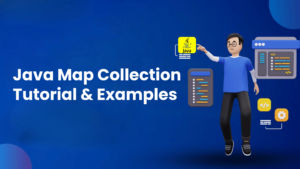
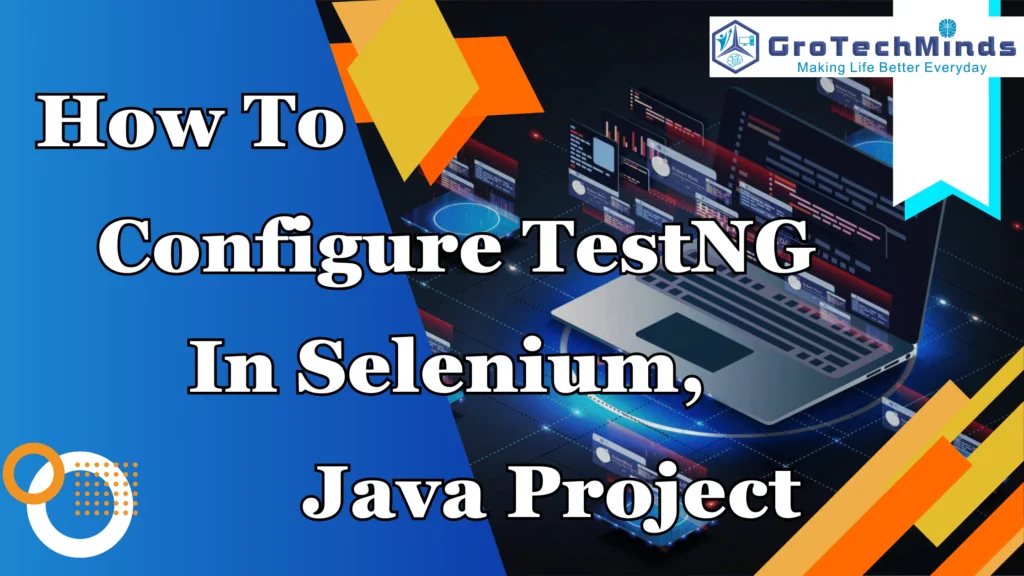
TestNG is a very powerful Java based and open source Automation framework with the help of which we can run multiple test cases together. It was developed by Cedric Beust whose objective was to create a robust Testing framework.In TestNG, NG stands for new generation. TestNG covers a wide variety of testing types like unit testing, functional testing, integration testing and system testing.TestNG is also useful in performing data driven testing, parallel testing and cross browser testing. Developers and testers use TestNG due to its usage in Grouping where we segregate different types of test scenarios, Assertions for comparing expected results with actual results.Of late,TestNG has become extremely popular because it helps in running multiple test cases in a systematic manner and ensures that the test scripts are easily readable. TestNG has a lot of annotations and parameters which act as a catalyst in ensuring smooth run of test scenarios.The various annotations according to their order of execution are @BeforeSuite,@BeforeTest@BeforeClass,@BeforeMethod,@Test, @AfterMethod,@AfterClass,@AfterTest and @AfterSuite.Among the list of various annotations, @Test is most important annotation and in the absence of @Test it is impossible for output to be displayed. The various TestNg parameters are priority,invocation count, enabled or disabled and timeout. The default priority of TestNG is always zero.In TestNG we can also run failed test cases as well as retry failed test cases and for retrying failed test cases we use an interface called IRetryAnalyzer. One unique feature of TestNg is that it provides a report called as emailable report which consists of detailed information about the total number of test cases run, total number of test scenarios passed, total number of test scenarios failed, total scenarios retried and total time in milli seconds for running of all scenarios.To print something on emailable report we use reporter.log(). Without the use of TestNg it will be difficult to run multiple test scripts together and we wont be able to get the report of total number of test cases ran, passed, failed and time duration of test scripts.
TestNG Annotations are piece of codes which are written inside the program to ensure smooth running of test scripts.TestNG annotations are written before every method of the program. Without TestNG annotations it will be impossible to run the tests. TestNG has various annotations which are categorized into before and after annotations. The before annotations are @BeforeSuite,@BeforeTest,@BeforeClass and @BeforeMethod. The after annotations are @After Method, @After Class, @After Test and@AfterSuite. The most important annotation among the before and after annotations is @Test. @Test annotation ensures that the program runs. All the other annotations are dependent on @Test. Without@Test the output of the program will not be displayed as a result of which Test suites wont run. Below is the code containing the 9 main annotations along with output showing the order of execution of the annotations.
package apollo;
import org.testng.annotations.AfterClass;
import org.testng.annotations.AfterMethod;
import org.testng.annotations.AfterSuite;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.BeforeMethod;
import org.testng.annotations.BeforeSuite;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
public class Chester {
@BeforeSuite
public void bs()
{
System.out.println("BeforeSuite");
}
@BeforeTest
public void bt()
{
System.out.println("BeforeTest");
}
@BeforeClass
public void bc()
{
System.out.println("BeforeClass");
}
@BeforeMethod
public void bm()
{
System.out.println("BeforeMethod");
}
@Test
public void testannotation()
{
System.out.println("TestAnnotation");
}
@AfterMethod
public void am()
{
System.out.println("AfterMethod");
}
@AfterClass
public void ac()
{
System.out.println("AfterClass");
}
@AfterSuite
public void as()
{
System.out.println("AfterSuite");
}
}
[RemoteTestNG] detected TestNG version 7.4.0
BeforeSuite
BeforeTest
BeforeClass
BeforeMethod
TestAnnotation
AfterMethod
AfterClass
AfterSuite
===============================================
Suite
Total tests run: 1, Passes: 1, Failures: 0, Skips: 0
===============================================
TestNG Parameters refers to the magnitudes or values that we set in all the TestNG annotations. With the help of TestNG parameters we will be able to run our Test suites in whichever manner we feel like to run. The various TestNG parameters are priority, enabled or disabled, invocation count and Timeout. Priority is a TestNG parameter which we assign in @Test annotation to ensure that the Test Suite runs in an orderly way.With priority parameter test suite will always run in ascending order. If Priority of a Test Suite is one, and other test suites have priority as 2 ,3 then test suite with priority 1 will run first followed by 2 and 3. Priority can be zero, negative value and positive value. Whenever priority is not assigned to any Test suite then the priority is by default taken as zero.Wherever multiple Test suites are present containing negative priority,default priority and positive priority then negative priority test suite will execute first followed by default priority and finally positive priority. Enabled or Disabled is used to ensure that those particular test suites executes which we want to run. If enabled=true and disabled = false then that test suite will run and if enabled=false and disabled=true then that test suite will not execute. Invocation count is used to run the same test suite multiple times. Timeout parameter is used to run the test suite within a particular time limit beyond which it shows timeout exception.
The Programming code along with output for all the TestNG parameters are:
a)priority :The output of the below code shows the order of execution due to priority parameter.
package testng;
import org.testng.annotations.Test;
public class TestNGPriorityParameter {
@Test(priority=2)
public void add()
{
System.out.println("add");
}
@Test(priority=3)
public void subtract()
{
System.out.println("subtract");
}
@Test(priority=1)
public void multiply()
{
System.out.println("multiply");
}
@Test
public void divide()
{
System.out.println("divide");
}
@Test
public void divide1()
{
System.out.println("divide1");
}
}
[RemoteTestNG] detected TestNG version 7.4.0
divide
divide1
multiply
add
subtract
PASSED: divide
PASSED: divide1
PASSED: multiply
PASSED: add
PASSED: subtract
===============================================
Default test
Tests run: 5, Failures: 0, Skips: 0
===============================================
===============================================
Default suite
Total tests run: 5, Passes: 5, Failures: 0, Skips: 0
===============================================
- b) enabled: The output of only that particular test case is displayed where enabled is assigned as true.
package testenabled;
import org.testng.Reporter;
import org.testng.annotations.Test;
public class TestEnabled {
@Test(enabled=true)
public void add()
{
System.out.println("hi");
Reporter.log("Heaven");
}
@Test(enabled=false)
public void sub()
{
System.out.println("hello");
}
}
[RemoteTestNG] detected TestNG version 7.4.0
hi
PASSED: add
===============================================
Default test
Tests run: 1, Failures: 0, Skips: 0
===============================================
===============================================
Default suite
Total tests run: 1, Passes: 1, Failures: 0, Skips: 0
===============================================
- c) Invocation Count
package testng;
import org.testng.annotations.Test;
public class SuryadeepInvocationCount {
@Test(invocationCount=10)
public void add()
{
System.out.println("addition");
}
}
[RemoteTestNG] detected TestNG version 7.4.0
addition
addition
addition
addition
addition
addition
addition
addition
addition
addition
PASSED: add
PASSED: add
PASSED: add
PASSED: add
PASSED: add
PASSED: add
PASSED: add
PASSED: add
PASSED: add
PASSED: add
===============================================
Default test
Tests run: 1, Failures: 0, Skips: 0
===============================================
===============================================
Default suite
Total tests run: 10, Passes: 10, Failures: 0, Skips: 0
===============================================
d) Timeout
package testng;
import org.testng.annotations.Test;
public class Timeout23 {
@Test(timeOut=3000)
public void add()
{
System.out.println("I am Timeout");;
}
}
[RemoteTestNG] detected TestNG version 7.4.0
I am Timeout
PASSED: add
===============================================
Default test
Tests run: 1, Failures: 0, Skips: 0
===============================================
===============================================
Default suite
Total tests run: 1, Passes: 1, Failures: 0, Skips: 0
===============================================
TestNG is a very powerful Java based and open source Automation framework with the help of which we can run multiple test cases together. It was developed by Cedric Beust whose objective was to create a robust Testing framework.In TestNG, NG stands for new generation. TestNG covers a wide variety of testing types like unit testing, functional testing, integration testing and system testing.TestNG is also useful in performing data driven testing, parallel testing and cross browser testing. Developers and testers use TestNG due to its usage in Grouping where we segregate different types of test scenarios, Assertions for comparing expected results with actual results.Of late,TestNG has become extremely popular because it helps in running multiple test cases in a systematic manner and ensures that the test scripts are easily readable. TestNG has a lot of annotations and parameters which act as a catalyst in ensuring smooth run of test scenarios.The various annotations according to their order of execution are @BeforeSuite,@BeforeTest@BeforeClass,@BeforeMethod,@Test, @AfterMethod,@AfterClass,@AfterTest and @AfterSuite.Among the list of various annotations, @Test is most important annotation and in the absence of @Test it is impossible for output to be displayed. The various TestNg parameters are priority,invocation count, enabled or disabled and timeout. The default priority of TestNG is always zero.In TestNG we can also run failed test cases as well as retry failed test cases and for retrying failed test cases we use an interface called IRetryAnalyzer. One unique feature of TestNg is that it provides a report called as emailable report which consists of detailed information about the total number of test cases run, total number of test scenarios passed, total number of test scenarios failed, total scenarios retried and total time in milli seconds for running of all scenarios.To print something on emailable report we use reporter.log(). Without the use of TestNg it will be difficult to run multiple test scripts together and we wont be able to get the report of total number of test cases ran, passed, failed and time duration of test scripts.
TestNG contains XML file which facilitates smooth execution of multiple test suites together. Before running any Test suite we first need to convert the test suite into XML file. The extension of Xml file is .xml. Running suites in Xml results in easy understanding of codes. We generate XML after configuring TestNG into eclipse. Xml file is extremely important in parallel testing where we run the different test suites simultaneously in different browsers and cross browser testing for running multiple suites in different browsers one by one.For running parallel and cross browser testing we make code changes in xml file. Xml file is also used in performing Assertion where expected and actual results are compared and Grouping where we segregate different types of testing and for each testing types we use a separate xml file where we do some code changes in the xml file. The Xml file contains various attributes and some of the attributes are xml version,suite name, test thread count, name, classes and class name as shown below in given figure.
Emailable reports are generated in TestNG after execution of all the test suites.Emailable reports are generally html reports. Automation testers share the emailable report to the team members to apprise them about the current status of the project. Emailable report contains all the informations about the status of the test suites like number of tests passed, number of tests failed, number of tests skipped, time duration of each test and number of times test suites retried. In an emailable report we can also display a message using Reporter.log() as shown in below figure.
TestNG is a very powerful Java based and open source Automation framework with the help of which we can run multiple test cases together. It was developed by Cedric Beust whose objective was to create a robust Testing framework.In TestNG, NG stands for new generation. TestNG covers a wide variety of testing types like unit testing, functional testing, integration testing and system testing.TestNG is also useful in performing data driven testing, parallel testing and cross browser testing. Developers and testers use TestNG due to its usage in Grouping where we segregate different types of test scenarios, Assertions for comparing expected results with actual results.Of late,TestNG has become extremely popular because it helps in running multiple test cases in a systematic manner and ensures that the test scripts are easily readable. TestNG has a lot of annotations and parameters which act as a catalyst in ensuring smooth run of test scenarios.The various annotations according to their order of execution are @BeforeSuite,@BeforeTest@BeforeClass,@BeforeMethod,@Test, @AfterMethod,@AfterClass,@AfterTest and @AfterSuite.Among the list of various annotations, @Test is most important annotation and in the absence of @Test it is impossible for output to be displayed. The various TestNg parameters are priority,invocation count, enabled or disabled and timeout. The default priority of TestNG is always zero.In TestNG we can also run failed test cases as well as retry failed test cases and for retrying failed test cases we use an interface called IRetryAnalyzer. One unique feature of TestNg is that it provides a report called as emailable report which consists of detailed information about the total number of test cases run, total number of test scenarios passed, total number of test scenarios failed, total scenarios retried and total time in milli seconds for running of all scenarios.To print something on emailable report we use reporter.log(). Without the use of TestNg it will be difficult to run multiple test scripts together and we wont be able to get the report of total number of test cases ran, passed, failed and time duration of test scripts.
The TestNG framework possesses a lot ofadvantages which are as follows:
- TestNG is a very flexible framework which contains a variety of annotations thus enabling smooth running of Test suites in a sequential manner.
- TestNG annotations are used to run same test cases multiple times using invocation count.
- With the help of TestNG we can perform data driven testing using data provider annotations.
- TestNG is used in Page Object Model which prevents code repeatability and allows code reusability thus saving our time.
- We can use TestNG in grouping to segregate different types of tests scenarios so that we can run any type of scenarios separately.
- TestNG contains XML files which helps in running multiple test suites together.
- In TestNG we can also run failed test cases.
- TestNG also help to generate emailable report which shows the number of test scenarios run, the number of test scenarios passed, the number of test scenarios failed, the time duration of test scenarios and the number of retries of scenarios.
- In TestNG we can perform parallel testing, cross browser testing by doing code changes in XML and using @Parameter annotations.
- TestNG contains data provider annotations to create multiple test data for testing single test scenario.
- In TestNg we can assign priority to every test methods.
- Open Eclipse then go to help and click on Eclipse Marketplace
Dialog Box will appear. In the search text field, type TestNG and press Enter.
The TestNG icon will appear and click on Install button beside TestNG icon
3. After installing TestNG right click on the new Java Project named Apple which is configured with Selenium server and from list of options click on Build Path. After clicking on Build Path click on Configure Build Path option.
4. After clicking on Configure Build Path option Properties for Apple dialog box will appear.
Then click on Add Library option present on right side.
After clicking Add Library option the Add Library dialog box will appear
and from list of library types select TestNG and click on Next button.
- After selecting TestNG the TestNG library will appear and then click on Finish Button.
6. After clicking on Finish Button TestNG will get added in the library section as shown below. Then click on Apply and Close Button.
- The TestNG is successfully into Apple Java Project configured as shown in below figure.
TestNG is a powerful framework which ensures smooth execution of Automation Test suites in selenium. It also generates html based testreports
called as emailable reports containing detailed status of the project in a very brief and lucid manner which testers share with Qa manager and other members of the team. TestNg enablesgrouping of different types of test cases which helps testers to execute the particular type of test cases instead of all the types of test cases. The console of the eclipse shows the detailed information of total tests run, the number of tests passed, failed and skipped along with the output of all the test methods run and all these will be shown in a sequential order based on the annotations and parameters used in programming code. Besides the console window there is a TestNg window which gives a mathematical representation of tests run, passed,failed and skipped along with the duration of each test run. All these features make TestNg a very important framework in Selenium for developers and testers.
Selenium is a powerful tool for automating web testing and can greatly improve the efficiency and accuracy of software testing. By enrolling in an automation testing course with Selenium, you can gain valuable skills and knowledge that will make you a more effective and efficient tester. The ability to write automated test scripts using Selenium can open up new career opportunities and set you apart as a top-notch tester in the industry. As technology continues to advance, the demand for skilled automation testers will only grow, making Selenium knowledge a valuable asset for any software testing professional. So, don’t hesitate to take the next step in your career by mastering automation testing with Selenium.
Consult Us