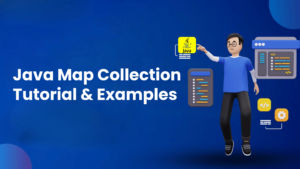
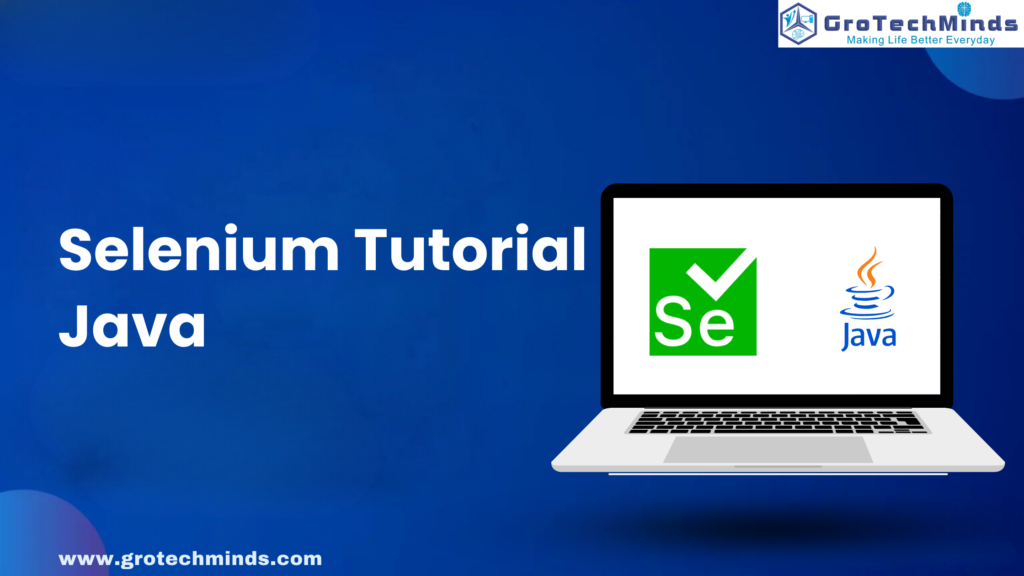
Introduction to Selenium Java
A popular tool to figure out whether web-based applications are functioning as intended is Selenium testing. It is widely preferred by testers for cross-browser testing and is thought to be among the most reliable solutions for assessing web application automation. Because Selenium is platform-neutral, it can do distributed testing over the Selenium Network.
Automation scripts are created by the user. These scripts include directions and instructions for interacting with web pages and web elements.
This module serves as a conduit between WebDriver and user code. It offers multiple APIs that enable web browser control and web interaction instructions to be facilitated by user code.
Custom instructions are defined for internet interfaces by the WebDriver API. Many browser makers have implemented WebDriver implementations of their own that work well with this API. It creates a general vocabulary for browser automation.
Every browser has its own WebDriver implementation (ChromeDriver, GeckoDriver, for example), such as Firefox and Chrome. These drivers are in charge of opening a particular browser and creating a channel of communication with the WebDriver.
The Webdriver gives the browser instructions on how to perform particular tasks, such as clicking parts or entering text, and the browser complies.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class SimpleTestScript {
public static void main(String[] args) {
// Set the path to ChromeDriver executable
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// Instantiate ChromeDriver
WebDriver driver = new ChromeDriver();
// Open a webpage
driver.get("https://www.example.com");
// Perform basic interactions
// For example, clicking a link
// driver.findElement(By.linkText("Some Link")).click();
// Print the title of the page
System.out.println("Page title: " + driver.getTitle());
// Close the browser
driver.quit();
}
}
The quickest and most recommended method for finding required WebElements on the page is to use Selenium’s ID locator. Every element in the DOM has a different ID location.
It is said to be the quickest and safest way to discover elements because IDs are unique for every element on the page. Regretfully, since browsers permit circumvention of this restriction, developers might or might not abide by it.
In particular, based on the data in the database, the IDs in a list or table may populate progressively or dynamically. In these situations, Selenium WebDriver’s other locators are used by testers to find the desired element on the page.
Since the ID locator in Selenium is the fastest locator available, making use of its features is one of the best practices for using Selenium. Therefore, selecting the ID locator in Selenium WebDriver over alternative locators will significantly accelerate the execution of Selenium test cases.
Name is one of the attributes that can be used to define an element. Similar to an ID locator, a Name locator in Selenium WebDriver may also detect elements.
The Name locator may or may not have a unique value, in contrast to ID locators, which are exclusive to a page. The locator chooses the first element on the page with that name if there are many WebElements with the same name.
Web pages are styled using CSS (Cascading Style Sheets). In addition, CSS is one of the most used methods for locating WebElements within the Document Object Model.
If you are unable to find an element using the ID or Name locators, you should use Selenium’s CSS Selector. It is an option instead of the XPath locator.
Their application could differ depending on how complex the situation is, as they both frequently engage in arguments. However, because CSS Selectors are faster than XPath, the majority of users prefer to use them.
As other locating methods—such as ID, class name, or name attribute—fail, XPath is a useful tool for finding elements on a webpage.
A language for queries called XPath (XML Path Language) is used to go through XML documents and choose components. When it comes to online automation, XPath makes it possible to go through a page’s HTML structure and locate certain items according to their properties, hierarchy, or connections to other elements.
Explanation of implicit and explicit waits in Selenium WebDriver with Java.
When it comes to running Selenium tests, the wait commands are crucial. They help in the observation and resolution of problems that might result from variations in time lag.
It is typical for testers to get the error message “Element Not Visible Exception” when executing Selenium tests. This shows up when the loading of a specific web element that WebDriver needs to interact with takes longer than expected. The use of Selenium Wait Commands is required to stop this Exception.
Selenium Webdriver wait instructions tell test execution to halt for a certain period of time before proceeding to the next stage in automated testing. This allows WebDriver to determine whether one or more web items are visible, clickable, enhanced, or otherwise present.
The Selenium WebDriver can be instructed to wait a specific amount of time before throwing an exception by using the implicit wait command. WebDriver will wait for the element before the exception occurs once this time is configured.
Implicit Wait lasts for the length that the browser is open after the command is executed. Its default value is 0, and the protocol that follows must be used to determine the precise wait duration.
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import java.util.concurrent.TimeUnit;
public class ImplicitWaitExample {
public static void main(String[] args) {
// Set the path to the geckodriver executable
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
// Create a new instance of the Firefox driver
WebDriver driver = new FirefoxDriver();
// Set the implicit wait time to 10 seconds
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
// Navigate to a webpage
driver.get("http://example.com");
// Try to find an element (the driver will wait up to 10 seconds before throwing a NoSuchElementException)
WebElement element = driver.findElement(By.id("some-element-id"));
// Do something with the element
element.click();
// Close the driver
driver.quit();
}
}
The WebDriver can be told to pause code execution until a certain condition is met by utilising the Explicit Wait command.
It’s crucial to set an explicit wait when some parts load more slowly than others by nature. The browser will wait the same amount of time before loading each web element if an implicit wait command is set. The test script’s execution is needlessly delayed as a result of this.
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWaitExample {
public static void main(String[] args) {
// Set the path to the geckodriver executable
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
// Create a new instance of the Firefox driver
WebDriver driver = new FirefoxDriver();
// Navigate to a webpage
driver.get("http://example.com");
// Set an explicit wait of up to 10 seconds
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(
ExpectedConditions.presenceOfElementLocated(By.id("some-element-id"))
);
// Do something with the element
element.click();
// Close the driver
driver.quit();
}
}
Implicit Wait | Explicit Wait |
With the WebDriver instance, implicit wait is set globally, and it impacts all following interactions with web elements. Unless overridden, it is in effect for the duration of the WebDriver session after it is set. | Clearly When more time is needed for certain web element interactions, wait is applied selectively. It is more focused and detailed since it lets you provide a wait condition for a specific element or group of components. |
Implicit Wait waits for an amount of time that is set by the driver.handle().timeouts().implicitlyWait()) before throwing a NoSuchElementException in the event that the element cannot be located right away. It covers all interactions between web elements and is universal. | Clearly Wait waits for a specific condition to be satisfied before executing the test script’s following commands. With Expected Conditions, you can provide unique requirements such as the element’s visibility, clickability, and presence. |
Implicit Wait offers an overall waiting method that may result in wait times that are longer than necessary, particularly if elements are located before the designated timeout. Because it applies to every element in the same way, it lacks specificity. | Developers may wait for specified conditions on specific items with Explicit Wait, giving you more freedom and control. To increase test efficiency and reliability, you may determine distinct wait times for various elements according to their expected behaviour. |
Test execution time may be impacted by implicit wait, particularly if it is set for an extended amount of time. It may result in longer total test execution times since it introduces an implicit wait before each element lookup. | Naturally Wait performs more effectively since it just waits when required within the specified conditions. It maximises test execution speed by minimising needless delays and waiting for certain events or conditions to occur. |
The Selenium design pattern called Page Object Model, or POM, creates an object repository to house all web elements. It improves test case management and lessens code duplication. Think of every web page in an application as a class file in the Page Object Model.
When you make a modification to an action or UI element, POM is helpful. A good example might be: a radio button is substituted for a drop-down menu. POM aids in locating the page or screen that has to be changed in this situation. This identification is required to make changes to the appropriate files because each screen will have a different set of Java files. This lowers errors and facilitates the maintenance of test cases.
Methods for interacting with the web elements on the page are included in Page Object classes. The interactions, like clicking a button, typing text into a field, or getting text out of an element, are captured by these methods. These methods can be applied to different test cases that interact with the same items because they are defined inside Page Object classes.
When each screen has its own separate Java file, it is easy to go through the file and immediately find the activities taken on a given screen. If a certain code part needs to be changed, it can be done quickly and effectively without affecting other files.
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class JUnitExplicitWaitExample {
private WebDriver driver;
@Before
public void setUp() {
// Set the path to the geckodriver executable
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
// Create a new instance of the Firefox driver
driver = new FirefoxDriver();
}
@Test
public void testWithExplicitWait() {
// Navigate to a webpage
driver.get("http://example.com");
// Set an explicit wait of up to 10 seconds
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(
ExpectedConditions.presenceOfElementLocated(By.id("some-element-id"))
);
// Do something with the element
element.click();
}
@After
public void tearDown() {
// Close the driver
driver.quit();
}
}
In conclusion, mastering a Selenium tutorial for beginners with Java can indeed open up new doors in the field of automation testing. As you’ve seen in this tutorial, the robust capabilities of Selenium combined with the flexibility and power of Java can revolutionise your testing process. By continuously expanding your knowledge and skills in this area, you can stay ahead in the rapidly evolving world of software testing. Be sure to apply what you’ve learned and never stop exploring the endless possibilities that Selenium and Java have to offer. Thank you for joining us on this journey to enhance your automation testing prowess.
Consult Us