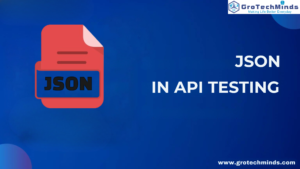

Top 23 Automation Testing Interview Questions and Answers
Q1. What is Abstraction?
Hiding the implementation and exposing only what is required is known as abstraction. With the help of an interface, we can achieve 100% abstraction. With the help of abstract class, we can achieve 0 to 100% abstraction because in abstract class we have abstract methods and concrete methods.
Q2. Explain this calling statement? Talk about its use and types?
- This Calling statement is the first line in every constructor.
- In java, one constructor and the other constructor are present in the same class.
- There are two types of this calling statement parameterized and non-parameterized
- It removes the confusion between parameters with the same name.
1- Parameterized :This is used to call a constructor which is parameterized within the same class.
2- Non-parameterized :This is used to call a constructor which is non-parameterized.
- In java, super calling is the first line in each constructor.
- It is used to call parent class constructors from child class constructors.
There are two types of super calling statement:
1-Parametrized: It is used to call parameterized parent class constructors.
2-Non-parameterized:It is used to call a non-parameterized parent class.
Yes
- int rollno[] = new int[2];
- rollno[0]= 1;
- rollno[1] = 2;
- rollno[1] = 5; //updating the value
- rollno[2] = 10;
public is access specifier:- access specifier has 4 types which is
- public
- private
- default/package
- protected
static is a modifier which has 2 types static and non-staticvoid is return type which has
- byte
- short
- int
- char
- float
- double
- long
- String
- boolean
main is the method name.
JVM always looks for a public static void main to run the program.
Java internally works in three phases that are :
- Human Readable language
- Compilation
- Interpretation
1.Human Readable language:- Source code of java don’t understand Human Readable language, So we have to saved it in .java file
2 Compilation:- In Compilation .java file gets converted or translated into .class file. Before compilation it goes into three phase-
- Syntax:- If there is any violation in code then it will give a compile time error.
- Run the code line by line
- Translate .java into .class file
3. Interpretation :- In Interpretation, .class file convert into Binary Language, Interpretation also goes to three phase
- Execute
- Read line by line
- Translate the .class file into binary language.
For Interpretation we need JDK, JRE, JVM and JIT. Finally we will get output in OS
Yes
- To call the static method we need to print the system.out.println(); in the main method,
- To call the non-static method we need to create an object that is classname rv.=new classname();
- To call the constructor we need to create an object new classname ();
Inheritance is a fundamental concept in Java that allows one class to inherit properties and behaviours (methods) from another class. This feature promotes code reusability, organisational structure, and a hierarchical classification, making it easier to manage and understand complex systems.
Line by line
- By.Id
- By.className
- By.tagName
- By.cssselector
- By.xpath
- By.linkText
- By.partialLinkText
- By.name
There are 2 ways to launch the website
1.Using driver.get() method
2.using driver.navigate() method
Implicit wait – driver.manage().timeouts().implicitlywait(Duration.ofSeconds(10));
Explicit wait – WebDriverWait w1=new WebDriverWait(driver, Duration.ofSeconds(10));
w1.until(ExpectedConditions.list of methods);
Q15.What do you mean by class as public and default?
- Class as public means it can be accessed within the package and also outside the package.
- Class as default means it can only be accessed within the package.
Q16.What do you mean by Collection and Collections in Java?
Collection
- It is an interface. The interface can also contain abstract and default methods.
- It extends the iterable interface.
- Collection has many interfaces and classes in it.
- It doesn’t have any direct implementation as it is an interface. But you can implement the collection interface by using various Java classes, like ArrayList, HashSet, and PriorityQueue.
Collections
- It is a class
- It extends the Object class.
- It has a lot of methods that can be used to work on the collection.
- As it is a final class, meaning it can not be subclassed. It doesn’t have any public constructors, so its methods are accessed statically.
Q17.Explain the difference between findelement and findelements in selenium.
FindElement
- Return type is WebElement
- If element is not found it will give NoSuchElementException
FindElements
- Return Type is List<Webelement>
- If element is not found it will give empty list
Q18.What is the difference between close and quit in selenium?
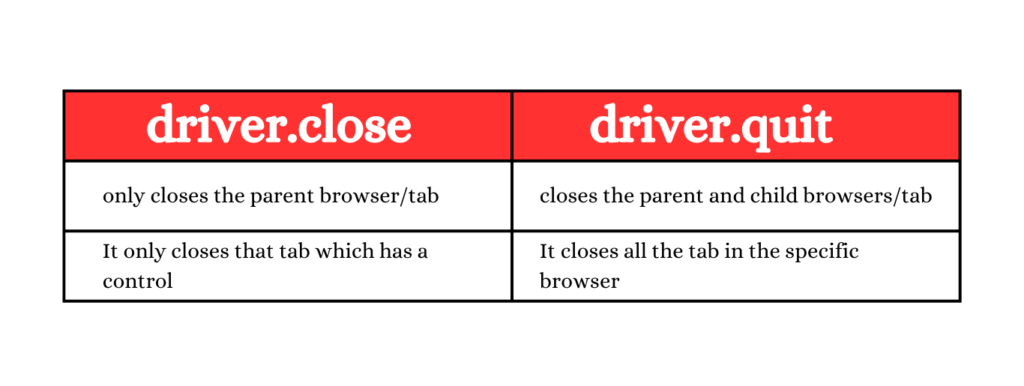
Q19.What is the difference between implicit wait and explicit wait?
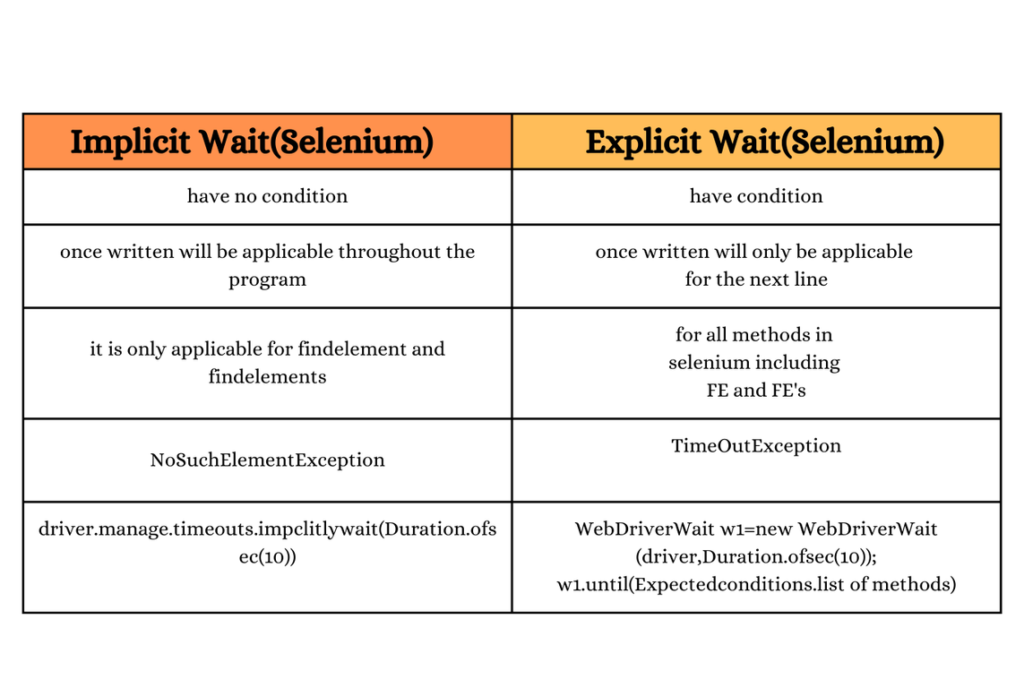
Q20.Explain method overloading and constructor overloading
- Method overloading can have multiple methods with the same name but with the different arguments, this will return some value . Also method names can not be the same as class names.
- Constructor overloading means constructor name is same as class name and this can have multiple method with the same name but with the different parameters is called constructor overloading and does not have return type wherein.
Q21. Explains Cursors in Java.
A Java Cursor is an Iterator, which is used to iterate or traverse or retrieve a Collection or Stream object’s elements one by one. There are three cursors in Java.
- Iterator
- ListIterator
- Enumeration
Q22. Arraylist belongs to which package?
Java.util package.
Q23. Name some Arraylist methods.
- add() :- is used to add the elements or objects in an ArrayList or say collection object
- addAll() :- addAll() method is used to add or append all the elements in the specified collection to the end of ArrayList or collection object
- remove() :- It is used to remove the element at the specified position in the ArrayList
- removeAll() :- It is used to remove the elements from the current ArrayList which are contained in the specified ArrayList or say collectionobject
- clear() :- It is used to remove all of the elements from the ArrayList or say collection object
- contains() :- It is used to check if the specified element is present in the given ArrayList or not, if its present it will return true else false
- size() :- It is used to return the number of elements in the ArrayList i.e.the size
- get() :- It is used to get the element of a specified index within the ArrayList
- set() :- It is used to replace the element at the specified index position in the ArrayList with the specified element
- indexOf() :- It is used to return the index of first occurrence ofthe element in the ArrayList. If this list does not contain the element then it will return -1
- 11.iterator() :- It is used to get an iterator over the elements in this list in proper sequence
Also read:
Consult Us