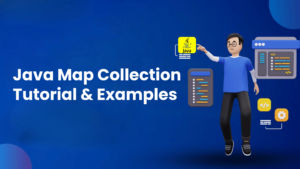
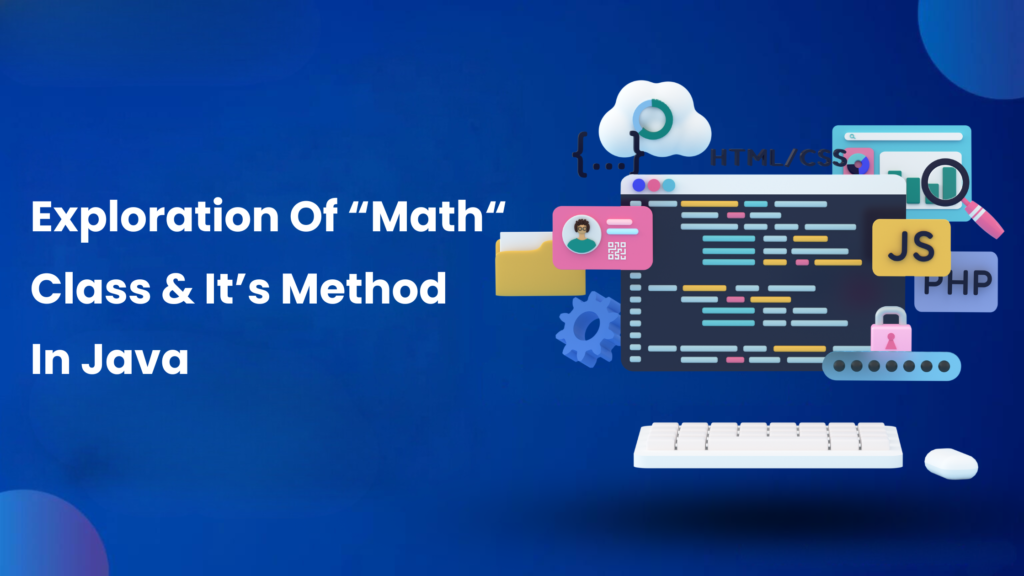
Exploration of ‘Math’ Class and its methods in Java
‘Math’ is one of the predefined classes in Java, which comes from the ‘java.lang’ package. To use the various methods of math class, we don’t have to create an instance(object)of the ‘Math’ class because of the static nature of those methods. To call the methods of the ‘Math’ class, we can call them by their class name (Math.addExact(int x, int y)). If the output value that is returned by a method in the ‘Math’ class is beyond the range of the return type, those methods throw ‘ArithmeticException’.
- ’ Math.addExact(int x, int y)’
This method returns the sum of two numbers of int data type.
Program:
public static void main(String[] args)
{
int x = 10;
int y = 20;
System.out.println("The sum of two numbers " + x + " and " + y + " = " + Math.addExact(x, y));
}
Output:
The sum of two numbers 10 and 20 = 30
- ’’ Math.subtractExact(int x, int y)’
This method returns the difference of two numbers of int data type.
Program:
public static void main(String[] args)
{
int x = 20;
int y = 10;
System.out.println("The difference of two numbers " + x + " and " + y + " = " + Math.subtractExact(x, y));
}
Output:
The difference of two numbers 20 and 10 = 10
- ’ Math.multiplyExact(int x, int y)’
This method returns the product of two numbers of int data type.
Program:
public static void main(String[] args)
{
int x = 10;
int y = 20;
System.out.println("The product of two numbers " + x + " and " + y + " = " + Math.multiplyExact(x, y));
}
Output:
The product of two numbers 10 and 20 = 200
- ’ Math.floorDiv(int x, int y)’
This method returns the quotient of the division of two numbers of int data type.
Program:
public static void main(String[] args)
{
int x = 20;
int y = 10;
System.out.println("The quotient of the division of two numbers " + x + " and " + y + " = " + Math.floorDiv(x, y));
}
Output:
The quotient of the division of two numbers 20 and 10 = 2
- ‘Math.max(int a, int b)’
This method returns the maximum of two numbers of int data type.
Program:
public static void main(String[] args)
{
int x = 10;
int y = 20;
System.out.println("The maximum of two numbers " + x + " and " + y + " = " + Math.max(x, y));
}
Output:
The maximum of two numbers 10 and 20 = 20
- ‘Math.min(int a, int b)’
This method returns the minimum of two numbers of int data type.
Program:
public static void main(String[] args)
{
int x = 10;
int y = 20;
System.out.println("The minimum of two numbers " + x + " and " + y + " = " + Math.min(x, y));
}
Output:
The minimum of two numbers 10 and 20 = 10
- ‘Math.pow(double a, double b)’
This method returns the value of the first argument of the double data type raised to the power of the second argument of the double data type.
Program:
public static void main(String[] args)
{
int x = 2;
int y = 10;
System.out.println("The value of " + x + " raised to the power of " + y + " = " + Math.pow(x, y));
}
Output:
The value of 2 raised to the power of 10 = 1024.0
- ‘Math.sqrt(double a)’
This method returns the square root of a number, of double data type.
Program:
public static void main(String[] args)
{
int x = 100;
System.out.println("The square root of "+x+" = " + Math.sqrt(x));
}
Output:
The square root of 100 = 10.0
- ‘Math.cbrt(double a)’
This method returns the cube root of a number, of double data type.
Program:
public static void main(String[] args)
{
int x = 125;
System.out.println("The cube root of "+x+" = " + Math.cbrt(x));
}
Output:
The cube root of 125 = 5.0
- ‘Math.random()’
This method returns a double value with a positive sign, greater than or equal to 0.0 and less than 1.0. A random value is generated every time this method is called.
Program:
public static void main(String[] args)
{
System.out.println("The random value >0.0 and less than 1.0 is "+Math.random());
System.out.println("The random value >0.0 and less than 1.0 is "+Math.random());
//since we used math.Random() twice, we will get two random values as output.
}
Output:
The random value >0.0 and less than 1.0 is 0.11543162701329845
The random value >0.0 and less than 1.0 is 0.49125555345217475
- ‘Math.abs(int a)’
This method returns the absolute value of an int value. If the argument is not negative, the argument is returned. If the argument is negative, the negation of the argument is returned(positive value is returned for negative value).
Program:
public static void main(String[] args)
{
int x = 10;
int y = -20;
System.out.println("The absolute value of " + x + " = " + Math.abs(x));
System.out.println("The absolute value of " + y + " = " + Math.abs(y));
}
Output:
The absolute value of 10 = 10
The absolute value of -20 = 20
- ‘Math.decrementExact(int a)’
This method returns the argument decremented by one, throwing an exception if the result overflows an int data type range. The input value gets reduced by one unit.
Program:
public static void main(String[] args)
{
int x = 10;
System.out.println("The decremented value of " + x + " = " + Math.decrementExact(x));
}
Output:
The decremented value of 10 = 9
- ‘Math.incrementExact(int a)’
This method returns the argument incremented by one, throwing an exception if the result overflows an int data type range. The input value gets increased by one unit.
Program:
public static void main(String[] args)
{
int x = 10;
System.out.println("The incremented value of " + x + " = " + Math.incrementExact(x));
}
Output:
The incremented value of 10 = 11
- ‘Math.negateExact(int a)’
This method returns the negation of the argument. Any positive int value will be converted into a negative value and any negative int value will be converted into a positive value of int data type.
Program:
public static void main(String[] args)
{
int x = 10;
int y = -10;
System.out.println("The negated value of " + x + " = " + Math.negateExact(x));
System.out.println("The negated value of " + y + " = " + Math.negateExact(y));
}
Output:
The negated value of 10 = -10
The negated value of -10 = 10
- ’ Math.floorMod(int x, int y)’
This method returns the remainder of the division of two numbers of int data type.
Program:
public static void main(String[] args)
{
int x = 20;
int y = 10;
System.out.println("The remainder of the division of two numbers " + x + " and " + y + " = " + Math.floorMod(x, y));
}
Output:
The remainder of the division of two numbers 20 and 10 = 0
Also Read : Difference Between Throw and Throws in Java
The ‘Math’ class in Java has a very useful and indispensable set of static methods for performing mathematical operations. Understanding and utilizing these methods can make our Java programs more powerful and efficient.
Consult Us