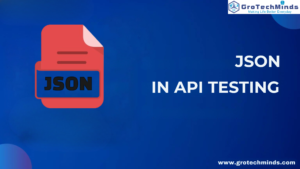
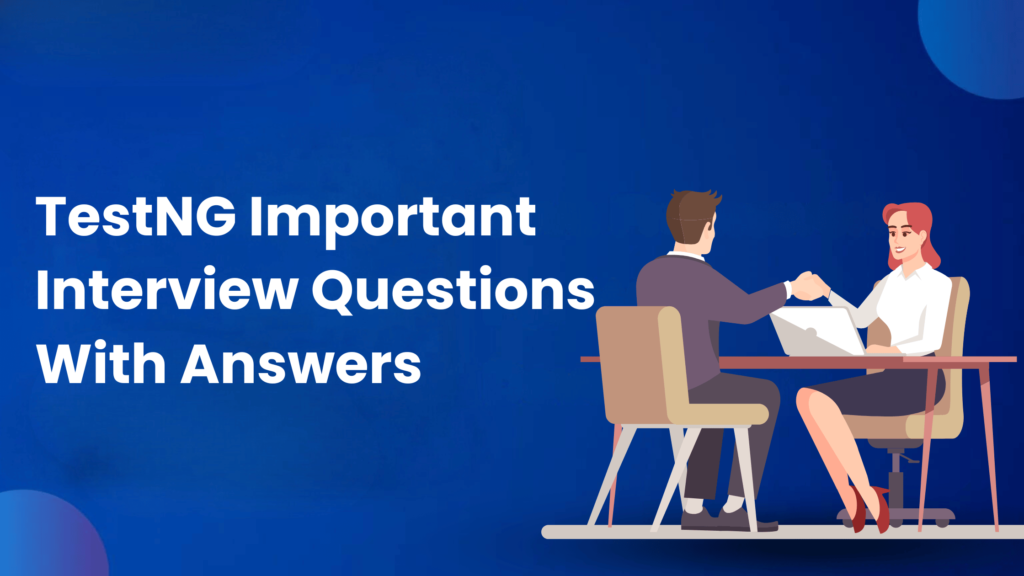
TestNG Important Interview Questions With Answers
1.What is TestNG?
TestNG stands for Test Next Generation. It is a tool that can be integrated with Selenium to make power Test Automation Framework which enables Testers to perform below things in easier way
- Support Data Driven Testing
- Support for Parameter(This allows Parallel Testing)
- Allows to generate report
- Allows to run many test cases together
2.What is a Test Suite?
Two or more Test Cases combine together to form a Test Suite.
3.What are Annotations in TestNG?
Annotations are used to control the execution of test methods and configure test cases to decide which one has to execute first and which one to execute later.
4.How many annotations do we have in TestNG?
- @Test
- @BeforeSuite
- @AfterSuite
- @BeforeClass
- @AfterClass
- @BeforeMethod
- @AfterMethod
- @BeforeTest
- @AfterTest
5. Is TestNG only for Software Testers?
No TestNG is not just for Software Testers but Developers can also utilize it to do Unit regression Testing.
6. What are the advantages of TestNG in Selenium?
- With the help of TestNG we can not just run Automation Test Cases but we can also generate reports for the same.
- We can also run multiple test cases together.
- We can group test cases based on Testing types.
7.How to run a Test in TestNG?
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class dummyclass {
@Test
public void login()
{
ChromeDriver driver=new ChromeDriver();
driver.get("https://www.google.com/");
}
@Test
public void logout()
{
ChromeDriver driver=new ChromeDriver();
driver.get("https://www.google.com/");
}
}
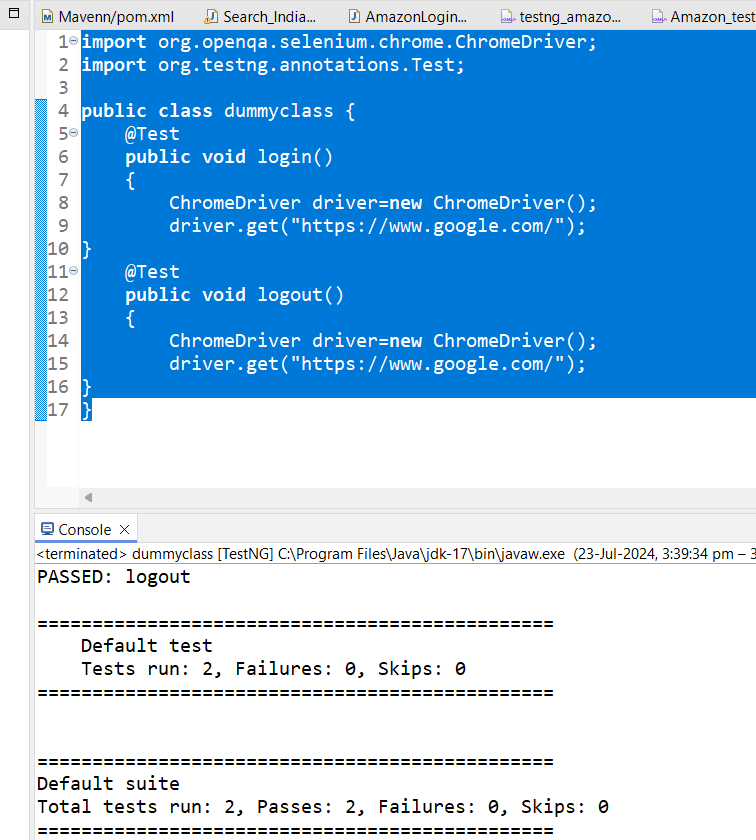
8.How to run a Test Suite in TestNG?
Step 1: Create individual classes with @Test
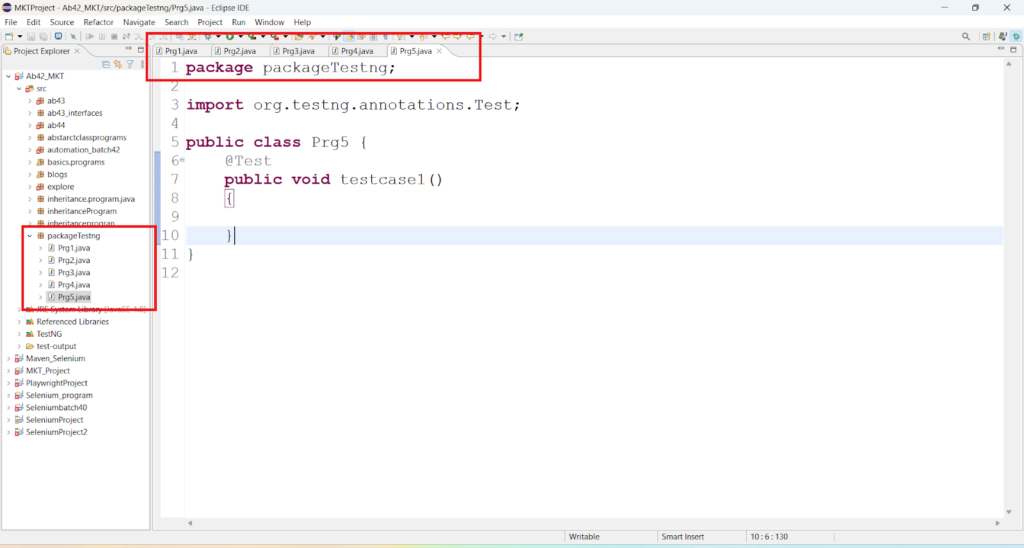
Step 2: Right click on given package->TestNG->Convert to TestNG
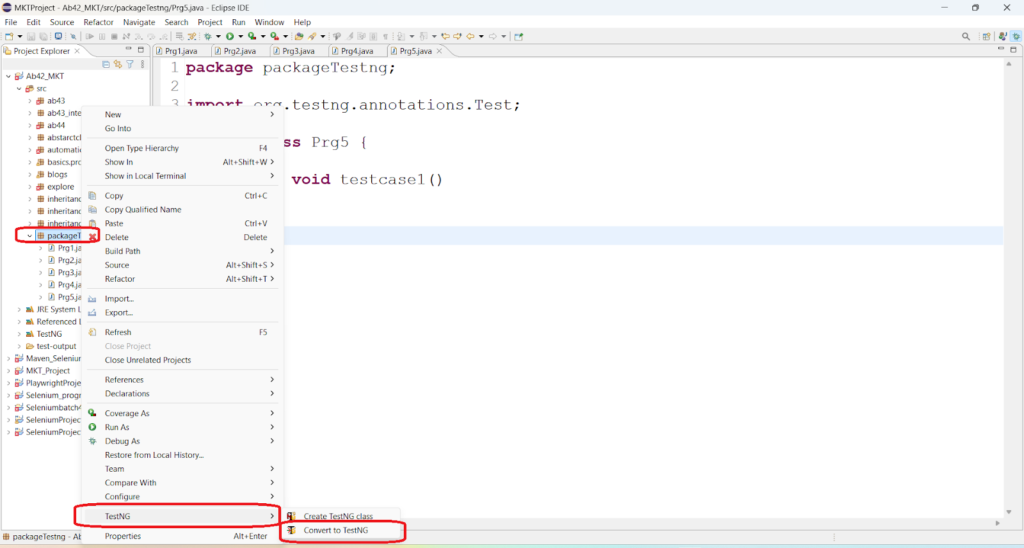
Step 3:Generate XML File->Click on Finish button
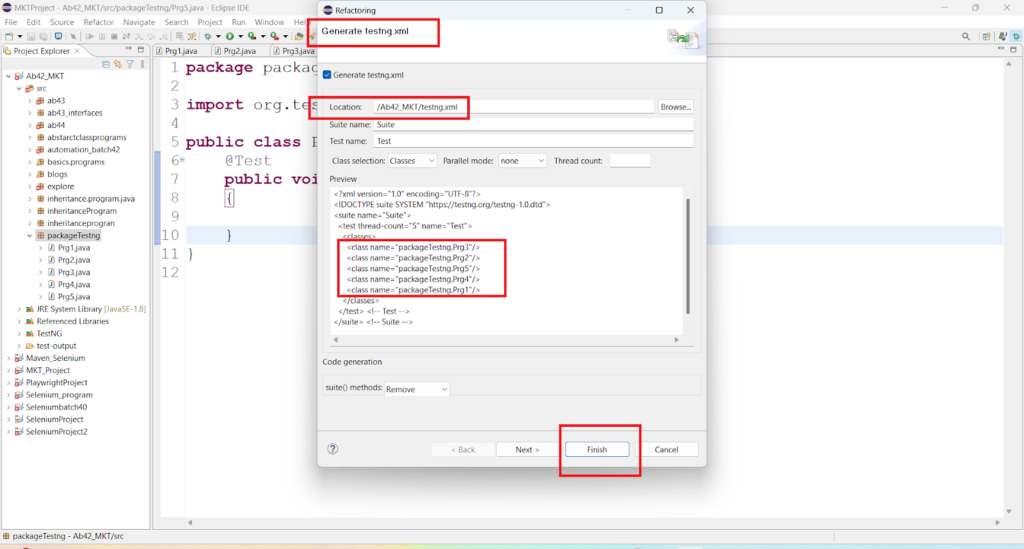
Step 4:TestNG.xml file is generated and this is how it will look like as shown below
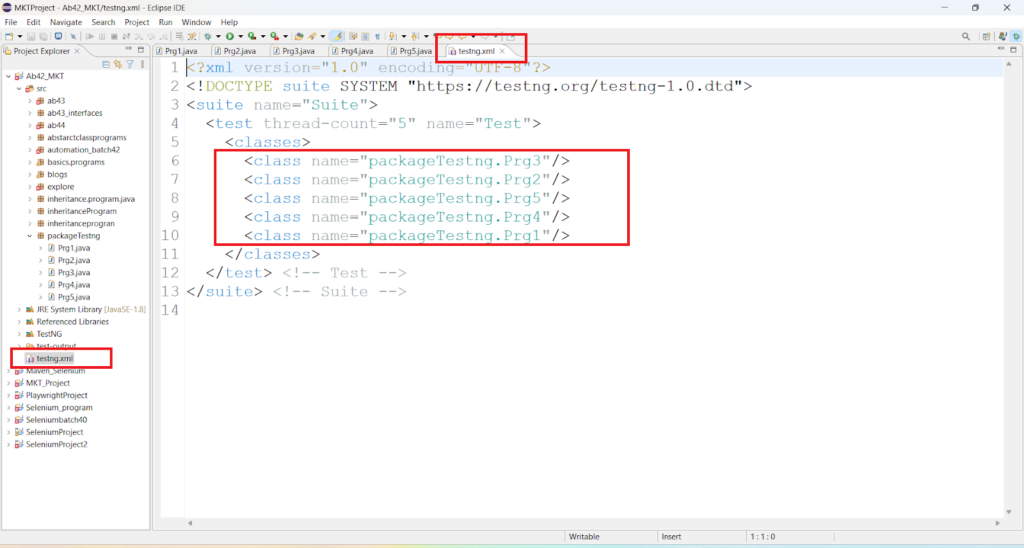
Step 5:Right click on TestNG.xml and run it as TestNG Suite
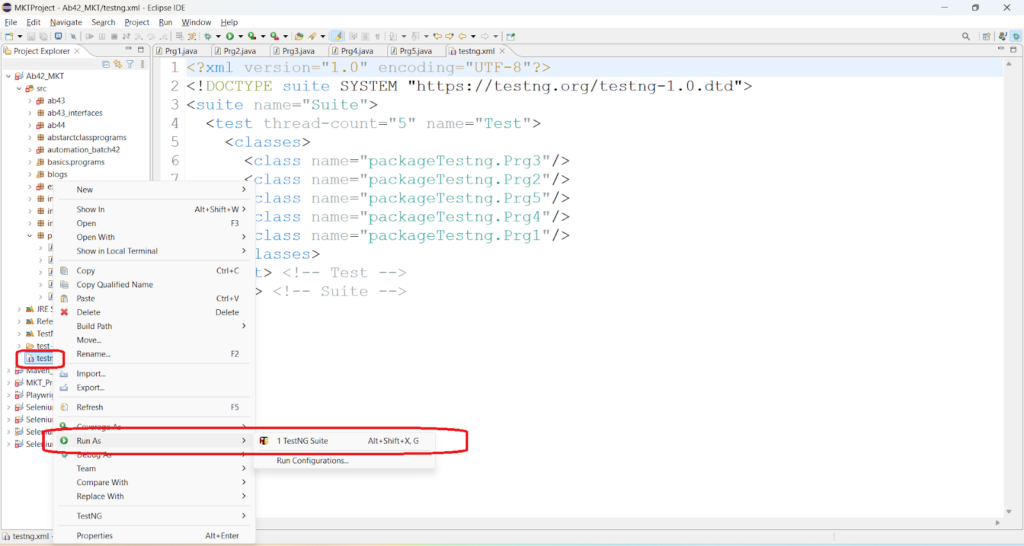
In the below screenshot you can see the console output in which it shows how many test cases are passed and how many are fail.
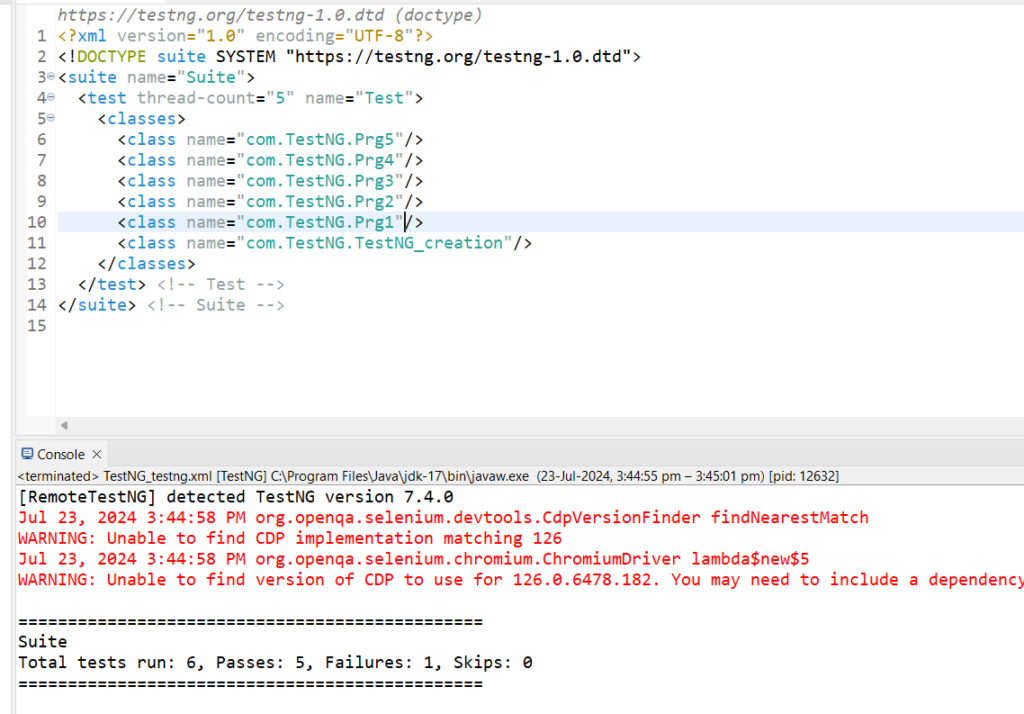
Below Screenshot shows the TestNG Emailable Report for the same.
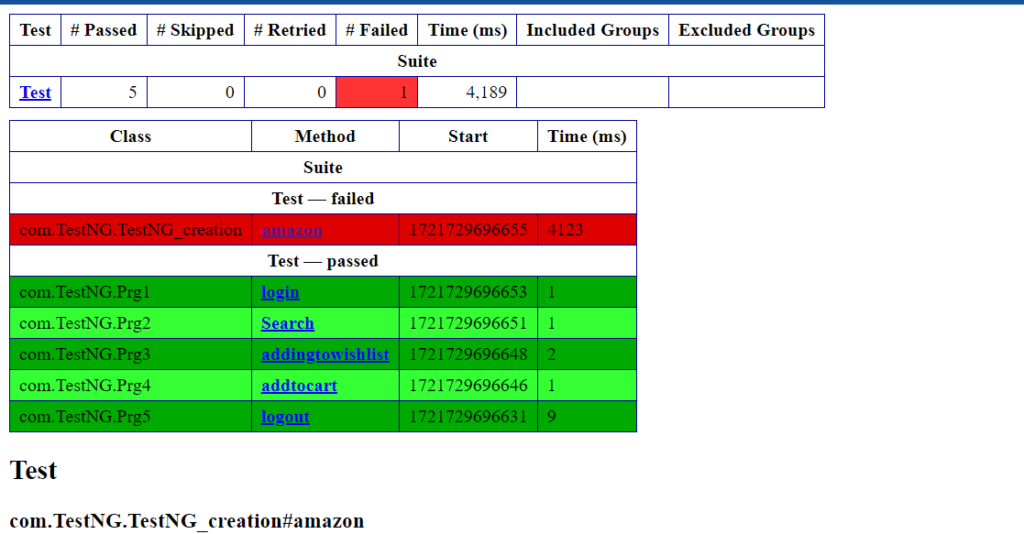
9.What is the sequence of annotation execution in TestNG?
- @BeforeSuite
- @BeforeTest
- @BeforeClass
- @BeforeMethod
- @Test
- @AfterMethod
- @AfterClass
- @AfterTest
- @AfterSuite
10.What is the default priority of any test in TestNG?
Default priority of any test case in TestNG is 0.
Example:
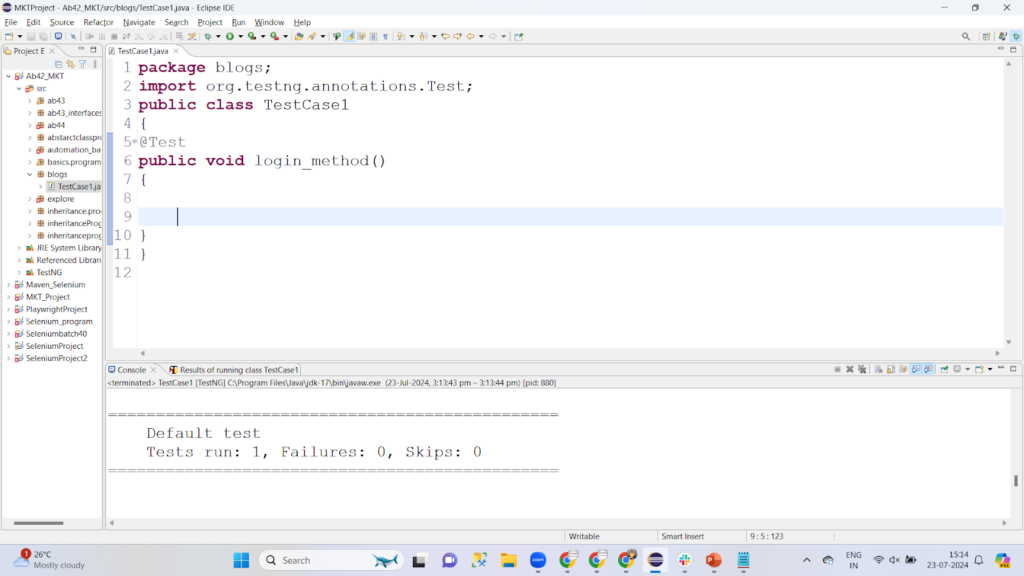
Here in the below screenshot, you can see we have added two test cases:
- Login[It has the priority 2]
- Logout[It has the priority 0 default]
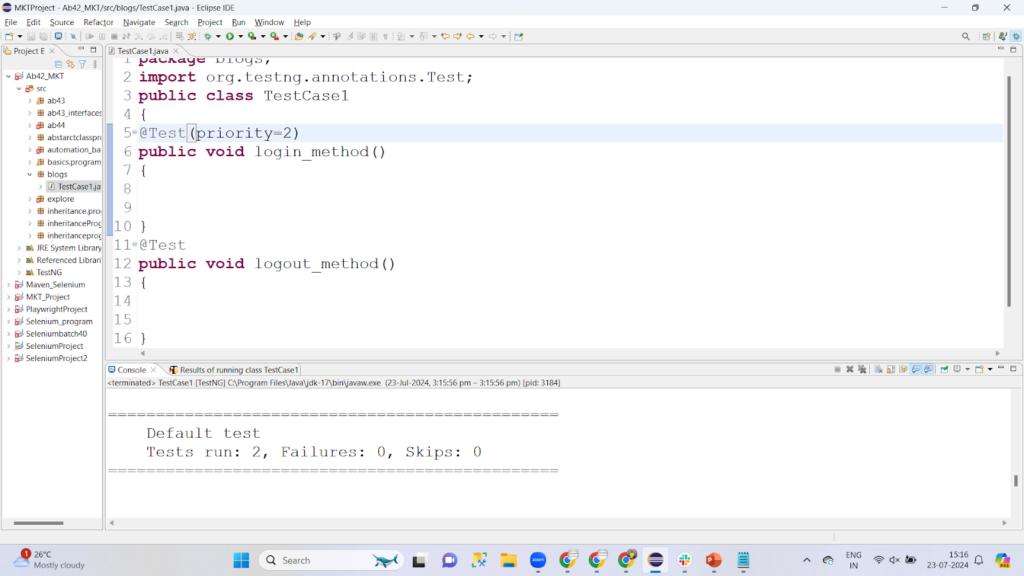
Below is the Result of Running Test:
- Logout method executed first and login method executed last is all because logout method default priority is 0.
- Lower the priority first it will execute.
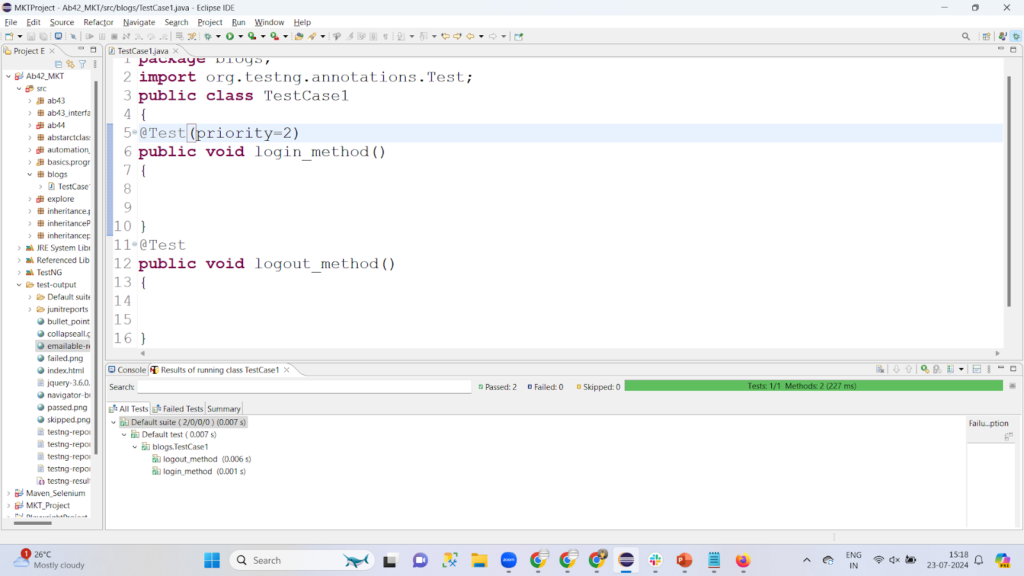
11.What will happen if we don't set the priority of test cases in TestNG?
Example 1: In the screenshot below, you can see there are a total of 2 test cases and just because we have not given any priority, it will take the alphabetic order of the method name. That’s why the first login method executed and later Registration method executed.
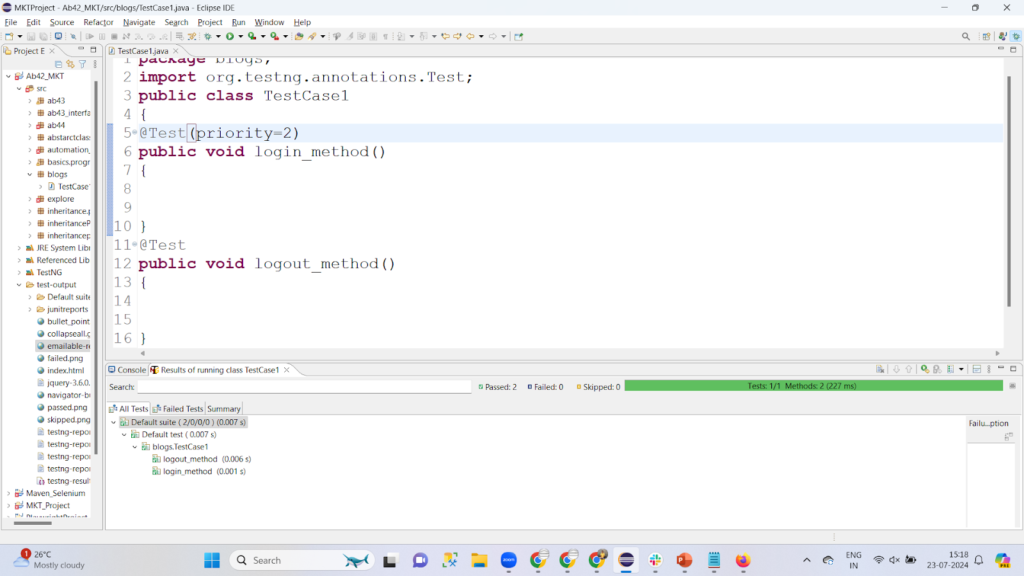
Example 2: In the below screenshot you can see there are a total 3 test cases and just because we have not given any priority it will take the alphabetic order of the method name. That’s why login executed first, Registration executed second and Searching executed last.
package blogs;
import org.testng.annotations.Test;
public class TestCase1
{
@Test
public void login()
{
}
@Test
public void registration()
{
}
@Test
public void searching()
{
}
}
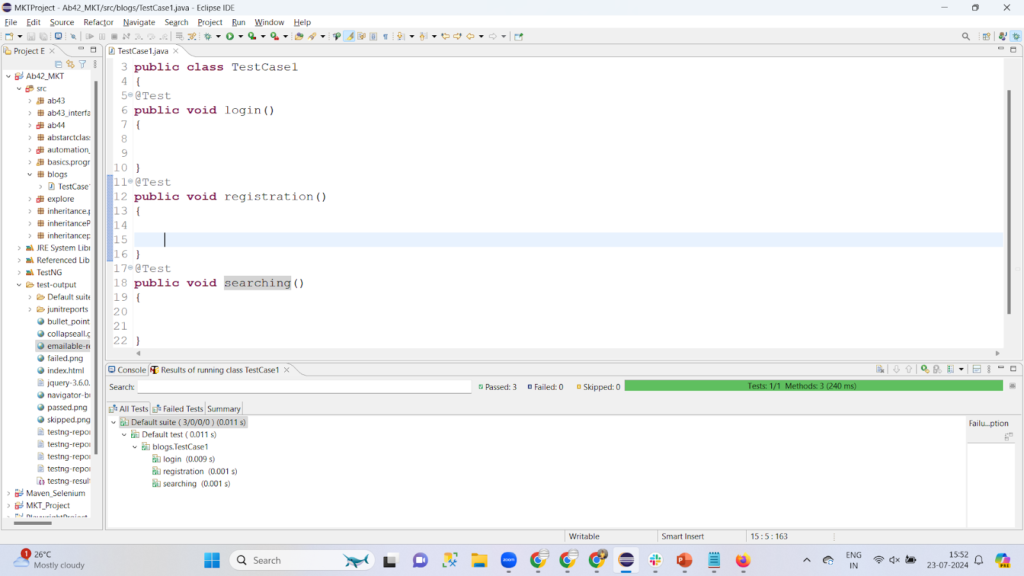
12.How to run the same test cases multiple times in TestNG?
Same Test Case can run multiple times with the help of invocationCount parameter in Annotations. Just because in the screenshot below we have passed invocationCount as 10, the same test case was executed 10 times.
package blogs;
import org.testng.annotations.Test;
public class SameTestCase
{
@Test(invocationCount=10)
public void login()
{
}
}
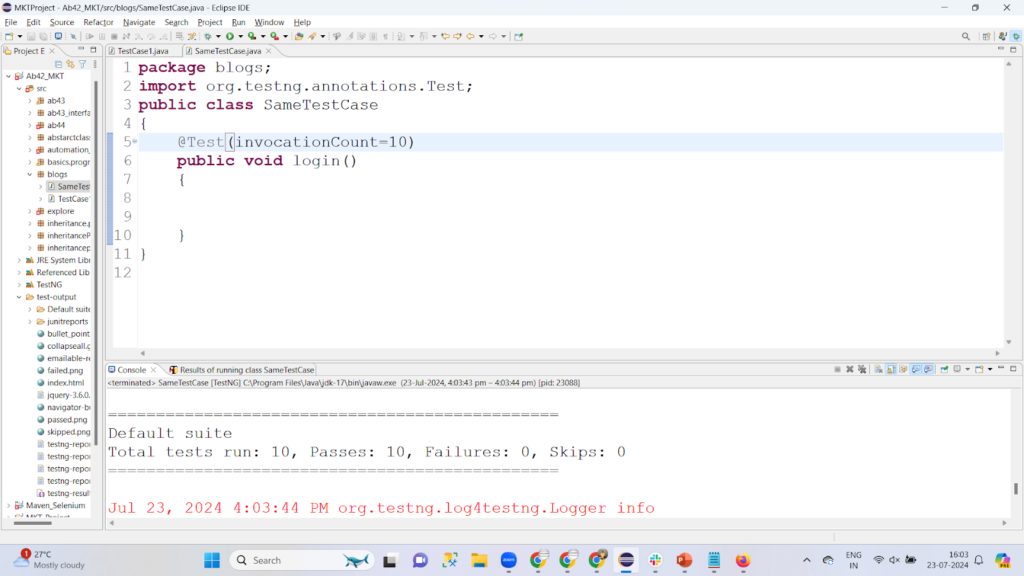
13. How to disable any test case in TestNG?
Test cases can be disabled using enabled=false parameter in Annotations. Just because in the screenshot below we have passed enabled=false, the same test case will never execute.
package TestNg_2AndMoreTestAnnotations;
import org.testng.annotations.Test;
public class Disable_Test {
@Test (enabled=false) // it will disable the test case
public static void logout()
{
System.out.println("logout");
}
}
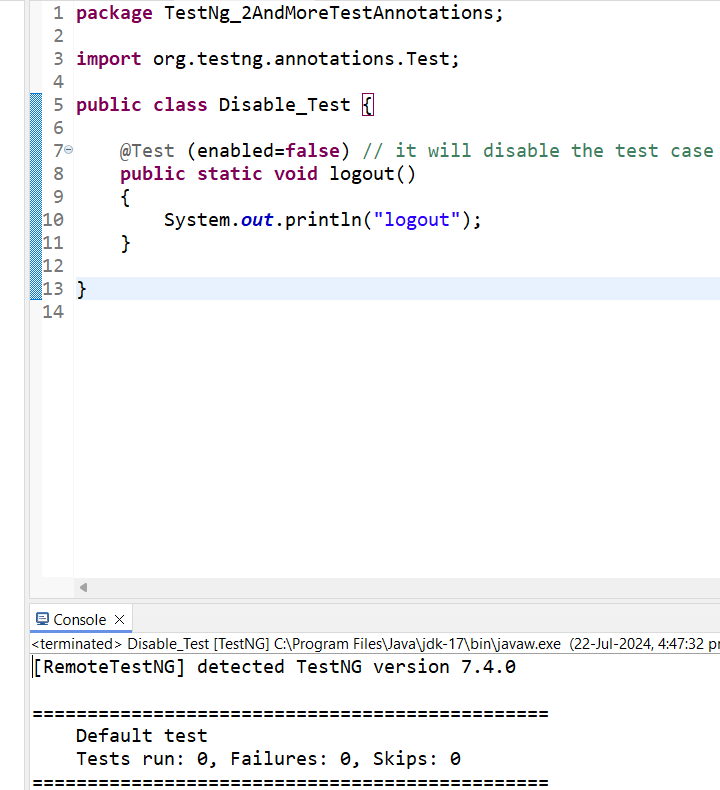
14. How to use any disable and priority parameter in the same test case?
Case 1: If any single class has 2 @Test then it will show us 2 Test Cases run as shown below.
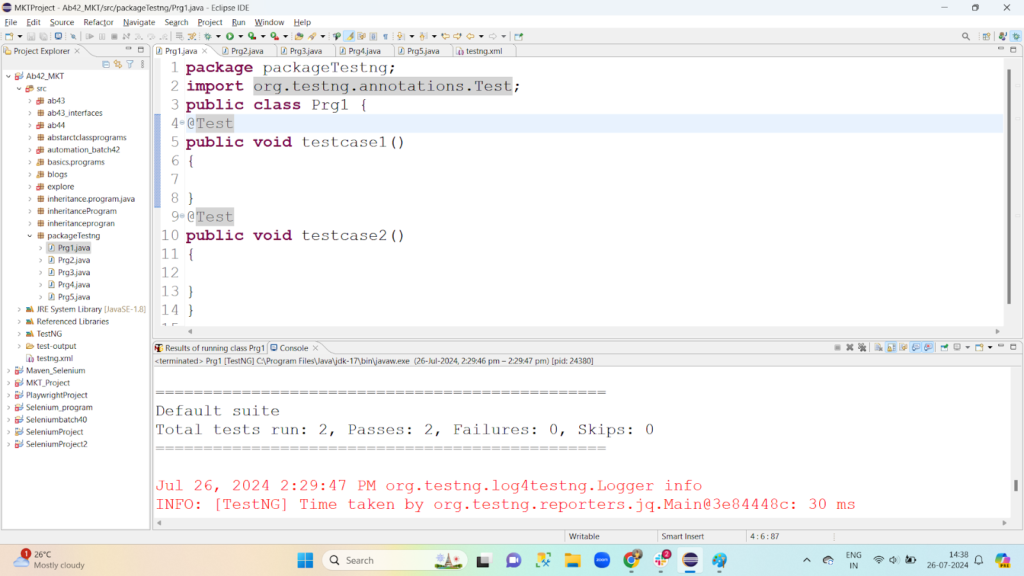
Case 2: If any Class if you have 2 @Test out of which one is having one is enabled=true then also both test cases will execute because by default enabled will be true itself.
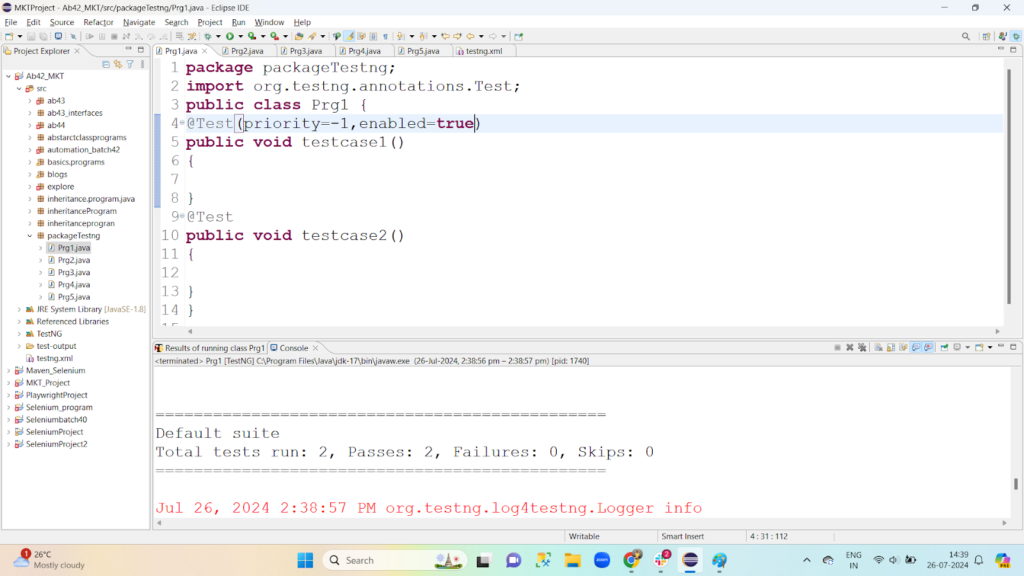
Case 3: Look at the line no 4 in below screenshot it is marked as enabled=false which means this particular test case will be ignored by TestNG.
package packageTestng;
import org.testng.annotations.Test;
public class Prg1 {
@Test(priority=-1,enabled=false)
public void testcase1()
{
}
@Test
public void testcase2()
{
}
}
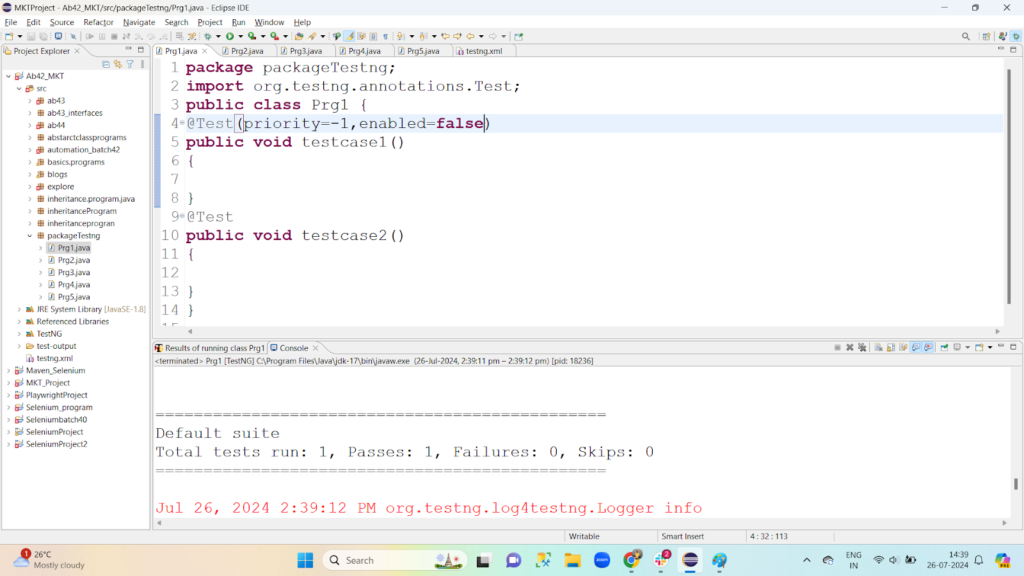
15. How to group the test cases in TestNG?
Grouping the test cases is important in Automation testing so that we can run only particular testing type test cases together when required. Example:
- If you wish to run only Smoke Test cases.
- If you wish to run complete Regression Test Cases.
In the below screenshot you can see in a single class we have 4 @Test and they are grouped into different types of testing types.
- The last 3 @Test has been marked as “system” which means System Test Case.
- The first @Test in line no 4 has been marked as “smoke” which means Smoke Test Case and “system” which means System Test Case.
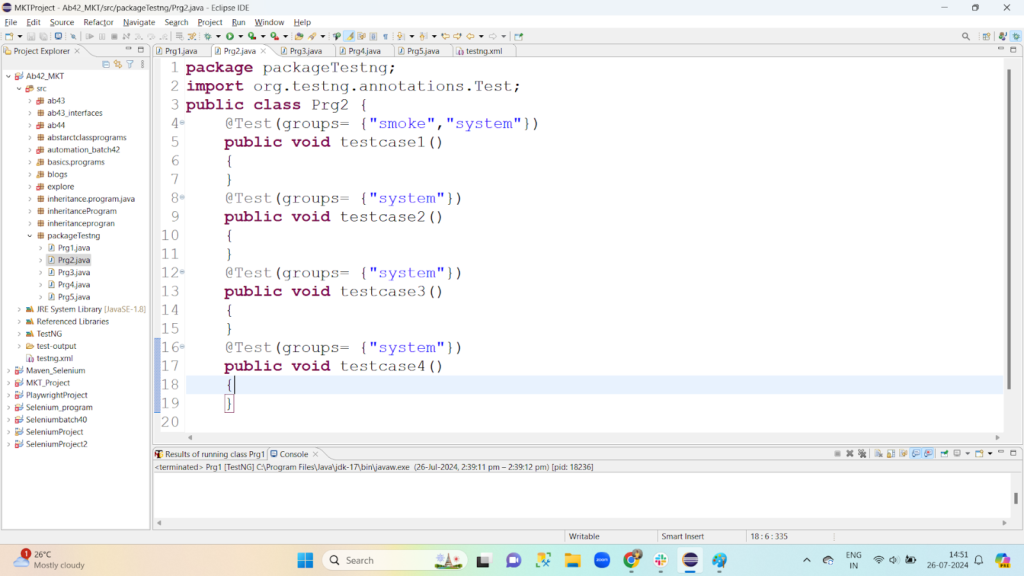
Right click on the class ->Click on TestNG->Convert to TestNG
package Grouping_Testing;
import org.testng.annotations.Test;
public class Group_Class {
@Test(groups= {"smoke","system"})
public void testcase1()
{
}
@Test(groups= {"system"})
public void testcase2()
{
}
@Test(groups= {"system"})
public void testcase3()
{
}
@Test(groups= {"system"})
public void testcase4()
{
}
}
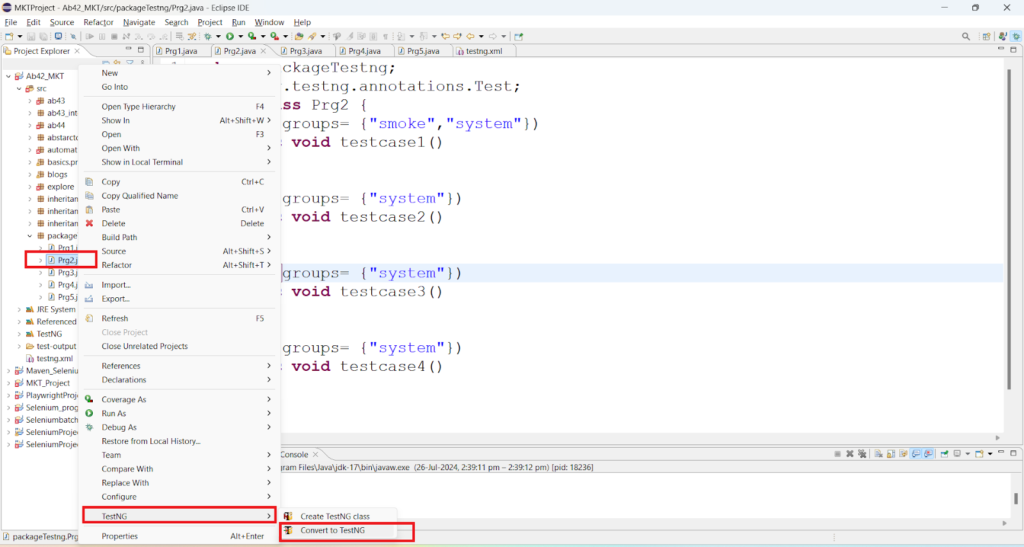
Make sure you are including smoke under <run and <run under <groups as shown below.
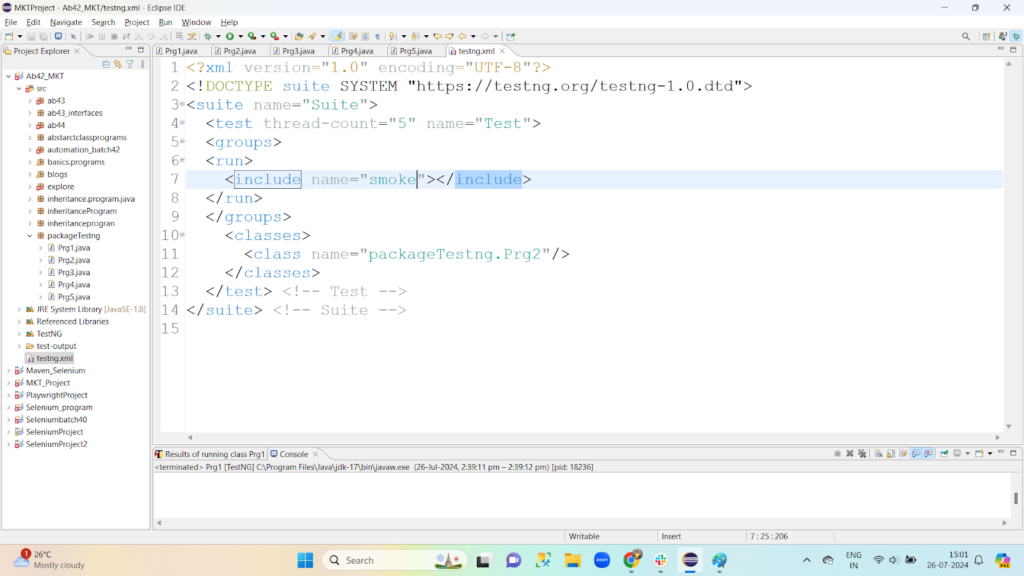
XML File as attached below for your reference:
16.How to use timeOuts parameter in TestNG?
TimeOuts parameter is used to make sure to finish the test case within the specified period time frame.It accepts the time in milliseconds after the threshold time it will be marked as Failed.
package TestNg_2AndMoreTestAnnotations;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class TimeOut_Testcase
{
@Test(timeOut=10000)
public void login()
{
ChromeDriver driver=new ChromeDriver();
driver.get("https://www.google.com/");
}
}
Example 1: In the above example the total time we have provided to finish the login step is 10 seconds. If login happens within that period the test case will be passed else fail.

Example 2: In the above example the total time we have provided to finish the login step is 2 seconds. If login happens within that period the test case will be passed else fail.

17. How to create a xml file in TestNG?
Step 1: Create individual classes with @Test
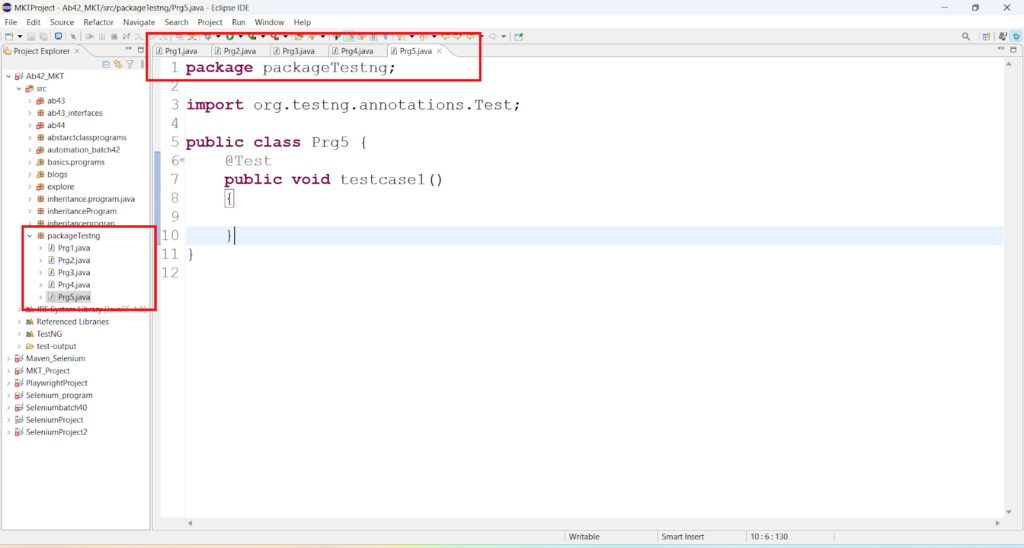
Step 2: Right click on given package->TestNG->Convert to TestNG
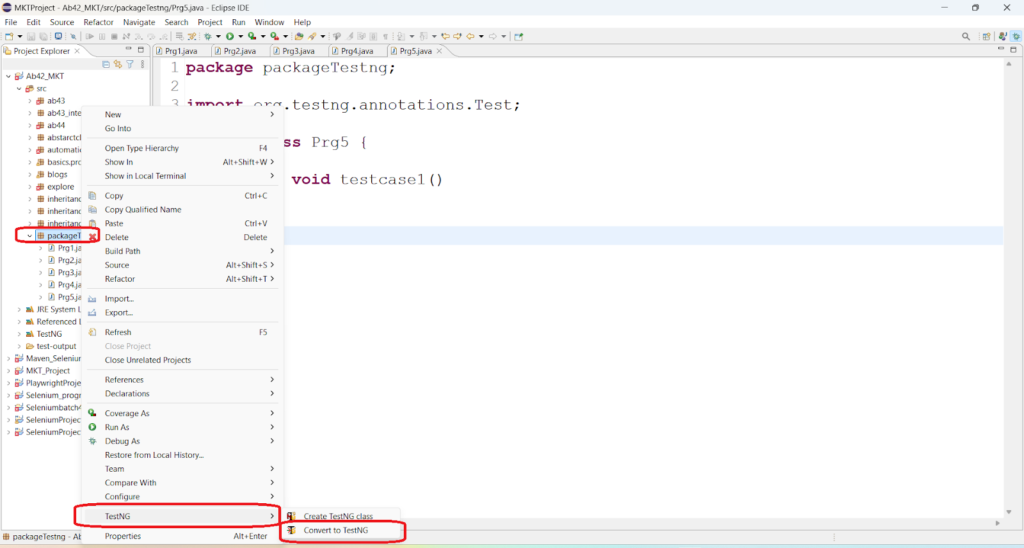
Step 3:Generate XML File->Click on Finish button
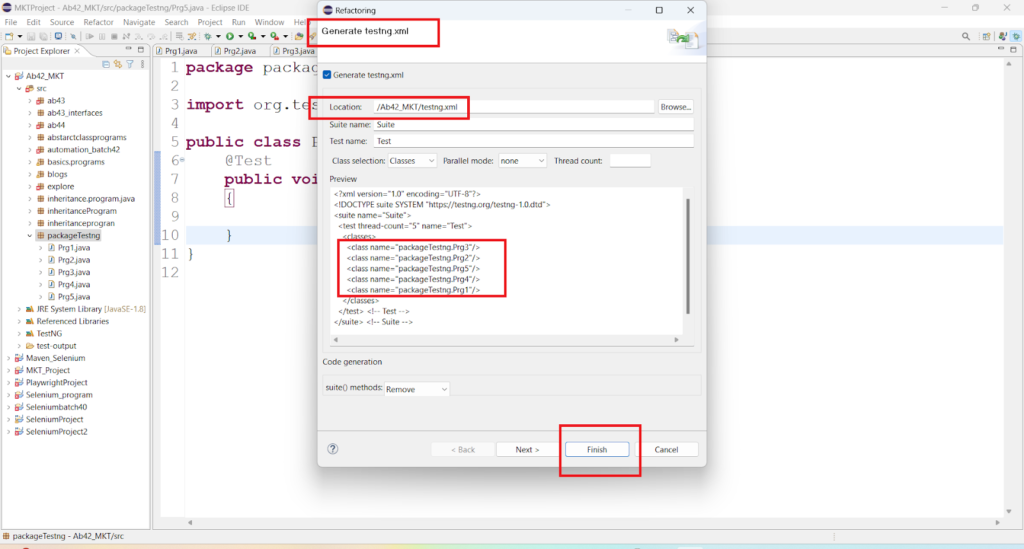
Step 4:TestNG.xml file is generated and this is how it will look like as shown below
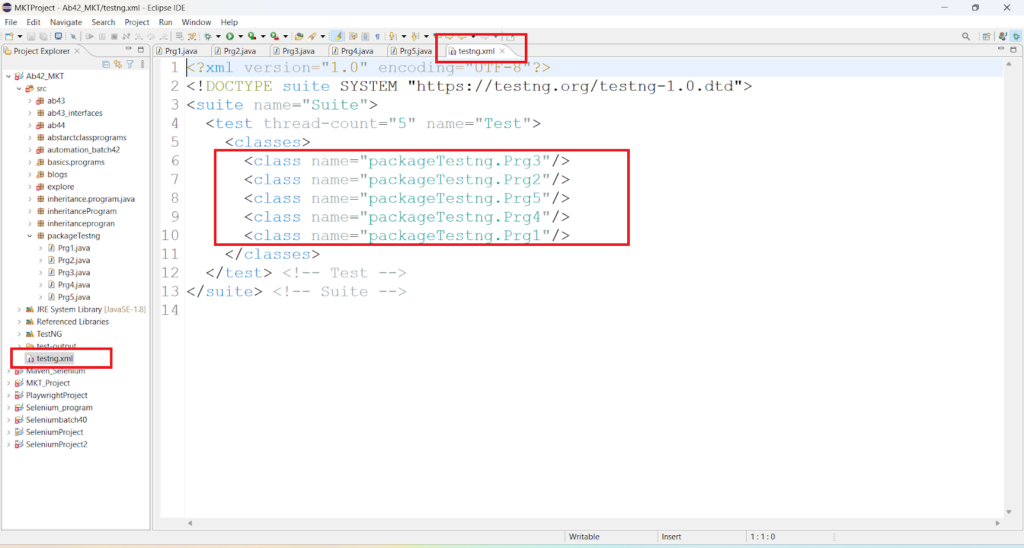
Step 5: Right click on TestNG.xml and run it as TestNG Suite
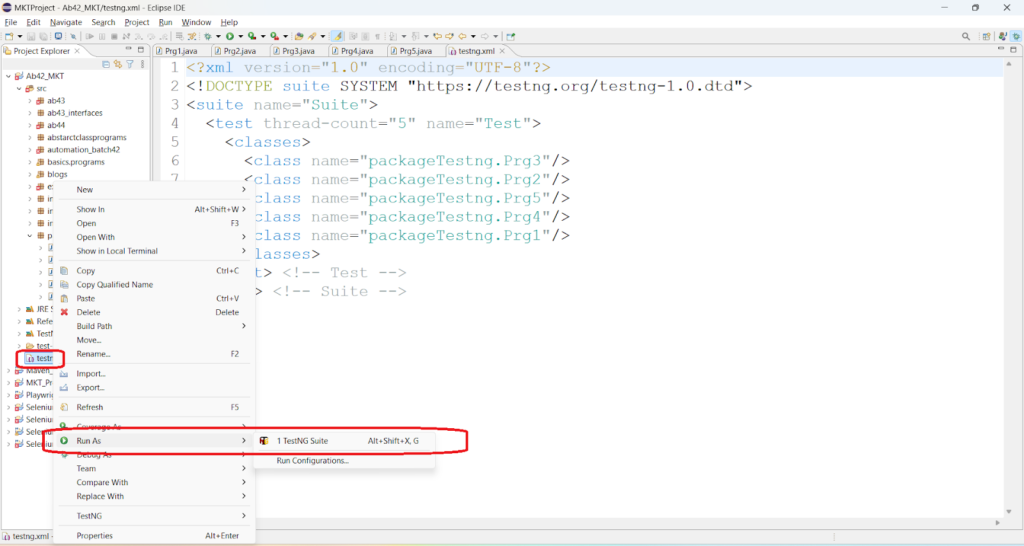
18. How to run the failed test cases in TestNG?
Let’s discuss the steps to steps procedure to run the failed test cases in testng.
Step 1:Run all tests together as a Suite and note down the Passes and Failures.
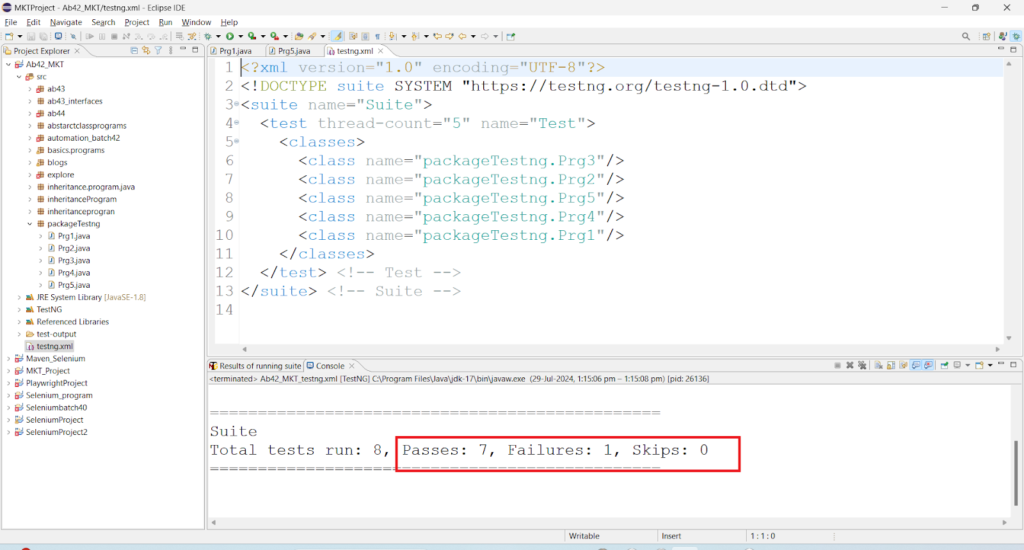
Step 2: Click on the Test Output Folder in Project Explorer, you will find “TestNG-Failed.XML and Double Click on the TestNG-Failed.xml
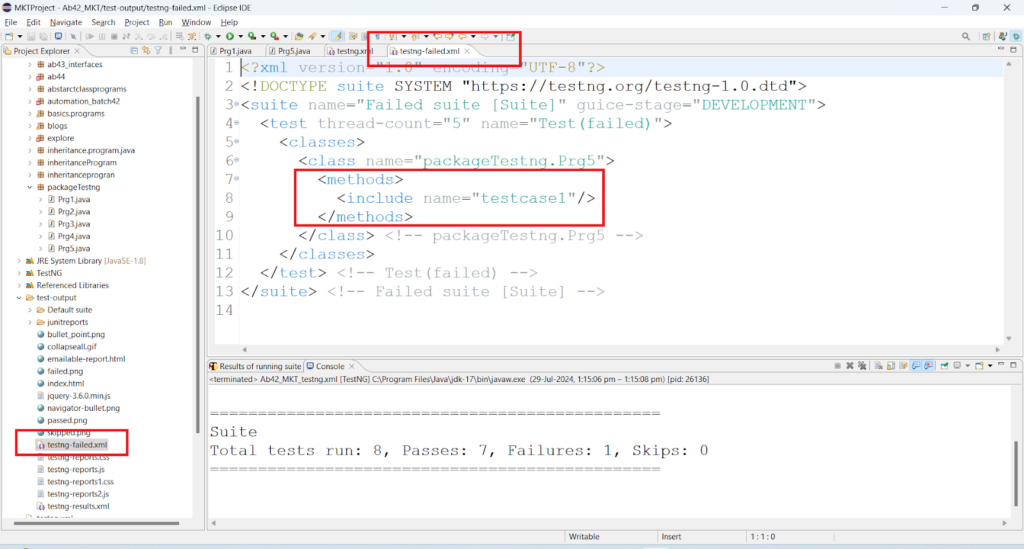
Step 3: Right Click on TestNG-Failed.XML
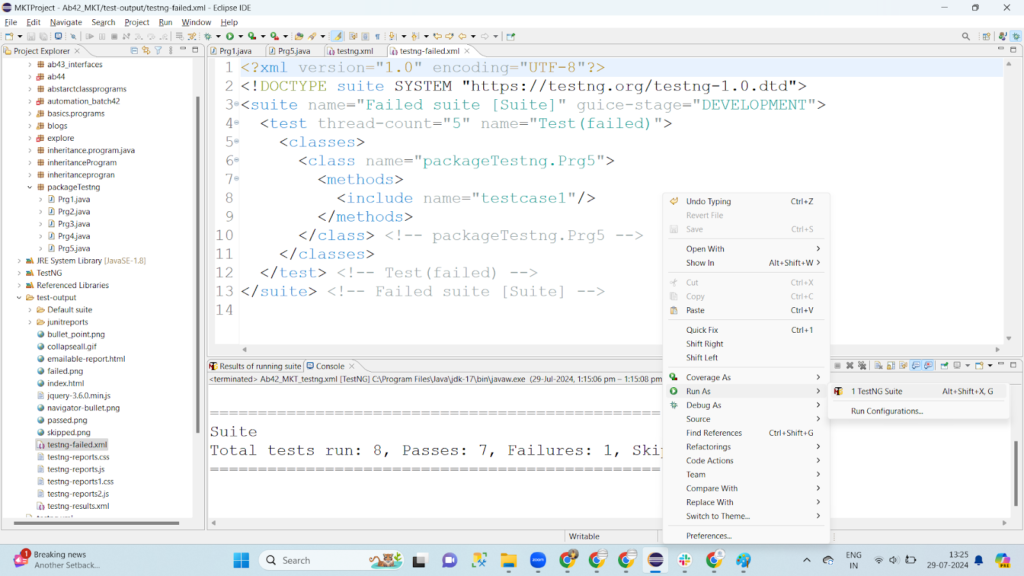
Step 4: Run it as TestNG Suite

19. What is the difference between a TestNG test and a TestNG test suite?
- TestNG Test: Single Test cases.
- TestNG Test Suite: Many TestCases combine to form a Test Suite.
20. Why do we need TestNG in Selenium?
Selenium needs TestNG for following reasons:
- For reporting as selenium does not have its own way of reporting.
- To run many test cases together
- TestNG provides Annotations
- Cross Browser Testing / Parallel Testing is possible using TestNG.
21. How to do Parallel Testing in Selenium using TestNG?
Parallel Testing is done in Selenium using TestNG to make sure the same test cases run on different browsers together which helps Automation Testers to achieve compatibility Testing in the same time frame as those test cases are running parallel.
package Parallel_Tetsing;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.edge.EdgeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.testng.annotations.Parameters;
import org.testng.annotations.Test;
//Lets Launch Google->Type India->and press Enter button
public class Prallel_testing_google
{
WebDriver driver;//globally declaring WebDriver
@Test
@Parameters("browser")//this value of browser should come from XML file
public void google(String Nameofthebrowser)//send the Nameofthebrowser from XML file
{
if(Nameofthebrowser.equals("chrome"))//Using String functions “equals” to check if
{ //browser is chrome
driver=new ChromeDriver();
}
if(Nameofthebrowser.equals("edge"))//Using String functions “equals” to check if /browser is edge
{
driver=new EdgeDriver();
}
driver.get("https://www.google.com/");
WebDriverWait w1=new WebDriverWait(driver,Duration.ofSeconds(10));
w1.until(ExpectedConditions.titleContains("Goo")); // G is also case sensitive
WebElement w=driver.findElement(By.id("APjFqb"));
w.sendKeys("india");
w.sendKeys(Keys.ENTER);
}
}
XML File
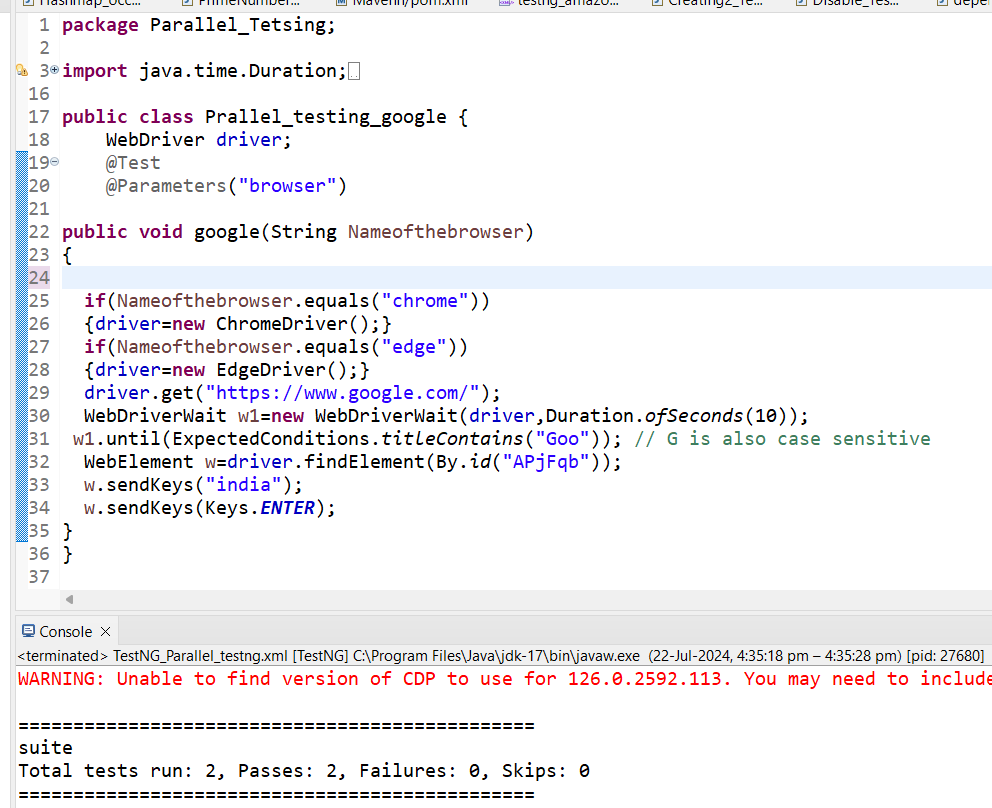
22. How to do Cross Browser Testing in Selenium using TestNG?
Cross Browser Testing is done in Selenium using TestNG to make sure the same test cases run on different browsers one by one which helps Automation Testers to achieve compatibility Testing. Time taken in Cross Browser Testing will be more than Parallel Testing as test cases are executed step by step in different browsers.
package Parallel_Tetsing;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.edge.EdgeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.testng.annotations.Parameters;
import org.testng.annotations.Test;
public class Cross_Browser_testing_google
{
WebDriver driver;
@Test
@Parameters("browser")
public void google(String Nameofthebrowser)
{
if(Nameofthebrowser.equals("chrome"))
{
driver=new ChromeDriver();
}
if(Nameofthebrowser.equals("edge"))
{
driver=new EdgeDriver();
}
driver.get("https://www.google.com/");
WebDriverWait w1=new WebDriverWait(driver,Duration.ofSeconds(10));
w1.until(ExpectedConditions.titleContains("Goo")); // G is also case sensitive
WebElement w=driver.findElement(By.id("APjFqb"));
w.sendKeys("india");
w.sendKeys(Keys.ENTER);
}
}
XML File
?xml version="1.0" encoding="UTF-8"?>
23. How to test the same test cases using multiple inputs using DataProvider in TestNG?
package Data_Provier;
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
public class DataProvier_program {
@DataProvider(name ="data1")
public Object method1()
{
return new Object[][] {{"Ram"},{"sita"},{"Lakshman"}};
}
@Test(dataProvider="data1")
public void tetscase1(String s1)
{
System.out.println(s1.concat(" ramayanam"));
}
}

24. How to Use Listeners Annotations in TestNG?
TestNG Listeners are used to constantly listen to all the test activities.Listeners listen to the event defined in the selenium script and behave accordingly.
Step 1:Create a class and implements ITestListener
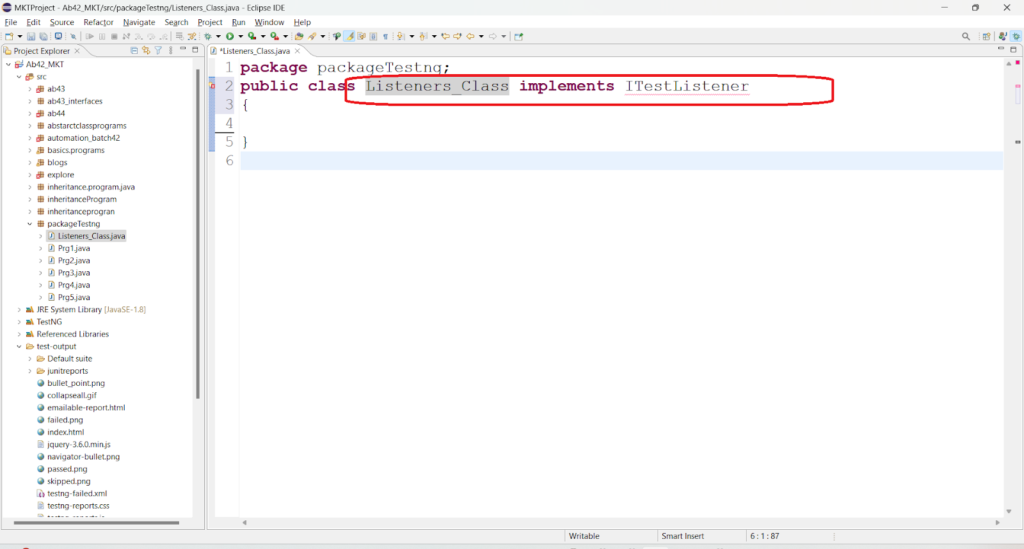
Step 2:Import the Interface
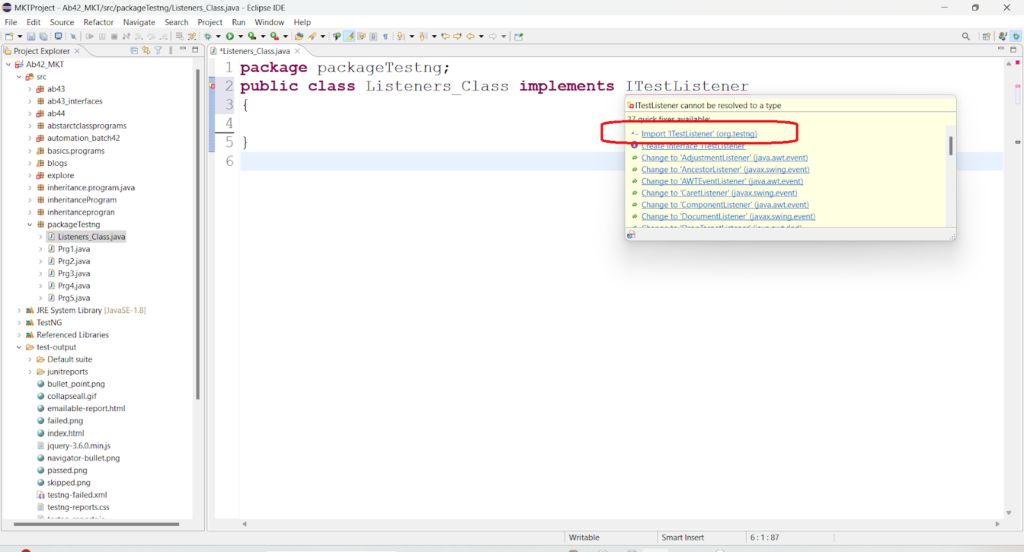
Step 3: Do the right click on class and click on source->click on override/implementation Method.
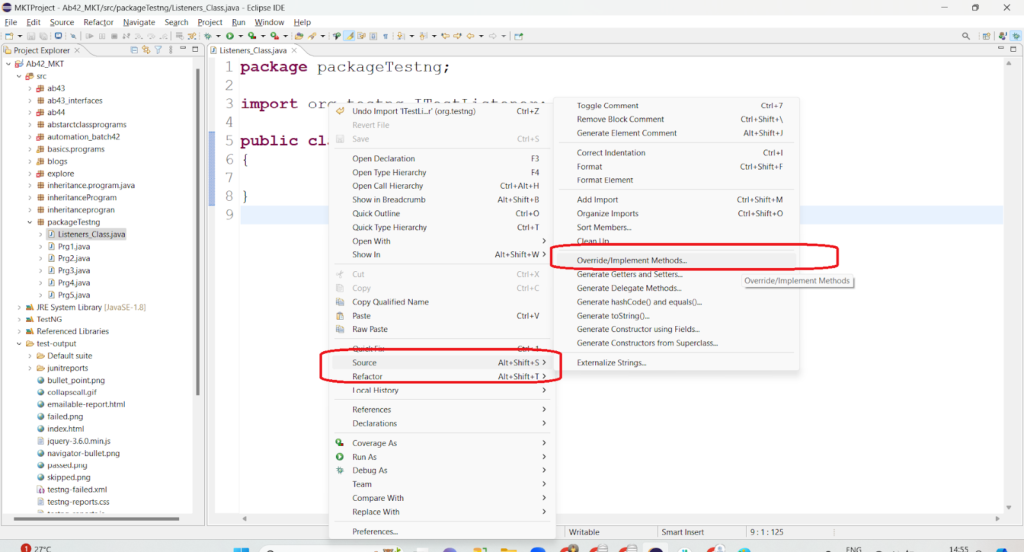
Step 4: ITestListener Interface abstract methods will come automatically we need to select all and Click on ok button.
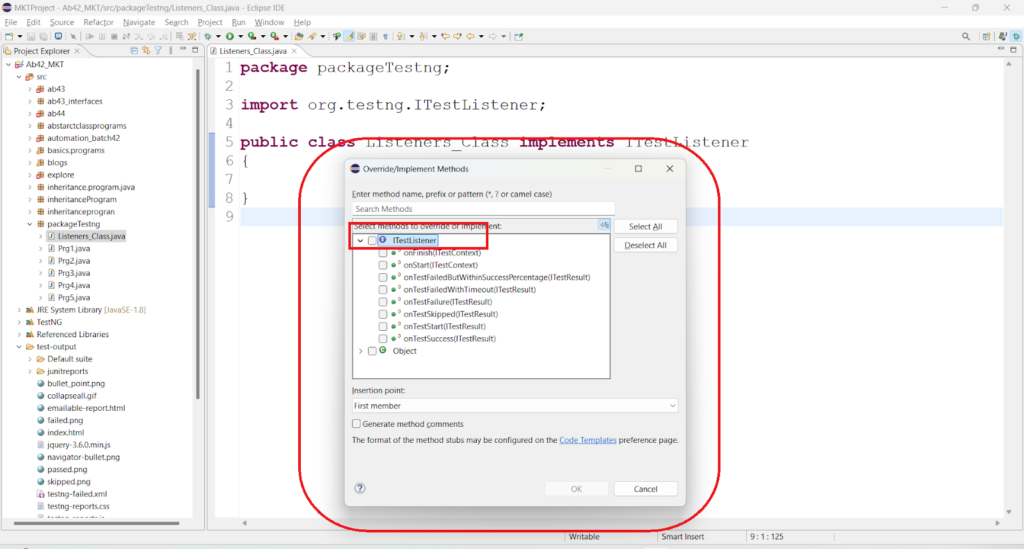
Step 5: IT will modify the code as following
package packageTestng;
import org.testng.ITestContext;
import org.testng.ITestListener;
import org.testng.ITestResult;
public class Listeners_Class implements ITestListener
{
@Override
public void onTestStart(ITestResult result) {
// TODO Auto-generated method stub
ITestListener.super.onTestStart(result);
}
@Override
public void onTestSuccess(ITestResult result) {
// TODO Auto-generated method stub
ITestListener.super.onTestSuccess(result);
}
@Override
public void onTestFailure(ITestResult result) {
// TODO Auto-generated method stub
ITestListener.super.onTestFailure(result);
}
@Override
public void onTestSkipped(ITestResult result) {
// TODO Auto-generated method stub
ITestListener.super.onTestSkipped(result);
}
@Override
public void onTestFailedButWithinSuccessPercentage(ITestResult result) {
// TODO Auto-generated method stub
ITestListener.super.onTestFailedButWithinSuccessPercentage(result);
}
@Override
public void onTestFailedWithTimeout(ITestResult result) {
// TODO Auto-generated method stub
ITestListener.super.onTestFailedWithTimeout(result);
}
@Override
public void onStart(ITestContext context) {
// TODO Auto-generated method stub
ITestListener.super.onStart(context);
}
@Override
public void onFinish(ITestContext context) {
// TODO Auto-generated method stub
ITestListener.super.onFinish(context);
}
}

Step 6:Create a Test case and inherit the above Listener class.
Lets Automate launch google->Type India and press Enter button
package Listeners;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.Assert;
import org.testng.annotations.Listeners;
import org.testng.annotations.Test;
@Listeners(Listenerlogic.class)
public class Search_India extends Listenerlogic
{
@Test
public void Google_india()
{
driver=new ChromeDriver();
driver.get("https://www.google.com/");
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(3));
WebElement w1= driver.findElement(By.id("APjFqb"));
w1.sendKeys("india");
//driver.findElement(By.name("btnK")).click();
w1.sendKeys(Keys.ENTER);
Assert.assertEquals(false, true);
}
}
Step 6:Copy paste the screenshot login under onTestSuccess method and onTestFailure method
Code Snippet:
Listener_Interface_Abstarctmethods
package Listeners;
import java.io.File;
import java.io.IOException;
import org.openqa.selenium.OutputType;
import org.openqa.selenium.TakesScreenshot;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.io.FileHandler;
import org.testng.ITestContext;
import org.testng.ITestListener;
import org.testng.ITestResult;
public class Listenerlogic implements ITestListener
{
static WebDriver driver;
@Override
public void onTestStart(ITestResult result)
{
ITestListener.super.onTestStart(result);
}
@Override
public void onTestSuccess(ITestResult result)
{
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
ITestListener.super.onTestSuccess(result);
TakesScreenshot t1= (TakesScreenshot) driver;
File source=t1.getScreenshotAs(OutputType.FILE);
File destination=new File("C:\\Users\\HP\\OneDrive\\Desktop\\Selenium_Screenshots\\Pass\\Listener"+Math.random()+".jpg");
try {
FileHandler.copy(source, destination);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Override
public void onTestFailure(ITestResult result) {
ITestListener.super.onTestFailure(result);
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
ITestListener.super.onTestSuccess(result);
TakesScreenshot t1= (TakesScreenshot) driver;
File source=t1.getScreenshotAs(OutputType.FILE);
File destination=new File("C:\\Users\\HP\\OneDrive\\Desktop\\Selenium_Screenshots\\Fail\\Listener"+Math.random()+".jpg");
try {
FileHandler.copy(source, destination);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Override
public void onTestSkipped(ITestResult result) {
ITestListener.super.onTestSkipped(result);
}
@Override
public void onTestFailedButWithinSuccessPercentage(ITestResult result) {
ITestListener.super.onTestFailedButWithinSuccessPercentage(result);
}
@Override
public void onTestFailedWithTimeout(ITestResult result) {
ITestListener.super.onTestFailedWithTimeout(result);
}
@Override
public void onStart(ITestContext context) {
// TODO Auto-generated method stub
ITestListener.super.onStart(context);
}
@Override
public void onFinish(ITestContext context) {
// TODO Auto-generated method stub
ITestListener.super.onFinish(context);
}
}
Step 7:Now run the test case when the test case will be passed it will take the screenshot in the pass folder and when the test case is failed it will take the screenshot in the fail folder.
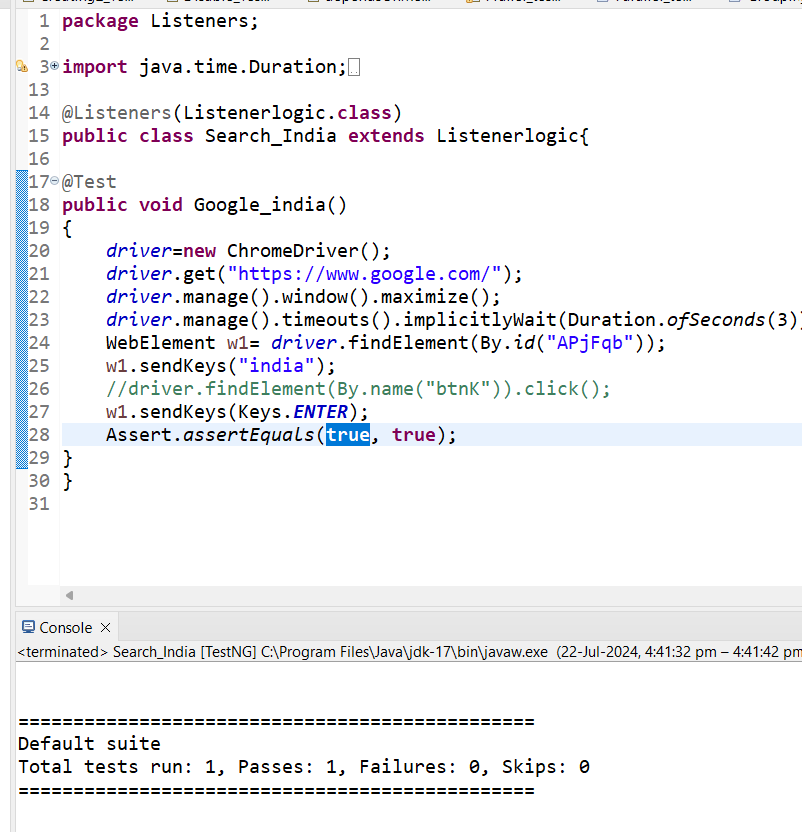
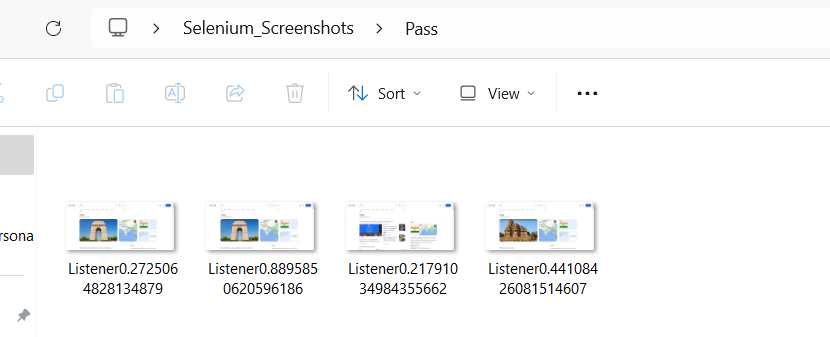
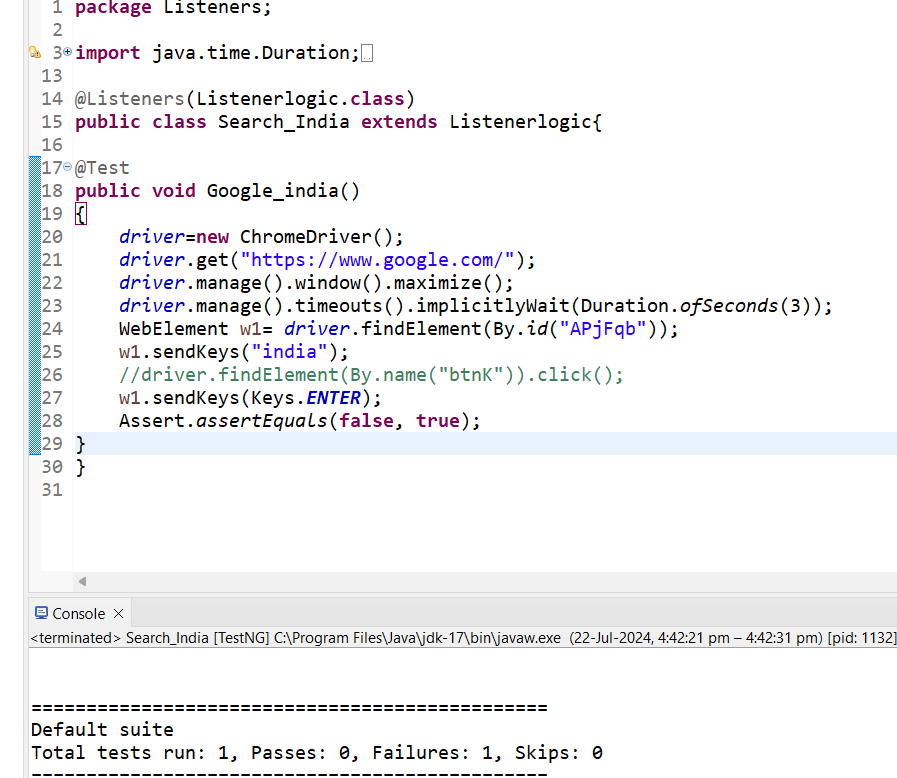
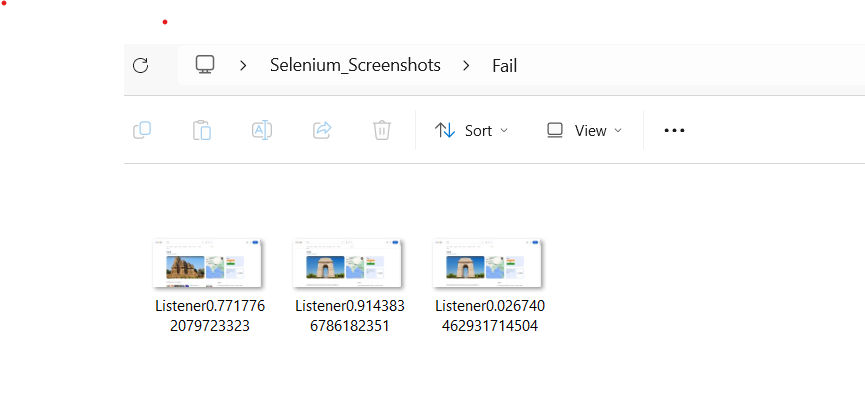
25. What is dependsOnMethod in TestNG?
DependsonMethod parameter is used in TestNG to make sure one test case should only run if the test case it depends on is passed else it will be skipped.
package TestNg_2AndMoreTestAnnotations;
import org.testng.Assert;
import org.testng.annotations.Test;
public class dependsOnMethods_Class
{
@Test //we are purposefully making it fail
public void login()
{
Assert.assertEquals(false, true);
}
@Test(dependsOnMethods="login") // This will skip as login is failed
public void logout()
{
}
}
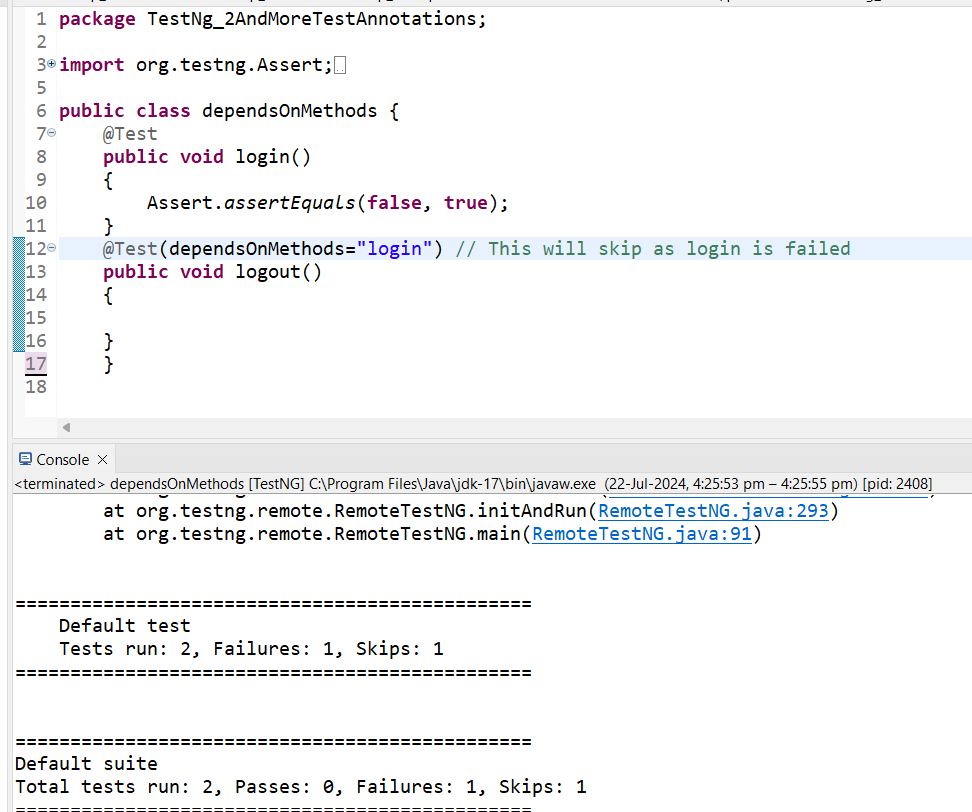
26. What is the order of execution when you have two @Test and one BeforeMethod and one AfterMethod present in the same class.
In a class if you have two @Test and one @BeforeMethod and one @AfterMethod than the order of execution will be
- @BeforeMethod
- @Test
- @AfterMethod
- @BeforeMethod
- @Test
- @AfterMethod
package packageTestng;
import org.testng.annotations.AfterMethod;
import org.testng.annotations.BeforeMethod;
import org.testng.annotations.Test;
public class Prg4
{
@BeforeMethod
public void launch()
{
System.out.println("launch");
}
@Test
public void testcase1()
{
System.out.println("test case 1");
}
@Test
public void testcase2()
{
System.out.println("test case 2");
}
@AfterMethod
public void quit()
{
System.out.println("quit");
}
}
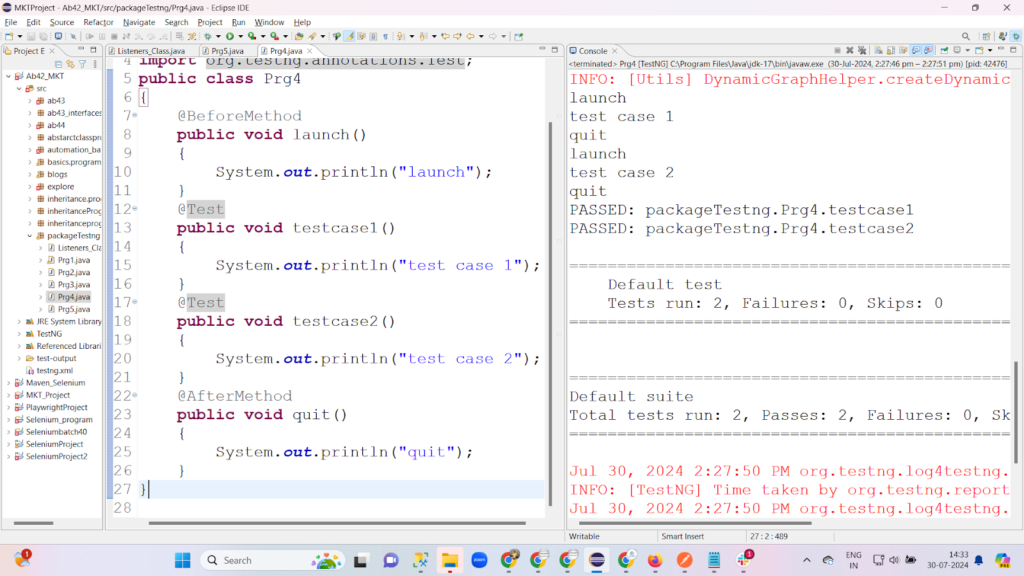
27. How to use TestNG Assertions?
TestNG assertion can be used with the Assert class as shown below.
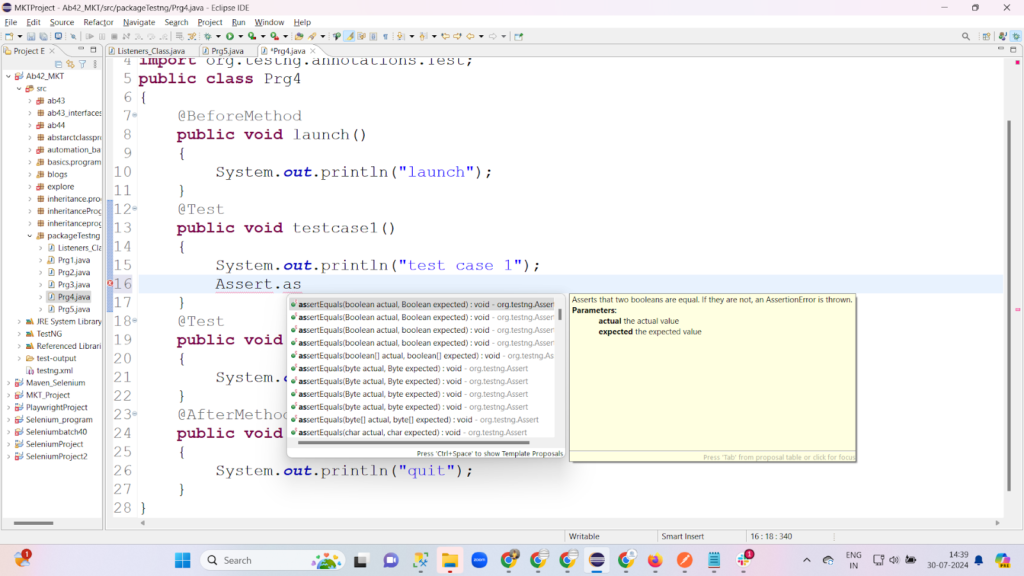
Different types of Assertion we have in TestNG are:
assertequals(boolean actual,boolean expected) | |
assertequals(byte actual,byte expected) | |
assertequals(int actual,int expected) | |
assertequals(short actual,short expected) | |
assertequals(long actual,long expected) | |
assertequals(String actual,String expected) | |
assertequals(char actual,char expected) | |
assertequals(float actual,float expected) | |
assertequals(double actual,double expected) | |
assertequals(boolean actual,boolean expected,String message) | |
assertequals(byte actual,byte expected,String message) | |
assertequals(int actual,int expected,String message) | |
assertequals(short actual,short expected,String message) | |
assertequals(long actual,long expected,String message) | |
assertequals(String actual,String expected,String message) | |
assertequals(char actual,char expected,String message) | |
assertequals(float actual,float expected,String message) | |
assertequals(double actual,double expected,String message) | |
assertequals(boolean[] actual,boolean[] expected) | |
assertequals(byte[] actual,byte[] expected) | |
assertequals(int[] actual,int[] expected) | |
assertequals(short[] actual,short[] expected) | |
assertequals(long[] actual,long[] expected) | |
assertequals(String[] actual,String[] expected) | |
assertequals(char[] actual,char[] expected) | |
assertequals(float[] actual,float[] expected) | |
assertequals(double [] actual,double[] expected) | |
assertequals(boolean []actual,boolean expected,String message) | |
assertequals(byte actual[],byte expected,String message) | |
assertequals(int [] actual,int[] expected,String message) | |
assertequals(short [] actual,short []expected,String message) | |
assertequals(long []actual,long []expected,String message) | |
assertequals(String []actual,String[] expected,String message) | |
assertequals(char[] actual,char[] expected,String message) | |
assertequals(float []actual,float [] expected,String message) | |
assertequals(double[] actual,double[] expected,String message) |
package Assertion;
import java.time.Duration;
import java.util.Set;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.Assert;
import org.testng.annotations.Test;
public class Assertion_Amazonshoe_WindowSize {
@Test
public void amazon()
{
ChromeDriver driver=new ChromeDriver();
driver.get("https://www.amazon.in/");
WebElement w1=driver.findElement(By.id("twotabsearchtextbox"));
w1.sendKeys("shoe");
w1.sendKeys(Keys.ENTER);
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(40));
WebElement first_shoe= driver.findElement(By.xpath("(//a[@class='a-link-normal s-no-outline'])[1]"));
first_shoe.click();
Set s1=driver.getWindowHandles();
System.out.println(s1.size());
//Assert.assertEquals(s1.size(), 2,"value is different");
//Assert.assertEquals(s1.size(), 1,"value is different");
Assert.assertEquals(s1.size(), 2);
}
}
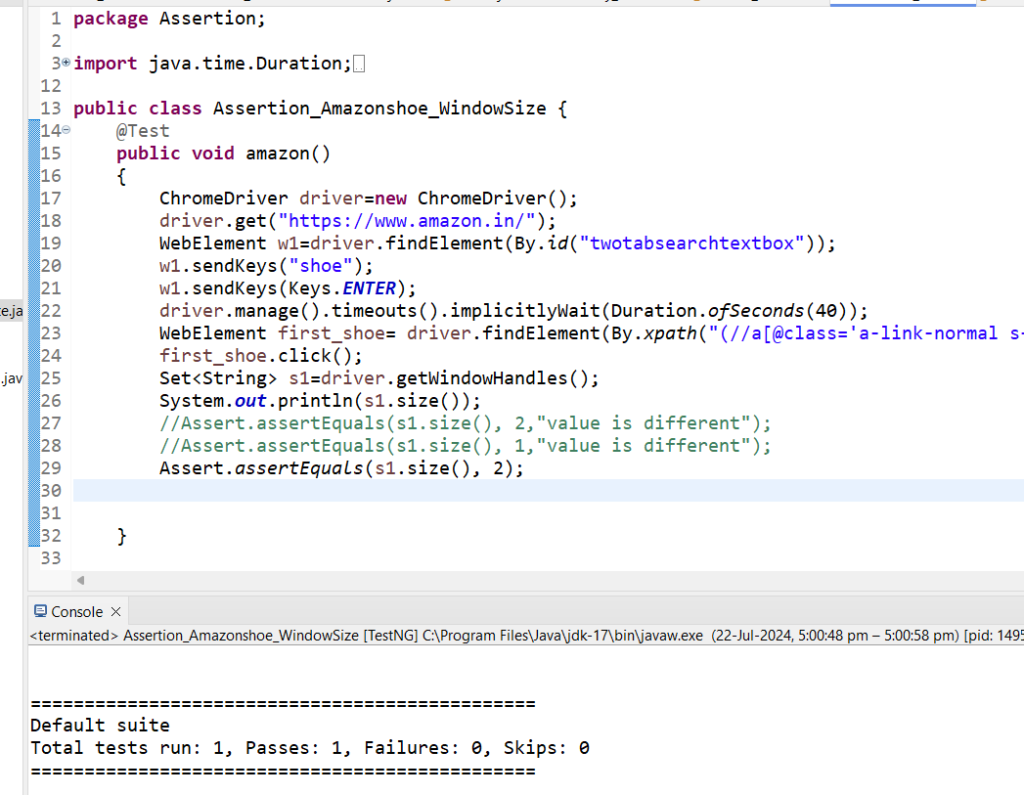
Checkout my most viewed blogs:
Important 56 Automation Testing Interview Questions &Answers
TOP 20 Automation Testing Interview Questions for Experienced
Top 23 Automation Testing Interview Questions and Answers
Java Interview Questions and Answers
Manual testing interview questions for experienced
API Testing interview questions
Consult Us