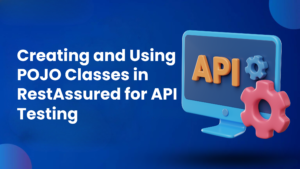

How to check if text box is present or not in Selenium
Selenium has proven to be one of the best and most widely used tools for automation testing of web applications. It is also the most preferred tool for browser automation through parallel and cross browser testing. Selenium is an open source and freely available tool which also supports cross platform testing and is compatible with various programming languages. Selenium on configuration with various programming languages offer various in build methods like isDisplayed, isEnabled etc. which ensures faster and seamless automation thus saving time.
isDisplayed() is an inbuild method already present in selenium which checks the presence of any element or component like checkbox, textfield etc. in a webpage. isDisplayed is an abstract method of WebElement interface and its return type is Boolean. If the Boolean value is true then it means that the element is present in the webpage and it will be displayed. If the Boolean value is false then the element is not present in webpage and it will not be displayed. To check that the element is present in a webpage, Selenium first navigates into the webpage then it tries to interact with the element of the webpage by checking the presence of CSS properties like visibility,display etc. If the element has visibility:hidden and display:none then the element is not present in the webpage and isDisplayed will return value as false but if the element has visibility and display value then the element is present and isDisplayed will return true value.
The below is the screenshot showing the visibility as hidden and display as none in the element section of inspect console
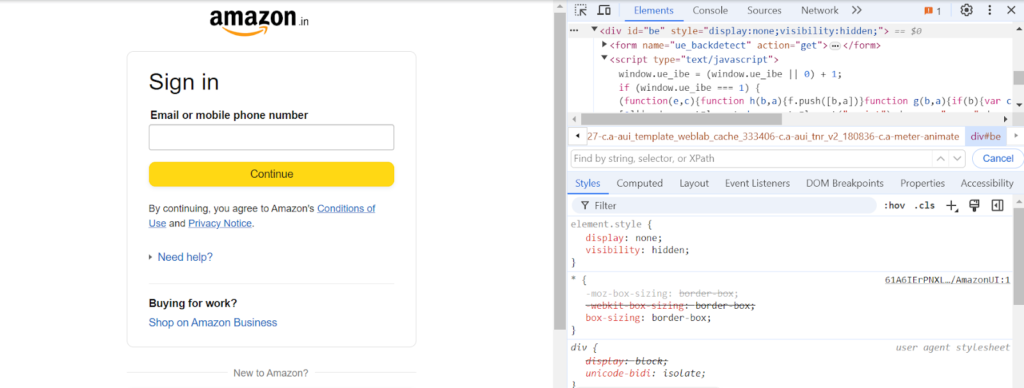
Procedure to check the presence of textbox in a webpage
- Create a new class in the existing package which contains selenium jar
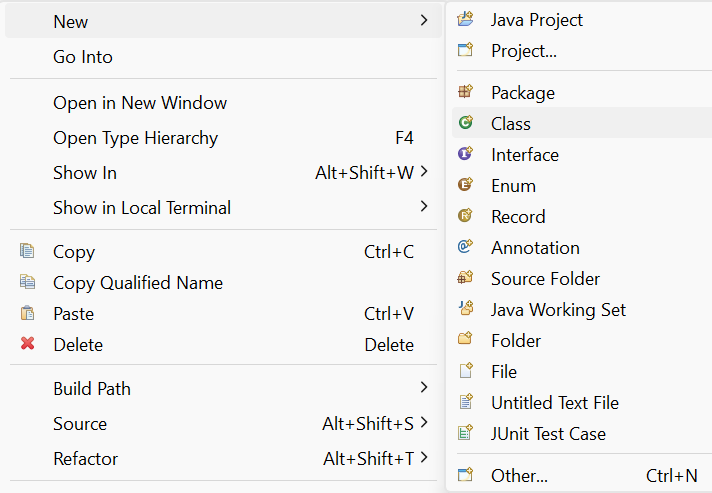
2. Declare driver globally using WebDriver class and use @Test annotation. Inside the @Test annotation create a method and inside that method launch empty Chrome browser using ChromeDriver class.
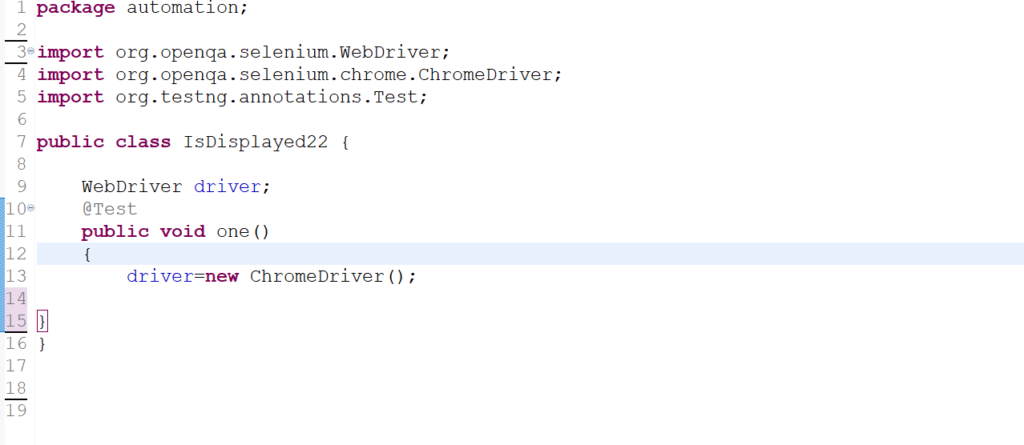
3. Maximize the chromebrowser using reference variable named ‘driver’of WebDriver class and maximize method. Then launch the amazon sign in webpage. Use implicit wait for proper synchronisation of web page by giving ‘10’ as waiting time duration in seconds.
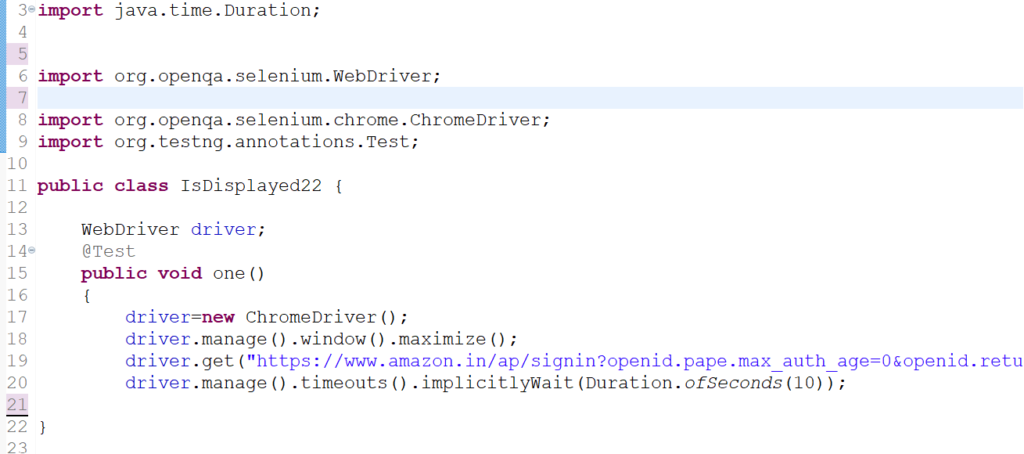
4. Using the findelement method and name locator,locate the email or mobile number text field of the amazon signin web page. Keep that entire line of code under the return type of WebElement interface and reference variable of ‘check’. The screenshot of the webpage and program code is mentioned below.
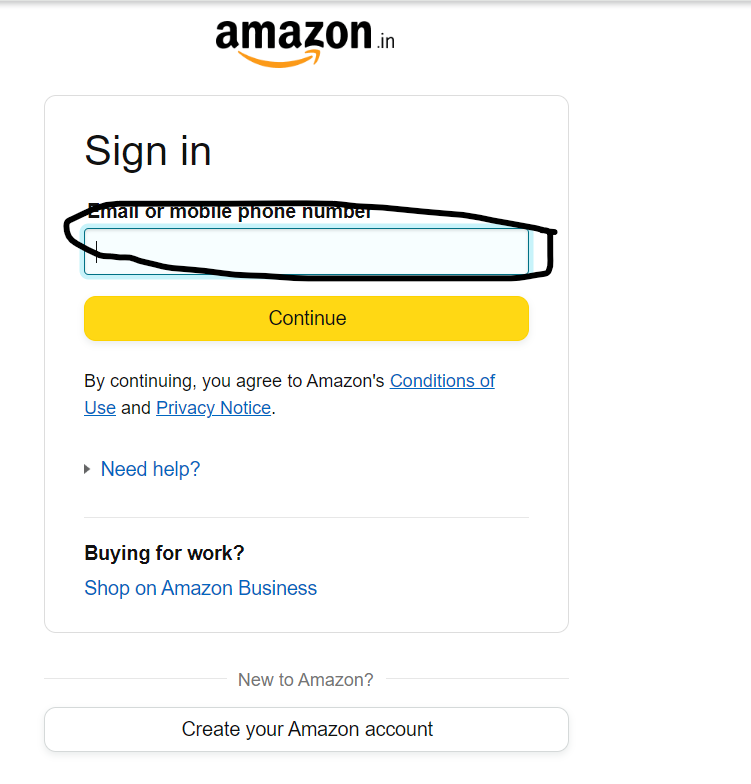

5. Using isDisplayed() method check the presence of ‘email’ textbox field of amazon login page using reference variable of WebElement interface and put the entire line of code in the boolean return type. Finally print the reference variable of boolean and its value will be printed in output console which will determine the presence of textbox. If value is true checkbox is present but if the value is false checkbox is not present.
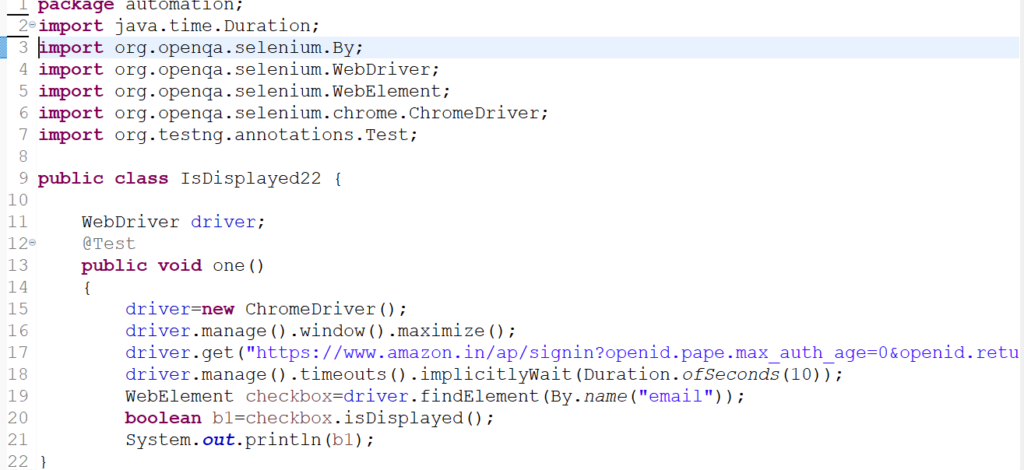
- The below screenshot shows the output of the entire code which mentions the boolean value as true indicating the presence of checkbox in the web page.
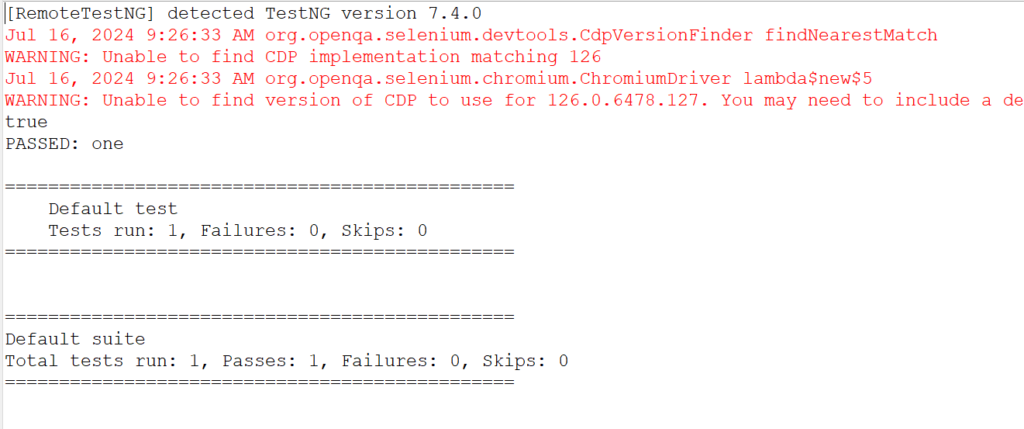
The below screenshot shows the code snippet and output of the code snippet where
isDisplayed() method is used on the element which is not present in the webpage and as a result boolean value is false in the output.
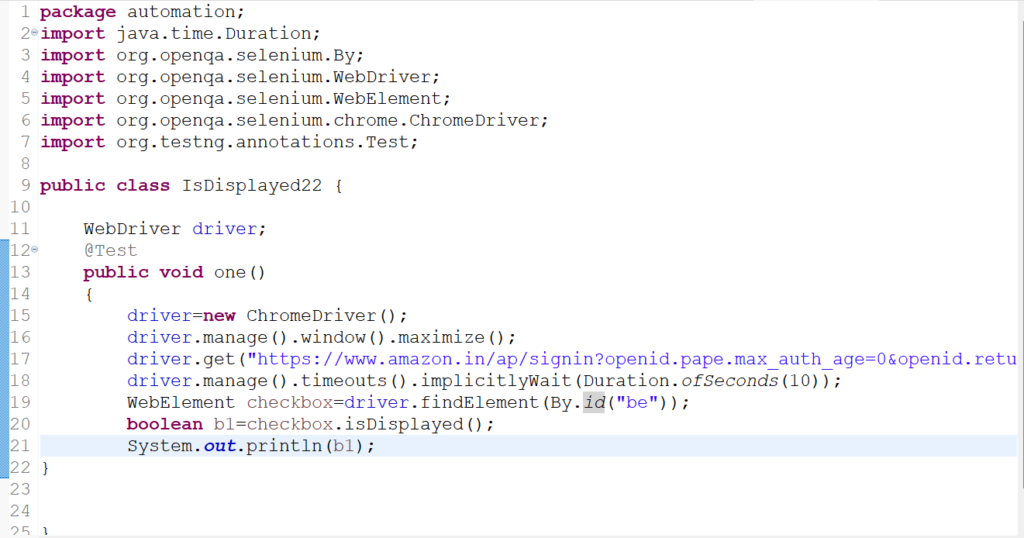
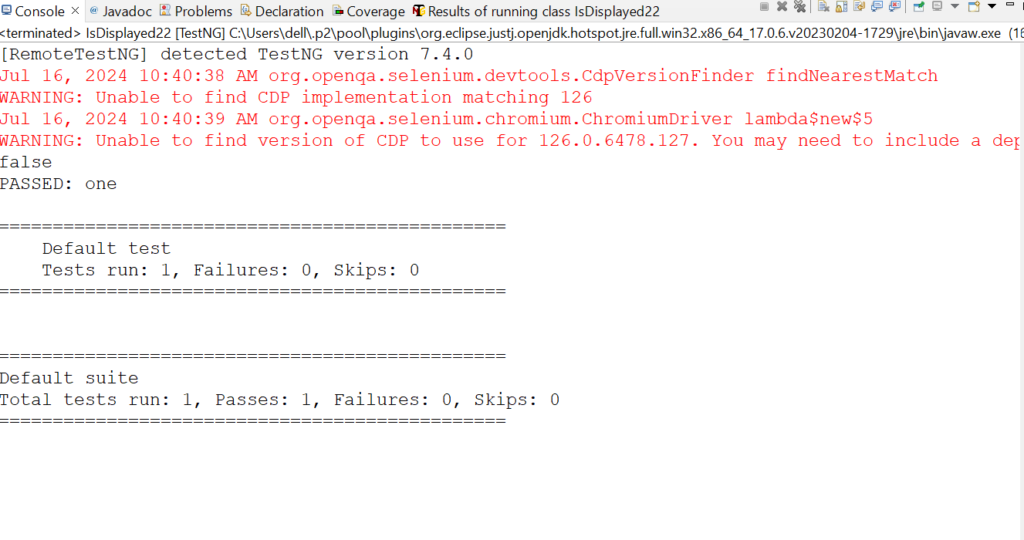
The entire code snippet for using isDisplayed method on an element which is not present in the webpage is shown below:
package automation;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class IsDisplayed22 {
WebDriver driver;
@Test
public void one()
{
driver=new ChromeDriver();
driver.manage().window().maximize();
driver.get("https://www.amazon.in/ap/signin?openid.pape.max_auth_age=0&openid.return_to=https%3A%2F%2Fwww.amazon.in%2F%3F%26tag%3Dgooghydrabk1-21%26ref%3Dnav_custrec_signin%26adgrpid%3D155259815513%26hvpone%3D%26hvptwo%3D%26hvadid%3D678802104188%26hvpos%3D%26hvnetw%3Dg%26hvrand%3D4249053511185199095%26hvqmt%3De%26hvdev%3Dc%26hvdvcmdl%3D%26hvlocint%3D%26hvlocphy%3D9299264%26hvtargid%3Dkwd-10573980%26hydadcr%3D14453_2371562&openid.identity=http%3A%2F%2Fspecs.openid.net%2Fauth%2F2.0%2Fidentifier_select&openid.assoc_handle=inflex&openid.mode=checkid_setup&openid.claimed_id=http%3A%2F%2Fspecs.openid.net%2Fauth%2F2.0%2Fidentifier_select&openid.ns=http%3A%2F%2Fspecs.openid.net%2Fauth%2F2.0");
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
WebElement checkbox=driver.findElement(By.id("be"));
boolean b1=checkbox.isDisplayed();
System.out.println(b1);
}
}
The entire code snippet for checking the presence of checkbox is shown below
package automation;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class IsDisplayed22 {
WebDriver driver;
@Test
public void one()
{
driver=new ChromeDriver();
driver.manage().window().maximize();
driver.get("https://www.amazon.in/ap/signin?openid.pape.max_auth_age=0&openid.return_to=https%3A%2F%2Fwww.amazon.in%2F%3F%26tag%3Dgooghydrabk1-21%26ref%3Dnav_custrec_signin%26adgrpid%3D155259815513%26hvpone%3D%26hvptwo%3D%26hvadid%3D678802104188%26hvpos%3D%26hvnetw%3Dg%26hvrand%3D4249053511185199095%26hvqmt%3De%26hvdev%3Dc%26hvdvcmdl%3D%26hvlocint%3D%26hvlocphy%3D9299264%26hvtargid%3Dkwd-10573980%26hydadcr%3D14453_2371562&openid.identity=http%3A%2F%2Fspecs.openid.net%2Fauth%2F2.0%2Fidentifier_select&openid.assoc_handle=inflex&openid.mode=checkid_setup&openid.claimed_id=http%3A%2F%2Fspecs.openid.net%2Fauth%2F2.0%2Fidentifier_select&openid.ns=http%3A%2F%2Fspecs.openid.net%2Fauth%2F2.0");
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
WebElement checkbox=driver.findElement(By.name("email"));
boolean b1=checkbox.isDisplayed();
System.out.println(b1);
}
}
Conclusion
So isDisplayed is one of the most important methods of Selenium to check the presence of element in a webpage which help in seamless automation testing in short period of time.Remember to practise, stay updated with the latest trends in Automation Software Testing Course,and maintain a positive attitude throughout your interview process.
Also read:
Consult Us