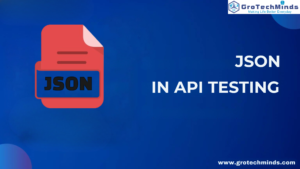

StringBuffer and methods
Java StringBuffer class is used to create mutable (modifiable) String objects. The StringBuffer class in Java is the same as the String class except it is mutable i.e. it can be changed. Java StringBuffer class is thread-safe(It is synchronized) i.e. multiple threads cannot access it simultaneously. So it is safe and will result in an order.

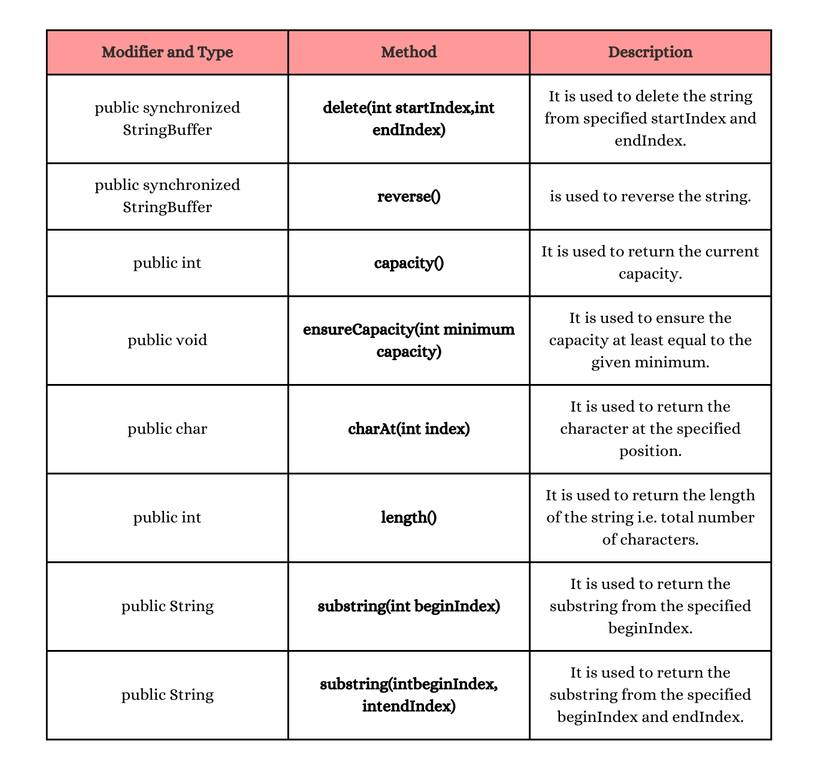
When something is “immutable,” it means it cannot be changed after it is created. In Java, a String is immutable, which means once you create a string, you cannot change the characters that make up that string.
Java uses a special memory area called the “string pool” to store strings efficiently. When you create a string, Java checks if the same string is already in the pool. If it is, Java uses that existing string instead of making a new one. This saves memory because multiple parts of your code can use the same string without creating a duplicate because strings can’t change, they are safe to use in different parts of your program without worrying that their values will be altered unexpectedly. Example
String name = “Alex”;
String fullname = name + ” Thomas”;
Here, the name is like the first tag. When you add “Thomas” to the name, Java doesn’t change the original name tag. Instead, it creates a new string (a new tag) with “Alex Thomas” and assigns it to fullname.
When something is “mutable,” it can be changed after it is created. StringBuffer is mutable, which allows us to change the characters inside it after it has been made. When we create a StringBuffer, we start with an empty or a preset sequence of characters that we can change anytime. We can add to it, remove parts of it, or even insert new characters in the middle. Unlike strings, StringBuffer objects do not use the string pool. Each StringBuffer is a separate object in memory, and it’s more like a flexible container that you can keep adding to or changing without needing to create new containers.
package strings_assisgnment;
public class Program {
public static void main(String[] args) {
String name="Alex";
System.out.println(name.concat("Thomas"));//created two strings
StringBuffer name1=new StringBuffer();
StringBuffer name2=name1.append("Thomas");
System.out.println(name2);//add new String
}
}
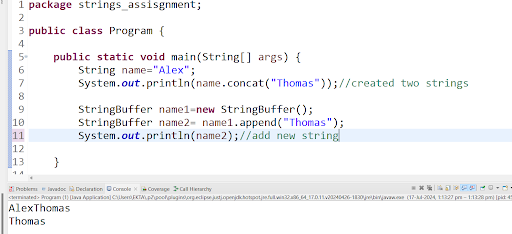

StringBuffer is mutable which allows us to change the characters inside it after it has been made. When we create a StringBuffer, we start with an empty or a preset sequence of characters that we can change anytime. Remember to practise, stay updated with the latest trends in Automation Software Testing Course,and maintain a positive attitude throughout your interview process. We can add to it, remove parts of it, or even insert new characters in the middle. Unlike strings, StringBuffer objects do not use the string pool. The StringBuffer class is synchronized.
Also read:
Consult Us