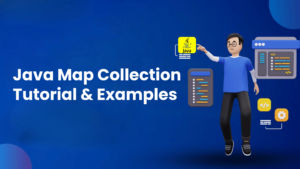
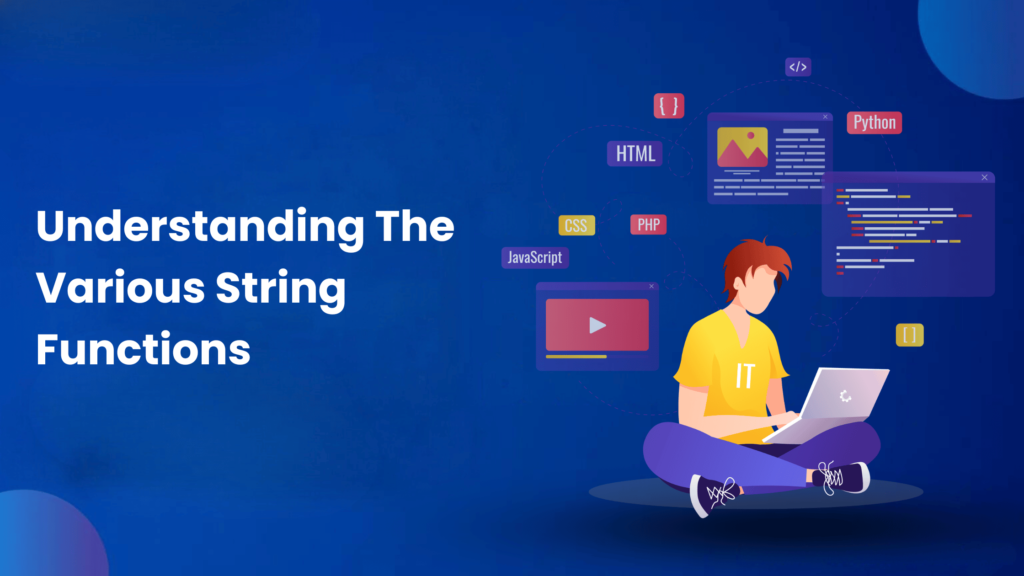
Understanding the Various String Functions
‘String’ is a class-based non-primitive or reference data type. Whenever we store any value in a String variable, we are storing references to String objects and not the actual String data. It is unlike primitive data types like byte, short, int, long, float, double, boolean, and char where the variables stored are actual values we want to store. String is one of the widely used data type which is represented by the sequence of characters. The ‘String’ class has a long list of methods that can be used for the manipulation of the given string value.
The two most popular ways of creating a String variable are,
- By declaring a variable as String and initialising the variable by assigning a value to that variable in double quotes””.
- By declaring a variable as String and using the new keyword to initialise the variable and assigning a value in double quotes”” as the parameter.
Code Snippet:
public static void main(String[] args)
{
//method 1
String s1 = "Manish Kumar Tiwari";
System.out.println("The declared and intialized String is : "+s1);
//method 2
String s2 = new String("Grotechminds");
System.out.println("The declared and intialized String is : "+s2);
}
Output:
The declared and initialise String is : Manish Kumar Tiwari
The declared and initialise String is : Grotechminds
String Functions:
String functions are the methods predefined in the String class. Some of the widely used String functions that can be categorised as ‘must know’ are as follows.
1. To calculate the length of a given String, we use a method of String class called ’length()’.
Code Snippet:
public static void main(String[] args)
{
String s2 = new String("Grotechminds");
System.out.println("The Length of the given String is : "+s2.length());
}
Output:
The Length of the given String is : 12
2. To find the ‘char’ value present in any index position of a String we can use the method of the String class called ‘charAt(int index)’.
Code Snippet:
public static void main(String[] args)
{
int i=0;
int j=7;
int k=13;
String s2 = new String("Manish Kumar Tiwari");
System.out.println("The char of the index position "+i+" is : "+s2.charAt(i));
System.out.println("The char of the index position "+j+" is : "+s2.charAt(j));
System.out.println("The char of the index position "+k+" is : "+s2.charAt(k));
}
Output:
The char of the index position 0 is : M
The char of the index position 7 is : K
The char of the index position 13 is : T
3. To create a substring with an existing String using two index positions, we use a method of String class called ‘substring(int beginIndex, int endIndex)’
Code Snippet:
public static void main(String[] args)
{
int i=0;
int j=3;
int l=7;
int n=12;
String s2 = new String("Grotechminds");
System.out.println("The substring for the given index positions "+i+" and "+j+" is : "+s2.substring(i,j));
System.out.println("The substring for the given index positions "+j+" and "+l+" is : "+s2.substring(j,l));
System.out.println("The substring for the given index positions "+l+" and "+n+" is : "+s2.substring(l,n));
}
Output:
The substring for the given index positions 0 and 3 is : Gro
The substring for the given index positions 3 and 7 is : tech
The substring for the given index positions 7 and 12 is : minds
4. To create a substring using an existing ‘String’ using only one index position we use a method of String class called ‘substring(int beginIndex)’
Code Snippet:
public static void main(String[] args)
{
int i=0;
int j=3;
int l=7;
String s2 = new String("Grotechminds");
System.out.println("The substring for the given index positions "+i+" is : "+s2.substring(i));
System.out.println("The substring for the given index positions "+j+" is : "+s2.substring(j));
System.out.println("The substring for the given index positions "+l+" is : "+s2.substring(l));
Output:
The substring for the given index positions 0 is : Grotechminds
The substring for the given index positions 3 is : techminds
The substring for the given index positions 7 is : minds
5. To join two or more ‘String’ variables into one ‘String’ variable we can use a method of String class called ‘concat(String str)’
Code Snippet:
public static void main(String[] args)
{
String s1 = new String("Software testing");
String s2 = new String(" by ");
String s3 = new String("Manish Kumar Tiwari");
String s4 = s1.concat(s2).concat(s3);
System.out.println("The concatenated String is : "+s4);
}
Output:
The concatenated String is : Software testing by Manish Kumar Tiwari
6. To find the index position of any character in a ‘String we can use a method of String class called, ‘indexOf(int ch)’
Code Snippet:
public static void main(String[] args)
{
String s1 = new String("Software testing by MKT");
int s2 = s1.indexOf('s');
int s3 = s1.indexOf('b');
int s4 = s1.indexOf('M');
System.out.println("The index position of the given char in the String is : "+s2);
System.out.println("The index position of the given char in the string is : "+s3);
System.out.println("The index position of the given char in the String is : "+s4);
}
Output:
The index position of the given char in the String is : 11
The index position of the given char in the string is : 17
The index position of the given char in the String is : 20
- To find the index position of the first occurrence of the specified substring, we use a method of String class called ‘indexOf(String str)’
Code Snippet:
public static void main(String[] args)
{
String s1 = new String("Software testing by MKT");
int s2 = s1.indexOf("Soft");
int s3 = s1.indexOf("test");
int s4 = s1.indexOf("MKT");
System.out.println("The starting index position of the given substring in the String is : "+s2);
System.out.println("The starting index position of the given substring in the string is : "+s3);
System.out.println("The starting index position of the given substring in the String is : "+s4);
}
Output:
The starting index position of the given substring in the String is : 0
The starting index position of the given substring in the string is : 9
The starting index position of the given substring in the String is : 20
8. To find the last occurrence of a char value that is present inside a String, we use a method of String class called ‘lastIndexOf(int ch)’
Code Snippet:
public static void main(String[] args)
{
String s1 = new String("Software testing by MKT @ Grotechminds");
int s2 = s1.lastIndexOf('S');
int s3 = s1.lastIndexOf('m');
int s4 = s1.lastIndexOf('K');
System.out.println("The ending index position of the given char in the String is : "+s2);
System.out.println("The ending index position of the given char in the string is : "+s3);
System.out.println("The ending index position of the given char in the String is : "+s4);
}
Output:
The ending index position of the given char in the String is : 0
The ending index position of the given char in the string is : 33
The ending index position of the given char in the String is : 21
- To find the last occurrence of a substring inside a String we use a method of String class called ‘lastIndexOf(String str)’
Code Snippet:
public static void main(String[] args)
{
String s1 = new String("Software testing by MKT @ Grotechminds");
int s2 = s1.lastIndexOf("in");
int s3 = s1.lastIndexOf("te");
int s4 = s1.lastIndexOf("KT");
System.out.println("The ending index position of the given substring in the String is : "+s2);
System.out.println("The ending index position of the given substring in the string is : "+s3);
System.out.println("The ending index position of the given substring in the String is : "+s4);
}
Output:
The ending index position of the given substring in the String is : 34
The ending index position of the given substring in the string is : 29
The ending index position of the given substring in the String is : 21
10.To check if an user-specified char sequence is present in a String, we use a method of String class called ‘contains(CharSequence s)’
Code Snippet:
public static void main(String[] args)
{
String s1 = new String("Grotechminds");
boolean s2 = s1.contains("tech");
boolean s3 = s1.contains("MKT");
System.out.println("Is the given char sequence present in the String ? "+s2);
System.out.println("Is the given char sequence present in the String ? "+s3);
}
Output:
Is the given char sequence present in the String ? true
Is the given char sequence present in the String ? false
11. To check if the given char sequence is present at the start of String we have a method of String class called ‘startsWith(String prefix)’
Code Snippet:
public static void main(String[] args)
{
String s1 ="Grotechminds";
boolean s2 = s1.startsWith("tech");
boolean s3 = s1.startsWith("Gro");
System.out.println("Is the given char sequence present at the starting of String ? "+s2);
System.out.println("Is the given char sequence present at the starting of String ? "+s3);
}
Output:
Is the given char sequence present at the starting of String ? false
Is the given char sequence present at the starting of String ? true
12. To check if the given char sequence is present at the ending of String we have a method of String class called ‘endsWith(String suffix)’
Code Snippet:
public static void main(String[] args)
{
String s1 ="Grotechminds";
boolean s2 = s1.endsWith("minds");
boolean s3 = s1.endsWith("Gro");
System.out.println("Is the given char sequence present at the end of String ? "+s2);
System.out.println("Is the given char sequence present at the end of String ? "+s3);
}
Output:
Is the given char sequence present at the end of String ? true
Is the given char sequence present at the end of String ? false
13. To make any character in any given String to lower Case from upper case, we use a method of String class called, ‘toLowerCase()’
Code Snippet:
public static void main(String[] args)
{
String s1 ="Grotechminds";
String s2 =s1.toLowerCase();
System.out.println("The String converted to all lowercase is = "+s2 );
}
Output:
The String converted to all lowercase is = grotechminds
14.To make any character in any given String to Upper Case from lower case, we use a method of String class called, ‘toUpperCase()’
Code Snippet:
public static void main(String[] args)
{
String s1 ="Grotechminds";
String s2 =s1.toUpperCase();
System.out.println("The String converted to all Upper case is = "+s2 );
}
Output:
The String converted to all Upper case is = GROTECHMINDS
- To trim all the spaces at the end and the beginning of the String, we can use a method of String class called ‘trim()’
Code Snippet:
public static void main(String[] args)
{
String s1 =" Software Testing ";
String s2 =s1.trim();
System.out.println("The String trimmed is = "+s2 );
}
Output:
The String trimmed is = Software Testing
16. To replace all the similar char values in a String, with a new char value, we use a method of String class called ‘replace(CharSequence target, CharSequence replacement)’
Code Snippet:
public static void main(String[] args)
{
String s1 =" Software Testing ";
String s2 =s1.replace('t', 'z');
System.out.println("The String after replacing a char value is = "+s2 );
}
Output:
The String after replacing a char value is = Sofzware Teszing
17. To replace a specific substring in a String, with a new substring, we use a method of we use a method of String class called ‘replace(char oldChar, char newChar)’
Code Snippet:
public static void main(String[] args)
{
String s1 =" Software Testing ";
String s2 =s1.replace("re", "te");
System.out.println("The String after replacing a char value is = "+s2 );
}
Output:
The String after replacing a char value is = Softwate Testing
18. To replace a substring having regular expression in a String, with a new substring, we use a method of String class called ‘replaceAll(String regex, String replacement)’
Code Snippet:
public static void main(String[] args)
{
String s1 ="Software Testing by MKT";
String s2 =s1.replaceAll("[aeiouAEIOU]", "#");
System.out.println("The String after replacing all the vowels with # is is = "+s2 );
}
Output:
Replacing all vowels: R*pl*c* v*w*ls *n th*s str*ng
19. To replace the first instance of the substring having regular expression in a String, with a new substring, we use a method of String class called ‘replaceFirst(String regex, String replacement)’
Code Snippet:
public static void main(String[] args)
{
String s1 ="Software Testing by MKT";
String s2 =s1.replaceFirst("[aeiouAEIOU]", "#");
System.out.println("The String after replacing the First instance of all the vowels with # is is = "+s2 );
}
Output:
The String after replacing the First instance of all the vowels with # is is = S#ftware Testing by MKT
20.To check if an object has the same char value sequence as that of the String variable (another object), we use a method of String class called ‘equals(Object anObject)’
Code Snippet:
public static void main(String[] args)
{
String s1 ="Software Testing by MKT";
String s2 ="Software Testing by GTM";
String s3 ="Software Testing by MKT";
boolean s4 =s1.equals(s3);
boolean s5 =s1.equals(s2);
System.out.println("Is the String "+s1+" equal to "+s3+" ? "+s4 );
System.out.println("Is the String "+s1+" equal to "+s2+" ? "+s5 );
}
Output:
Is the String Software Testing by MKT equal to Software Testing by MKT ? true
Is the String Software Testing by MKT equal to Software Testing by GTM ? false
21. To check if an object has the same char value sequence as that of the String variable (another object), ignoring the case sensitivity of the char sequence in both the objects, we can use a method of String class called ‘equalsIgnoreCase(String anotherString)’
Code Snippet:
public static void main(String[] args)
{
String s1 ="SOFTWARE Testing by mkt";
String s2 ="Software Testing by GTM";
String s3 ="Software Testing by MKT";
boolean s4 =s1.equalsIgnoreCase(s3);
boolean s5 =s1.equalsIgnoreCase(s2);
System.out.println("Is the String "+s1+" equal to "+s3+" ? "+s4 );
System.out.println("Is the String "+s1+" equal to "+s2+" ? "+s5 );
}
Output:
Is the String SOFTWARE Testing by mkt equal to Software Testing by MKT ? true
Is the String SOFTWARE Testing by mkt equal to Software Testing by GTM ? false
22. To split a given string based on the regular expression, we use a method of String class called ‘split(String regex)’
Code Snippet:
public static void main(String[] args)
{
String s1 ="Software Testing by MKT";
String[] s2=s1.split(" ");
System.out.println("The String Array made by splitting "+s1+" is "+Arrays.toString(s2));
}
Output:
The String Array made by splitting Software Testing by MKT is [Software, Testing, by, MKT]
23. To join a group of char sequences, with a char sequence that repeats between those individual char sequences in a group, we use a method of String class called ‘join(CharSequence delimiter, CharSequence… elements)’
Code Snippet:
public static void main(String[] args)
{
String s1 =String.join(" * ", "Software","Testing","by","MKT");
System.out.println("The String after joining the char sequences is : "+s1);
}
public static void main(String[] args)
{
String s1 =String.join(" * ", "Software","Testing","by","MKT");
System.out.println("The String after joining the char sequences is : "+s1);
}
Output:
The String after joining the char sequences is : Software * Testing * by * MKT
24. To get the String representation of a variable of a primitive data type as well as an object, we use a method of String class called ‘valueOf(Object obj)’
Code Snippet:
public static void main(String[] args)
{
int a=100;
double b=50.5;
char c='x';
boolean d=true;
float f=33.34f;
String s1=String.valueOf(a);
String s2=String.valueOf(b);
String s3=String.valueOf(c);
String s4=String.valueOf(d);
String s5=String.valueOf(f);
System.out.println("A int variable is converted to a String variable, and its value is : "+s1);
System.out.println("A double variable is converted to a String variable, and its value is : "+s2);
System.out.println("A char variable is converted to a String variable, and its value is : "+s3);
System.out.println("A boolean variable is converted to a String variable, and its value is : "+s4);
System.out.println("A float variable is converted to a String variable, and its value is : "+s5);
}
Output:
A int variable is converted to a String variable, and its value is : 100
A double variable is converted to a String variable, and its value is : 50.5
A char variable is converted to a String variable, and its value is : x
A boolean variable is converted to a String variable, and its value is : true
A float variable is converted to a String variable, and its value is : 33.34
- To convert all the individual char values in a String object (String variable) to individual char values of char array in its respective index positions we use a method of String class called ‘toCharArray()’
Code Snippet:
public static void main(String[] args)
{
String s ="Grotechminds";
char[]c = s.toCharArray();
System.out.println("The char Array created by the String "+s+" is : "+Arrays.toString(c));
}
Output:
The char Array created by the String Grotechminds is : [G, r, o, t, e, c, h, m, i, n, d, s]
26. To check if a String is empty, and has not been assigned to any value (even null) to it, we use a method of String class called ‘isEmpty()’
Code Snippet:
public static void main(String[] args)
{
String s1 ="Grotechminds";
String s2="";
boolean b1=s1.isEmpty();
boolean b2=s2.isEmpty();
System.out.println("Is the given variable "+s1+" of String data type is emply? "+b1);
System.out.println("Is the given variable "+s2+" of String data type is emply? "+b2);
}
Output:
Is the given variable Grotechminds of String data type is emply? false
27. To check if a String is blank, and has been assigned with only white spaces, we use a method of String class called ‘isBlank()’
Code Snippet:
static void main(String[] args)
{
String s1 ="Grotechminds";
String s2=" ";
boolean b1=s1.isBlank();
boolean b2=s2.isBlank();
System.out.println("Is the given variable "+s1+" of String data type is blank? "+b1);
System.out.println("Is the given variable "+s2+" of String data type is blank? "+b2);
}
Output:
Is the given variable Grotechminds of String data type is blank? false
Is the given variable of String data type is blank? true
- To match the desired String exactly with a regular expression (describes the format of a phone number, format of a date, etc.,), we use a method of String class called ‘matches(String regex)’
Code Snippet:
public static void main(String[] args)
{
String s1 = "2025-01-01";
String s2 = "1-1-2025";
String rexp = "^\\d{4}-\\d{2}-\\d{2}$";
boolean b1 = s1.matches(rexp);
boolean b2 = s2.matches(rexp);
System.out.println("Is the given date "+s1+" matches with the regular expression? "+b1);
System.out.println("Is the given date "+s2+" matches with the regular expression? "+b2);
}
Output:
Is the given date 2025-01-01 matches with the regular expression? true
Is the given date 1-1-2025 matches with the regular expression? false
- To add or remove indentation at the beginning of the String value, we use a method of String class called ‘indent(int n)’
Code Snippet:
public static void main(String[] args)
{
String s1 ="Grotechminds";
String s2 =" Grotechminds";
int i=3;
String b1=s1.indent(i);
String b2=s2.indent(-i);
System.out.println("The intended value of string "+s1+" for "+i+" indents is : "+b1);
System.out.println("The intended value of string "+s2+" for "+-i+" indents is : "+b2);
}
Output:
The intended value of string Grotechminds for 3 indents is : Grotechminds
The intended value of string Grotechminds for -3 indents is : Grotechminds
- To repeat a string as many numbers of times within the range of int data type, we use a method of String class called ‘repeat(int count)’
Code Snippet:
public static void main(String[] args)
{
String s1 ="Grotechminds ";
int i=3;
String b1=s1.repeat(i);
System.out.println("The repeated value of string "+s1+" for "+i+" times is : "+b1);
}
Output:
The repeated value of string Grotechminds for 3 times is : Grotechminds Grotechminds Grotechminds
Also Read : learn java math class with methods
String functions play a very important role in the manipulation of any initialized value declared with the String data type, for any Java developer or an automation tester who uses Java for scripting. Knowing how to utilize the above-mentioned methods of the String class can be an advantage to make more efficient scripts, especially for Automation testers. With the given examples for each of the String functions above, we can be confident to perform our desired action on any String variable or an object of the String class.
Consult Us