver;
public class UsingSendKeys {
public static void main(String[] args) {
ChromeDriver driver=new ChromeDriver();
driver.get("https://www.google.com");
driver.manage().window().maximize();
driver.findElement(By.name("q")).sendKeys("India");
driver.findElement(By.name("q")).sendKeys(Keys.ENTER);
}
}
Screenshot:
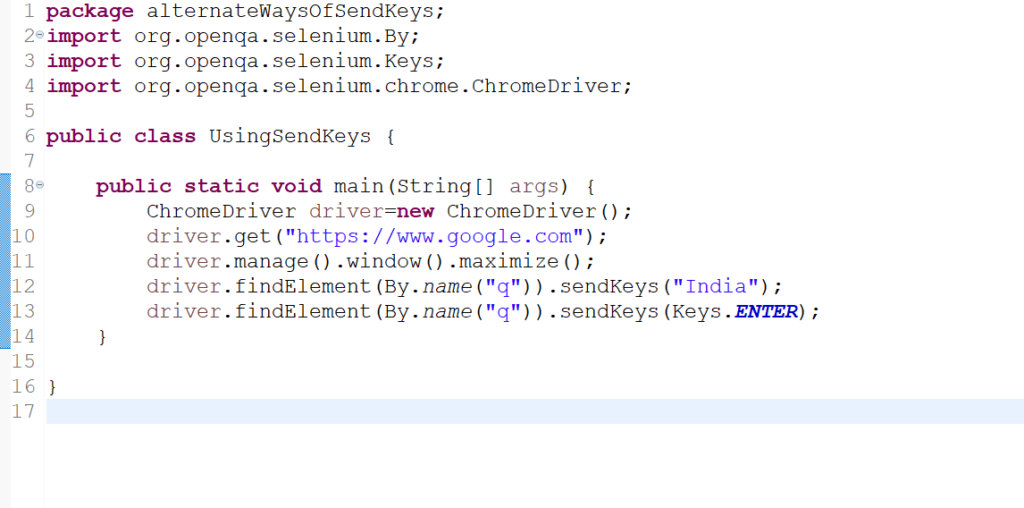
Output: The below output is displayed after providing “India” as input using sendkeys method.
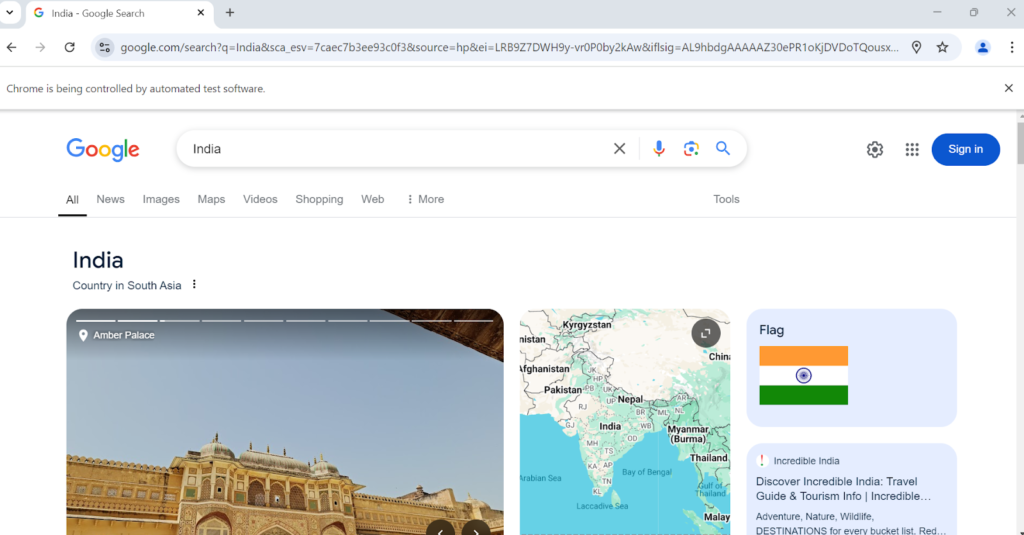
2.By using JavaScriptExecutor interface
There are two ways of using JavaScriptExecutor which are as follows:
a) With Web Element
The steps involved in providing input to textfields using JavaScriptExecutor with Web Element are as follows:
- Launch the ChromeBrowser using ChromeDriver class by importing the class from “org.openqa.selenium.chrome” package.
- Launch the google.com webpage using get() method.
- Use find element method and name locator to locate the search text field
- Upcast the variable of ChromeDriver to Java Script Executor interface
- Utilise the method called executescript() to perform action on the text field by typing something in it.
- Use Robot class and Virtual keys to execute the search operation.
Code Snippet:
package alternateWaysOfSendKeys;
import java.awt.AWTException;
import java.awt.Robot;
import java.awt.event.KeyEvent;
import org.openqa.selenium.By;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class UsingJavaScriptExecutorWithWebElement
{
public static void main(String[] args) throws AWTException {
ChromeDriver driver= new ChromeDriver();
driver.get("https://www.google.com");
WebElement e1= driver.findElement(By.name("q"));
JavascriptExecutor js= driver;
js.executeScript("arguments[0].value='Rama'",e1);
Robot e2= new Robot();
e2.keyPress(KeyEvent.VK_ENTER);
}
}
Screenshot:
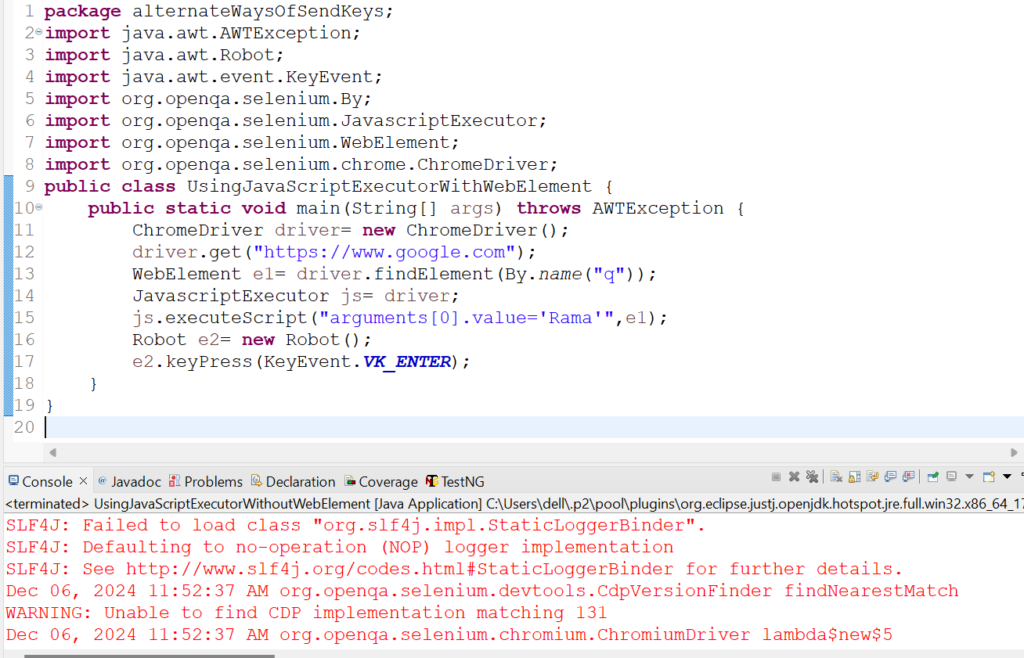
Output:The below output is displayed after providing “India” as input using JavaScriptExecutor with WebElement.
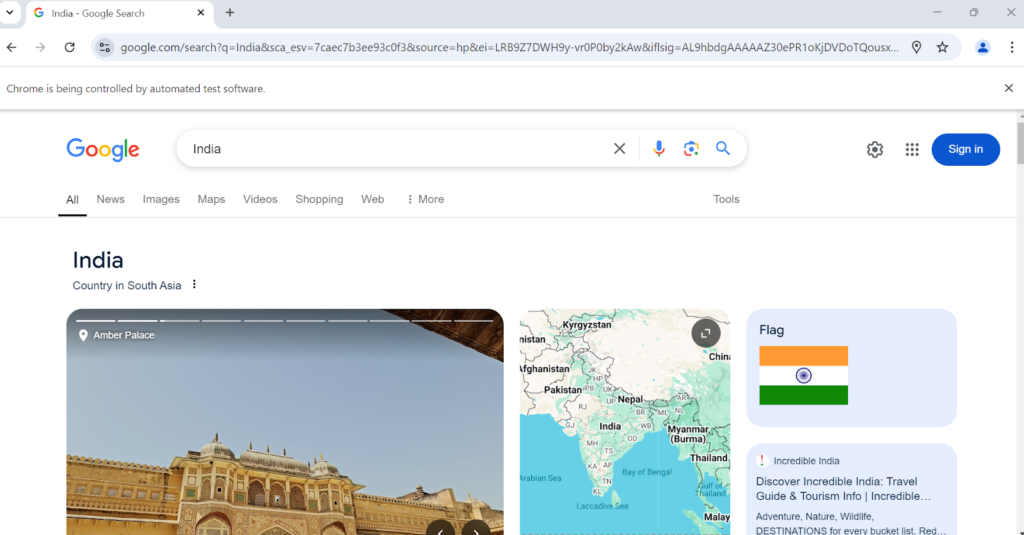
b) Without Web Element
The steps involved in providing input to textfields using JavaScriptExecutor without Web Element are as follows:
- Launch the ChromeBrowser using ChromeDriver class by importing the class from“org.openqa.selenium.chrome” package.
- Launch the google.com webpage using get() method.
- Upcast the variable of ChromeDriver to Java Script Executor interface.
- Utilise the method called executescript() to locate the text field by name and perform action on the text field by typing something in it.
- Use Robot class and Virtual keys to execute the search operation.
Code Snippet:
import java.awt.AWTException;
import java.awt.Robot;
import java.awt.event.KeyEvent;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.chrome.ChromeDriver;
public class UsingJavaScriptExecutorWithoutWebElement {
public static void main(String[] args) throws AWTException {
ChromeDriver driver= new ChromeDriver();
driver.get("https://www.google.com");
JavascriptExecutor js= driver;
js.executeScript("document.getElementsByName('q')[0].value='Rama'");
Robot e2= new Robot();
e2.keyPress(KeyEvent.VK_ENTER);
}
}
Screenshot:
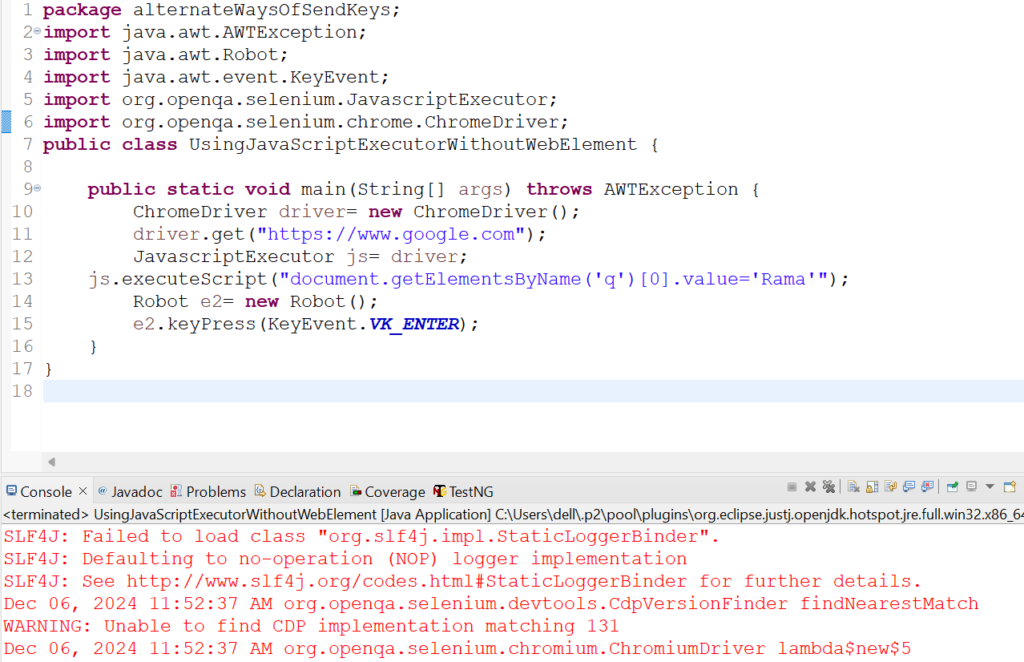
Output:The below output is displayed after providing “India” as input using JavaScriptExecutor without WebElement.
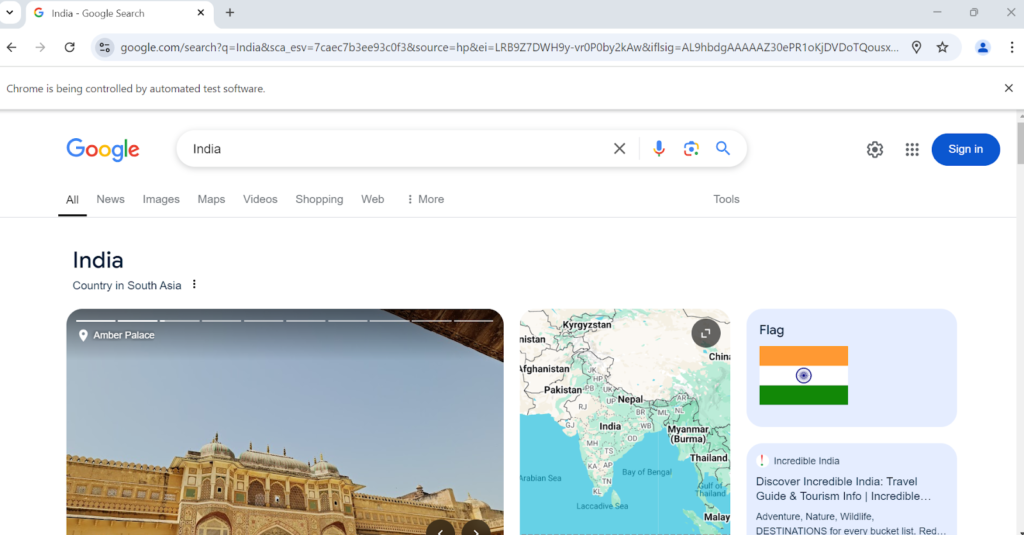
3. By using Actions class
The steps involved in providing input to textfields using Actions class are as follows:
- Launch the ChromeBrowser using ChromeDriver class by importing the class from “org.openqa.selenium.chrome” package.
- Launch the google.com webpage using get() method.
- Use find element method and name locator to locate the search text field
- Create Object of the Actions Class
- Inside the parameter of the sendkeys method of Actions class add the WebElement and input text of the text field component and perform the actions.
- Use Robot Class to execute the action of typing in the search text field.
Code Snippet:
package alternateWaysOfSendKeys;
import java.awt.AWTException;
import java.awt.Robot;
import java.awt.event.KeyEvent;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
public class UsingActionsClass {
public static void main(String[] args) throws AWTException {
ChromeDriver driver= new ChromeDriver();
driver.get("https://www.google.com");
driver.manage().window().maximize();
WebElement e1= driver.findElement(By.name("q"));
Actions a1= new Actions(driver);
a1.sendKeys(e1, "India").build().perform();
Robot e2= new Robot();
e2.keyPress(KeyEvent.VK_ENTER);
}
Screenshot:
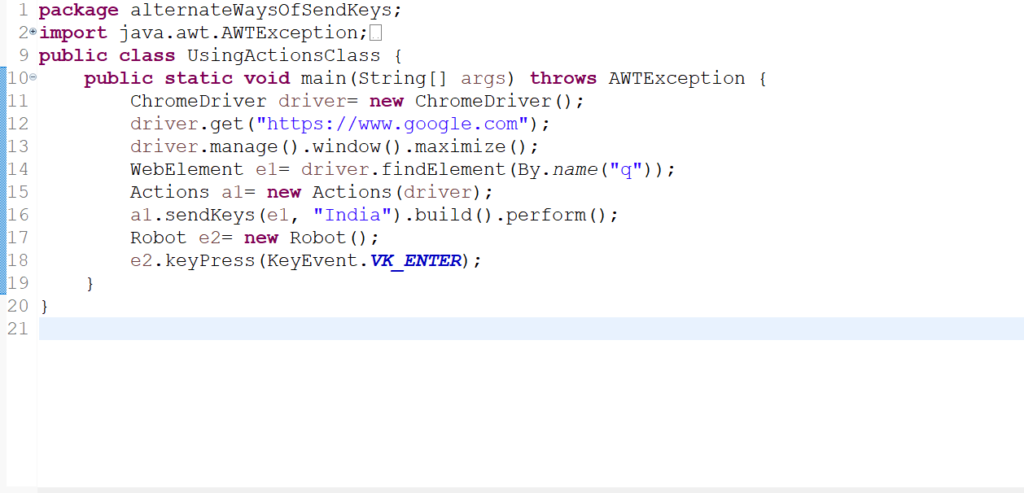
Output: The below output is displayed after providing “India” as input using Actions class with sendkeys method.
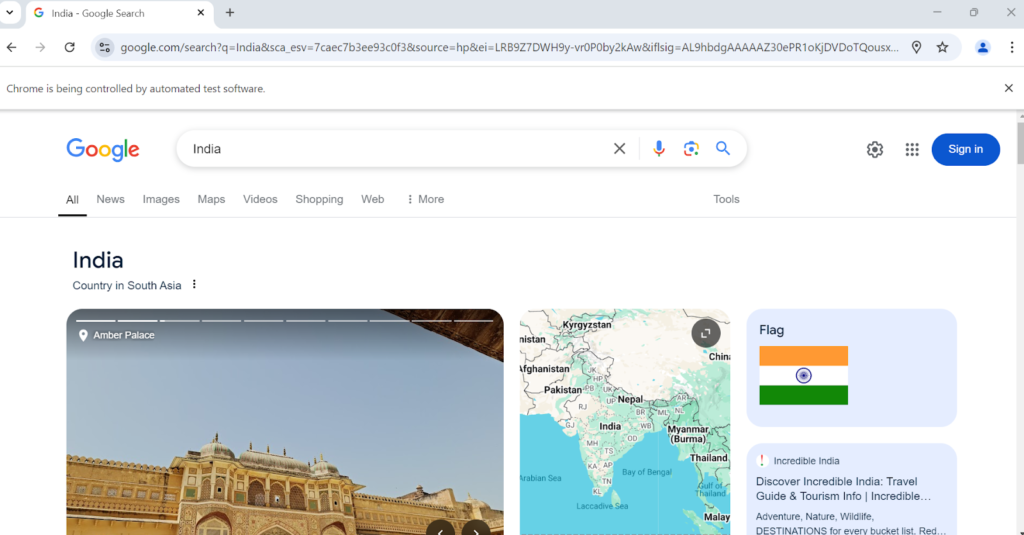
Conclusion
One of the key functionalities in web applications is user input in the text field. Selenium. Using sendkeys method, JavaScript Executor interface and Action class, testers can perform automation testing of user inputs into text field which is essential for validation of registration page, login page and search tabs of e commerce applications. Remember to practice, stay updated with the latest trends in Java with Selenium, and maintain a positive attitude throughout your interview process. Using locators like name, xpath we can detect the text fields in both static and dynamic webpages.
Also read :
Automation testing is a software testing technique that uses specialised tools and scripts to run predefined test cases, compare the results to what was expected, and report the results.
The fundamental purpose of automated testing is to improve the efficiency, effectiveness, and coverage of the testing process by automating repetitive and time-consuming processes that would otherwise be completed manually
Consult Us