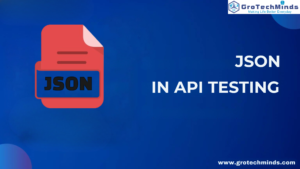
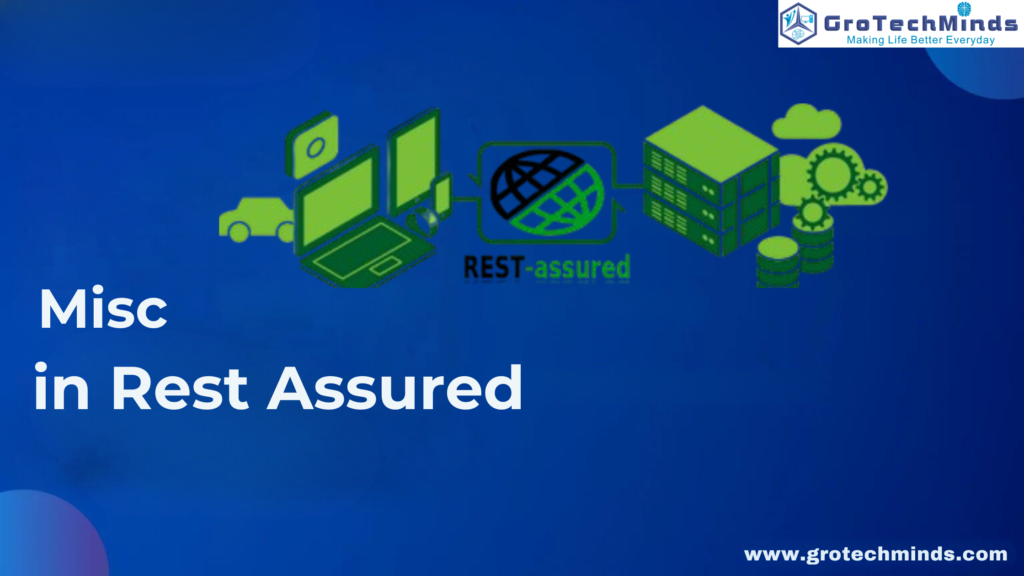
Miscellaneous in RestAssured
RestAssured is a powerful tool for automating REST API testing in Java. While most testers focus on core functionalities like sending requests and validating responses, RestAssured also provides miscellaneous (misc) features that enhance efficiency, flexibility, and security in API testing. Some key miscellaneous features include:
Form-Data- When we want to send anything apart from text data, we will send it through form-data.In the postman request body, we will select form-data as we need to pass the file. There are three fields that we need to fill:
- Key= f1
- Choose File
- Values- select the file “new file from local machine”
And then we need to send whatever details mentioned in the file will be reflected in the response body.In RestAssured, we can send the same though multipart () method through which we can send multiple files. In headers, Content-Type will be multipart/form-data.
Code Snippet:
public static void main(String[] args) {
File f1=new File("C:\\Users\\EKTA\\Downloads\\Test18thNov.txt");
RestAssured.baseURI="https://httpbin.org";
String response= given().log().all().headers("Content-Type","multipart/form-data")
.multiPart("file",f1)
.when().post("post")
.then().log().all().extract().response().asString();
System.out.println(response);
}
Output:
Request method: POST
Request URI: https://httpbin.org/post
Proxy:
Request params:
Query params:
Form params:
Path params:
Headers: Accept=*/*
Content-Type=multipart/form-data
Cookies:
Multiparts: ------------
Content-Disposition: form-data; name = file; filename = Test18thNov.txt
Content-Type: application/octet-stream
C:\Users\EKTA\Downloads\Test18thNov.txt
Body:
HTTP/1.1 200 OK
Date: Tue, 11 Mar 2025 07:35:27 GMT
Content-Type: application/json
Content-Length: 531
Connection: keep-alive
Server: gunicorn/19.9.0
Access-Control-Allow-Origin: *
Access-Control-Allow-Credentials: true
{
"args": {
},
"data": "",
"files": {
"file": "Hello all "
},
"form": {
},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip,deflate",
"Content-Length": "232",
"Content-Type": "multipart/form-data; boundary=iFQwbiaGBy5leJwKPBFfWwRne4_C2z",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/4.5.13 (Java/17.0.11)",
"X-Amzn-Trace-Id": "Root=1-67cfe7bf-283acff47b6e2e262725d759"
},
"json": null,
"origin": "122.161.48.140",
"url": "https://httpbin.org/post"
}
{
"args": {},
"data": "",
"files": {
"file": "Hello all "
},
"form": {},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip,deflate",
"Content-Length": "232",
"Content-Type": "multipart/form-data; boundary=iFQwbiaGBy5leJwKPBFfWwRne4_C2z",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/4.5.13 (Java/17.0.11)",
"X-Amzn-Trace-Id": "Root=1-67cfe7bf-283acff47b6e2e262725d759"
},
"json": null,
"origin": "122.161.48.140",
"url": "https://httpbin.org/post"
}
Screenshot:

Session Filter- Whenever we log in to the application, we have a specific session ID associated, which remains active till the time we are logged into the application. In RestAssured, if we want to execute a CRUD operation with the same session ID, we capture the session filter, which will give us the ability to perform multiple actions in the same session ID.Steps to be followed in RestAssured:
- We will create an object of SessionFilter @BeforeTest, which will give us the session ID.
- given() section, we will use method filter() where we will pass reference variable of the object created.
Code Snippet:
public class SessionFilterEx {
SessionFilter s;
@BeforeTest
public SessionFilter prerqst()
{
s=new SessionFilter();
return s;
}
@Test
public void test()
{
RestAssured.baseURI="https://httpbin.org";
String response=given().log().all().filter(s).when().get("get").then().log().all().assertThat().statusCode(200)
.extract().response().asString();
System.out.println(response);
}
Output:
{
"args": {
},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip,deflate",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/4.5.13 (Java/17.0.11)",
"X-Amzn-Trace-Id": "Root=1-67cff46d-3f1b37b52b4bd38b7380ee32"
},
"origin": "122.161.48.140",
"url": "https://httpbin.org/get"
}
{
"args": {},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip,deflate",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/4.5.13 (Java/17.0.11)",
"X-Amzn-Trace-Id": "Root=1-67cff46d-3f1b37b52b4bd38b7380ee32"
},
"origin": "122.161.48.140",
"url": "https://httpbin.org/get"
}
Screenshot:

Relaxed HTTPS Validation- When we want to access real-time API’s that are hosted by the https protocol, we need to bypass SSL(Secure Sockets Layer). When we use relaxed HTTPSValidation by default, they are bypassed. We are bypassing SSL authentication if our machine doesn’t have a valid SSL certificate we can access that API through relaxed HTTPSValidation.
Code Snippet:
public class RelaxedHttpsvalidation {
@Test
public void test()
{//for real api we have to bypass ssl (secured socket layer ) so we use relaxedHTTPSValidation
RestAssured.baseURI="https://httpbin.org";
String response=given().log().all().relaxedHTTPSValidation().get("get").then().log().all().assertThat().statusCode(200)
.extract().response().asString();
System.out.println(response);
}
}
Output:
{
"args": {
},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip,deflate",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/4.5.13 (Java/17.0.11)",
"X-Amzn-Trace-Id": "Root=1-67d164ca-32857efe5d291dd73908cae1"
},
"origin": "122.161.48.56",
"url": "https://httpbin.org/get"
}
{
"args": {},
"headers": {
"Accept": "*/*",
"Accept-Encoding": "gzip,deflate",
"Host": "httpbin.org",
"User-Agent": "Apache-HttpClient/4.5.13 (Java/17.0.11)",
"X-Amzn-Trace-Id": "Root=1-67d164ca-32857efe5d291dd73908cae1"
},
"origin": "122.161.48.56",
"url": "https://httpbin.org/get"
}
PASSED: mISC.RelaxedHttpsvalidation.test
Conclusion:
When we want to send anything apart from text data, we will send it through form-data. In RestAssured, we can send the same though multipart () method through which we can send multiple files. In headers, Content-Type will be multipart/form-data. In RestAssured, if we want to execute a CRUD operation with the same session ID, we will use the concept of session filter, which will give us the session ID. Then, we log in to the application with the same session ID. By following this tutorial and gaining hands-on experience, you are equipping yourself with valuable knowledge that can propel your career in Software Testing Cucumber to new heights. We are bypassing SSL authentication if our machine doesn’t have a valid SSL certificate still we can access that API through relaxed HTTPSValidation.
Also Read:
Consult Us