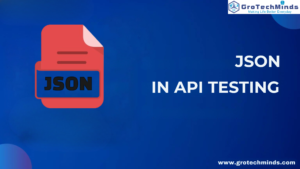
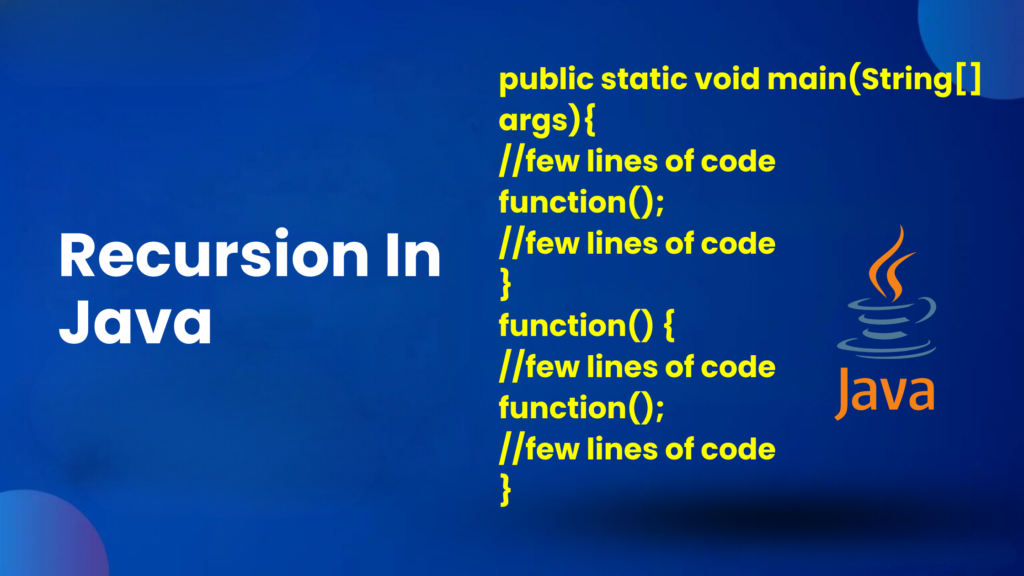
Recursion in Java
Recursion in java refers to the process where the method calls itself again and again. A method which calls itself is known as recursive method. Using Recursion concept various complex problems can be solved easily.A Recursion function consists of two parts
- Base case: This is the situation where the method stops calling itself thus preventing an infinite for loop.
- Recursive case: Here the method calls itself with a proper parameter thus moving towards the base case.
Syntax of Recursion:
methodName()
{
// any code to be executed
methodName()// calling of the same method
}
Examples of Recursion
The following are the examples of usage of Recursion in Java
1.Solving Factorial Problem using recursion
Code Snippet:
package automation;
class FactorialProblem {
// recursive method
int factorial(int number)
{
int result;
if (number == 1)
return 1;
result = factorial(number - 1) * number;
return result;
}
}
public class RecursiveMethod {
public static void main(String[] args) {
FactorialProblem f = new FactorialProblem();
System.out.println("Factorial of 3 is "
+ f.factorial(3));
System.out.println("Factorial of 4 is "
+ f.factorial(4));
System.out.println("Factorial of 5 is "
+ f.factorial(5));
}
}
Output:
Factorial of 3 is 6
Factorial of 4 is 24
Factorial of 5 is 120
Screenshot:

2. Solving Fibonacci series using recursion
Code Snippet:
package automation;
public class RecursiveMethod {
static int Fib(int a)
{
if (a == 0 || a == 1)
return a;
return Fib(a - 1) + Fib(a - 2);
}
public static void main(String[] args) {
// Fibonacci of 3
System.out.println("Fibonacci of " + 3 + " is "
+ Fib(3));
// Fibonacci of 4
System.out.println("Fibonacci of " + 4 + " is "
+ Fib(4));
// Fibonacci of 5
System.out.println("Fibonacci of " + 5 + " is "
+ Fib(5));
}
}
Output:
Fibonacci of 3 is 2
Fibonacci of 4 is 3
Fibonacci of 5 is 5
Screenshot:

3. Displaying numbers from 1 to 10
Code Snippet:
package automation;
public class RecursiveMethod {
static int count=1;
static void number(){
if(count<=10){
System.out.println(count);
count++;
number();
}
}
public static void main(String[] args) {
number();
}
}
Output:
1
2
3
4
5
6
7
8
9
10
Screenshot:

Advantages of Recursion
The various advantages of Recursion are as follows
- Using Recursion concepts codes can be written in a simple and clean way
- Helps to solve complex problems like tree traversal.
Disadvantages of Recursion
- Recursive codes require lot of space than any iterative codes as all the methods will be present in stack memory.
- It has more time requirements due to calling of methods.
Conclusion
Recursion is a very powerful programming process in java where a method calls itself. This helps in solving many complex problems like tree traversal, calculation of factorial and Fibonacci series. Remember to practice, stay updated with the latest trends in Java with Selenium, and maintain a positive attitude throughout your interview process.This technique is used to simplify solutions to problems that have recursive structure.
Also read :
Consult Us