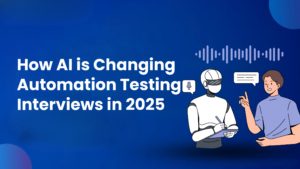

Important Automation Testing Interview Questions & Answers
String | Immutable |
StringBuffer | Mutable |
StringBuilder | Mutable |
package automation_batch42;
public class StringBuffer_program
{
public static void main(String[] args)
{
StringBuffer s1=new StringBuffer("Automation");
s1.append(" Testing");
System.out.println(s1);
}
}
Answer:
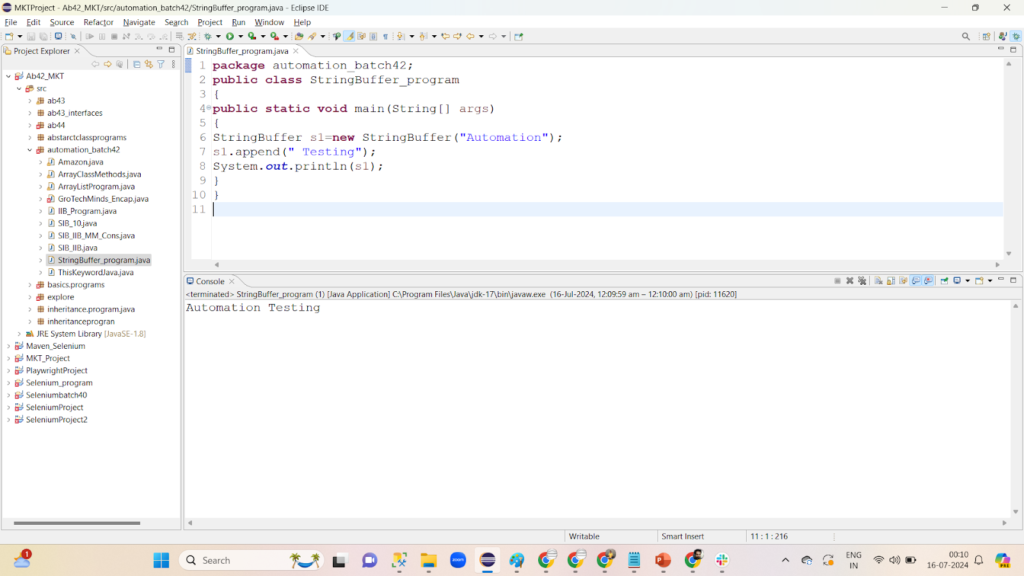
3. Guess the output of the program below?
package automation_batch42;
public class StringBuilder_Program {
public static void main(String[] args)
{
StringBuilder s1=new StringBuilder("Automation");
s1.append(" Testing");
System.out.println(s1);
}
}
Answer:
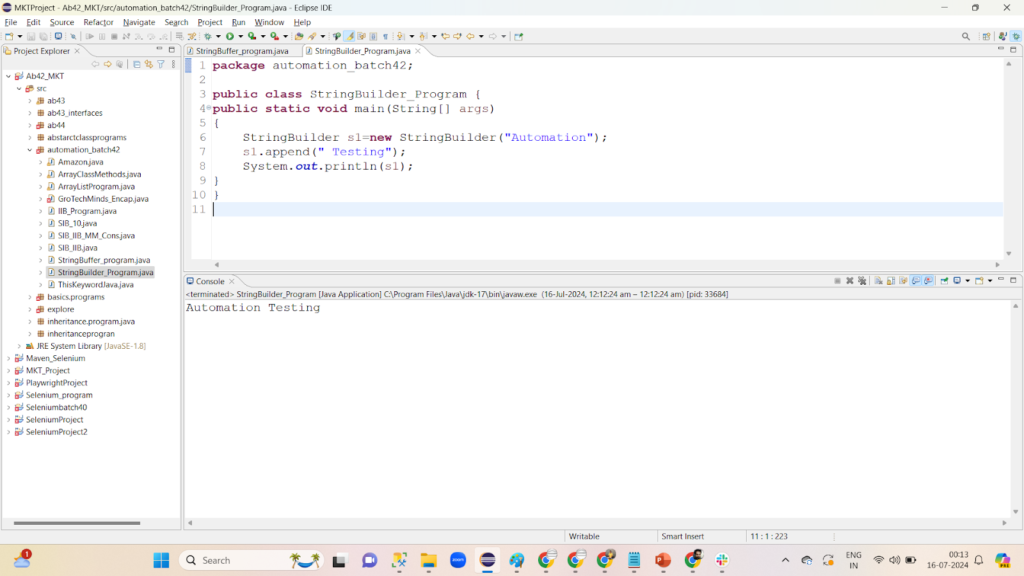
package selenium_Basic_Programs;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class BrokenLinks
{
public static void main(String[] args) throws IOException
{
ChromeDriver driver = new ChromeDriver();
driver.get("https://www.google.com/");
driver.manage().window().maximize();
List links = driver.findElements(By.tagName("a"));
//As we know all links will have tagname as a so we are locating each one of them
int links_Count = links.size();
//calculating the size of each links
System.out.println("Total Links are ->"+links_Count);
for(int i = 0; i "+c.getResponseMessage()+" -> "+"Link is valid");
}
else
{
System.out.println(c.getResponseCode()+" -> "+c.getResponseMessage()+" -> "+"Link is Invalid");
}
}
}
Answer:
Implicit Wait
- Waits for a certain amount of time before throwing a NoSuchElementException.
- Applies to all elements in the entire WebDriver instance.
- can be written just once
- driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
- Fixed (every 500 milliseconds by default).
- Least flexible, applies universally to all elements.
- Simple and quick wait setup for all elements.
Explicit Wait
- Waits for a certain condition to be true before proceeding.
- Applies to a specific element or condition.
- Set each time it is used.
- WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
- wait.until(ExpectedConditions.visibilityOfElementLocated(By.id(“elementId”)));
- Fixed (every 500 milliseconds by default).
- More flexible, specific to certain conditions or elements.
- Specific waiting conditions for individual elements.
Fluent Wait
- Waits for a certain condition to be true before proceeding with flexibility in polling frequency and exception handling.
- Applies to a specific element or condition.
- Set each time it is used.
- FluentWait<WebDriver> wait = new FluentWait<>(driver).withTimeout(Duration.ofSeconds(30)).pollingEvery(Duration.ofSeconds(5))
- .ignoring(NoSuchElementException.class);
- It is Configurable.
- Most flexible, allows custom polling intervals and exception handling.
- Advanced waiting scenarios requiring fine-tuned control over polling and exceptions.
6. How to select options of dropdown in Selenium using Keys?
package batch41.nightbatch;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class Amazon_Dropdown
{
public static void main(String[] args) {
ChromeDriver driver=new ChromeDriver();
//Launching chrome browser
driver.get("https://www.amazon.in");
//launching amazon India
driver.manage().window().maximize();
//Maximize your browser
WebElement dd=driver.findElement(By.id("searchDropdownBox"));
//locate your dropdown
dd.sendKeys(Keys.ARROW_DOWN);
//using Keys press arrow down button
dd.sendKeys(Keys.ARROW_DOWN);
//using Keys press arrow down button
dd.sendKeys(Keys.ARROW_DOWN);
//using Keys press arrow down button
dd.sendKeys(Keys.ARROW_DOWN);
//using Keys press arrow down button
}
}

package batch39;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class ScrollDown_youTube
{
@Test
public void youtube_page() throws InterruptedException
{
ChromeDriver driver=new ChromeDriver();
//Launching chrome browser
driver.get("https://www.amazon.in");
//launching amazon India
driver.manage().window().maximize();
//Maximise your browser
for(int i=0;i>-1;i++)
//keep running loop
{
JavascriptExecutor j1= driver;
//upcasting driver to JavascriptExecutor Interface
j1.executeScript("window.scrollBy(0,500)");
//Utilising JavascriptExecutor method executeScript
Thread.sleep(2000);
//After every scrolling give a sleep of 2 second
}
}
}
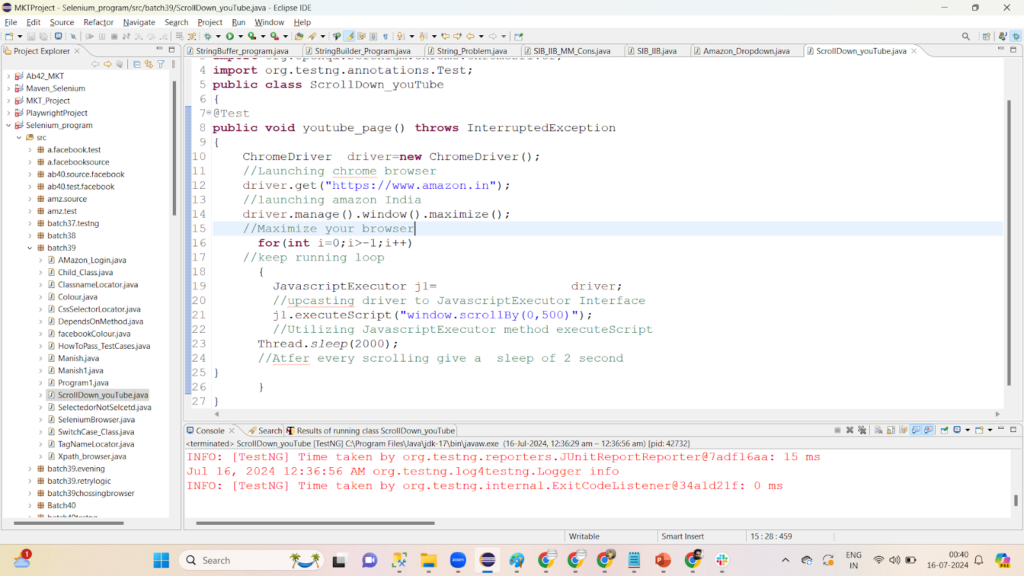
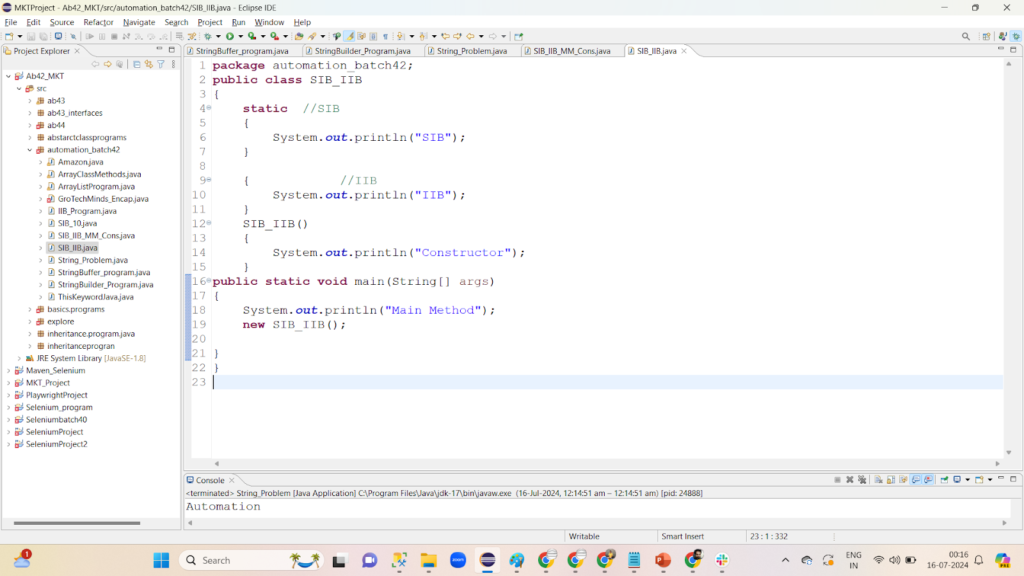
package automation_batch42;
public class SIB_IIB
{
static //SIB
{
System.out.println("SIB");
}
{ //IIB
System.out.println("IIB");
}
SIB_IIB()
{
System.out.println("Constructor");
}
public static void main(String[] args)
{
System.out.println("Main Method");
new SIB_IIB();
}
}
Answer: Whenever we have SIB,IIB,Constructor and Main Method present in a single program so output will always be SIB->Main Method->IIB->Constructor
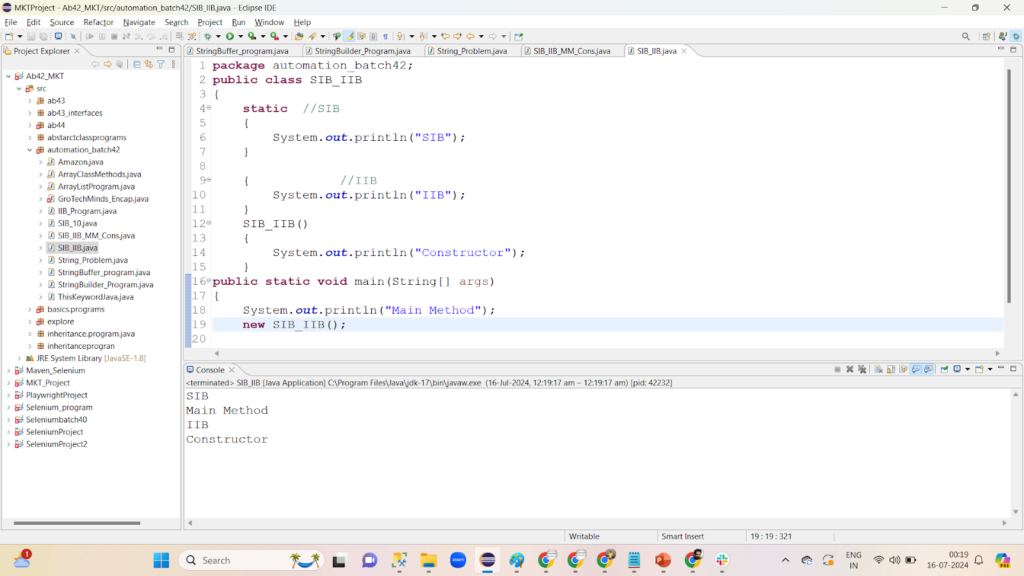
9. Guess the output of the program below?
package automation_batch42;
public class String_Problem
{
public static void main(String[] args)
{
String s1=new String("Automation");
s1.concat(" Testing");
System.out.println(s1);
}
}
Answer:
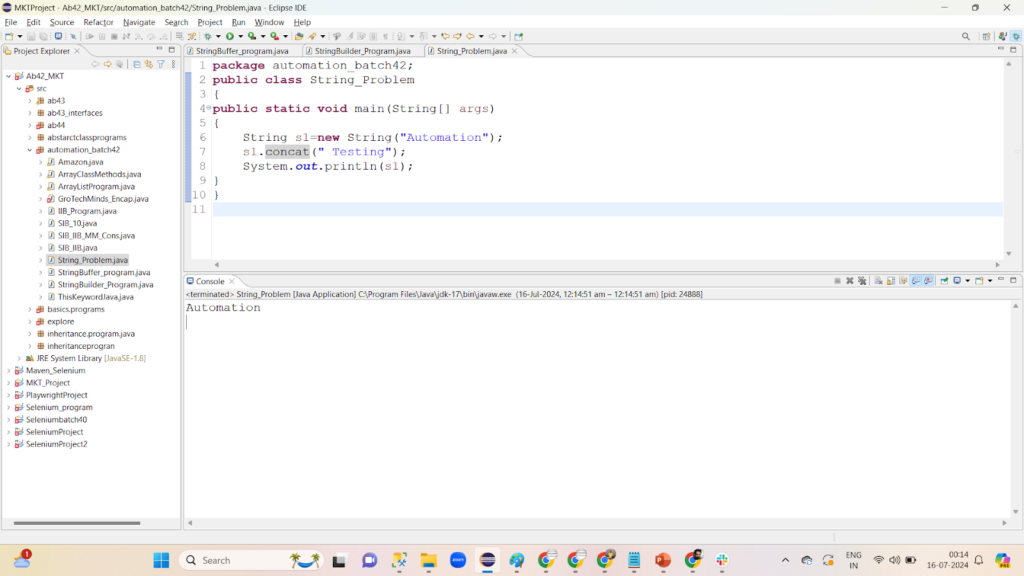
Answer:
- Can’t Automate Mobile based or Standalone application
- Can’t Automate OTP, Captcha, Barcode.
- Timely new new update from Selenium
- Can’t test Image
Answer:
Table For AND Operator | c1 | c2 | OUTPUT |
TRUE | FALSE | FALSE | |
TRUE | TRUE | TRUE | |
FALSE | TRUE | FALSE | |
FALSE | FALSE | FALSE |
Table For OR Operator | c1 | c2 | OUTPUT |
TRUE | FALSE | TRUE | |
TRUE | TRUE | TRUE | |
FALSE | TRUE | TRUE | |
FALSE | FALSE | FALSE |
Answer:
Functional Testing | Non Functional Testing | |
Testing the Functionality of the application by executing test cases is called Function Testing. | Testing the speed of the application,Reliable of the application,Recovery,compatibility of the application,Web security of the application ,Response time of the application etc | |
Ex:Smoke Testing,Component testing,Integration Testing,System Testing,Acceptance testing,Retesting,Regression Testing etc | Ex: Recovery Testing,Performance Testing, Compatibility Testing, Reliable Testing,Web Security Testing | |
Should be done first | Should be done later | |
It doesn’t need a tool to do it | IT needs a tool to do it. Ex: Jmeter-Performance testing, BurpSuite-Web security Testing |
|
We write the test cases for it | We write the test cases for it | |
It is Mandatory to do in any application | Non Functional Testing we do to make our customer happy |
Answer:
- Env issues[Tester raised the bug in testing env buy dev is reproducing it in dev env]
- If both tester and developer are not testing the same build
- Also because of compatibility issue Tester got the issue in Browser-Chrome 113, OS-Windows and Developer got the issue in OS-Windows, Firefox browser
- If tester have not mentioned the steps to reproduce clearly
Answer:
Blocker :
- If it is completely breaking the major functionality of the application
- If your basic or critical flow is breaking it is blocker bug
- If any page is redirecting to blank page
Example::
- a) Video feature not working in zoom application
- b) In whatsapp is text messages are not being sent
- c) In whatsapp->Registration is not happening
Critical: If a user is not blocked to use a feature but the feature is not working as per the requirement is called as Critical Severity Issue.
Example:
a) . In zoom application if recording is made to happen in cloud but happening in local.
b). If user A sent 10 currency to user B , user A got only 9.9 , 0.1 currency is deducted by the bank.
c). In whatsapp user A is sending 10 images but user B got 11 images [1 image duplicate]
Major:
- If you think something is missing in the application can be said to be a major issue.
- All the notification issues are always major issues only
Example:
- When you send email to user B but no message has been popped up.[Email has been sent]
- While booking cab , user A is not able to track the location of driver
- On ordering a product on amazon , user A has not received any sms confirmation
- After changing password , did not receive the password change notification
Minor: These issue are not impacting your application at all but these are some look and feel issue, scrolling bar issue, spelling mistake issue, compatibility issue etc
Example:
- In amazon application under about us, spelling of customer is wrong
- In Edge browser facebook application colour is looking very dull.
Answer:
Delete | Drop | Truncate | |
Definition | It deletes the row | It deletes the table including the structure of the table. | It deletes the table but not the structure of the table. |
Type | Data Manipulation Language | Data Definition Language | Data Control Language |
Structure | No impact on structure | yes there is impact on structure | No impact on structure |
Permanent/Temporary | Temporary Change | Permanent Change | Permanent Change |
Transaction Control Language | Yes | No | No |
Syntax | delete from TableName Condition if any; |
drop table TN; | truncate table TN; |
Answer:
What happens within the class? | What happens within the package? | What happens outside the package by becoming a subclass? | What happens outside the package without becoming sub class? | |
public | TRUE | TRUE | TRUE | TRUE |
protected | TRUE | TRUE | TRUE | FALSE |
default/package | TRUE | TRUE | FALSE | FALSE |
private | TRUE | FALSE | FALSE | FALSE |
Answer:
package basics.programs;
public class StringFunctions
{
public static void main(String[] args)
{
String a="Manish";
System.out.println(a.matches("M(.*)"));
//here we are checking if String a starts with M o not
System.out.println(a.equals("Manish"));
//here we are checking if String a is same as Manish
System.out.println(a.equalsIgnoreCase("manish"));
//here we are checking if Manish is same as manish[if we ignore its upper and lower case]
System.out.println(a.contains("ish"));
//here we are checking if String a have "ish" or not in it
}
}
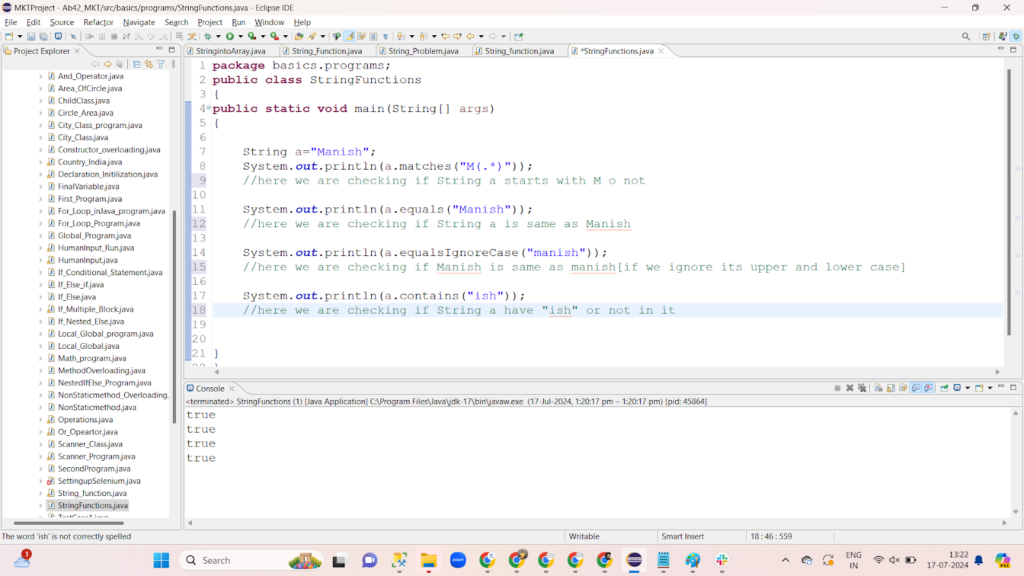
Answer:
toCharArray() can be used in Java to convert string to a new character array.
Below is the program for your reference:
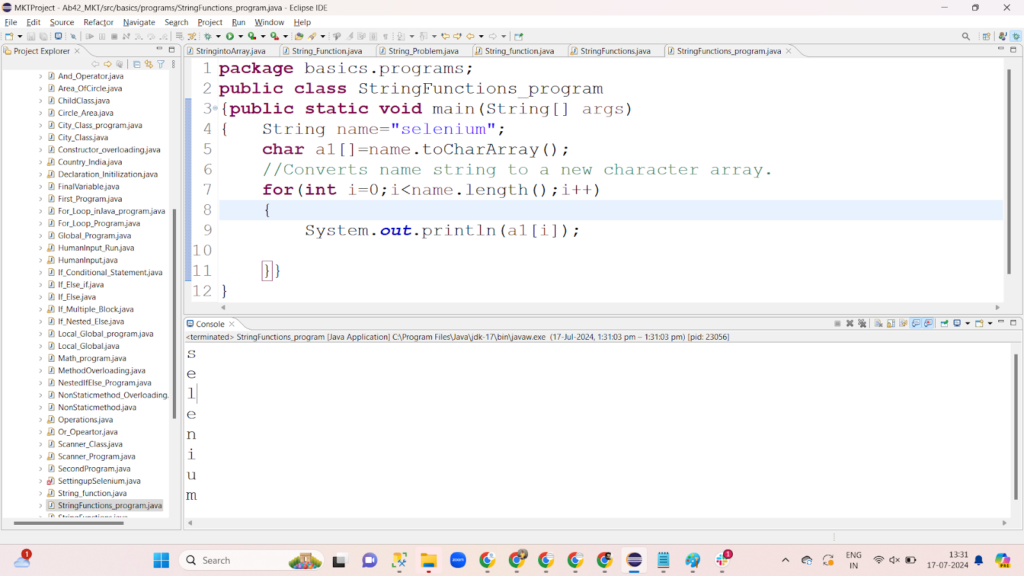
This is another way to print the value of char array.
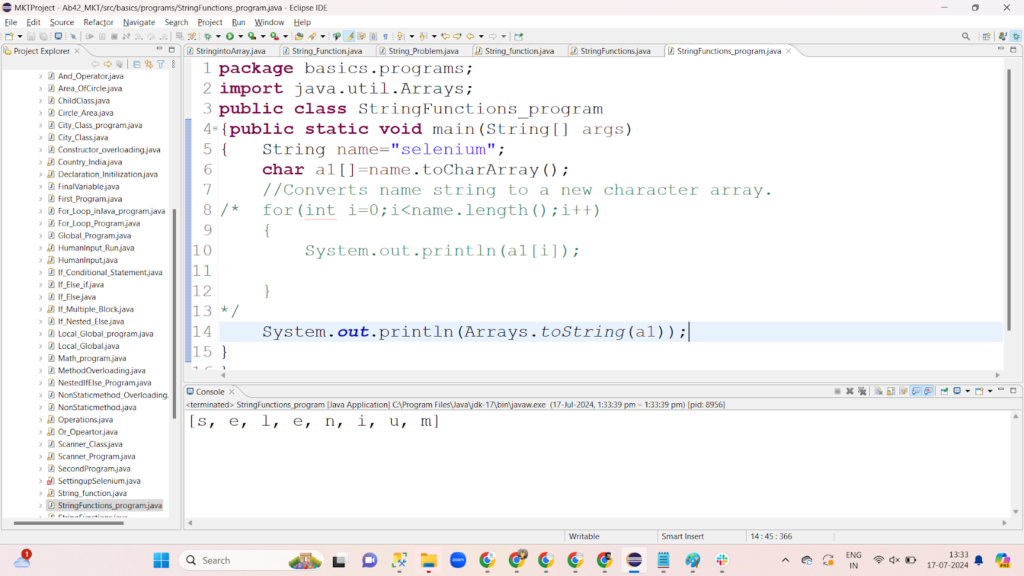
Answer:
- Which ever line is giving you exception should be in try block
- And it must be handled in the catch block
- Writing exception handling code doesn’t mean exception will come,it may come or it may not come
- If exception is coming then try block will not execute and catch block will execute
Example of Exceptional Handling:
try
{
System.out.println(""hello"");
int c=1/0;
System.out.println(c);
}
catch(ArithmeticException a)
{
System.out.println("I have handled the exception");
}
e). If exception is not coming then try block will execute and catch block will not execute
f). For every try block we can have single catch block also and many catch block also
Exception handling is the code to handle any runtime exception in our code.
To handle the exception, we need try,catch block.Finally block also there.Try block: It will execute when error not occur.
Catch block: It will execute if exception occur in program
Syntax of try block:try
{
}catch(ExceptionName variable)
{
}We can use ‘throw’ and ‘throws’ keywords also in exception handling.
throws — With the help of ‘throws’ we can tell that particular method has some exception.’ throws’ keyword can be use for multiple exception. It always written together with method name.
throw–single ‘throw’ can be use for single exception
It always written inside methodSyntax:void add() throws ExceptionName
{
statement 1
statement 2
statement 3
throw ExceptionName}
Answer:
- Fixed size
- Homogeneous value only
- You can’t change the value of array size at run time
Answer:
Yes I know how to push my code to github from a local repository.
- First we need to make sure in our laptop we have installed the git bash.
- We must have a github account.
- We must do following steps to push your code to git repository:
- Make sure in your github you have created the repository
- Now In your local do cloning of remote repository with local repository
- Do git status to check if what has to be pushed
- Git add it
- Git commit it
- Git Push it
The git syntax are:
Git clone repository_url.git
Git status
Git add . or git add filename
Git push –all repository_url.git
Answer:
-
We do regression testing when there is a addition of the new feature or removing the feature or modifying the feature or the bug fixes that should not have any impact on the existing features then we will be doing regression testing
-
Testing the unchanged feature to make sure that addition of new feature,deletion of the feature or any modification or the bug fixes to ensure that it should have any impact on the unchanged feature is called as regression testing
-
Based on the sprint analysis meeting with developer will decide the which type of regression testing should follow
<test>
<suite>
<class>
<methods>
<classes>
Answer:
The correct order is:
- <suite>
- <test>
- <classes>
- <class>
- <methods>
Answer: Any language that shows the properties of Class, Object, Abstraction, Polymorphism, Inheritance and Encapsulation is called as Object Oriented Programming Language.
Ex: Java, C#, Ruby, Python, TypeScript, and PHP etc.
Languages which are not Object Oriented Programming Languages are
Ex: Fortan, Algol, Cobol, Basic, Pascal, C, Ada
Answer:
class ArrayProgram {
public static void main(String[] args) {
int no[]= {10,5,10,15};
int sum=0;
for(int i=0;i<=no.length-1;i++)
{
sum=sum+no[i];
}
System.out.println(sum);
double average=sum/no.length;
double reminder=sum%no.length;
System.out.println(average);
System.out.println(reminder);
}
}
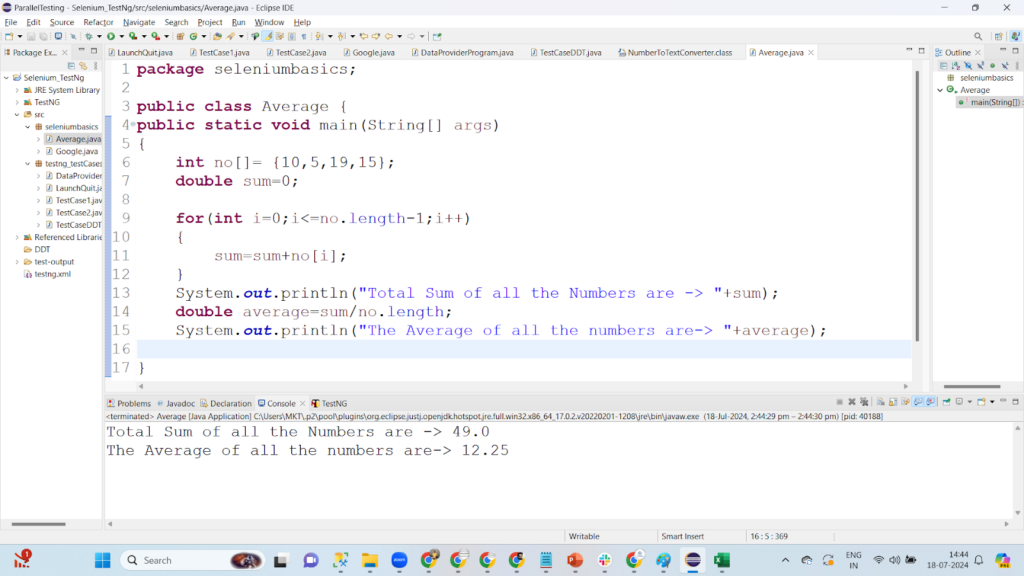
Answer:
package seleniumbasics;
import java.util.Arrays;
public class CopyArray
{
public static void main(String[] args)
{
int no[]= {543,76,98};
int newarray[]=new int[3];
for(int i=0;i<=no.length-1;i++)
{
newarray[i]=no[i];
}
System.out.println("The new Array is:");
System.out.println(Arrays.toString(newarray) );
System.out.println("The old Array is:");
System.out.println(Arrays.toString(no) );
}
}
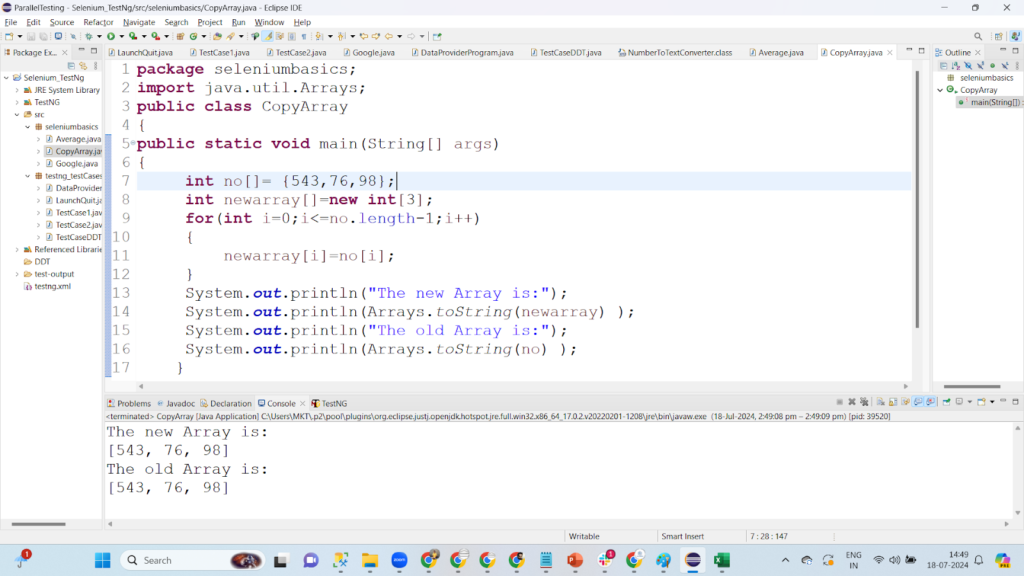
Answer:
ReTesting | Regression Testing | |
After dev fix we can do this to confirm if a bug is really fixed. | Testing the unchanged feature of the application because of changes like Adding a feature,Removing a feature,Fixing a bug or Code modification is called Regression testing to insure the unchanged part of the application is not impacted. | |
This has to be done first | This has to be done later | |
We can’t automate this | We can automate Regression Suite | |
We do ReTesting for failed Test Cases | We do Regression for pass Test Cases | |
It takes less time | It takes more time | |
Retesting can be done by both developer and Tester | Regression Testing should be done by Testers only. |
Answer: It can be accessed within the package and outside the package also.
Answer: Yes we can do it.
Collection c1=new ArrayList();
Answer:
- if the bug that you have raised is according to the previous requirement
- Any meaning less bug that you have raised[Its not a bug]
Answer: When we are releasing the software into the production, we will give documents to client, that document is called release notes.
Release notes consists of :
- Procedure for how to install the software.
- Procedure for how to uninstall the software.
- What are the bug fixes done.
- What are the bugs we are releasing with software.
- What are the features we are giving.
- What are the features deleted.
Answer: We will review the test cases on below based on below factors:
a). If tester have followed the test case template or not.
b). If tester have followed the simple English language
c). If the tester covered all the scenarios (Both positive and Negative)
d). If the tester has covered test case coverage.
Answer: To disable a test in TestNG, we have to use the “enabled” attribute as follows:
@Test(enabled=”false”)
By default the enabled will be equals to true.
Answer:
- We cannot develop 2 super calling statement in subcass to call the super class constructor which violates the rule here.
- Sublass will have the ambiqity which super class property need to be utiise which leads to diamond problem.
- since The Object class is the parent class of all the classes in java by default.
For | While |
When we are aware of the number of iteration we can follow for loop | When we don’t know the number of iteration we go for while loop |
Syntax: |
Syntax: declaration while(Condition) { Your logic increment/decrement operator; } |
Also read : Java Interview Questions and Answers
Answer: dependsOnMethods parameter is used in TestNG to make sure a particular test case only runs if the other test case[on which it is depending] is success else it will be skip.
package batch37.testng;
import org.testng.annotations.Test;
public class Class1
{
@Test
public void login()
{
}
@Test(dependsOnMethods="login")
public void logout()
{
}
}
In the above program testcase logout is depending on testcase login which means logout will only be starting if login will be successful.
Else logout test case will be skipped.
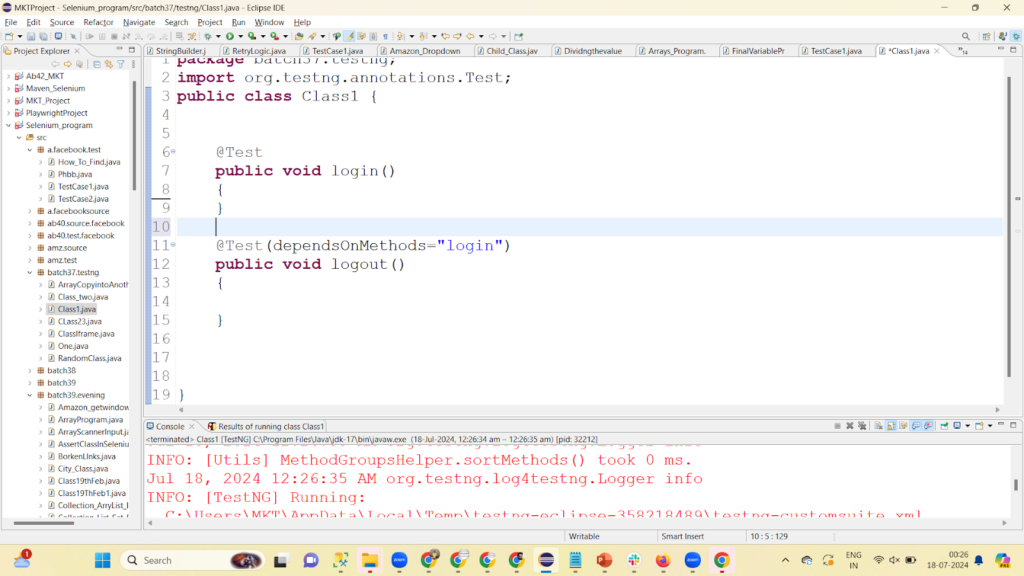
Answer:
- Before Suite
- Before Test
- Before Class
- Before Method
- Test
- After Method
- After Class
- After Test
- After Suite
- Bugs that we release into the production intentionally because of its lower Severity and Lower Priority is called a Bug Release.
- Bugs that have been leaked into the production environment from the Testing Environment unintentionally are called Bug Leakage.
Answer: After running many test cases in TestNG together if one of the test case is failing we can run the testng-failed.xml file as shown below.

Answer:
Error | Mistakes done in the programs which results into Compile time error or Run Time error |
Defect | If developer found a issue in development phase itself results into Defect |
Bug | If testers find any mistake between actual and expected behaviour results into bug in the Testing Environment |
Failure | If enduser/customer found any issue |
Answer: 508 compliance testing is accessibility testing to make sure individuals with disabilities can access and use your software.
Answer: It is an interface and it consist of 17 abstract method those areclear, findelement, findelements, getlocation, size, isenabled, sendkeys,getscreenshotAs ,gettext,. click, isdisplayed, isselected etc
Answer: We can press Enter button it using Keys Functionality.
package seleniumbasics;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.chrome.ChromeDriver;
public class Google
{
public static void main(String[] args)
{
ChromeDriver driver=new ChromeDriver();
driver.get("https://www.google.com/");
driver.manage().window().maximize();
driver.findElement(By.linkText("Gmail")).sendKeys(Keys.ENTER);
}
}
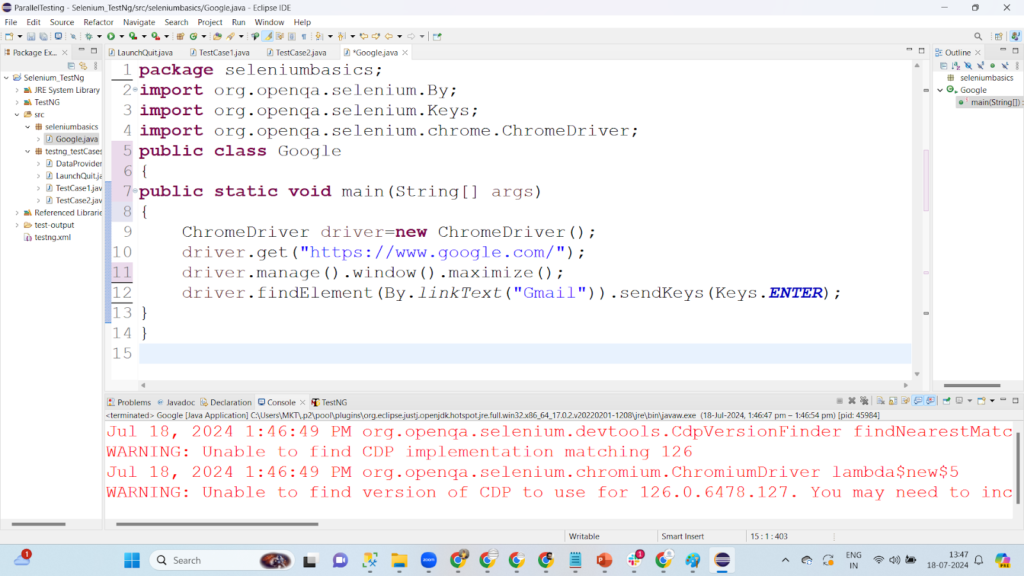
Answer:
SL no | Bug | Severity | Priority |
1 | Login is not working in amazon with valid un | Blocker | p0 |
2 | Logout is not working in net banking application | Blocker | p0 |
3 | In amazon application Cash on delivery is not working | Blocker | p0 |
4 | Registration is not happening in gmail application | Blocker | p0 |
5 | Zoom application audio is not working while listening to the recording | Blocker | p0 |
6 | In UPI application money is getting transferred but always 1 INR less | Critical | P0 |
7 | In amazon application logo has replaced with google logo | Minor | P0 |
8 | In amazon India application under about us page-spelling of customer is wrong | Minor | P2 |
9 | On Facebook log in page, the spelling of Facebook is wrong | Minor | P0 |
10 | On gmail application about us is redirecting us to the blank page | Blocker | p1 |
11 | In whatsapp application call got disconnected but there is no message for that | Major | p0 |
12 | In zoom application zoom chats notification is not working for any image only | Major | p1 |
13 | I sent you one email on gmail application but notification is not working for browser only | Major | p0 |
14 | In zoom application Pinned is not working | Critical | P0 |
15 | Even after locking the meeting by the host participants are able to join the meet | Critical | P0 |
16 | In zoom application scheduling a meeting and inviting the participants is not working by gmail | Critical | P0 |
17 | Zoom application mute feature is not working | Blocker | P0 |
18 | Calculations mistake of yearly report of salary calculations in the application finance application | Critical | P0 |
19 | An application not used by many people,contact us is redirecting us to the blank page | Blocker | P2 |
Answer:
- As we know selenium versions keep changing so to get the latest update it becomes easy to work on maven because we just need to update the latest version ID.
- Often used when a very large number of dependencies are needed in the project.
Answer:
- TestNG allows you to conveniently group test cases based on testing types.
- TestNG has extensive reporting options.
- TestNG allows you to run many test cases together.
Answer:
Just with Id | #ID_value |
With just class name | .Classname_value |
tag and id | tag#id |
tag and class | tag.class |
Just with arrribute and its value | [attribute=’value’] |
Wth tagname and classvalue | tagname.classname_value |
tag and attribute | tag[attribute=value] |
tag ,class and attribute | tag.class[attribute=value] |
Using SubString | Tagname[AttributrName^=’AV’] |
- Just with Id
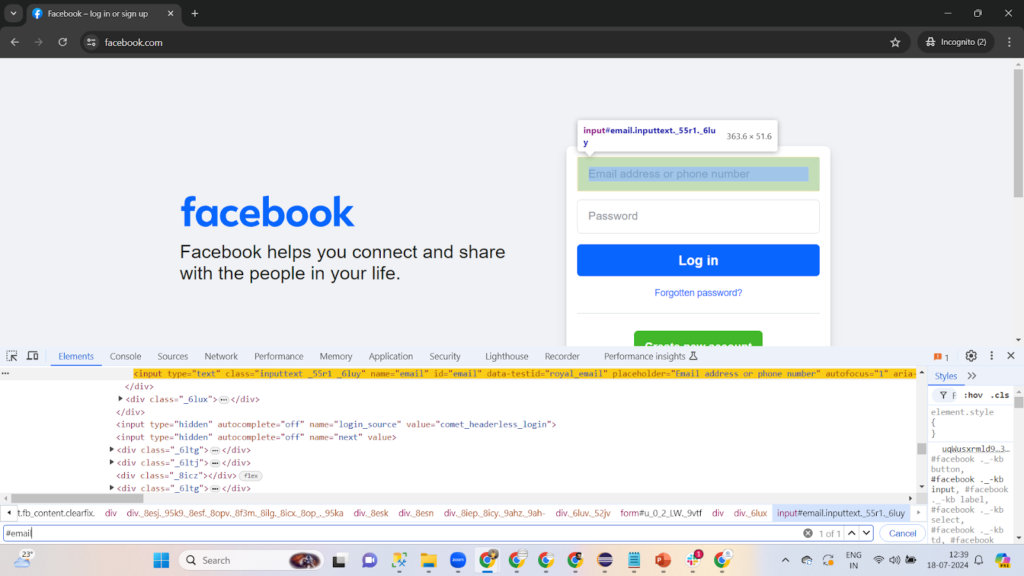
2. Just with Class Name
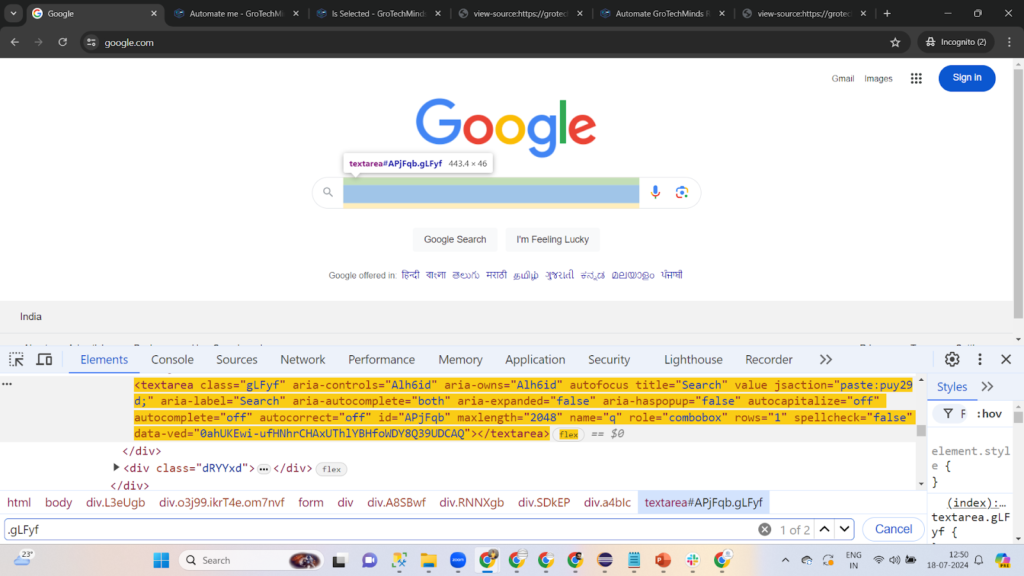
3. Tagname and Id
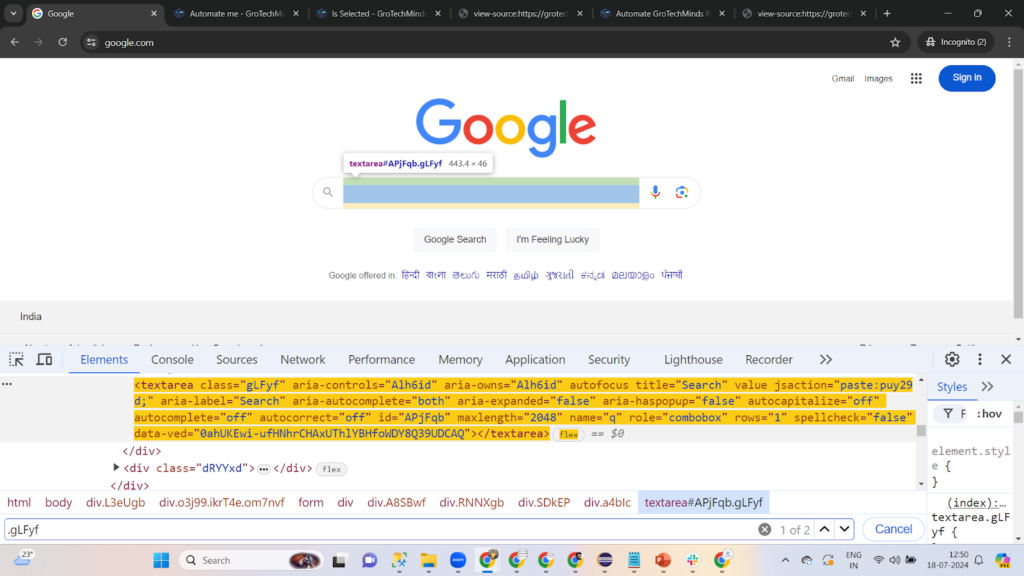
4. Tagname and ClassName
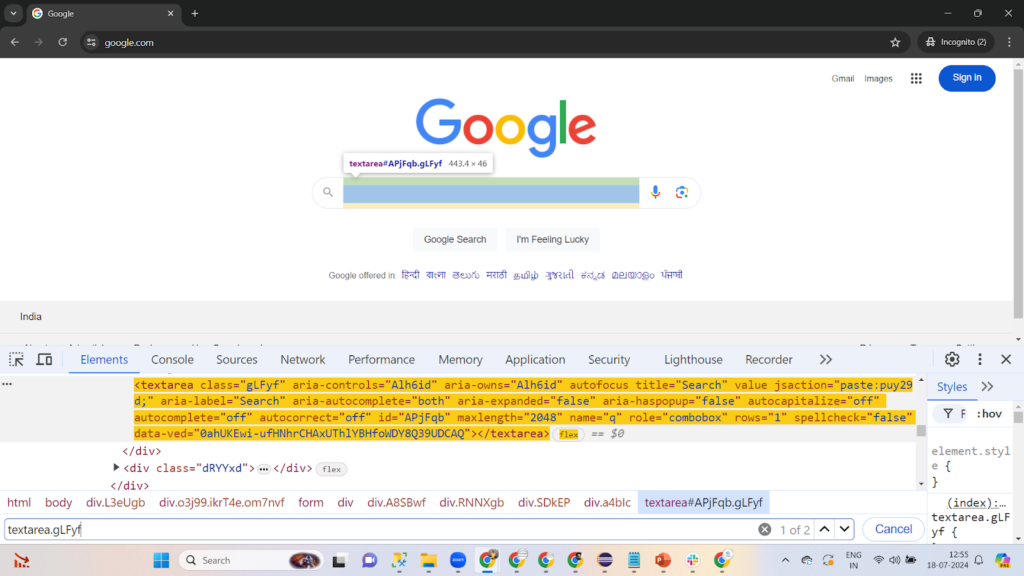
5. Attribute name and its value
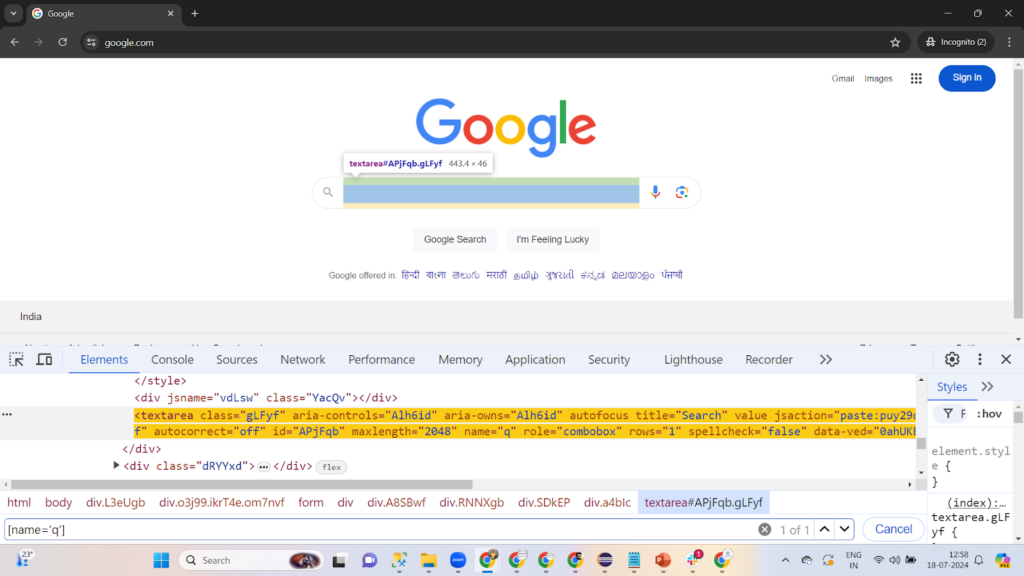
6. With tagname and ClassName Value
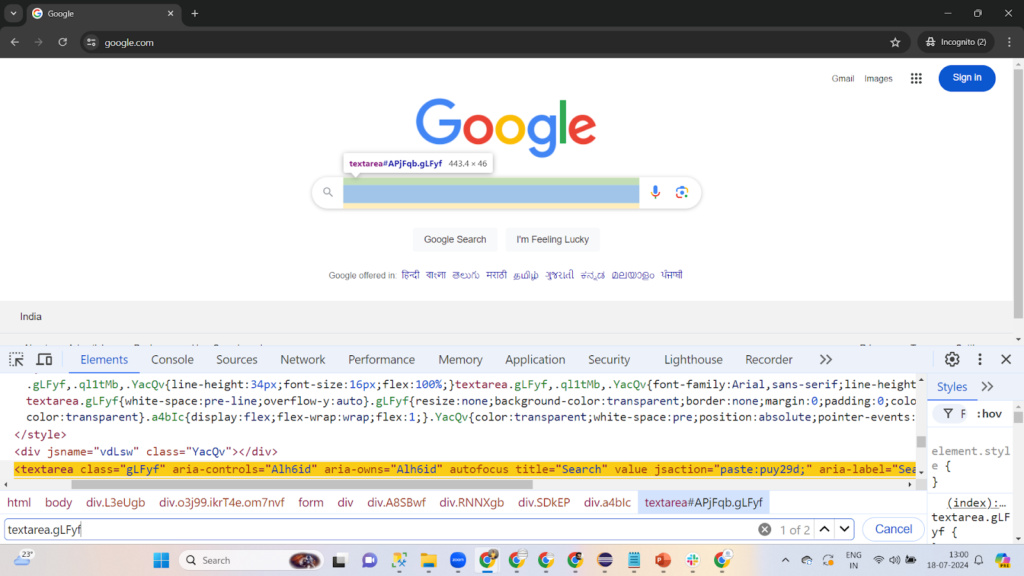
7. Tagname and Attribute
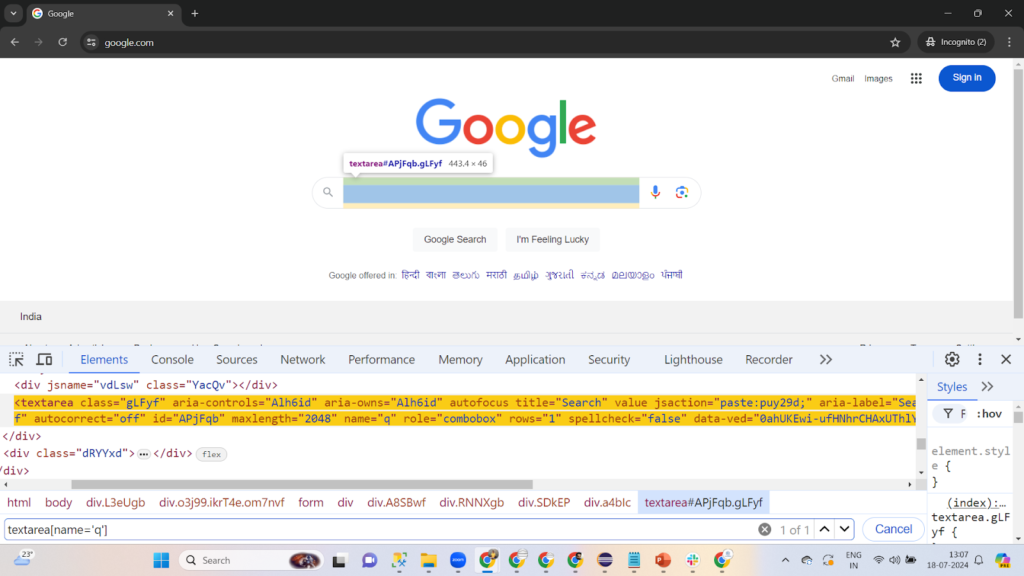
8. Tagname,Classname and Attribute
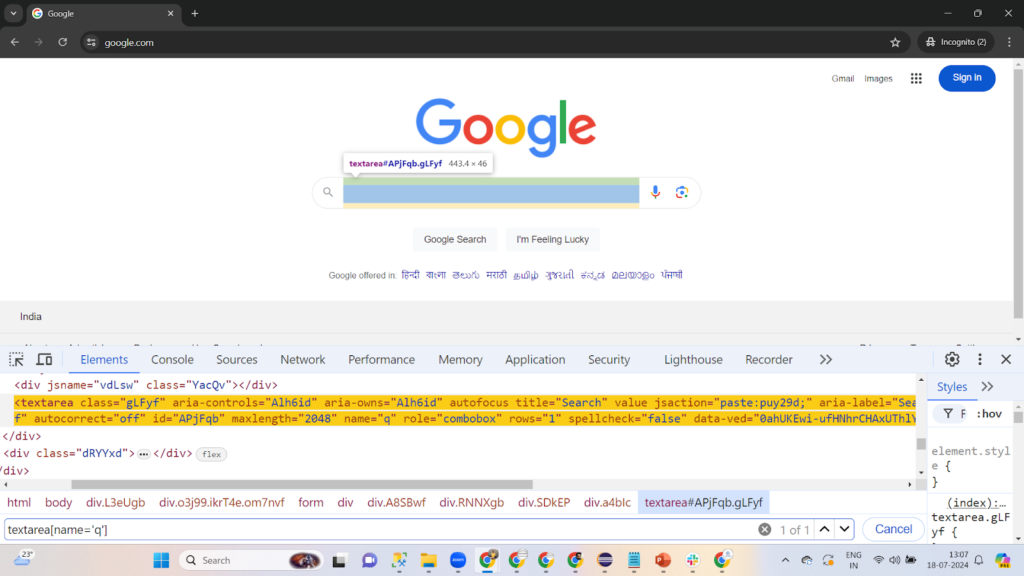
9. Using Substring
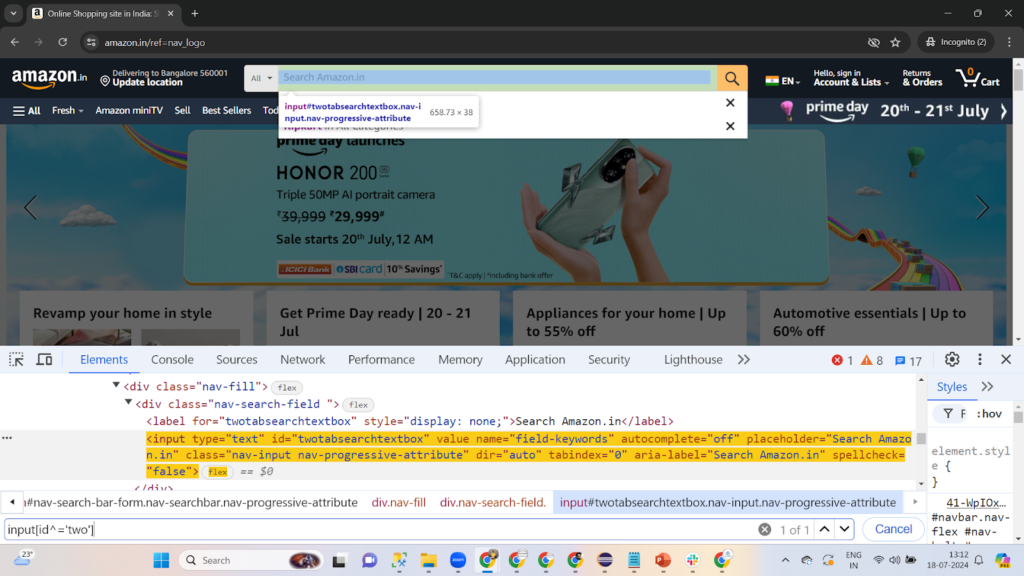
Answer: In the case of DDT(Data Driven Testing) if we fetch the numeric value from cell we get an exception called as IllegalStateException.
package testng_testCases;
import java.io.FileInputStream;
import java.io.IOException;
import org.apache.poi.EncryptedDocumentException;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
public class TestCaseDDT
{
public static void main(String[] args) throws EncryptedDocumentException, IOException
{
FileInputStream f1 = new FileInputStream("E:\\MKTProject\\ParallelTesting\\Selenium_TestNg\\DDT\\login.xlsx");
Workbook w1= WorkbookFactory.create(f1);
Sheet s1= w1.getSheet("Credentails");
String un= s1.getRow(0).getCell(1).getStringCellValue();
System.out.println(un);
String pwd= s1.getRow(1).getCell(1).getStringCellValue();
System.out.println(pwd);
}
}
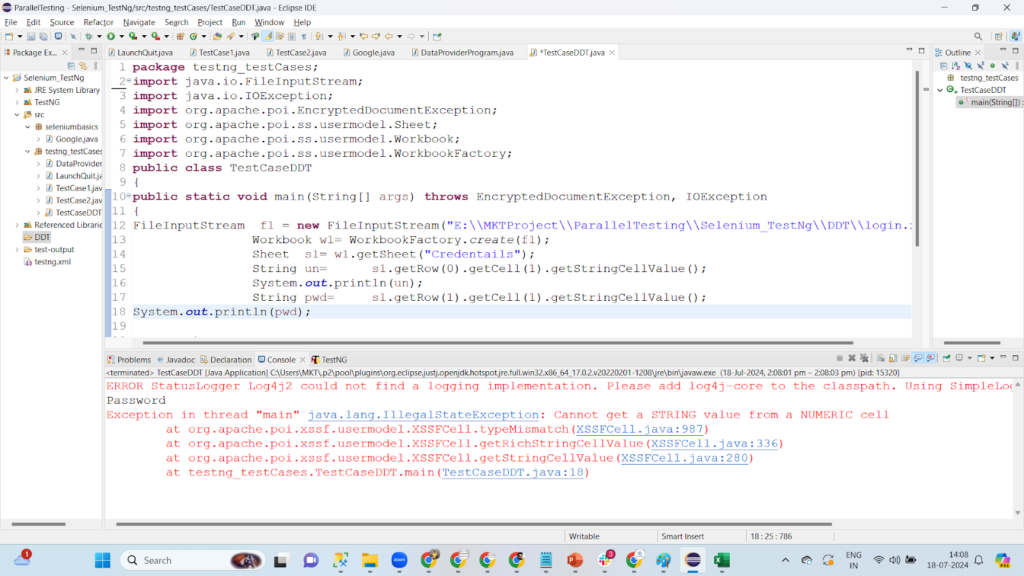
The excel sheet is like this:

So we need to fix this using the NumberToTextConverter class.
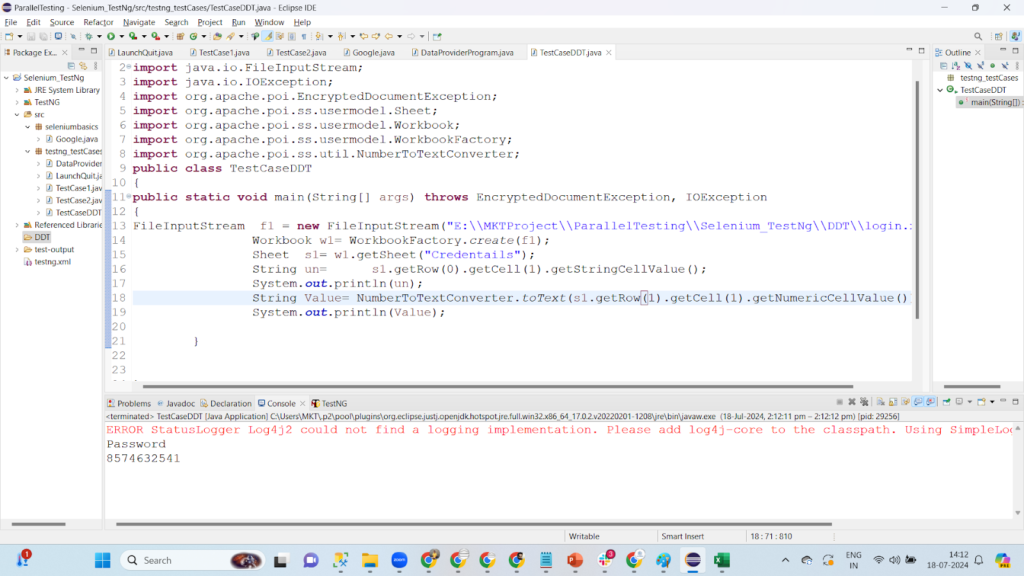
Answer:
- If the Response body is correct
- If the every Object is Coming properly
- If the every Objects have every key and values present
- If the status code is proper
- If the response time is as per the requirement
- If the response headers are correct
- If the response body size is as per the expectations
- If the the api reflection is in the Database
- Application Logs
- If the response body format is as per the expectations
Answer: In a Maven project, pom.xml (Project Object Model) is the fundamental configuration file that defines the project’s configuration, dependencies, plugins. This XML file is located in the root directory of the project and is used by Maven to manage the project’s build
Types | Commands |
Data Query Language[DQL] | Select |
Data Definition Language[DDL] | Create,Alter,Truncate,Drop,Rename |
Data Manipulation Language[DML | Insert,Update,Delete |
Transaction Control Language[TCL] | Rollback,SavePoint,Commit |
Data Control Language[DCL] | Grant,Revoke |
Answer:
Every bug will have the following components:
Title, Description, Severity, Priority, Attachment, Environment Details, Assign .
- Title: It should be short and crisp aout your bug in less than 20 to 40 characters.
Ex: User is not able to send text message
- Description: Under this you can write the steps here so that it will be easy for developers to raise a bug. Under Description You can also mention the pre condition, Expected Behavior and Actual Behaviour.
- Severity: Here testers need to select the severity from the blocker,major,minor and critical.
- Priority: Here the tester needs to select the priority from p0,p1 and p2.
- Attachment: Here the tester can attach any screenshot or video if he/she have.
- Environment Details: Tester can mention the environment details of the bug.
Ex: Testing Environment. OS: Windows 11, Browser: Google Chrome 125
- Assign: Here we can select the developer name.
Here is the sample Bug Steps:
Title: User is not able to send text message
Description:
Pre Condition:
- Internet connection is required
- Both user 1 and user 2 must be registered
- Both user 1 and user 2 must be logged in
Steps To Reproduce:
- Open the whatsapp app
- Tap on contact details
- Clicking on search text field and typing user 2 name
- Tap on user 2 name
- Type the message in the message text area field.
- Tap on the send button
Expected Behaviour:
- Message from user 1 should now be sent to user 2 whatsapp account
- user should the notification of the message received
- User 1 should be able to see the double click for the message.
Actual Behaviour:
- User 2 is not receiving messages from user 1.
Severity: Blocker
Priority: P0
Attachment: We can attach the screenshot here
Environment Details: Testing Environment, OS: Android 14
Assign: Suman Malik[Developer]
Answer:
- Tester will write the test scenarios and test cases
- Tester will send it to reviewer for review
- Reviewer will review it, check it and give comment if required and send it back to tester
- Tester will check the review comment and fix it.
- Reviewer will review it and if it is ok, he will send it to the approver to approve test cases.
- If the test case is not fixed, the reviewer will send it back to the tester to fix the comment.
- Approved will approve the test cases
- Tester will execute the test cases
- Tester will create a test report accordingly.
Answer:
- Beta Testing in which the testing server is similar to a production server but with no real traffic.
- It is done after doing complete testing in a testing environment before giving the software to the customer to use the software in the production environment.
Answer: Classes can never be private in Java.
Also read :
Consult Us