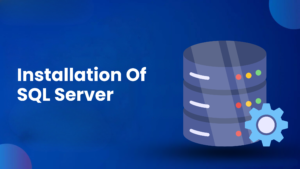

List Interface and its methods
Before we start with List Interface let us look at Collection Hierarchy
Collection Hierarchy
The below diagram shows the Collection hierarchy consisting of classes and interfaces.

As per the scope of the blog our discussion will be limited to the List Interface
What is List Interface ?
List Interface is the child Interface of Collection Interface and grand child of Iterable Interface which is used to store multiple elements. It comes from “java.utils” package. List Interface is an index based data structure. Duplicate elements can be stored in List Interface. List Interface allows any number of null values to be stored in it. List Interface follow the order of insertion. List Interface elements can be iterated by using Iterator and ListIterator methods. Since List is an interface we cannot create its object directly rather we should its child class into List Interface in order to create an object.
Methods of List Interface
Below are some of the methods of List Interface.
Method Name | Description |
add(Object e) | This method is used to add a new element of any datatype into the List Interface. |
addAll(Collection c) | This method is used to add all elements of different datatypes into the List Interface. |
clear() | It is used to clear all the elements from the Set interface but it does not clear the List Interface. |
contains(Object o) | This method is used to check the presence of a Collection element in the List Interface. It returns ‘true’ value if the element is present in List Interface and ‘false’ value if the element is not present in the List Interface. |
containsAll(Collection c) | This method is used to check if the List Interface contains all the collection elements. It returns ‘true’ value if all the elements are present in List Interface and ‘false’ value if any one of the element is not present in the List Interface. |
isEmpty() | This method checks if the given List Interface is empty |
iterator() | This method is used to iterate sequentially all the Collection elements present in a List Interface. |
remove(Object o) | This method is used to remove a specified collection element from the List Interface. |
removeAll(Collection c) | This method removes all those Collection elements from the current List Interface which are present in another List Interface. |
retainAll(Collection c) | This method retains all those elements from the current List Interface which are present in another List Interface and removes those elements which are present in current List Interface but not in another List Interface and vice versa. |
size() | This method is used to find out and display the number of Collection elements present in the List Interface. |
toArray() | This method is used to convert all collection elements present in List Interface into an array. |
toString() | This method is used to convert all collection elements present in List Interface into a String. |
equals() | This method is used to check if two List Interfacees are equal. It returns ‘true’ value if elements as well as size of elements present in one List Interface is equal to the elements and its size present in another List Interface. If the elements and the size of elements present in one Vectro class is not equal to the elements and its size present in another List Interface then it returns ‘false’ value. |
get(int index) | It is used to display an element of List Interface present at a particular index. |
set(int index,Object element) | It is used to replace a List Interface element present at a particular index position with another element. |
listIterator() | This method is used to iterate sequentially in forward as well as backward direction all the Collection elements present in the List Interface. |
indexOf(Object o) | It is used to display the index of first occurrence of List Interface element.It gives-1 if the specified element is not present in List Interface. |
- Let us check the usage of add(Object e) method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
System.out.println(a1);
}
}
Output: [Hi, Hey, 16, 16, 20, 23, 34]
Screenshot:

2. Let us check the usage of addAll(Collection c) method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
List a2= new ArrayList ();
a2.addAll(a1);
System.out.println(a2);
}
}
Output: [Hi, Hey, 16, 16, 20, 23, 34]
Screenshot:
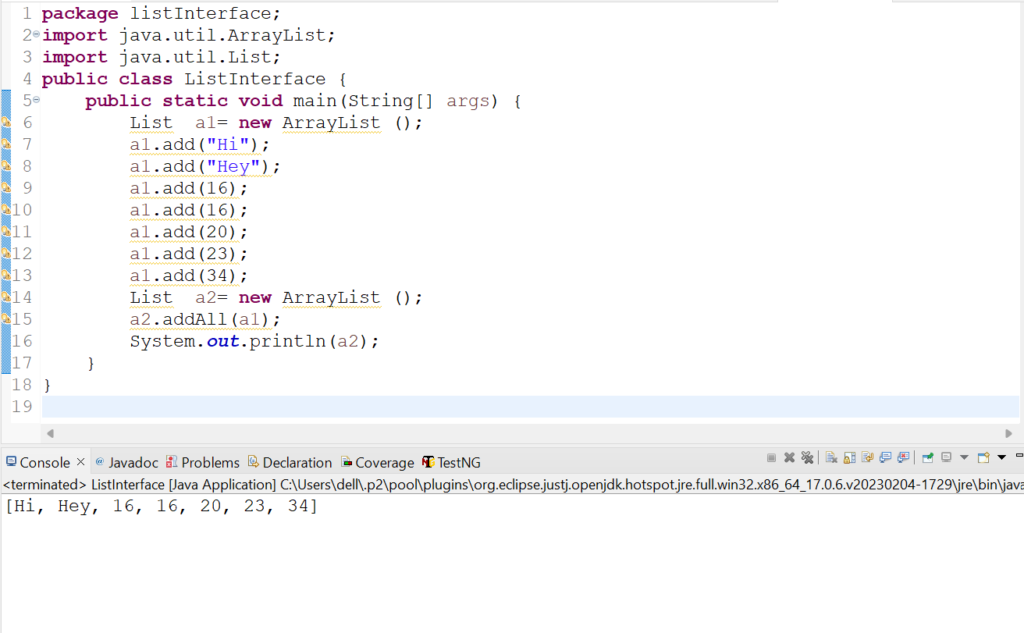
3. Let us check the usage of clear() method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
a1.clear();
System.out.println(a1);
}
}
Output: []
Screenshot:

4. Let us check the usage of contains(Object o) method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
System.out.println("The given List Interface contains: "+a1.contains(16));
System.out.println("The given List Interface contains: "+a1.contains("Harish"));
}
}
Output: The given List Interface contains: true
The given List Interface contains: false
Screenshot:

5. Let us check the usage of containsAll(Collection c) method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
List a2= new ArrayList ();
a2.add("Hi");
a2.add("Hey");
a2.add(16);
a2.add(20);
System.out.println("Does a2 contains all elements of a1:"+a2.containsAll(a1));
System.out.println("Does a1 contains all elements of a2:"+a1.containsAll(a2));
}
}
Output:
Does a2 contains all elements of a1:false
Does a1 contains all elements of a2:true
Screenshot:

6. Let us check the usage of isEmpty() method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
List a2= new ArrayList ();
System.out.println("Is a1 List Interface empty?: "+a1.isEmpty());
System.out.println("Is a2 List Interface empty?: "+a2.isEmpty());
}
}
Output:
Is a1 List Interface empty?: false
Is a2 List Interface empty?: true
Screenshot:

7. Let us check the usage of iterator() method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.Iterator;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new List ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
Iterator a2= a1.iterator();
System.out.println("The collection elements values are: ");
while(a2.hasNext())
{
System.out.println(a2.next());
}
}
}
Output:
The collection elements values are:
Hi
Hey
16
16
20
23
34
Screenshot:

8. Let us check the usage of remove(Object o) method in List Interface.
Case 1: For removing an element containing words:
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
a1.remove("Hey");
a1.remove("Hi");
System.out.println(a1);
}
}
Output: [16, 16, 20, 23, 34]
Screenshot:

Case 2: For removing an element containing numeric value:
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
a1.remove(2);
System.out.println("The elements present in List Interface after removing 16 from 2nd index:"+a1);
a1.remove(4);
System.out.println("The 4th index is chosen from the list of elements present after removing 16");
System.out.println("The elements present in List Interface after removing 23 from 4th index:"+a1);
}
}
Output: The elements present in List Interface after removing 16 from 2nd index:[Hi, Hey, 16, 20, 23, 34]
The 4th index is chosen from the list of elements present after removing 16
The elements present in List Interface after removing 23 from 4th index:[Hi, Hey, 16, 20, 34]
Screenshot:

9. Let us check the usage of removeAll(Collection c) method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
List a2= new ArrayList ();
a2.add("Hi");
a2.add("Hey");
a2.add(16);
a2.add(20);
a1.removeAll(a2);
System.out.println("Elements present in a1 after removing a2 elements from a1 are:"+a1);
}
}
Output:
Elements present in a1 after removing a2 elements from a1 are:[23, 34]
Screenshot:
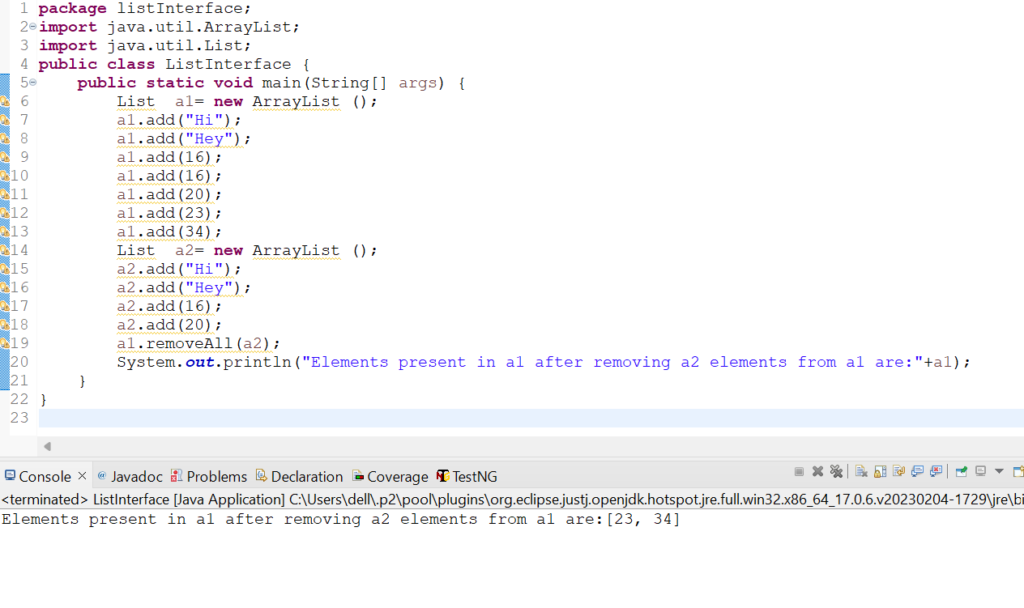
10. Let us check the usage of retainAll(Collection c) method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
List a2= new ArrayList ();
a2.add("Hi");
a2.add("Hey");
a2.add(16);
a2.add(20);
a1.retainAll(a2);
System.out.println("Elements present in a1 after retaining a2 elements are:"+a1);
}
}
Output: Elements present in a1 after retaining a2 elements are:[Hi, Hey, 16, 16, 20]
Screenshot:

11. Let us check the usage of size() method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new List ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
System.out.println("The size of the collection elements present in the List Interface is: "+a1.size());
}
}
Output: The size of the collection elements present in the List Interface is: 7
Screenshot:

12. Let us check the usage of toArray() method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
Object a2[]= a1.toArray();
System.out.println("The list of array elements are:");
for(int i=0;i<=a2.length-1;i++)
{
System.out.println(a2[i]);
}
}
}
Output: The list of array elements are:
Hi
Hey
16
16
20
23
34
Screenshot:
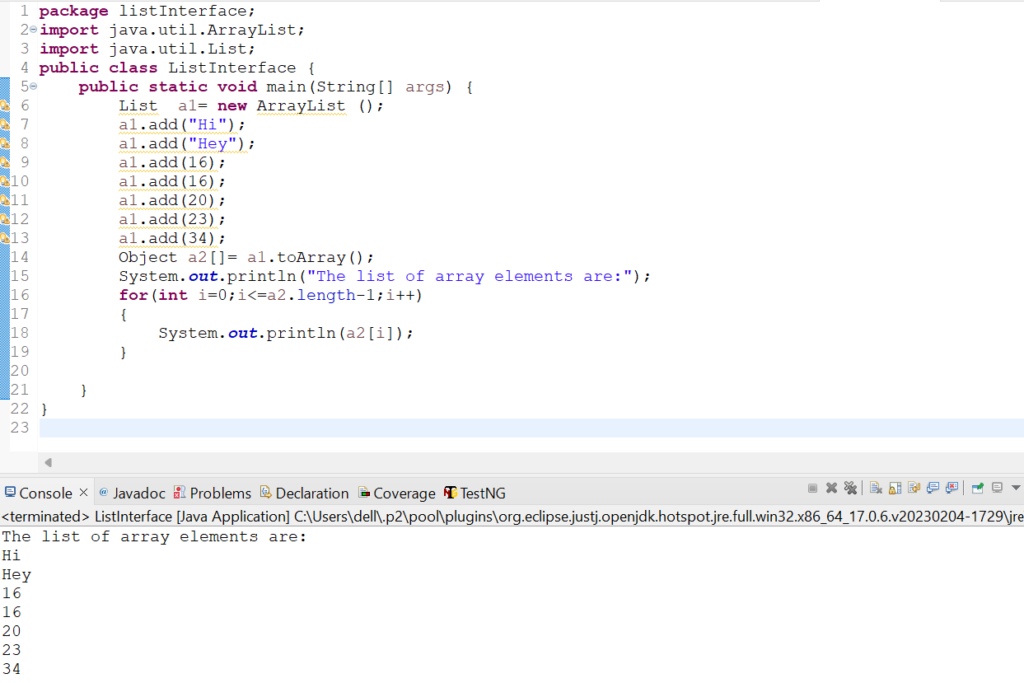
13. Let us check the usage of toString() method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
System.out.println(a1.toString());
}
}
Output: [Hi, Hey, 16, 20, 23, 34]
Screenshot:

14. Let us check the usage of equals() method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
List a2= new ArrayList ();
a2.add("Hi");
a2.add("Hey");
a2.add(16);
a2.add(16);
a2.add(20);
a2.add(23);
a2.add(34);
List a3= new ArrayList ();
a3.add("Hi");
a3.add("Hey");
a3.add(16);
a3.add(20);
System.out.println("Is List Interface a1 equal to a2 ?:"+ a1.equals(a2));
System.out.println("Is List Interface a2 equal to a3 ?:"+ a2.equals(a3));
}
}
Output: Is List Interface a1 equal to a2 ?:true
Is List Interface a2 equal to a3 ?:false
Screenshot:

15. Let us check the usage of get(int index) method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
System.out.println("List of elements in List Interface are: "+a1);
System.out.println("The element present in index position 2 is: "+a1.get(2));
}
}
Output: List of elements in List Interface are: [Hi, Hey, 16, 16, 20, 23, 34]
The element present in index position 2 is: 16
Screenshot:

16. Let us check the usage of set(int index,Object element) method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
System.out.println("List of elements in List Interface are: "+a1);
System.out.println("The element to be replaced is: "+a1.set(2,56));
System.out.println("New List of elements after replacement are :" +a1);
}
}
Output: List of elements in List Interface are: [Hi, Hey, 16, 16, 20, 23, 34]
The element to be replaced is: 16
New List of elements after replacement are :[Hi, Hey, 56, 16, 20, 23, 34]
Screenshot:
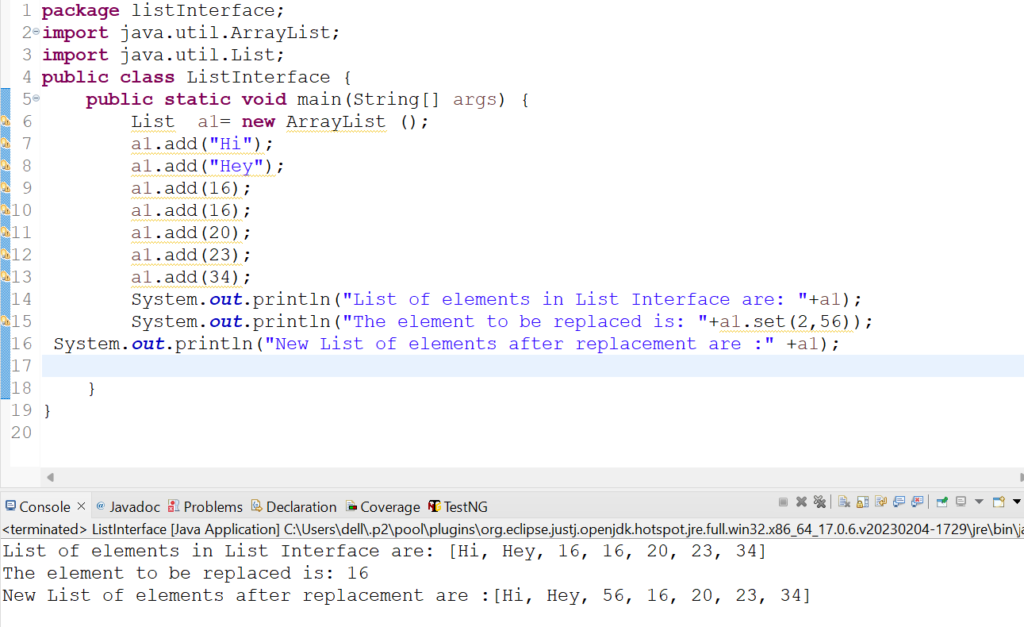
17. Let us check the usage of listIterator() method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
import java.util.ListIterator;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
ListIterator a2= a1.listIterator();
while(a2.hasNext()==true)
{
System.out.println("Next method "+a2.next());
}
while(a2.hasPrevious()==true)
{
System.out.println("Previous Method "+a2.previous());
}
}
}
Output: Next method Hi
Next method Hey
Next method 16
Next method 16
Next method 20
Next method 23
Next method 34
Previous Method 34
Previous Method 23
Previous Method 20
Previous Method 16
Previous Method 16
Previous Method Hey
Previous Method Hi
Screenshot:

18. Let us check the usage of indexOf(Object o) method in List Interface.
Code Snippet:
package listInterface;
import java.util.ArrayList ;
import java.util.List ;
public class ListInterface {
public static void main(String[] args) {
List a1= new ArrayList ();
a1.add("Hi");
a1.add("Hey");
a1.add(16);
a1.add(16);
a1.add(20);
a1.add(23);
a1.add(34);
System.out.println("Index of 16 is :"+a1.indexOf(16));
System.out.println("Index of 45 is :"+a1.indexOf(45));
}
}
Output: Index of 16 is :2
Index of 45 is :-1
Screenshot:

Difference between List and Stack
SL NO: | List | Stack |
1 | It is not a Legacy class | It is a Legacy class |
2 | Belongs to Collection framework | Belongs to original version of Java(1.0) |
3 | Iterator,ListIterator are used. | Enumeration,Iterator and ListIterator are used. |
4 | It is the child interface of Collection and Iterable interface | It is the sub class of Vector class and implemented class of List interface,Collection and Iterable interface. |
Conclusion
List Interface is used for handling all the Collection elements in an effective way. It is necessary to understand the usage of all the methods of List Interface for better handling of Collection elements. Remember to practice, stay updated with the latest trends in Automation Software Testing Course,and maintain a positive attitude throughout your interview process.By thoroughly understanding the List Interface and its methods developers and testers can utitlise those concepts in their real life tasks.
Also read:
Consult Us