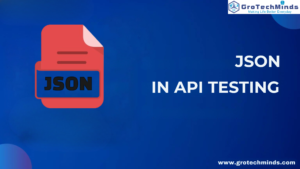

Handling Cookies in Selenium
What is a Cookie?
A Cookie refers to a small information that is sent by a website and stored in the local system.It helps users in saving their browsing history and other important informations.Many websites use cookies in the form of a popup where it is asks end users to give permission to website to run cookies.Once the user allows to run the cookies the website gets loaded fully. Cookie ensures that the webpage recognises the end user before allowing user access. In Selenium cookies are handled using WebDriver interface and various Selenium commands.
Selenium Commands for Handling Cookies
Below are the various Selenium Commands for handling cookies
1. getCookies() method: This method is used to get all the cookies of the website that is currently browsed.
driver().manage().getCookies();
Code Snippet:
Below is the Code Snippet of getCookies() method.Here we are printing getCookies() method in order to display the cookies in output console.
package cookiesGeneration;
import java.time.Duration;
import org.openqa.selenium.chrome.ChromeDriver;
public class CookiesGeneration {
public static void main(String[] args) {
ChromeDriver driver= new ChromeDriver();
driver.get("https://www.amazon.in/");
driver.navigate().refresh();
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(5));
System.out.println(driver.manage().getCookies());
}
}
Screenshot:
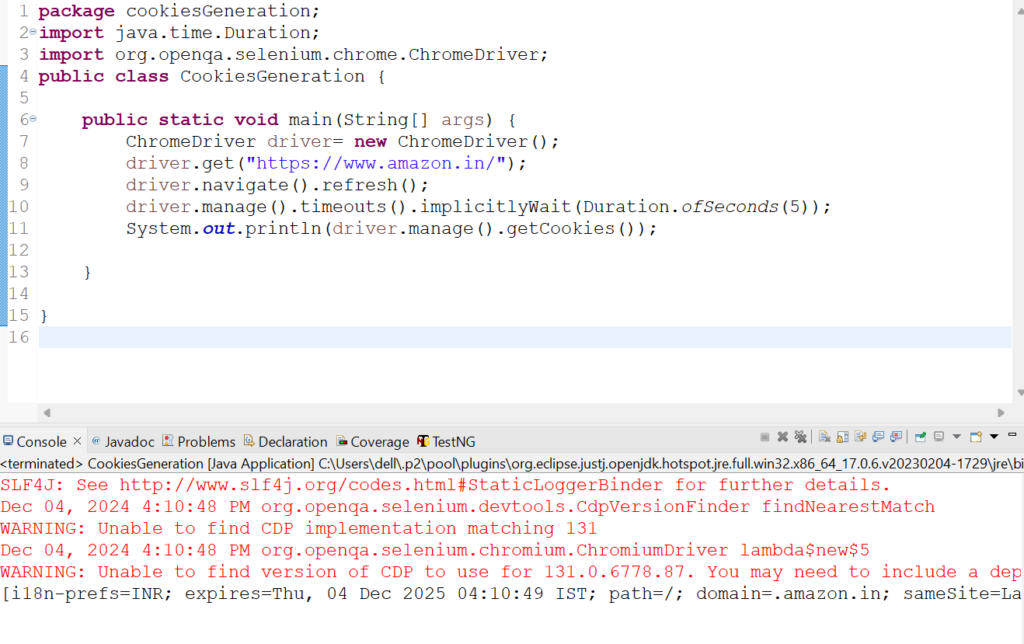
2. addCookie(Cookie cookie) method: This method is used to add a new cookie to the website that is currently browsed.
driver.manage().addCookie(new Cookie(“key”,“value”));
Code Snippet:
Below is the code Snippet of addCookie(Cookie,cookie) method. We first create the instance of Cookie class and then we call its parametrized constructor and finally we add parameters to the constructor in the form of key and value pairs. Finally we use getCookies() method to display all the cookies in the output console including the recently added Cookies using addCookie(Cookie cookie) method.
package cookiesGeneration;
import java.time.Duration;
import org.openqa.selenium.Cookie;
import org.openqa.selenium.chrome.ChromeDriver;
public class CookiesGeneration {
public static void main(String[] args) {
ChromeDriver driver= new ChromeDriver();
driver.get("https://www.amazon.in/");
driver.navigate().refresh();
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(5));
Cookie c1= new Cookie("q", "shoe");
driver.manage().addCookie(c1);
System.out.println(driver.manage().getCookies());
}
}
Screenshot:

3.deleteAllCookies() method: This method is used to delete all the cookies from the currently browsed website.
driver.manage().deleteAllCookies();
Code Snippet:
Below is the code snippet of deleteAllCookies() method.First we get all the cookies and print it in output console using getCookies() method then we use deleteAllCookies() method to delete the cookies.
package cookiesGeneration;
import java.time.Duration;
import org.openqa.selenium.chrome.ChromeDriver;
public class CookiesGeneration {
public static void main(String[] args) {
ChromeDriver driver= new ChromeDriver();
driver.get("https://www.amazon.in/");
driver.navigate().refresh();
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(5));
System.out.println(driver.manage().getCookies());
driver.manage().deleteAllCookies();
}
}
}
}
Screenshot:

4. deleteCookieNamed(String name)- This method is used to delete a specific cookie from all the cookies of the currently browsed website.
driver().manage().deleteCookieNamed(String name);
Code Snippet:
Below is the code snippet of deleteCookieNamed(String name) method.We first add cookie in form of key and value pairs using addCookie(Cookie cookie) method through instance of Cookie class then we use getCookie() method to display all cookies and finally we name a specific cookie to be deleted using deleteCookieName(String name) method.
package cookiesGeneration;
import java.time.Duration;
import org.openqa.selenium.Cookie;
import org.openqa.selenium.chrome.ChromeDriver;
public class CookiesGeneration {
public static void main(String[] args) {
ChromeDriver driver= new ChromeDriver();
driver.get("https://www.amazon.in/");
driver.navigate().refresh();
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(5));
Cookie c1= new Cookie("age","91");
driver.manage().addCookie(c1);
System.out.println(driver.manage().getCookies());
driver.manage().deleteCookieNamed("age");
System.out.println(driver.manage().getCookies());
}
}
Screenshot:
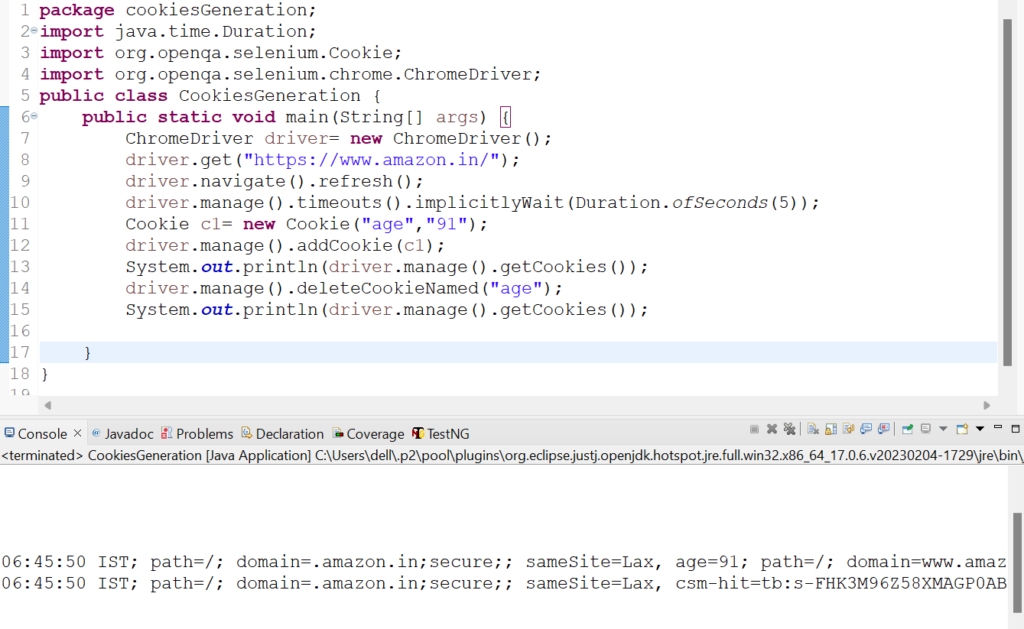
5.getCookieNamed(String name): This method is used to get the specific cookie among all cookies of the currently browsed website.
driver.manage().getCookieNamed(String name);
Code Snippet:
Below is the code snippet of getCookieNamed(String name) method.We first add cookie in form of key and value pairs using addCookie(Cookie cookie) method through instance of Cookie class then we use getCookie() method to display all cookies and finally we name a specific cookie to be displayed using getCookieNamed(String name) method.
package cookiesGeneration;
import java.time.Duration;
import org.openqa.selenium.Cookie;
import org.openqa.selenium.chrome.ChromeDriver;
public class CookiesGeneration {
public static void main(String[] args) {
ChromeDriver driver= new ChromeDriver();
driver.get("https://www.amazon.in/");
driver.navigate().refresh();
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(5));
Cookie c1= new Cookie("age","91");
driver.manage().addCookie(c1);
System.out.println(driver.manage().getCookies());
System.out.println(driver.manage().getCookieNamed("age"));
}
}
Screenshot:
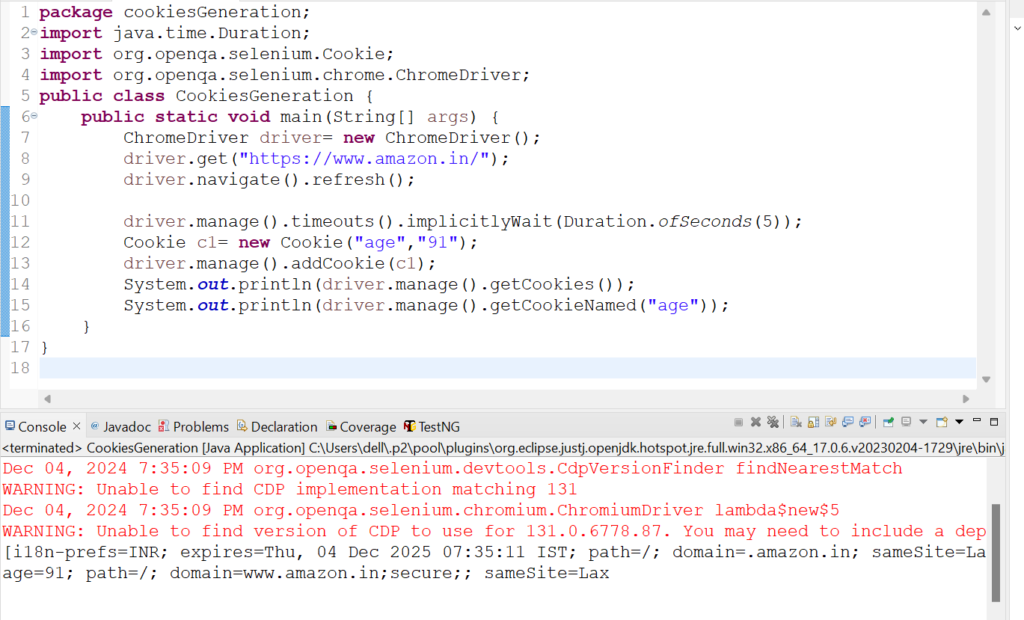
Importance of handling Cookie
There are various reasons for handling Cookies in selenium which are as follows:
- Efficient Testing: Handling of cookies ensures that the entire testing process is smooth and effective thus minimizing the risk of defects.
- Reducion in time of test execution: Saving of cookies in the local system reduces time for test execution especially when multiple scenarios require signing into the website.
- Creation of conducive test environment:Deleting cookies increases the effectiveness of testing as every test scenario is not dependent on test result of previous scenarios.
- Protection of privacy of users: Clearing of cookies ensures that all the personal details like username, email etc. are removed from the system thus prevent the access of data from unauthorized sources.
Conclusion
Cookie handling is an important aspect of automation testing. With the help of Selenium WebDriver API cookies are handled effectively thus speeding up the automation testing process.By utilising Selenium WebDriver s cookie handling methods testers can simulate real time use cases with better accuracy resulting in better test coverage. Remember to practice, stay updated with the latest trends in Java with Selenium, and maintain a positive attitude throughout your interview process.Handling of Cookies also saves time in regression and system testing process.
Also read :
Consult Us