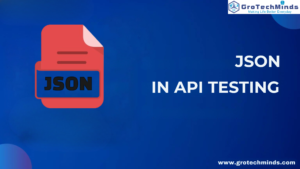
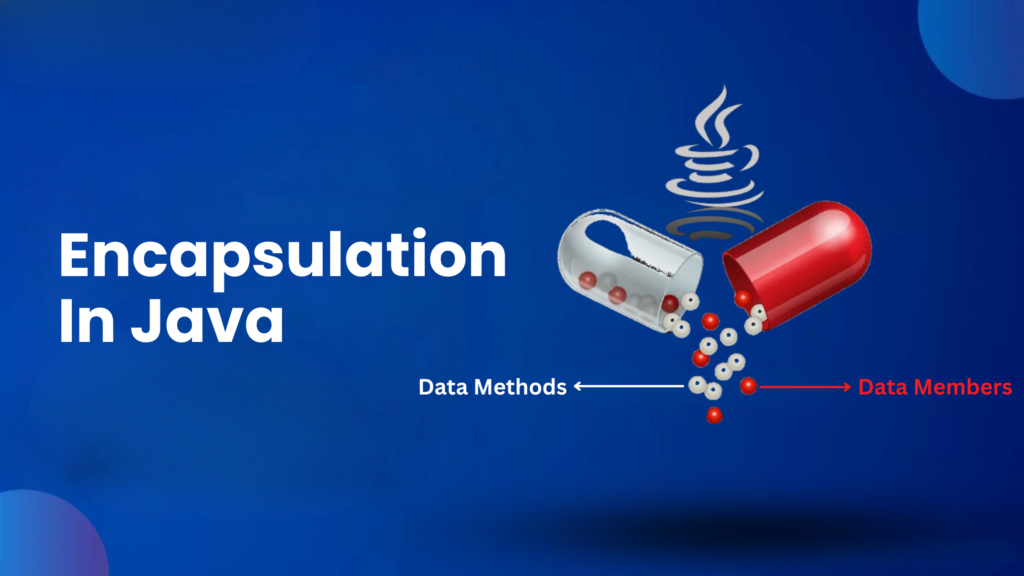
Encapsulation in Java
What is Encapsulation?
Encapsulation is the process of hiding the implementation or making any variable private and giving indirect access using getter and setter methods. It is a powerful principle in Java that promotes better organization, security, and maintainability of code.
Why do we make any information private?
We make any sensitive information private so no one can access it directly. We give indirect access by using getter and setter methods.
What are the benefits of Encapsulation?
There are some of the benefits of encapsulation in Java which are listed below-
- Sensitive data can be protected from unauthorized access and modification.
- Encapsulation protects the integrity of the data.
- Encapsulation enhances code reusability as components can be reused across different programs.
- Modifications to the encapsulation code can be made with minimal impact on other parts of the program.
- Encapsulation simplifies debugging and problem-solving.
- Encapsulation helps achieve abstraction by exposing only the necessary features and behavior of a system, making it easier to use and maintain.
- Encapsulation ensures that only the essential features and behaviors of a system are exposed, simplifying usage and maintenance.
- Encapsulation enables adaptable systems that can evolve with changing business needs.
Getter and setter methods
- Both access specifiers of get and set methods should be public.
- The set method is used to set the value.
- The get method is used to get the value.
Syntax for get method
void getVariable()
{
}
Syntax for set method()
void setVariable(Datatype variable_name)
{
}
Query Statement:
Write a program on encapsulation where age is private.
Method 1
In this program, we will write getter and setter methods.
Code Snippet:
package encapsulation;
public class Encapsulation {
private int age=18;
public int getAge()
{
return age;
}
public void setAge(int age)
{
this.age=age;
}
public static void main(String[] args) {
Encapsulation e1= new Encapsulation();
e1.setAge(25);
System.out.println(e1.getAge());
}
}
Screenshot:
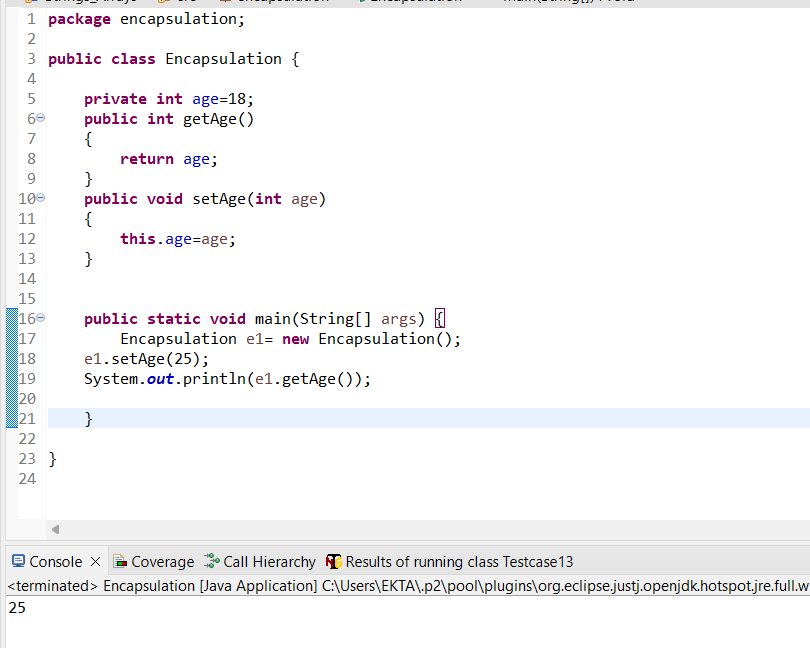
Method 2
In this program, we will auto-generate getter and setter methods.
Step 1:
We will right-click and select the source and Generate Getter and Setter.
Screenshot:
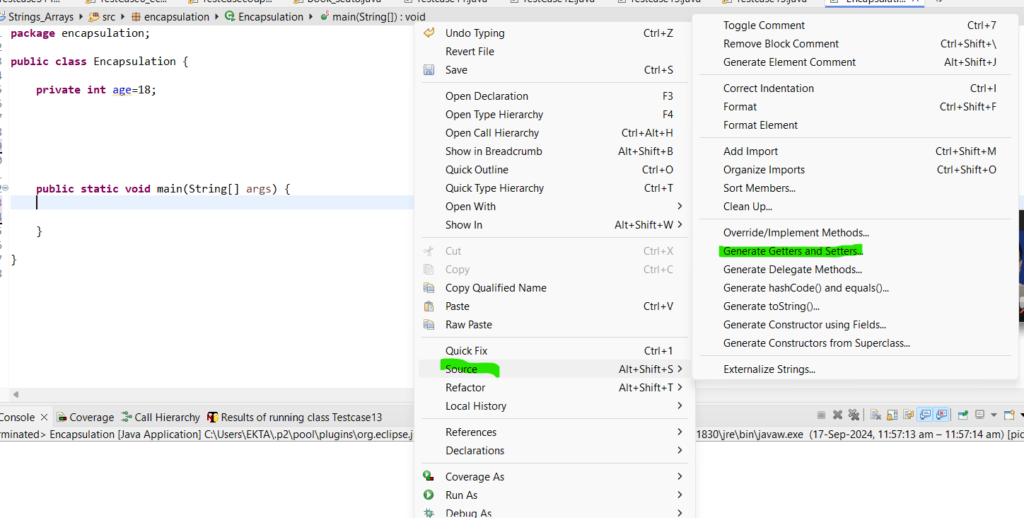
Step 2:
Then check the age box and click on Generate.
Screenshot:
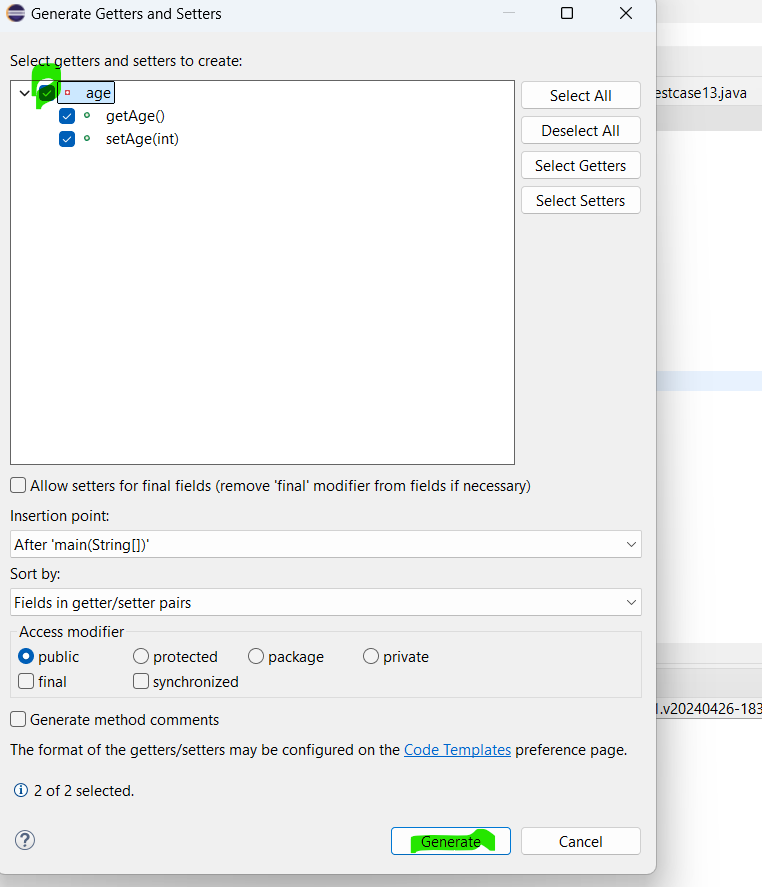
Step 3:
Then we can see getter and setter methods in the program.
Screenshot:

Step 4:
We now create an object of the class and call setter method to set the value and getter method to get the value.
Code Snippet:
package encapsulation;
public class Encapsulation {
private int age=18;
//getter method
public int getAge() {
return age;
}
//setter method
public void setAge(int age) {
this.age = age;
}
public static void main(String[] args) {
Encapsulation e1=new Encapsulation();//creating object
e1.setAge(25);//set the age
System.out.println(e1.getAge());//get the age
}
Screenshot:

Conclusion:
Encapsulation in Java is a powerful mechanism that enhances our code’s security, maintainability, and flexibility as it restricts direct access to some of an object’s components and provides controlled access through public methods (getters and setters). Remember to practise, stay updated with the latest trends in Automation Software Testing Course,and maintain a positive attitude throughout your interview process. Developers can create more robust, modular, and flexible applications using encapsulation.
Also read:
Consult Us