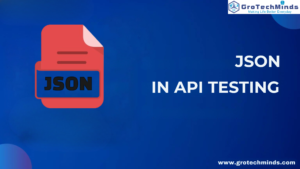
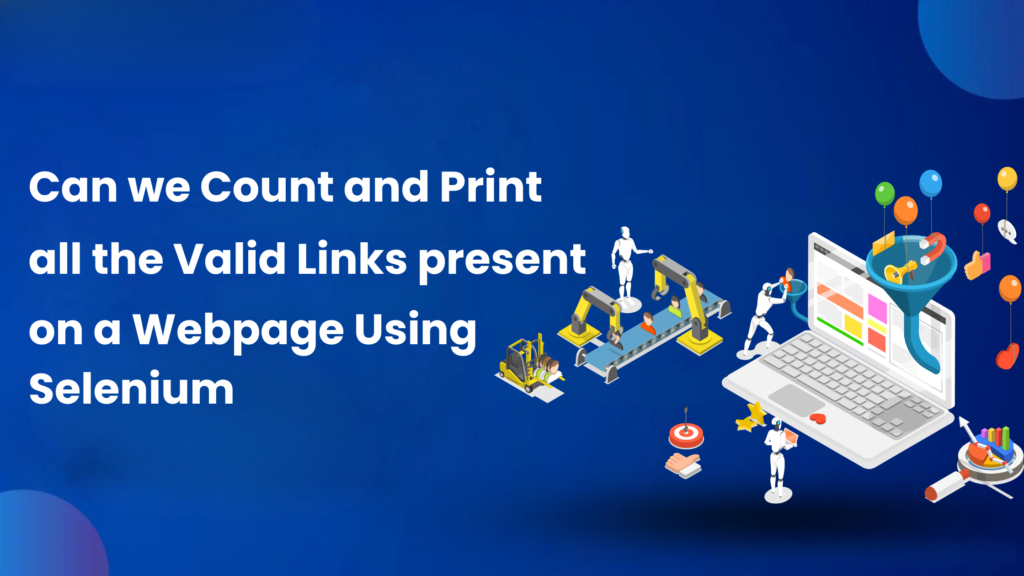
How to Count and Print all the Valid Links present on a Webpage Using Selenium?
Almost all web pages have clickable icons or links that take us to another webpage. For automation of those web pages for testing, it is crucial to validate the links present on that webpage. If a link is not redirecting the end user to the desired page, it may have a serious impact on business goals. Let us go through this Java program that utilises Selenium to count the total number of links, identify those links that are empty or null, and also identify the number of valid links. Here, we are going to find the links from the homepage of Amazon India (“https://www.amazon.in/”).
The program is structured as follows:
- Setting up the WebDriver: We Initialise the ‘WebDriver’ using ‘ChromeDriver();’ to open an empty Chrome browser.
- Navigation to the desired Webpage: We navigate to the homepage of Amazon India from the empty browser opened in the previous step.
- Finding All Links: We, then locate all elements that have the tag name ‘<a>’ on the homepage of Amazon India.
- Segregating the found Links: We first count all links present, then identify and count links that are empty or null, and count all the valid links separately.
- Printing the Output in the Console: In the output console, we print all the valid links, the total count of all links, the count of valid links, and the count of those links that are either null or empty.
- Closing the Browser: We then Quit the WebDriver session created for finding the links.
The step-by-step building of the program for counting the links is as follows:
- Setting up the WebDriver: We Initialize the ‘WebDriver’ using ‘ChromeDriver();’ to open an empty Chrome browser. We have given an implicit wait of 10 seconds here. When we navigate to one webpage from another, implicit wait gives the necessary time for the ‘WebElement’ in the webpage to load completely.
WebDriver driver=new ChromeDriver();
driver.manage().timeouts().implicitlyWait(Duration.ofMillis(10000));
2. Navigation to the desired Webpage: We navigate to the homepage of Amazon India from the empty browser opened in the previous step. We are navigating using the ‘get’ method of the ‘WebDriver’ Interface.
driver.get("https://www.amazon.in/");
3. Finding All Links: We, then locate all elements that has the tag name ‘<a>’ on the homepage of Amazon India. We can use either of these methods(using tagName locator or using XPath locator) to find all the ‘WebElement’ that has ‘<a>’ as its tag name.
//method 1
List tagname_a=driver.findElements(By.tagName("a"));
//method 2
List tagname_a=driver.findElements(By.xpath("//a"));
4. Segregating the found Links: We first count all links present, then identify and count links that are empty or null, and count all the valid links separately.
Using the method called ‘size()’ from the ‘List’ Interface, we calculate the count of all the links present in the homepage of Amazon India and store it in a variable of int data type. We initialize and declare two more variables for calculating the count of links that are either empty or null and for calculating the count of all valid links so that we can update it inside the for loop.
int Count_of_All_Links = tagname_a.size();
int Count_of_Empty_or_Null = 0;
int Count_of_Valid_Links = 0;
With the help of a for loop, we iterate the loop as many times as the value of the variable ‘Count_of_All_Links’ incrementing by one count for every iteration. Inside the for loop, we find individual ‘WebElement’ one by one using the ‘get’ method of the ‘List’ Interface.
For every web element we find in that particular iteration, we store the link’s value in a ‘String’ variable. To get the attribute values of all the ‘href’ attribute name, we use the ‘getAttribute’ method of the ‘WebElement’ Interface.
Then we check if that String variable’s(‘href’) value is either null or is empty (using the ‘isEmpty’ method of the ‘String’ class) and increment the value of the variable ‘Count_of_Empty_or_Null’ by one in the if block. Also, if it is a valid link, we increment the value of ‘Count_of_Valid_Links’ by one and print the attribute value of ‘href’ in the output console on every iteration.
for (int i = 0; i < Count_of_All_Links; i++) {
WebElement Individual_Links = tagname_a.get(i);
String href = Individual_Links.getAttribute("href");
if (href == null || href.isEmpty()) {
Count_of_Empty_or_Null++;
} else {
Count_of_Valid_Links++;
System.out.println("There is a valid link present, and it is : " + href);
}
}
5. Printing the Output in the Console: In the output console, we print the total count of all links, valid links, and those links which are either null or empty. Since, we have updated the count of the variables inside the for loop, using all the iterations performed, we can print the count in the output console as shown below. We use ‘println’ method of the ‘PrintStream’ class to perform this action.
System.out.println("The number of all links that are either valid or empty or null = "+Count_of_All_Links);
System.out.println("The number of links that are either empty or null = "+Count_of_Empty_or_Null);
System.out.println("The number of Valid links present = "+Count_of_Valid_Links);
6. Closing the Browser: We then Quit the WebDriver session created for finding the links. Using the ‘quit’ method of the ‘WebDriver’ Interface, we close the browser along with all the other tabs opened in the browser.
driver.quit();
Complete Program with Output
The complete program is as given below:
package selenium;
import java.time.Duration;
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class Count_of_Links {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
driver.manage().timeouts().implicitlyWait(Duration.ofMillis(10000));
driver.get("https://www.amazon.in/");
// method 1
List tagname_a = driver.findElements(By.tagName("a"));
// method 2
// List tagname_a=driver.findElements(By.xpath("//a"));
int Count_of_All_Links = tagname_a.size();
int Count_of_Empty_or_Null = 0;
int Count_of_Valid_Links = 0;
for (int i = 0; i < Count_of_All_Links; i++) {
WebElement Individual_Links = tagname_a.get(i);
String href = Individual_Links.getAttribute("href");
if (href == null || href.isEmpty()) {
Count_of_Empty_or_Null++;
} else {
Count_of_Valid_Links++;
System.out.println("There is a valid link present, and it is : " + href);
}
}
System.out.println("The number of all links that are either valid or empty or null = " + Count_of_All_Links);
System.out.println("The number of links that are either empty or null = " + Count_of_Empty_or_Null);
System.out.println("The number of Valid links present = " + Count_of_Valid_Links);
driver.quit();
}
}
The output of this program is as follows:
There is a valid link present, and it is : https://www.amazon.in/s/?_encoding=UTF8&i=fashion&bbn=16491484031&rh=n%3A16491484031%2Cp_89%3AAmazon%20Brand%20-%20Eden%20%26%20Ivy%7CAmazon%20Brand%20-%20House%20%26%20Shields%7CAmazon%20Brand%20-%20INKAST%7CAmazon%20Brand%20-%20Jam%20%26%20Honey%7CAmazon%20Brand%20-%20Myx%7CAmazon%20Brand%20
//<all the 339 valid links are printed one by one>
There is a valid link present, and it is : https://www.amazon.in/gp/help/customer/display.html?nodeId=202075050&ref_=footer_iba
The number of all links that are either valid or empty or null = 348
The number of links that are either empty or null = 9
The number of Valid links present = 339
We have understood how to calculate the count of all the links present in a website, classify them by valid or invalid, segregate them by either null or empty, and also print all the valid links in the output console of any IDE. Remember to practise, stay updated with the latest trends in Automation Software Testing Course,and maintain a positive attitude throughout your interview process. This program is very useful in automation testing to ensure that all clickable links have a valid URL.
Also read:
Consult Us