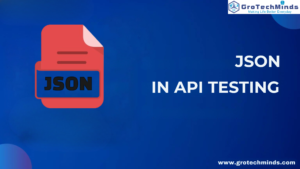

Interview Questions on StringBuffer and StringBuilder
1.What is a StringBuffer?
a)class
b)method
c)constructor
Ans class
2. StringBuffer is mutable.
a)True
b) False
Ans True
3.StringBuffer is not synchronized.
a)True
b) False
And False
4. String buffer creates an empty constructor with an initial capacity.
a)12
b)20
c)16
Ans 16
5. String buffer reverse() method.
a) It is used to return the current capacity.
b) It is used to reverse the string.
Ans It is used to reverse the string.
6. What is the return type of String buffer charAt(int index)?
a) char
b) int
c) StringBuffer
Ans char
7. Non-Synchronized means not thread-safe.
a)True
b)False
Ans True
8. Which method will be used in StringBuffer to add Jacob after Carlson?
String fname=“carlson mathew”;
a)insert
b)delete
c)reverse
Ans insert
9. What does ‘+’ mean in the below String?
String a1=a+” mathew”;
a)concat
b)reverse
Ans concat
10. StringBuffer(String str) is a parameterized constructor.
a)True
b)False
Ans True
11. StringBuffer was introduced with the initial release of Java.
a)1.0
b)1.5
Ans 1.0
12. StringBuffer uses a special memory string pool.
a)True
b) False
Ans False
13. StringBuffer we add, remove, and insert characters.
a) True
b)False
Ans True
14. StringBuffer uses which memory?
a)Heap
b) String pool
Ans Heap
15.StringBuffer name=new StringBuffer(“carlson mathew”);
System.out.println(name.length());
a)15
b)14
Ans 14
16. StringBuilder is mutable.
a)True
b) False
Ans True
17. StringBuilder is not synchronized.
a)True
b) False
Ans True
18. StringBuilder and StringBuffer methods are same?
a)True
b) False
Ans True
19. StringBuilder uses which memory?
a)Heap
b) String pool
Ans Heap
20. StringBuilder was introduced with the initial release of Java.
a)1.0
b)1.5
Ans 1.5
21. StringBuilder is not thread-safe hence faster than String Buffer.
a)True
b) False
Ans True
22. Which class reverse() method belongs to?
a) StringBuffer
b)String Builder
c) String
d) Both a) and b)
Ans Both a) and b)
23.The method of StringBuffer and StringBuilder class which return the current capacity is called as?
a)ensureCapacity(int minimumCapacity)
b)capacity()
Ans capacity()
24. What is StringBuilder() called?
a)constructor
b)class
c)interface
Ans constructor
25. What is the return type of ensureCapacity() method of StringBuilder class?
a)void
b)int
c)String
Ans void
26. Which of the following is not synchronized?
a)StringBuffer
b)StringBuilder
Ans StringBuilder
27. What is the default capacity of the StringBuilder?
a)10
b)16
c)12
Ans 16
28. What will be the output of the following Java program?
class Hello
{ public static void main(String args[])
{ StringBuilder c = new StringBuilder("Hello"); c.delete(0,2);
System.out.println(c); } }
a)he
b)hel
c)lo
d)llo
Ans llo
29. What is the output of the following code snippet?
public class Alex
{ public static void main(String[] args)
{ String str = "Java"; str.concat(" Programming");
System.out.println(str); } }
a)Java
b)Java Programming
Ans Java
30. Which method is used to delete the string from the specified startIndex and endIndex?
a)delete(int startIndex,int endIndex)
b)substring(intbeginIndex, intendIndex)
Ans delete(int startIndex,int endIndex)
Read blog on StringBuffer and methods
Consult Us