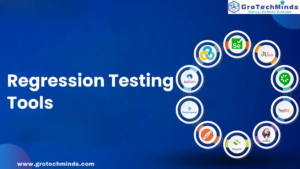
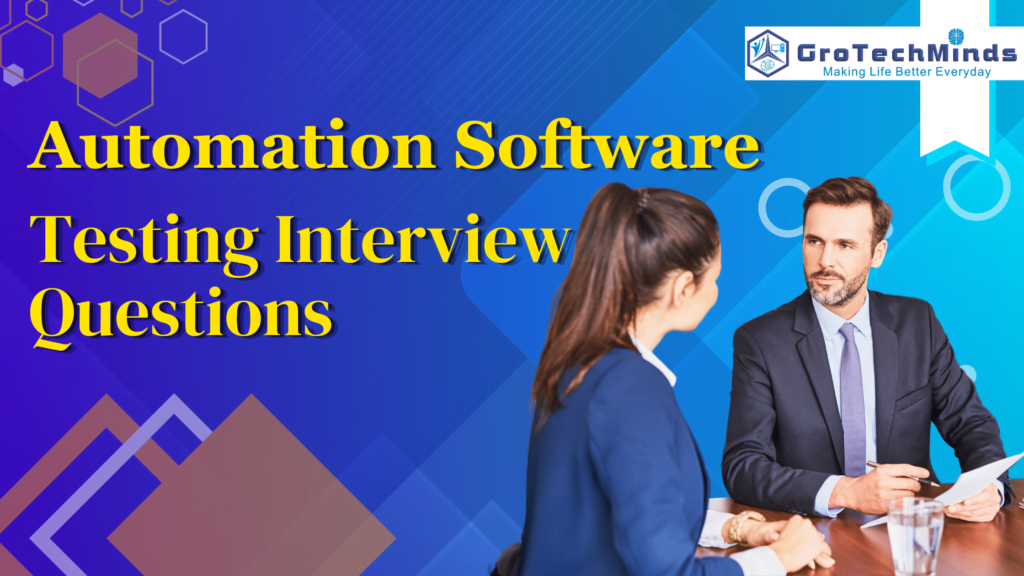
Public static void main(String[]args) is the main method in java. Main Method is the heart of the Java program. Without the main method it is not possible to run the java program and as a result output will not be displayed. In public static void main(String[]args) public is access specifier, static is modifier, void is return type, main is method name and (String[]args) is method parameter which is parameterized and String[]args is called String argument.
Developing multiple methods with same name but variation in argument list
or method parameters is called method overloading.We can overload both static and non static methods.We can also overload parameterized and non parameterized methods even though it is not required to use non parameterized methods for method overloading but it is necessary to use parameterized methods for method overloading. Below is the code snippet for method overloading
package package22;
public class Methodoverloading {
static void add(double c)
{
System.out.println("1");
}
static void add()
{
System.out.println("2");
}
static void add(int a)
{
System.out.println("3");
}
static void add(int a, String b)
{
System.out.println("4");
}
static void add(int a, char b)
{
System.out.println("5");
}
public static void main(String[] args)
{
add();
add(3.12);
add(300);
add(-13,"Hi");
add(12,'A');
}
}
Output:
2
1
3
4
5
Developing a method in the parent class with the same name and signature as in child class but with different implementation. The rules to achieve method overriding are:
- Relationships must be there between 2 classes.
- Both the classes should have the same method name.
- Both the methods should be non-static.
- Implementation of both the methods has to be different.
Below is the code snippet for method overriding
package pocker;
class Arithmeticoperator4
{
void add()
{
System.out.println("Addition of 3 numbers");
}
}
public class MethodOverriding extends Arithmeticoperator4{
void add()
{
System.out.println("Addition of 2 numbers");
super.add();
}
public static void main(String[] args) {
MethodOverriding b1 = new MethodOverriding();
b1.add();
}
}
Output:
Addition of 2 numbers
Addition of 3 numbers
The different access specifiers are public,private, default or package and protected.
The different methods we have in javaare
- Predefined methods
- User Defined Static Parameterized methods
- User Defined Static non parameterized methods
- User Defined Non Static Parameterized methods
- User Defined Non Static Parameterized methods
- User Defined Abstract methods
- Constructors are special types of methods used in Java.
- Constructor name will be the same as the class name.
- Constructors will never have return type.
- Constructors will always be non-static in nature.
- Constructors can be parameterized or non parameterized
Below is the code snippet for constructor
package pokerr;
public class Constructorssss {
Constructorssss()
{
System.out.println("hi");
}
Constructorssss( int i, int j)
{
int add= (i+j);
System.out.println(add);
}
public static void main(String[] args) {
new Constructorssss();
new Constructorssss(-128,127);
}
Output
hi
-1
In the above code snippet Constructorssss() is non parameterized constructor and Constructorssss(int i, int j) is non parameterized constructor.
Keywords are reserved words in Java which have their own meaning. Any keyword cannot be an identifier and any identifier cannot be a keyword. The list of keyword in java are abstract, assert, boolean,break,byte,case,catch,char,class
continue,default,do,double,else,enum,extends,final,finally,float,for,if, implements,import,instanceof,int,interface,long,native,new,null,package, private,protected, public, return,short,static, strictfp,super,switch, synchronised,this,throw, throws,transient,try,void,volatile,while.const,goto.
Data types to type of values that variables hold as well as kind of operations performed on the variables. The list of data types are byte,short,int,long,float,double,boolean,String,char.
The syntax of array is
datatype variablename[]=new datatype[array size];
The formula to calculate range of datatype is
-2 to the power of n-1 to (+2 to the power of n-1)-1 where n is the size in bits.
The default value of the boolean data type in the global variable is false.
Oracle Corporation owns java.
Yes java is a platform independent language because it has a byte code which makes it run on any device which has a java virtual machine.
The java keywords are abstract, assert, boolean,break,byte,case,catch,char,class
continue,default,do,double,else,enum,extends,final,finally,float,for,if,implements, import,instanceof,int,interface,long,native,new,null,package,private,protected, public,return, short, static,strictfp, super, switch,synchronised,this,throw, throws,transient,try,void,volatile,while.const,goto.
Local variables
Any variable that is declared inside a method is local variable.
The scope of local variable is from beginning to end of method.
Local variables cannot be distinguished between static and non static.
Local variables cannot be utilised unless it is initialized.
Local variables datatypes do not have default values.
Syntax: void add()
{
Int a=100;
}
Global variables
Any variable that is declared outside the method but inside the class is a global variable.
The scope of global variables will be from starting to end of class
Global variables can be distinguished between static and non-static.
Global variables can be utilised even if not initialised.
Global variables data types have default values.
Syntax: classGlobal
{
int a=100;
Void add()
{
System.out. println(a);
}
}
String functions are length(),concat(),substring(),contains(),equals(),
replaceAll(),split(),toUpperCase(),toLowerCase(),charAt(),indexof();
Yes we can overload static methods.
No Constructor will never have a return type.
Compilation checks for Compile Time Error, it mainly checks for syntax, rules and then translates the .java file to .class file.
Interpretation checks for the Run Time Error, it reads the code line by line, then executes and then translates the .class file to binary.
Interpretation only runs the program if it has the following
1.JDK (java development kit) – jdk are the library files which are required to run or write the program.
2.JRE (java runtime environment) – This has to be present in all the devices to run any java program.
3.JIT (just in time) – It is responsible for the conversion of .class file to binary
JVM (java virtual machine) – It is not physically present in the machine but is responsible for the execution of the program starting from the main method
Java is a platform independent language. Whatever code we are writing is human understandable language and it cannot be understood by the system. Extension of a class is .java file. To convert the .java file to binary there are 2 stages compilation and interpretation.
Compilation checks for Compile Time Error, it mainly checks for syntax, rules and then translates the .java file to .class file.
Interpretation checks for the Run Time Error, it reads the code line by line, then executes and then translates the .class file to binary.
- JDK (java development kit) – jdk are the library files which are required to run or write the program.
- JRE (java runtime environment) – This has to be present in all the devices to run any java program.
- JIT (just in time) – It is responsible for the conversion of .class file to binary
- JVM (java virtual machine) – It is not physically present in the machine but is responsible for the execution of the program starting from the main method
Find Element is used to find a single element. The return type is Web Element. Find Element gives no such element exception.
Syntax of FindElement:
WebElement flipcart= c1.findElement(By.xpath("//span[.='✕']"));
flipcart.click();
where c1 is the reference variable of the web driver interface.
Find Elements is used to find multiple elements. The return type is List<WebElement>. FindElements returns an empty list.
Syntax of FindElements:
List autosuggestion = c1.findElements(By.xpath("//form[@class='_2rslOn header-form-search OpXDaO']/ul/li"));
int noofautosuggestion = autosuggestion.size();
There are 8 locators in Selenium which are : Name, Class Name,tagName,xpath, CSS Selector, link text, partial link text, id.
Driver.close will close the parent browser or that particular browser which has control.
Driver.quit will close both parent and child browsers.
- It is a free tool available in the market.
- With the help of Selenium, we can automate testing in multiple programming languages.
- It can be automated in multiple operating systems. (Windows, MacOS, Ubuntu)
- It can be automated in multiple browsers.
- Selenium reduces the effort required in Manual testing to a very great extent and also there is less probability of errors compared to manual testing.
- Testing process is more smooth and reliable than manual testing.
- Selenium configured with TestNG enables reusability of test scripts.
- With the help of Selenium, we can Automate only web-based applications, not mobile and standalone applications. So for automation of mobile applications and standalone applications we need to use some additional tools.
- Selenium does not have any official documentation.As a result of which testers have to depend on other third party testing tools like TestNG for generation of test reports.
- We will not be able to automate image, OTP, Scan code Captcha, and Barcode using Selenium.
- Test Scripts made through selenium needs to be updated every time due to constant updates of web applications.
- Through Selenium we cannot perform image based testing like screenshot comparison, which is needed for testing visual aspect of software application.
- Wherever there is no scope for code reusability, creation of Test Cases takes more time due to writing of programming code for every test cases
Yes we have and that is driver.navigate().refresh();
For maximize we use driver().manage().window().Maximize().
For minimize we use driver().manage().window().Minimize().
Selenium consists of various components that help testers perform automated testing like parallel testing, cross-browser testing on different browsers as well as record and play those actions. The various components of Selenium are:
- Selenium IDE
- Selenium Web Driver
- Selenium Grid
- Selenium Remote RC
Implicit wait
- In Implicit wait we don’t provide any condition
- If program does not run within prescribed time it will give no such element exception
- Implicit wait is only applicable to 2 methods of selenium find element and find elements.
- Once written implicit wait will be applicable throughout the program for find element and find elements.
Syntax:driver.manage().timeouts().implicitlyWait(Duration.of any timeunit( magnitude of duration));
Explicit wait
- In Explicit wait we pass conditions.
- If program does not run within prescribed time it will give timeout exception
- Explicit wait helps to synchronize any method and before that method we write explicit wait syntax.
- Explicit wait will only be applicable to those methods of selenium before which we use the explicit wait syntax.Once written only applicable for next line.
- Syntax: WebDriverWait wait =new WebDriverWait(driver,Duration.ofany time unit(magnitude of duration));
wait.until(ExpectedConditions.titleContains( ));
wait.until(ExpectedConditions.titleIs( ));
GetWindowhandle will always give the parent browser id. The return type is string Syntax of GetWindowHandle: String parentid= c1.getWindowHandle();
GetWindowhandles will give both parent and child browser id . The return type is Set. Syntax of GetWindowHandles: Set parentandchild= c1.getWindowHandles();
System.out.println(parentandchild);
There is nothing called the best locator. The use of locators totally depends upon the requirements as well as type of element to be tested in the application. For example we can use ID and name locator for various text fields and text area fields. We can use linktext and partial link text for various links components.Tagname is used mostly for links and dropdown handling because link and drop down have default tagname. Css selector is used for components having either tagname and id, tagname and class name,tagname and attribute name and combination of tagname,classname,attributename and attribute value. Classname is mostly used in those situations where none of locators work. Xpath even though we use for automation testing in most of the cases but still it does not work in some cases and as a result of which all 8 locators are important and every locator is used as per requirements.
To find out the coordinates of the x and y axis of any element we use get location class. The return type of the get location class is point and we have to import point from only Selenium.org.
The below is the code snippet for knowing of x and y coordinates:
package practice;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.Point;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class MKT
{
WebDriver driver;
@Test
public void one()
{
driver=new ChromeDriver();
driver.manage().window().maximize();
driver.get("https://www.amazon.in");
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
WebElement a1=driver.findElement(By.linkText("Canada"));
Point p1=a1.getLocation();
int x=p1.getX();
int y=p1.getY();
System.out.println(x);
System.out.println(y);
}
}
We first upcast the driver to javascript executor interface and then utilise its method called as execute script. Below is the code snippet for scrolling down:
package practice;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.Point;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class MKT
{
WebDriver driver;
@Test
public void one()
{
driver=new ChromeDriver();
driver.manage().window().maximize();
driver.get("https://www.amazon.in");
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
WebElement a1=driver.findElement(By.linkText("Canada"));
Point p1=a1.getLocation();
int x=p1.getX();
int y=p1.getY();
JavascriptExecutor jse= (JavascriptExecutor) driver;
jse.executeScript("window.scrollBy("+x+","+y+")");
}
}
The types of popup we have are JavaScript Popup, File Upload Popup, File Download PopUp, Simple Alert, Confirmation Alert,Prompt Alert, Authentication Alert.
Dragging and dropping of any element can be done using Actions class. Actions class Object will have a driver in it which is the reference variable for webdriver interface and it means that we are trying to call the parametrised constructor present in actions class.
Syntax: Actions a1= new Actions(driver);
a1.dragAnddrop(E1,E2).perform();
Where E1 and E2 are 2 parameters of drag and drop method.
The code snippet of drag and drop is shown below:
package practice;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.interactions.Actions;
import org.testng.annotations.Test;
public class MKT
{
WebDriver driver;
@Test
public void one()
{
driver=new ChromeDriver();
driver.manage().window().maximize();
driver.navigate().to("https://grotechminds.com/drag-and-drop/");
WebElement drag=driver.findElement(By.id("drag7"));
WebElement drop=driver.findElement(By.id("div2"));
Actions a1=new Actions(driver);
a1.dragAndDrop(drag, drop).perform();
}
}
Navigation methods are Navigate to,Navigate refresh,Navigate back,Navigate forward. Navigate to is used for launching any web page or website. Navigate refresh is used to refresh something after performing any activities on the website. Navigate back is used to go back to the previous web page. Navigate forward is used to proceed to the next page.
The below is the code snippet of navigation methods:
package practice;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class MKT
{
WebDriver driver;
@Test
public void one() throws InterruptedException
{
driver=new ChromeDriver();
driver.manage().window().maximize();
driver.navigate().to("https://grotechminds.com/");
Thread.sleep(2000);
driver.navigate().back();
Thread.sleep(2000);
driver.navigate().forward();
Thread.sleep(2000);
driver.navigate().refresh();
}
}
This can be done using a method called isDisplayed(). isDisplayed is one of abstract methods of Web Element interface.The return type of is displayed is boolean. If boolean value is false the element is not displayed but if boolean value is true then element is displayed and as a result required action can be performed on the element.
The below is the code snippet for is Displayed:
package practice;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class MKT
{
WebDriver driver;
@Test
public void one()
{
driver=new ChromeDriver();
driver.manage().window().maximize();
driver.get("https://www.amazon.in/ap/signin/");
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
WebElement checkbox=driver.findElement(By.name("email"));
boolean b1=checkbox.isDisplayed();
System.out.println(b1);
}
}
This can be done using a method called isEnabled(). isEnabled is one of abstract methods of Web Element interface.The return type of is displayed is boolean. If boolean value is false the element is not enabled but if boolean value is true then element is enabled and as a result required action can be performed on the element.
The below is the code snippet for is Enabled:
package practice;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class MKT
{
WebDriver driver;
@Test
public void one()
{
driver=new ChromeDriver();
driver.manage().window().maximize();
driver.get("https://www.amazon.in/ap/signin/");
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
WebElement checkbox=driver.findElement(By.name("email"));
boolean b1=checkbox.isEnabled();
System.out.println(b1);
}
}
This can be done using a method called is Selected(). Is Selected is one of abstract methods of Web Element interface.The return type of is displayed is boolean. If boolean value is false the element is not displayed but if boolean value is true then element is Selected and as a result required action can be performed on the element.
The below is the code snippet for is Selected:
package practice;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class MKT
{
WebDriver driver;
@Test
public void one() throws InterruptedException
{
driver=new ChromeDriver();
driver.manage().window().maximize();
driver.get("https://grotechminds.com/is-selected/");
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
WebElement checkbox=driver.findElement(By.id("vehicle2"));
checkbox.click();
Thread.sleep(2000);
boolean b1=checkbox.isSelected();
System.out.println(b1);
}
}
Navigation methods are Navigate to,Navigate refresh,Navigate back,Navigate forward. Navigate to is used for launching any web page or website. Navigate refresh is used to refresh something after performing any activities on the website. Navigate back is used to go back to the previous web page. Navigate forward is used to proceed to the next page.
There are two methods clear the values
By using key functionality Keys.BackSpace
WebElement newpassword = c1.findElement(By.name("reg_passwd__"));
newpassword.sendKeys("eheheheh");
newpassword.sendKeys(Keys.CONTROL+"a");
newpassword.sendKeys(Keys.BACK_SPACE);
By using Clear method
WebElement newpassword = c1.findElement(By.name("reg_passwd__"));
newpassword.sendKeys("eheheheh");
newpassword.sendKeys(Keys.CONTROL+"a");
newpassword.clear();
In conclusion, mastering the art of test automation courses is essential for staying competitive in the rapidly evolving tech industry. By familiarising yourself with the top automation testing interview questions and honing your skills in scripting languages and testing frameworks, you will be well-equipped to excel in your future job opportunities. Investing in a comprehensive test automation course can provide you with the knowledge and expertise needed to stand out in the job market and make meaningful contributions to your organisation. Keep learning, practising, and staying updated with the latest tools and techniques to lead the way in the dynamic field of test automation.
Consult Us