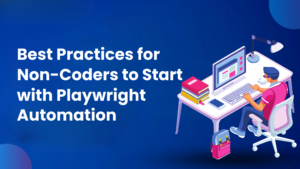
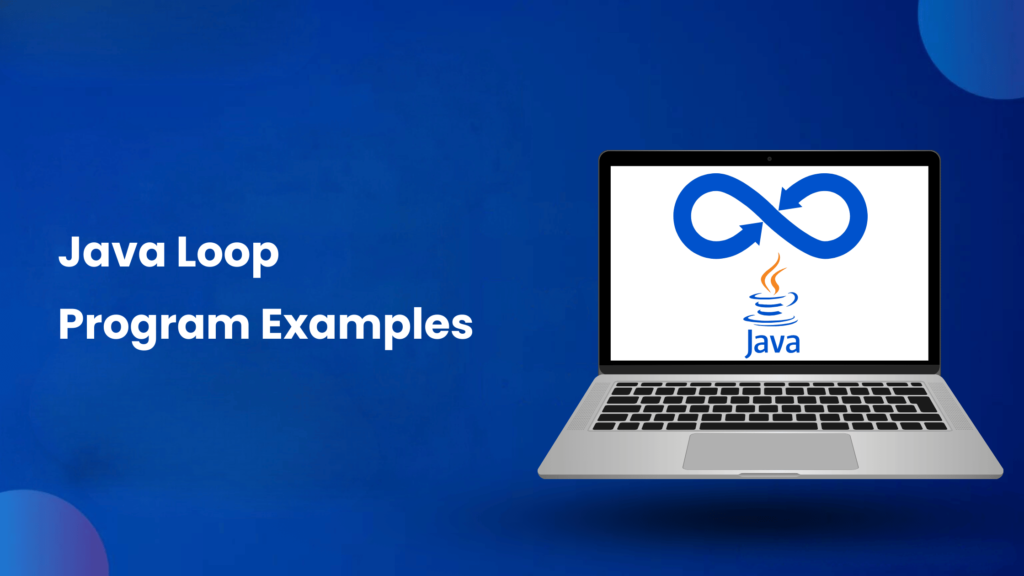
A Comprehensive Guide on the Basic For Loop in Java
Control structures are statements that allow us to manage the flow of execution of a block of code within a program. They determine how and when specific segments of code are executed, enabling you to implement complex logic and decision-making. For example, sequential execution (line by line), is the default natural order of execution in Java, whereas we have decision-making statements (if, if else, if else if, nested if), loop statements (for, while, do-while) and branching statements (break, continue) as well.
In Java, loops allow us to execute a block of statements in repetition. The for loop is one of the concise ways of writing the loop structure. It combines the initialization of variables, and conditions to specify the number of iterations, and either incrementing or decrementing in a single line, making it easy to read and understand.
The Syntax of the for loop is given as follows.
for (initialization; condition; increment/decrement)
{
‘loop body’
}
This is used to declare and initialize the loop control variable or variables. The loop control variable can also be declared globally and initialized inside the loop control statement.
Before each iteration of the loop, this condition is checked. If the condition is true, the loop continues. If it is false, the loop terminates.
After each iteration of the loop, this determines whether incrementing or decrementing should happen to the value of the loop variable (i.e.,) updating of the variable’s value.
The loop body is the executable code that is controlled by the control statement, in this case, it is the for loop.
For loop are of different types like nested for loop, enhanced for loop (or for each loop), and the basic for loop. The basic for loop and its variations will be discussed as the scope of this blog, and they are as follows.
The different variations of using the increment operator in a for loop are given as follows.
1. Using the increment operator ‘(the variable) ++’, we can increase the value of the variable by one unit on each iteration (e.g., i++).
public static void main(String[] args)
{
for (int i = 0; i < 5; i++)
{
System.out.println("This is iteration number " + i);
}
}

- Using the increment operator ‘(the variable) ++’ two times adjacent to each other separated by a comma, we can increase the value of the variable by two units on each iteration (e.g., i++,i++).
public static void main(String[] args)
{
for (int i = 0, j=0; i < 7; i++,i++)
{
System.out.println("The value of 'i' in this iteration is " + i+", and the iteration number is "+(j));
j++;
}
}

- Using one of the compound assignment operators ‘(the variable) += (count of increment)’, we can increase the value of the variable by desired units on each iteration (e.g., i+=10, increases the value of i by 10 on each iteration).
public static void main(String[] args)
{
for (int i = 0, j=0; i < 51; i+=10)
{
System.out.println("The value of 'i' in this iteration is " + i+", and the iteration number is "+(j));
j++;
}
}
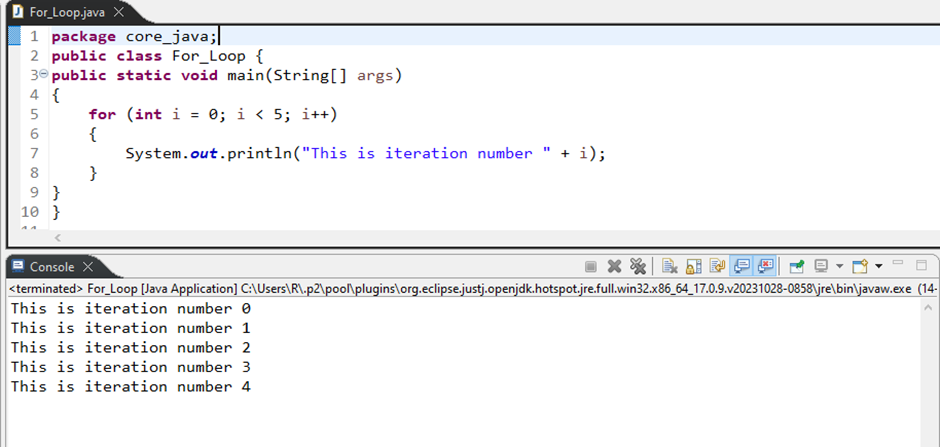
- Using the decrement operator ‘(the variable) –’, we can decrease the value of the variable by one unit on each iteration (e.g., i–).
public static void main(String[] args)
{
for (int i = 5, j=5; i > -1; i--)
{
System.out.println("The value of 'i' in this iteration is " + i+", and the iteration number is "+(j-i));
}
}
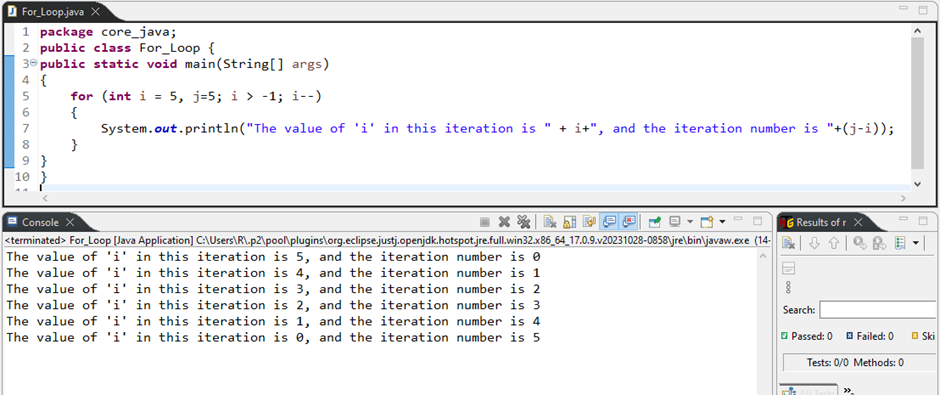
- Using the decrement operator ‘(the variable) –’ two times adjacent to each other separated by a comma, we can decrease the value of the variable by two units on each iteration (e.g., i–,i–).
public static void main(String[] args)
{
for (int i = 6, j=0; i > -1; i--,i--)
{
System.out.println("The value of 'i' in this iteration is " + i+", and the iteration number is "+(j));
j++;
}
}

- Using one of the compound assignment operators ‘(the variable) -= (count of decrement)’, we can decrease the value of the variable by desired units on each iteration (e.g., i-=10, decreases the value of ‘i’ by 10 on each iteration).
public static void main(String[] args)
{
for (int i = 50, j=0; i > -1; i-=10)
{
System.out.println("The value of 'i' in this iteration is " + i+", and the iteration number is "+(j));
j++;
}
}
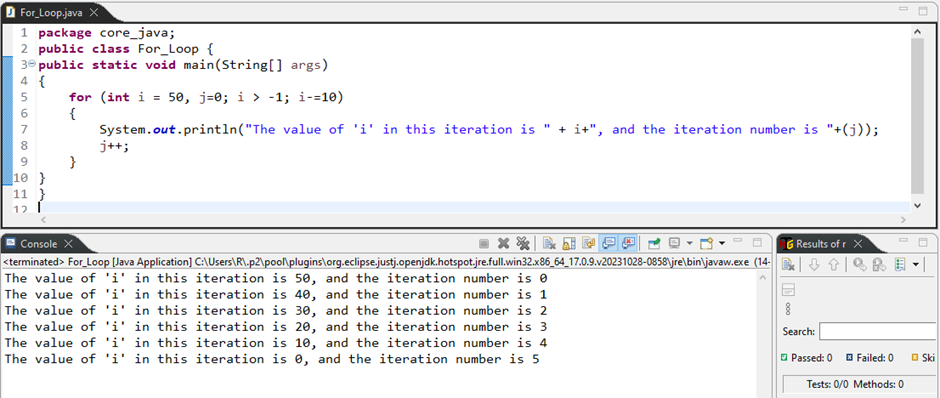
If the condition in the for loop is given in such a way that the loop condition will never get met (will never become false), the loop will run until its value runs out of the range of the data type of the variable given in the control statement.
- The infinite for loop with increment operator is as follows.
public static void main(String[] args) throws InterruptedException
{
for (byte i = 0, j=0; i > -1; i++)
{
System.out.println("The value of 'i' in this iteration is " + i+", and the iteration number is "+(j));
j++;
Thread.sleep(100);
}
}
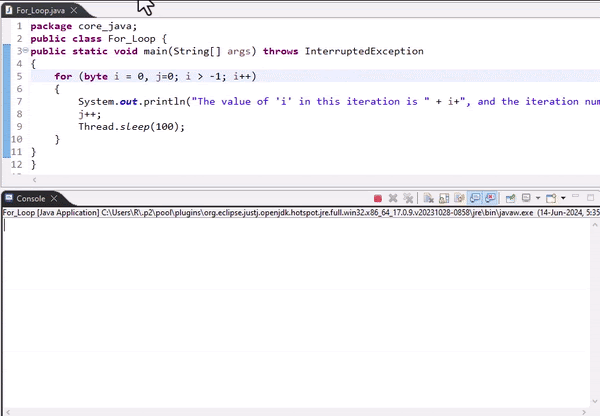
- The infinite for loop with decrement operator is as follows.
public static void main(String[] args) throws InterruptedException
{
for (byte i = 0, j=0; i < 1; i--)
{
System.out.println("The value of 'i' in this iteration is " + i+", and the iteration number is "+(j));
j++;
Thread.sleep(100);
}
}

If the condition in the for loop is given in such a way that the loop condition is empty, it will never get met (will never become false), the loop will never end until the human intervention, system shuts down or the memory runs out . The infinite for loop without initialization, condition, increment/decrement is as follows.
public static void main(String[] args) throws InterruptedException
{
for( ; ; )
{
System.out.println("This will never End");
Thread.sleep(50);
}
}

Problem Statement 1: Find out, the sum of the first 100 natural numbers using for loop.
Solution: To calculate the sum of the first 100 natural numbers using for loop, we iterate from 1 to 100 and add the value of the current iteration to the int variable ‘sum’ on every iteration.
public static void main(String[] args)
{
int sum=0;
int i;
for ( i=1; i<=100; i++)
{
sum=sum+i;
}
System.out.println("The sum of first "+(i-1)+" numbers is "+ sum);
}
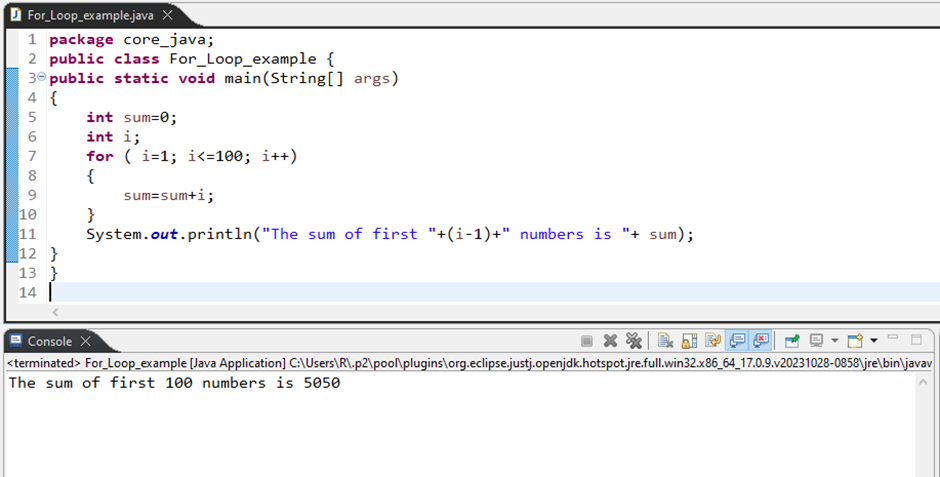
Problem Statement 2: Find out, the sum of all the even numbers from the first 100 natural numbers using for loop.
Solution: To calculate the sum of all the even numbers present in the first 100 natural numbers using a for loop, we iterate from 1 to 100 and increment every iteration by two units. We add the value of the variable ‘i’ of the current iteration to the int variable ‘sum’ on every iteration to get the sum of all the even numbers in the first 100 natural numbers.
public static void main(String[] args)
{
int sum=0;
for(int i=0;i<=100;i+=2)
{
sum=sum+i;
}
System.out.println("The sum of all the even numbers in the first 100 natural numbers is "+sum);
}
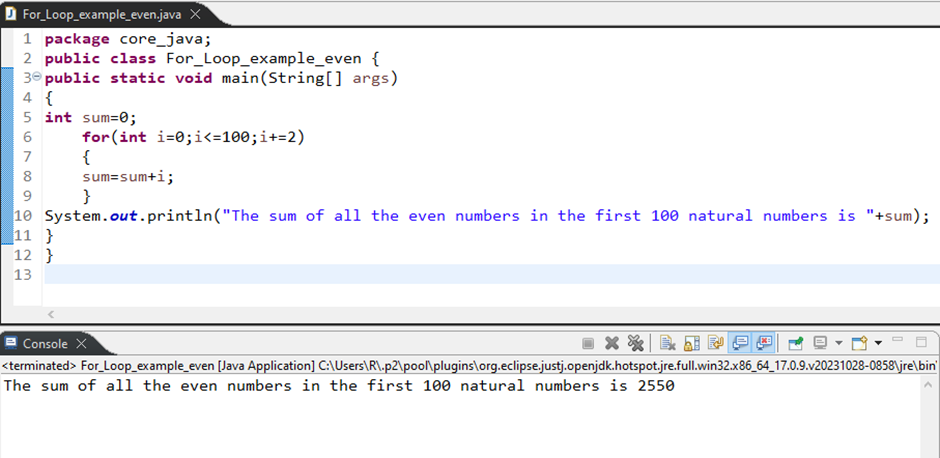
Problem Statement 3: Reverse the given String ‘Grotechminds’ using a for loop.
Solution: To reverse the index positions of the given String using a for loop, we iterate the char value of the String in the index position 0 to index position 11 (length of the string – 1). On the first iteration, we add the char value present in the last index position to the zeroth index position of the empty String. On the second iteration we add the char value present in the last but one’s index position to the first index position of the empty String, thereby reversing the string upon the completion of all the iterations.
public static void main(String[] args)
{
String gtm="Grotechminds";
String gtm_rev="";
for (int i=0; i
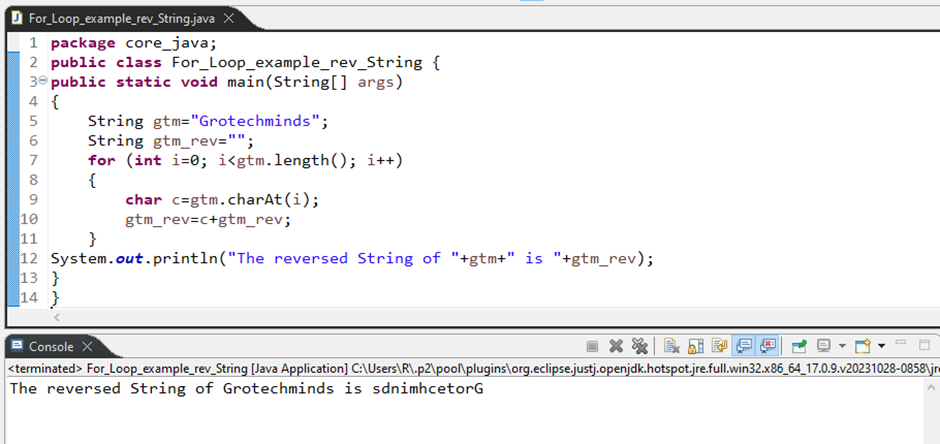
For loop is a reliable and very effective tool in Java for automating tasks like iterating through data, generating sequences, or performing complex calculations that involve iterations. Remember to practice, stay updated with the latest trends in Automation Software Testing Course,and maintain a positive attitude throughout your interview process. As we progress through the journey of application of our knowledge on loops, we get to know the possibilities loops offer.
Also read:
Consult Us