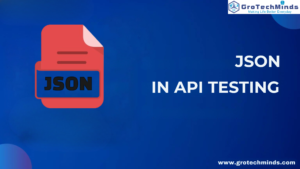
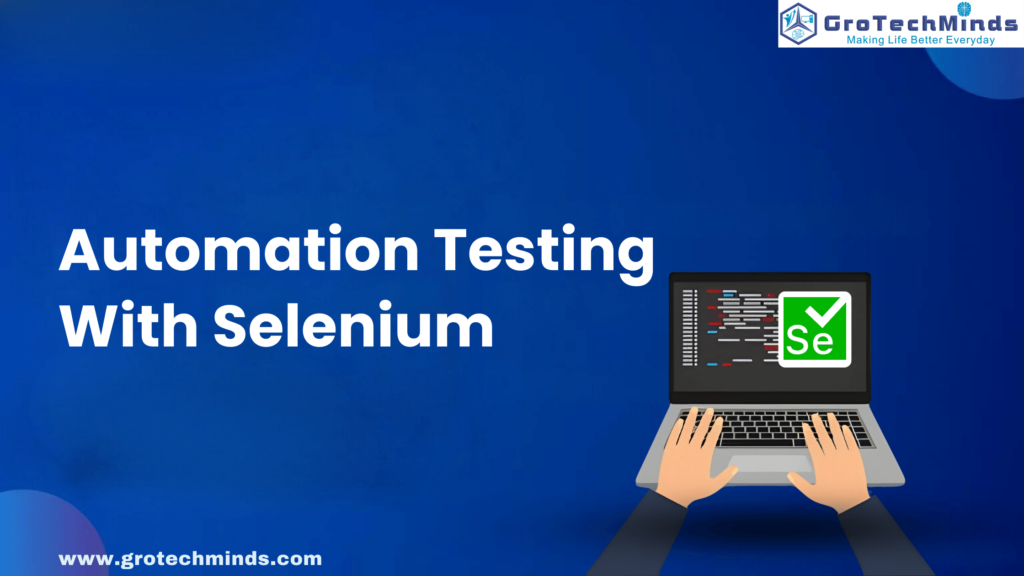
Understanding Selenium:
Selenium is An open-source platform for automated testing web applications . It offers a set of tools for automating different platform web browsers. Selenium is very flexible and can be tailored to meet the needs of various projects since it enables testers to build test scripts in a variety of computer languages, including Java, Python, C#, and others.
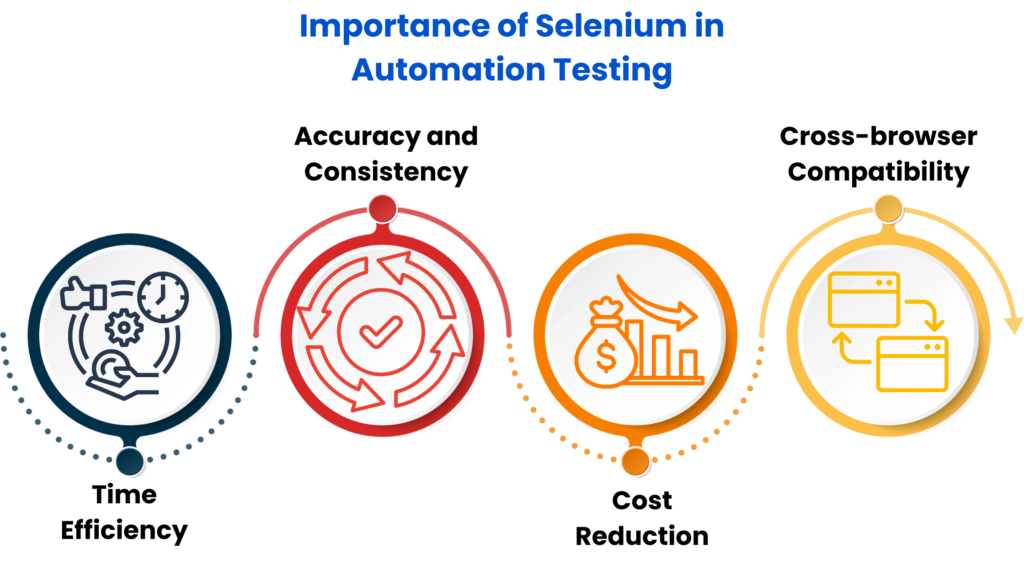
Testers may now spend more of their time exploring and edge-case testing because automated testing significantly cuts down on the amount of time needed to run repetitive test cases.
Automated tests follow instructions to the letter, removing human error and providing consistent test outcomes from one execution to the next.
Organisations may reduce the requirement for manual testing efforts which will lower overall testing expenses by automating repetitive test cases.
Selenium allows the seamless validation of application behaviour across various browser settings by supporting a multitude of web browsers.
The process of writing a basic Selenium test script using Java
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class SimpleSeleniumTest {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("https://www.example.com");
// Perform actions like clicking buttons, filling forms, etc.
driver.quit();
}
}
// Switching to a frame by index
driver.switchTo().frame(0);
// Switching to a frame by name or id
driver.switchTo().frame("frameName");
// Switching to a new window
String mainWindow = driver.getWindowHandle();
// Perform actions to open a new window
for (String handle : driver.getWindowHandles()) {
if (!handle.equals(mainWindow)) {
driver.switchTo().window(handle);
// Perform actions on the new window
}
}
Test scripts can be made easier to update and reusable by being divided into smaller, reusable modules.
To increase reliability, use explicit waits to make sure the automation script waits for specific elements to appear on the page before acting.
To improve structure and readability, use the Page Object Model approach to represent web page elements and their interactions.
Test scripts can be made more flexible and scalable by setting up test data, which makes it simple to change and conduct retesting with alternative inputs.
Create reporting and logging systems to monitor the status of test execution, spot errors, and provide stakeholders with results that are useful.
Automate test execution as an aspect of software delivery by integrating Selenium test automation with CI/CD pipelines. This will provide quick feedback loops and early bug detection.
In conclusion, adopting automation testing with Selenium can revolutionise the way you approach software testing. By harnessing the power of Selenium, you can save time, reduce manual effort, and enhance the quality of your applications. Its versatility, compatibility with multiple browsers, and ability to perform testing at scale make it a valuable tool for any testing team. Embrace automation testing with Selenium to drive your testing process forward and stay ahead in the competitive technological landscape. Start exploring the possibilities today and unlock the full potential of automation testing with Selenium.
Consult Us