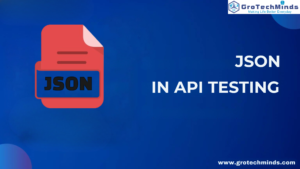

Types of BitWiseOperators In Java
What are Operators ?
Automation testing is a software testing technique that uses specialised tools and scripts to run predefined test cases, compare the results to what was expected, and report the results.
The fundamental purpose of automated testing is to improve the efficiency, effectiveness, and coverage of the testing process by automating repetitive and time-consuming processes that would otherwise be completed manually
In java operators are symbols that are used to perform operations on elements called as Operands. Here we will discuss about Types of Bitwise Operators.
Types of Bitwise Operators
The types of Bitwise Operators that are commonly used in Java are as follows:
- Bitwise And Operators
- Bitwise Exclusive OR Operators
- Bitwise Inclusive OR Operators
- Bitwise Compliment Operators
- Bitwise Shift Operators
Let us go through all the Bitwise Operators in detail
1.Bitwise AND Operators
AND is a bitwise operator denoted by ‘&’ symbol. It gives ‘1’ when both of the bit operands are ‘1’ or else it will give ‘0’ value.
a | b | a&b |
1 | 1 | 1 |
0 | 1 | 0 |
1 | 0 | 0 |
0 | 0 | 0 |
Code Snippet:
package bitWiseOperator;
public class BItwiseAnd {
public static void main(String[] args) {
int a=10; //binary of 10 is 1010
int b=12; // binary of 12 is 1100
//The result of 1010&1100 is 1000 and is decimal conversion is 8
System.out.println("The resultant value of a&b is "+(a&b));
}
}
Output: The resultant value of a&b is 8
Screenshot:

2. Bitwise Exclusive OR(XOR) Operators
It is a bitwise operator denoted by‘^’ symbol which is called as ‘caret’. It gives ‘0’ if both the bit operands are same otherwise it will give ‘1’ value.
a | b | a^b |
1 | 1 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
0 | 0 | 0 |
Code Snippet:
package bitWiseOperator;
public class BitWiseExlusiveOR {
public static void main(String[] args) {
int a=10; //binary of 10 is 1010
int b=12; // binary of 12 is 1100
//The result of 1010^1100 is 0110 and is decimal conversion is 6
System.out.println("The resultant value of a^b is "+(a^b));
}
}
Output:
The resultant value of a^b is 6
Screenshot:
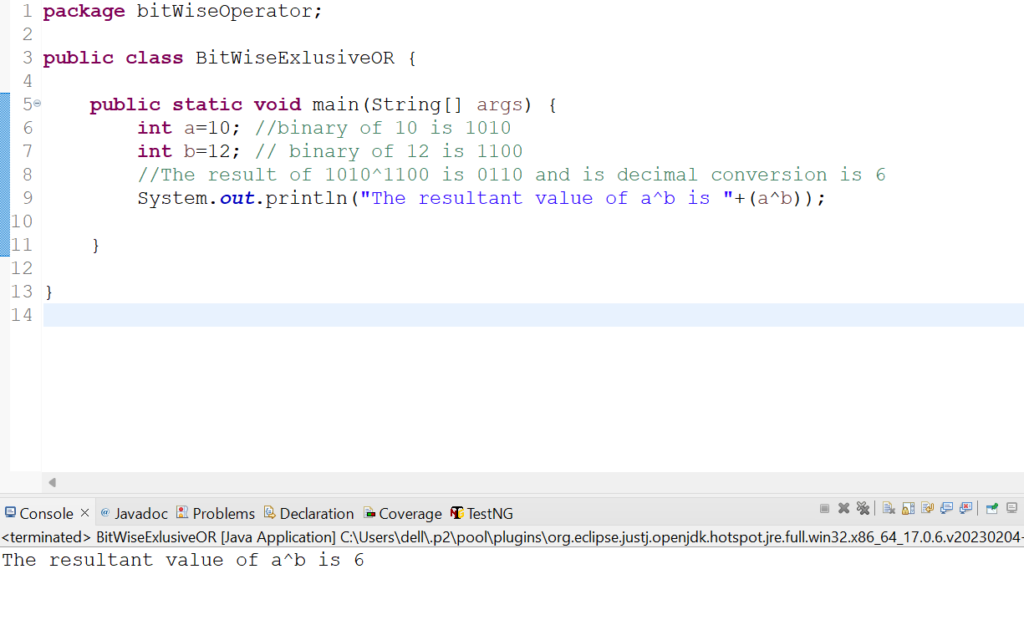
3. Bitwise Inclusive OR Operators
It is a bitwise operator denoted by ‘I’symbol where ‘I’ is called as ‘pipe’. It gives ‘1’ value if any of the bit operand is ‘1’ otherwise it gives ‘0’ value.
a | b | a|b |
1 | 1 | 1 |
0 | 1 | 1 |
1 | 0 | 1 |
0 | 0 | 0 |
Code Snippet:
package bitWiseOperator;
public class BitWiseInclusiveOR {
public static void main(String[] args) {
int a=10; //binary of 10 is 1010
int b=12; // binary of 12 is 1100
//The result of 1010^1100 is 1110 and is decimal conversion is 14
System.out.println("The resultant value of a|b is "+(a|b));
}
}
Output: The resultant value of a|b is 14
Screenshot:

4. Bitwise Compliment Operators
It is a bitwise operator denoted by ‘~’ symbol which is also called as ‘tilde’.It gives the inverse or complement of the operand. Suppose if the operand value is 1 its compliment will be zero. Similarly compliment of zero will be 1.
a | ~a |
1 | 0 |
0 | 1 |
Code Snippet:
package bitWiseOperator;
public class BitWiseCompliment {
public static void main(String[] args) {
int a=2;
//binary number of 2 is 0010
//compliment of 0010 is 1101
// decimal of 1101 is -3
System.out.println("Compliment of 2 is "+(~a));
}
}
Output: Compliment of 2 is -3
Screenshot:
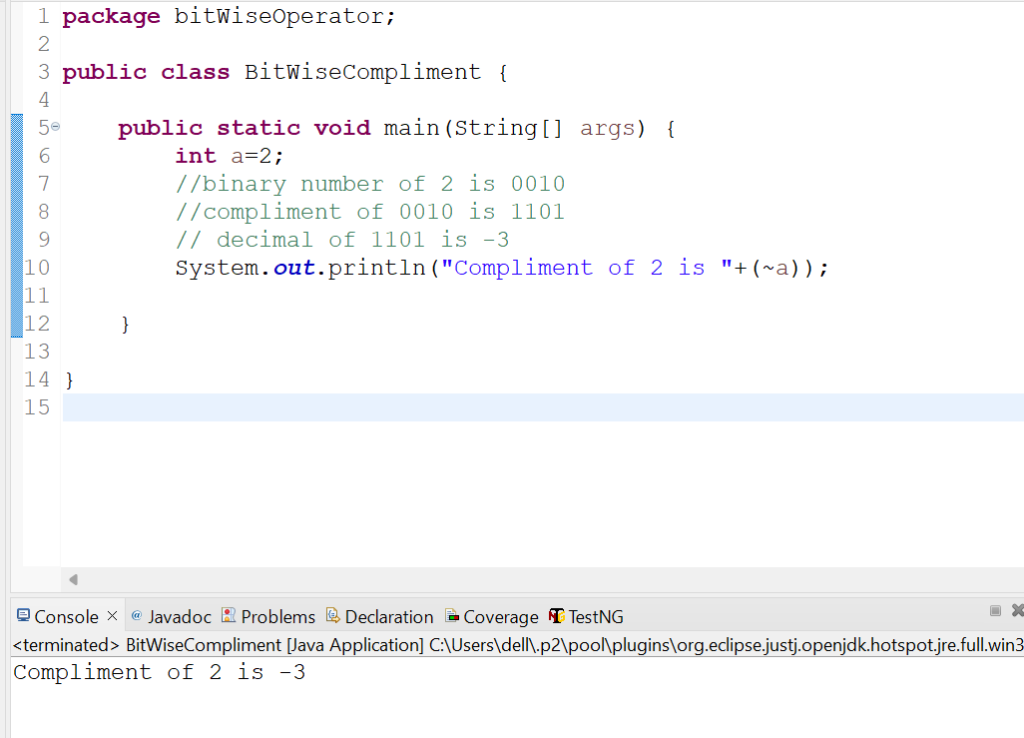
5.Bitwise Shift Operators
Shift Operators are used to shift the bit operands in left or right direction. Shift operator is used whenever any number is divided or multiplied by 2. The shift operator is represented in following way :
Variablename >> or Variablename<< where << and >> represents number of places to be shifted.
For example if a=12;
a>>2-shifts 2 bits
a>>3- shifts 3 bits
Types of Shift Operators
The various types of shift operators used in java are as follows
1 Signed Right Shift Operator or Bitwise Right Shift Operator
2.Signed Left Shift Operator or Bitwise Left Shift Operator
3.Unsigned Right Shift Operator
1.Signed Right Shift Operator
This operator is denoted by “>>” symbol is used to move the bits of a number towards right by a specified number of places. It also maintains the leftmost bit. If 0 is leftmost bit then the number is positive but if 1 is leftmost bit then the number is negative. The signed Right Shift Operator is represented as follows:
b=a>>n => b=a/(2^n) where a>>n means that bits of a value is shifted “n” places towards right.
Code Snippet:
package bitWiseOperator;
public class SignedRightShiftOperator {
public static void main(String[] args) {
int a = 10;
System.out.println("a>>2 ="+(a >>2));
}
}
Output: a>>2 =2
Screenshot:

Explanation: In this code snippet a=10; and a>>2 so it implies 10>>2 and as per formula b=a/(2^n) => b=10/(2^2) as n=2. So the result is 2.5 which is rounded up to 2. So the final answer is 2.
2.Signed Left Shift Operator
This operator is denoted by “<<” symbol is used to move the bits of a number towards left by a specified number of places. It does not maintain the leftmost bit. The signed Left Shift Operator is represented as follows:
b=a<<n => b=a*(2^n) where a<<n means that bits of a value is shifted “n” places towards left.
Code Snippet:
package bitWiseOperator;
public class SignedLeftShiftOperator {
public static void main(String[] args) {
int a = 10;
System.out.println("a<<2 ="+(a<<2));
}
}
Output: a<<2 =40
Screenshot:

Explanation: In this code snippet a=10; and a<<2 so it implies 10<<2 and as per formula b=a*(2^n) => b=10*(2^2) as n=2. So the result is 40.
3.Unsigned Right Shift Operator
It is represented by ‘>>>’ symbol. It is used for shifting zero at left most place and fills up 0.The leftmost place after >> depend on sign bit but it does not maintain sign bit.
Code Snippet:
package bitWiseOperator;
public class UnSignedRightShiftOperator {
public static void main(String[] args) {
int a = 10;
System.out.println("a>>>2="+(a>>>2));
}
}
Output: a>>>2=2
Screenshot:
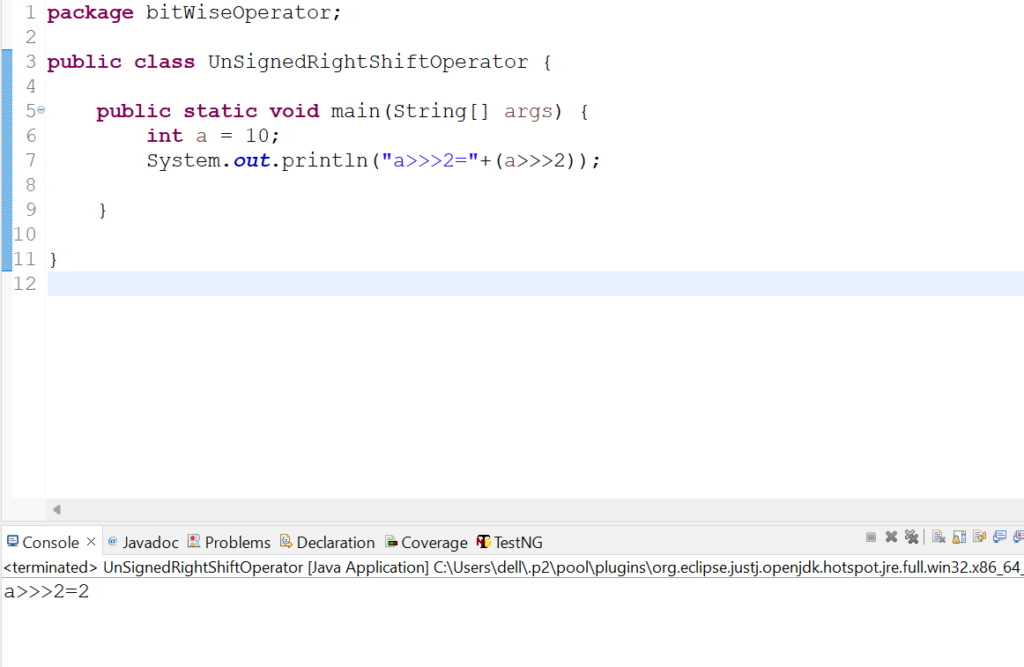
Explanation: Here a=10 and its binary value is 1010. After shifting 1010 2 places right it gives 0010 and its decimal value is 2 .In other words since last two digits are 1 and 0 so 1 is assumed as 0 and is shifted along with 0 to the left of first two digits and it gives 0010 as a result. So a>>>2 is 2.
Note: If the decimal value is 00100 then 00100>>>2 will shift last two zeros to left of first 2 digits resulting in 00001 and its decimal number is 1.
Conclusion:
Bitwise operators are used by Java developers to to perform low level operations and performance optimisation. They most effective tools in java and are widely used in System level programming and data encoding works. Remember to practice, stay updated with the latest trends in Java with Selenium, and maintain a positive attitude throughout your interview process. In selenium it is used for handling multiple browser configurations and huge amount of datas.
Also read :
Consult Us