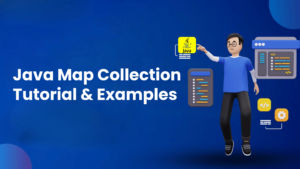
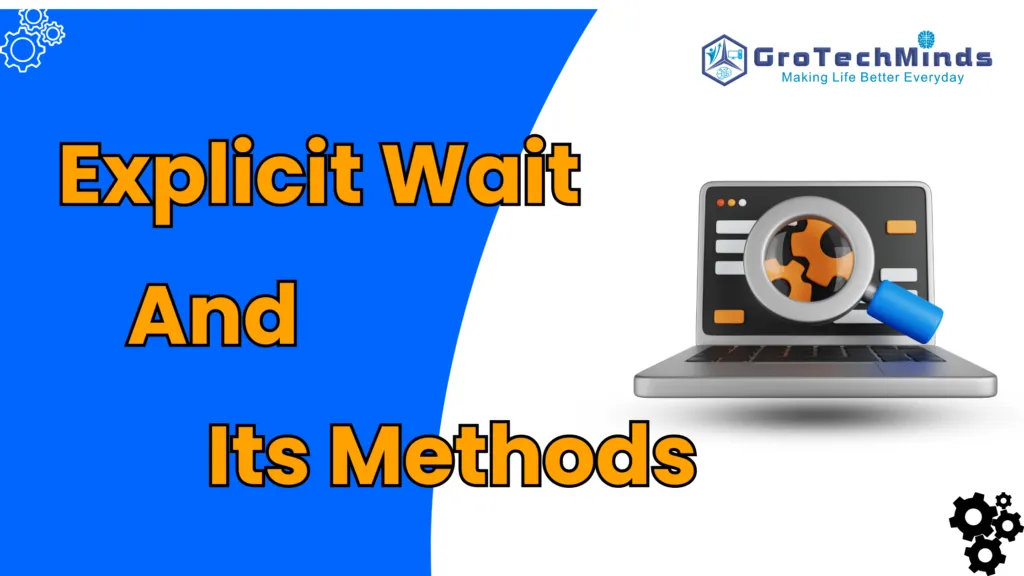
Explicit Wait and Its Methods
Selenium is the most popular and widely used web automation tool for developers and testers.Automation testing using Selenium reduces the time and effort of testers in testing software web applications which was consumed through manual testing. The Unique features of Selenium are its open source, free availability , easy to use, constant updates and overcoming drawbacks in every updates. The components of Selenium like Selenium Grid, Selenium WebDriver and Selenium IDE helps in automation of web application through interaction with various components of the web application.
Selenium is configured with various programming languages like Java,Python and Ruby for performing automation testing of websites and web applications through writing of automation scripts in those programming languages. Selenium is also compatible with various browsers like chrome firefox for performing parallel testing and cross browser testing and with different operating systems like windows and Mac Os for performing cross platform testing. But sometimes even after writing correct programming code to run automation test scripts we do not get the correct output but instead we get exception. The reason for this issue is that the Selenium speed is more than the internet speed. To overcome this drawback we use some wait technique for smooth execution of automation scripts. Explicit wait is one such technique where we give a specific time duration and condition and we get the correct output once the given conditions are satisfied within the specified time interval.
In Selenium, Automation testing is performed on various components like textfield, text area field , radio buttons, checkboxes and buttons and navigation to various links and websites. Those components might not have fully loaded as a result of which even after writing correct test script we do not get the correct output. This is because the component might not be getting time to load fully and in order to provide that time we use explicit wait.
In Explicit wait we provide a time duration and conditions in the test script. The time duration is provided with the help of WebDriverWait class, reference variable of WebDriverWait class, reference variable of WebDriver class and Duration as final class. Using reference variable of WebDriver wait we specify a condition and different methods associated with condition. By specifying condition and time duration we ensure that the WebDriver waits for the specific period of time until given conditions are satisfied so that correct output of test script will be executed. Explicit wait has to be given just before or after writing the code or syntax of locating a particular element. Explicit wait once written is not applicable for the entire test script and it is only applicable for specific syntax or code of locating a specific element.
Due to the usage of explicit wait, web element gets enough time to properly load as a result of which test scripts interacts with the element and gives correct output. There are several advantages of Explicit wait.
Due to asynchronous operators the web pages or websites take longer time to load or do not load properly. So Explicit wait ensures that the web page loads properly before any actions are performed on the web page using test automation script.
There are many webpages or website which take longer time to load. As a result of which inspite of writing correct test scripts we do not get the required result instead we get run time exception. Explicit wait helps to overcome this drawbacks thus ensuring that the web page loads properly before any action is performed on the web page. Once the web page is fully loaded we get the required results after running the same automation test scripts.
In many situations due to poor network connectivity web pages take longer to load as a result of which test script try to perform action on the web page and we get the run time exception. So explicit wait provides the time duration for the page to load completely and once loaded test automation script perform actions on that page and produce the required result.
Sometimes even after writing correct automation scripts to automate the web element we get the run time exception if some specific conditions are not satisfied.
So in explicit wait apart from time duration we provide some conditions on the web page. So the test script will perform actions on those elements until the specific condition is satisfied and once the conditions are satisfied we get the required output.
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(20));
wait.until(ExpectedConditions);
where WebDriverWait is a class , Duration is a final class and wait is reference variable of WebDriverWait class
The methods of explicit wait ensures that the webpages or websites loads properly and certain conditions are satisfied before actions are performed on them through test scripts.
The following are the methods of explicit wait alongwith their code snippets
package pokerr;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWaitAlertIsPresent {
public static void main(String[] args) {
ChromeDriver driver = new ChromeDriver();
driver.get("https://grotechminds.com/javascript-popup/");
driver.manage().window().maximize();
driver.findElement(By.xpath("//button[.='Click ']")).click();
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(10));
wait.until(ExpectedConditions.alertIsPresent());
driver.switchTo().alert().accept();
}
}
package pokerr;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWait {
public static void main(String[] args) {
ChromeDriver driver = new ChromeDriver();
driver.get("https://www.google.com/");
driver.manage().window().maximize();
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(20));
wait.until(ExpectedConditions.titleContains("Goo"));
wait.until(ExpectedConditions.titleIs("Google"));
WebElement search= driver.findElement(By.name("q"));
search.sendKeys("India");
search.sendKeys(Keys.ENTER);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.xpath("//h3[.='India']"))).click();
}
}
package pokerr;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWait {
public static void main(String[] args) {
ChromeDriver driver = new ChromeDriver();
driver.get("https://www.google.com/");
driver.manage().window().maximize();
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(20));
wait.until(ExpectedConditions.titleContains("Goo"));
wait.until(ExpectedConditions.titleIs("Google"));
WebElement search= driver.findElement(By.name("q"));
search.sendKeys("India");
search.sendKeys(Keys.ENTER);
wait.until(ExpectedConditions.elementToBeClickable(By.xpath("//h3[.='India']"))).click();
}
}
package pokerr;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWait {
public static void main(String[] args) {
ChromeDriver driver = new ChromeDriver();
driver.get("https://www.google.com/");
driver.manage().window().maximize();
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(20));
wait.until(ExpectedConditions.titleContains("Goo"));
wait.until(ExpectedConditions.titleIs("Google"));
WebElement search= driver.findElement(By.name("q"));
search.sendKeys("India");
search.sendKeys(Keys.ENTER);
wait.until(ExpectedConditions.elementToBeClickable(By.xpath("//h3[.='India']"))).click();
}
}
package pokerr;
import java.time.Duration;
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitwaitPresenceofallelements {
public static void main(String[] args) {
ChromeDriver g1= new ChromeDriver();
g1.get("https://www.google.com");
g1.manage().window().maximize();
By searchfieldgoogle = By.tagName("a");
WebDriverWait wait = new WebDriverWait(g1,Duration.ofSeconds(10));
List presence = wait.until(
ExpectedConditions
.presenceOfAllElementsLocatedBy
(searchfieldgoogle));
//get the number of authors found
int presentcount = presence.size();
System.out.println(presentcount);
}
}
package pokerr;
import java.time.Duration;
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitwaitPresenceofallelements {
public static void main(String[] args) {
ChromeDriver g1= new ChromeDriver();
g1.get("https://www.google.com");
g1.manage().window().maximize();
By searchfieldgoogle = By.tagName("a");
WebDriverWait wait = new WebDriverWait(g1,Duration.ofSeconds(10));
List presence = wait.until(
ExpectedConditions
.presenceOfAllElementsLocatedBy
(searchfieldgoogle));
//get the number of authors found
int presentcount = presence.size();
System.out.println(presentcount);
}
}
package pokerr;
import static org.testng.Assert.assertTrue;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitwaitSelectionElement {
public static void main(String[] args) {
ChromeDriver c1= new ChromeDriver();
c1.get("file:///C:/Users/dell/Downloads/learningHTML1.html");
WebElement a= c1.findElement(By.id("123"));
boolean boy = a.isSelected();
System.out.println(boy);
if(a.isSelected()==false)
{
a.click();
}
WebDriverWait wait = new WebDriverWait(c1,Duration.ofSeconds(10));
assertTrue(wait.until(ExpectedConditions.
elementToBeSelected(By.id("123"))));
}
}
import java.time.Duration;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWaitUrlContains {
public static void main(String[] args) {
ChromeDriver driver = new ChromeDriver();
driver.get("http://www.amazon.in");
driver.manage().window().maximize();
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(10));
boolean isUrlCorrect = wait.until (
ExpectedConditions .
urlContains("in"));
System.out.println(isUrlCorrect);
}
}
package pokerr;
import java.time.Duration;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWaitUrlContains {
public static void main(String[] args) {
ChromeDriver driver = new ChromeDriver();
driver.get("http://www.amazon.in");
driver.manage().window().maximize();
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(10));
boolean isUrlCorrect2 = wait.until(ExpectedConditions.urlMatches("amazon"));
System.out.println(isUrlCorrect2);
}
}
package pokerr;
import java.time.Duration;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWaitUrlContains {
public static void main(String[] args) {
ChromeDriver driver = new ChromeDriver();
driver.get("http://www.amazon.in");
driver.manage().window().maximize();
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(10));
boolean isUrlCorrect3= wait.until(ExpectedConditions.urlToBe("https://www.amazon.in/"));
System.out.println(isUrlCorrect3);
}
}
package automation;
import java.time.Duration;
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWaitVisibilityOfAllElementsLocated {
public static void main(String[] args) {
ChromeDriver g1= new ChromeDriver();
g1.get("https://www.flipkart.com");
g1.manage().window().maximize();
g1.findElement(By.name("q")).sendKeys("shoes");
By allelementsflipkart = By.xpath("//form[@class='_2rslOn header-form-search OpXDaO']/ul/li");
WebDriverWait wait = new WebDriverWait(g1,Duration.ofSeconds(10));
List elements = wait.until
(ExpectedConditions.
visibilityOfAllElementsLocatedBy
( allelementsflipkart));
int elementCount = elements.size();
System.out.println(elementCount);
}
}
package pokerr;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class InvisibilityExplicitwait {
public static void main(String[] args) {
ChromeDriver driver = new ChromeDriver();
driver.get("https://www.google.com/");
driver.manage().window().maximize();
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(20));
wait.until(ExpectedConditions.titleContains("Goo"));
wait.until(ExpectedConditions.titleIs("Google"));
WebElement search= driver.findElement(By.name("q"));
search.sendKeys("India");
search.sendKeys(Keys.ENTER);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.xpath("//h3[.='India']"))).click();
wait.until(ExpectedConditions.invisibilityOfElementLocated(By.xpath("//h3[.='India']")));
}}
package pokerr;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class InvisibilityExplicitwait {
public static void main(String[] args) {
ChromeDriver driver = new ChromeDriver();
driver.get("https://www.google.com/");
driver.manage().window().maximize();
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(20));
wait.until(ExpectedConditions.titleContains("Goo"));
wait.until(ExpectedConditions.titleIs("Google"));
WebElement search= driver.findElement(By.name("q"));
search.sendKeys("India");
search.sendKeys(Keys.ENTER);
wait.until(ExpectedConditions.visibilityOfElementLocated(By.xpath("//h3[.='India']"))).click();
// wait.until(ExpectedConditions.invisibilityOfElementLocated(By.xpath("//h3[.='India']")));
wait.until(ExpectedConditions.invisibilityOfElementWithText(By.xpath("//h3[.='India']"), "India"));
}}
package automation;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWaitTextTobePresent {
public static void main(String[] args) {
ChromeDriver c1= new ChromeDriver();
c1.get("http://www.amazon.in");
c1.manage().window().maximize();
WebDriverWait wait = new WebDriverWait(c1,Duration.ofSeconds(10));
boolean isTextPresent = wait.until(ExpectedConditions.textToBePresentInElement(c1.findElement(By.linkText("Best Sellers")), "Best"));
if (isTextPresent) {
System.out.println("Text is present in the element.");
} else {
System.out.println("Text is not present in the element.");
}
}
}
package automation;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWaitTextValueTobePresent {
public static void main(String[] args) {
ChromeDriver c1= new ChromeDriver();
c1.get("https://grotechminds.com/registration/");
c1.manage().window().maximize();
WebDriverWait wait = new WebDriverWait(c1,Duration.ofSeconds(10));
boolean isTextValuePresent = wait.until(ExpectedConditions.textToBePresentInElementValue(c1.findElement(By.name("Submit")), "Submit"));
if (isTextValuePresent) {
System.out.println("Text value is present in the element.");
} else {
System.out.println("Text value is not present in the element.");
}
}
}
package pokerr;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWaitTitleContains {
public static void main(String[] args) {
ChromeDriver driver = new ChromeDriver();
driver.get("https://www.google.com/");
driver.manage().window().maximize();
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(20));
wait.until(ExpectedConditions.titleContains("Goo"));
WebElement search= driver.findElement(By.name("q"));
search.sendKeys("India");
search.sendKeys(Keys.ENTER);
}
}
package pokerr;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWaitTitleIs {
public static void main(String[] args) {
ChromeDriver driver = new ChromeDriver();
driver.get("https://www.google.com/");
driver.manage().window().maximize();
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(20));
wait.until(ExpectedConditions.titleIs("Google"));
WebElement search= driver.findElement(By.name("q"));
search.sendKeys("India");
search.sendKeys(Keys.ENTER);
}
}
package pokerr;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWait {
public static void main(String[] args) {
ChromeDriver driver = new ChromeDriver();
driver.get("https://www.google.com/");
driver.manage().window().maximize();
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(20));
WebElement search= driver.findElement(By.name("q"));
search.sendKeys("India");
search.sendKeys(Keys.ENTER);
Boolean s1= wait.until(ExpectedConditions.stalenessOf(search));
if(s1==true)
{
System.out.println("Element will perform action");
}
else
{
System.out.println("Element will not perform action");
}
}
}
package pokerr;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class IFrame {
public static void main(String[] args) {
ChromeDriver driver = new ChromeDriver();
driver.get("file:///C:/Users/dell/Downloads/iframework.html");
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(20));
wait.until(ExpectedConditions.frameToBeAvailableAndSwitchToIt(By.id("formIframe")));
driver.findElement(By.id("name")).sendKeys("ee");
driver.findElement(By.xpath("//button[.='Submit']")).click();
}
}
Automation Testing with Selenium test scripts sometimes do not produce correct results after performing actions on some web pages or websites due to factors like web pages not getting fully loaded and some specific conditions not being satisfied. So explicit wait is used to ensure that the webpages load completely and some conditions are satisfied before any actions are performed on them using test scripts. Explicit wait also improves the accuracy and efficiency of test scripts.
Consult Us