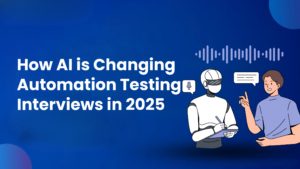

How to check if text box is enabled or not in Selenium
Introduction
Selenium is a tool which is open source,easy to use and freely available for performing automation testing of web applications and websites. Testers use Selenium tool for browser automation and automation of web applications to ensure seamless and rapid automation testing resulting in quality applications getting delivered to customers in short period of time. Automation testing using Selenium saves lot of time and effort compared to manual testing. Selenium has some predefined methods like isEnabled(), isDisplayed() which makes the automation testing process easier and effective.
isEnabled is a method which is used to check if a given element or checkbox is enabled or not. It is a method of WebElement interface and its return type is boolean. If a given element is enabled the boolean value will be printed true in output console and when we try to perform action on that element, that action gets successfully performed. But if the element is not enabled the boolean value will be printed as false in output console and when we try to perform actions on that element we will get “ElementNotInteractableException”.isEnabled is important method to know the status of the element present in the webpage before Selenium interacts with that element.
- Create a new class in the existing package which contains selenium jar.
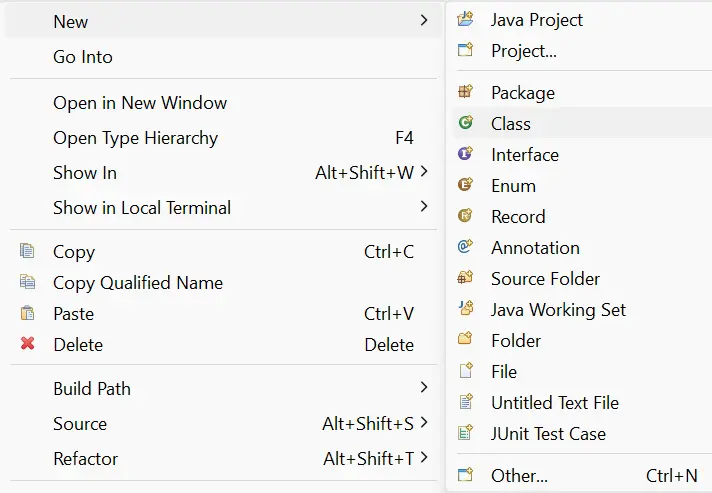
2. Launch empty browser using ChromeDriver class
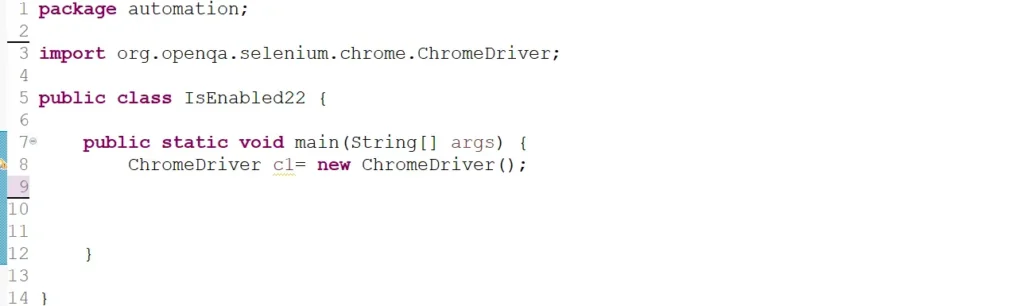
3. Navigate to the webpage using get method and reference variable of ChromeDriver class.
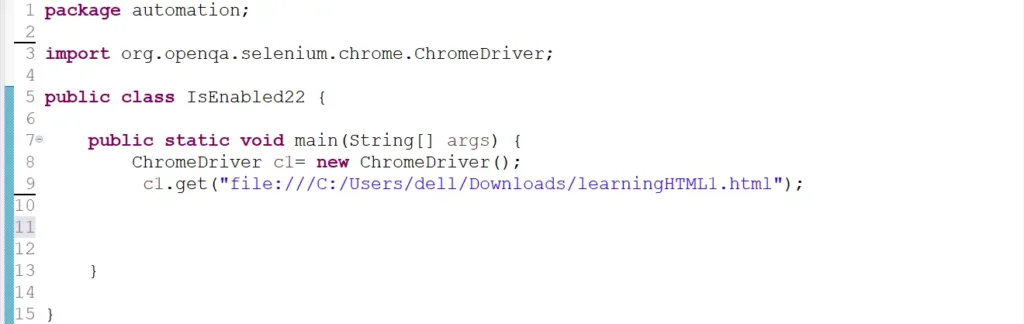
4. Locate the element using findelement method and id locator and put the entire line of code in a return type which is WebElement and WebElement is also an interface.
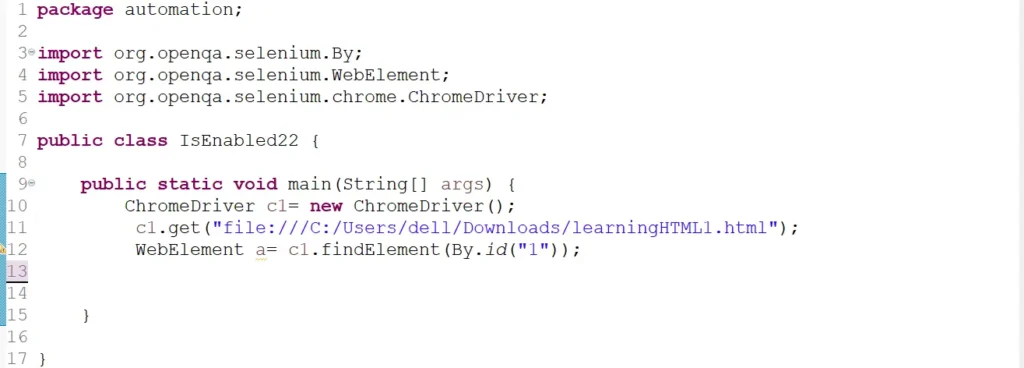
5. Using isEnabled() method and reference variable of WebElement interface check whether the username text field is enabled or disabled and put the entire line of code in boolean return type and then finally print the reference variable of boolean where its value will be printed in output console. If the element is enabled then true will be printed in output console but if the element is not enabled then false value will be printed in output console.
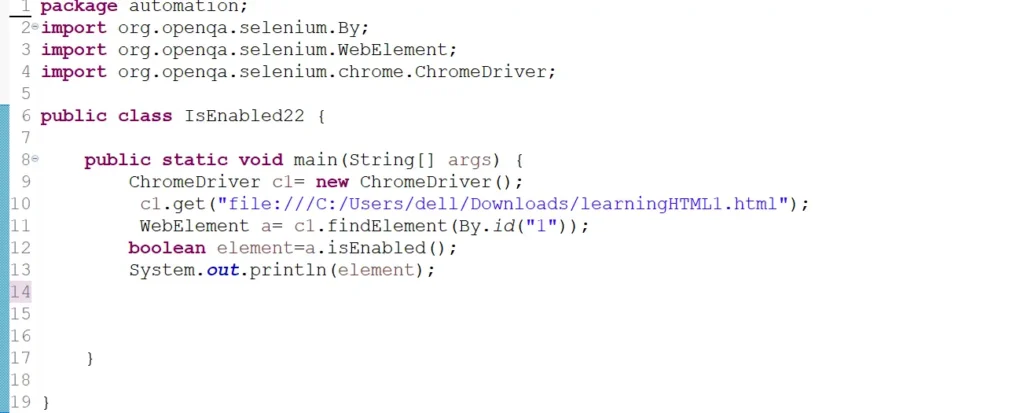
6. The below screenshot shows true value is printed in output console which indicates the textbox is enabled.

The below screenshot shows false value is printed in output console which indicates the textbox is not enabled.
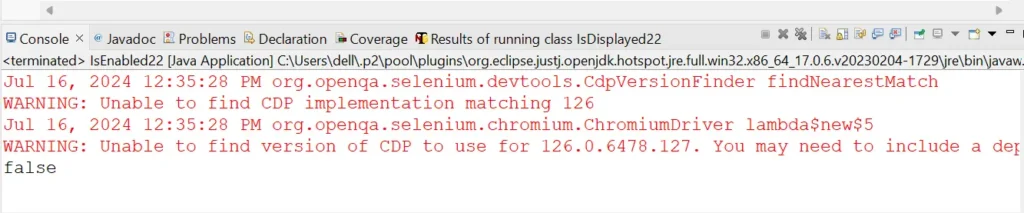
7. After checking whether the element is enabled or not we perform action on that element using sendkeys method and reference variable of WebElement interface.
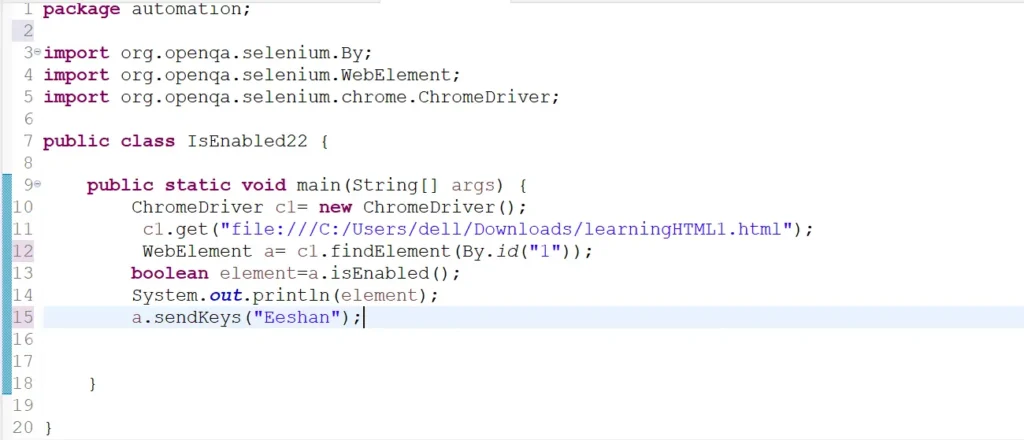
The below screenshot shows the output of the action performed on the ‘username’ text field which is enabled where ‘Eeshan’ is printed inside the text field.
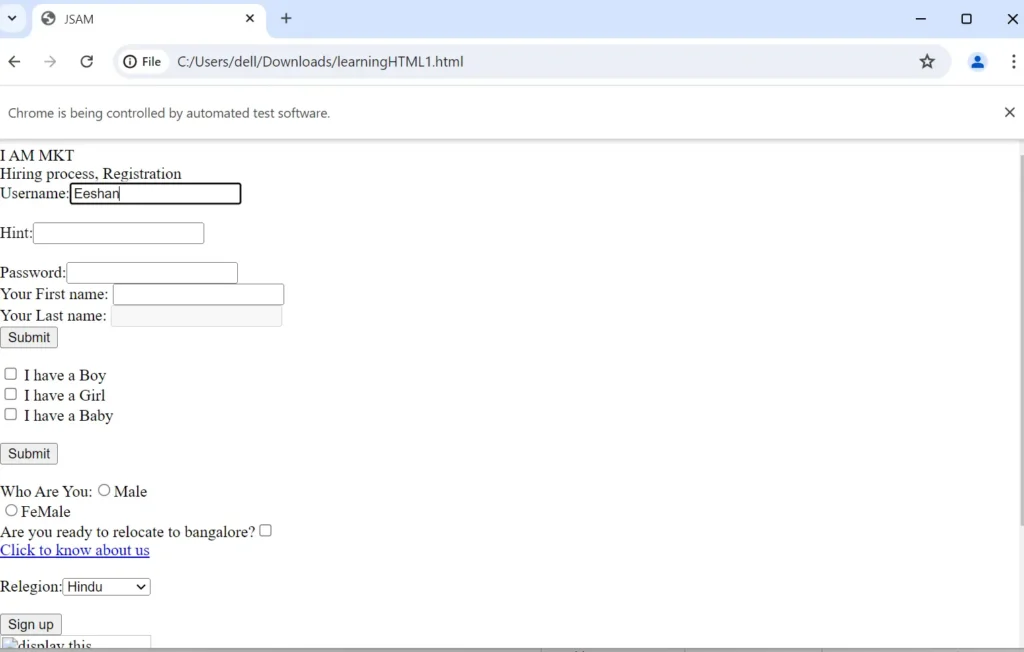
When an element is disabled and we try to perform actions like clicking on that element or writing something on that element we get “ElementNotInteractableException”. The below screenshot show the code snippet of locating the disabled element, using isEnabled method on that element and performing actions on that element using sendkeys method.
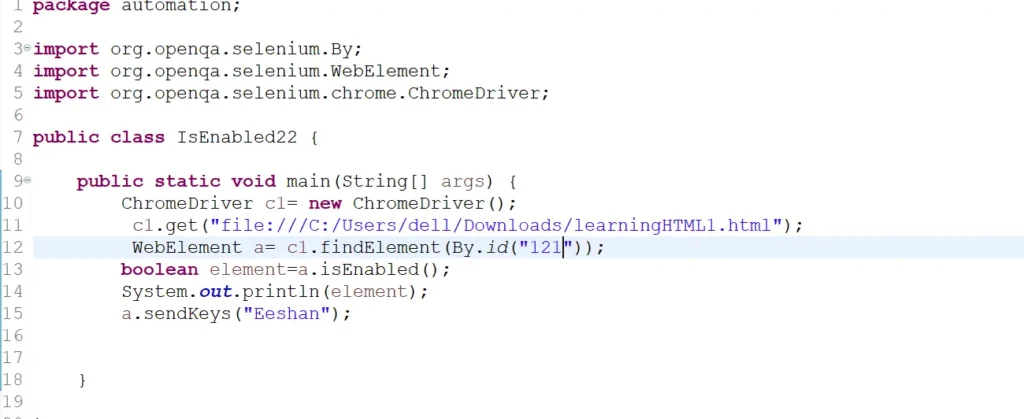
The below is the screenshot of output console showing “ElementNotInteractableException”
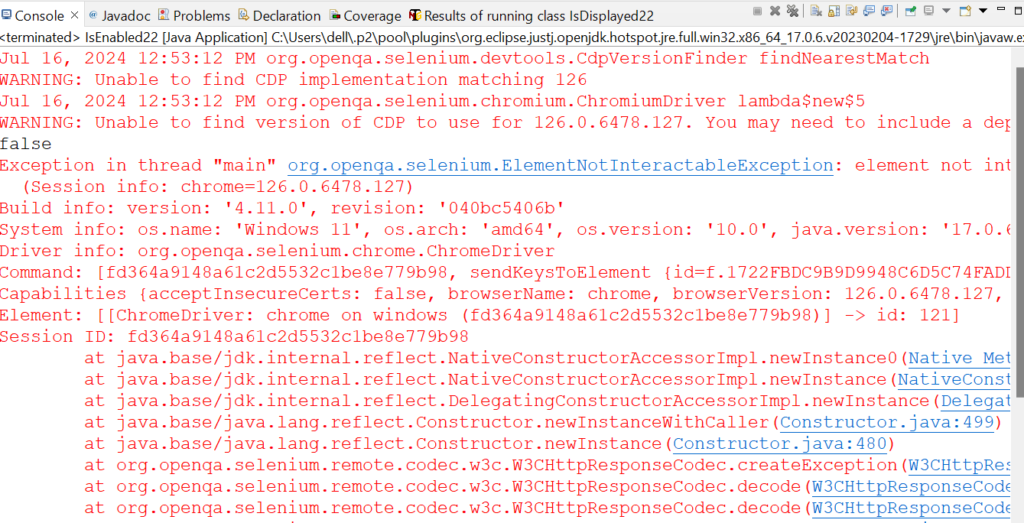
The below is the screenshot of output console showing “ElementNotInteractableException”.
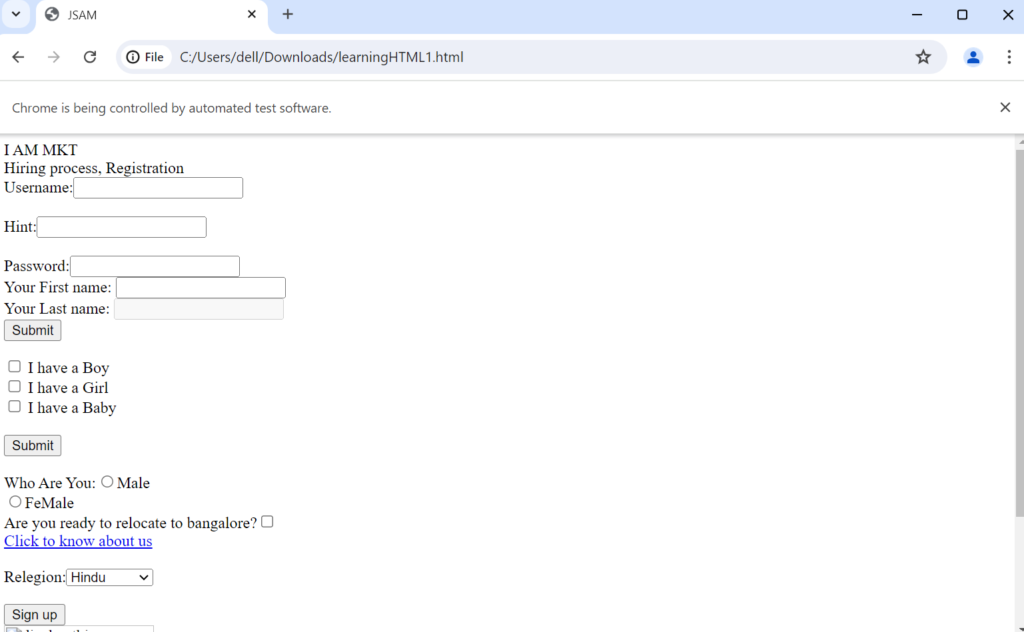
The code snippet for checking the textbox is enabled as well as performing actions on enabled elements is mentioned below
package automation;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class IsEnabled22 {
public static void main(String[] args) {
ChromeDriver c1= new ChromeDriver();
c1.get("file:///C:/Users/dell/Downloads/learningHTML1.html");
WebElement a= c1.findElement(By.id("1"));
boolean element=a.isEnabled();
System.out.println(element);
a.sendKeys("Eeshan");
}
}
The code snippet for checking the textbox is enabled as well as performing actions on disabled elements is mentioned below
package automation;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class IsEnabled22 {
public static void main(String[] args) {
ChromeDriver c1= new ChromeDriver();
c1.get("file:///C:/Users/dell/Downloads/learningHTML1.html");
WebElement a= c1.findElement(By.id("121"));
boolean element=a.isEnabled();
System.out.println(element);
a.sendKeys("Eeshan");
}
}
IsEnabled has proven to be one of the most effective predefined method of Selenium since detects the presence of component in a webpage before proceeding for Automation Testing. Remember to practise, stay updated with the latest trends in Automation Software Testing Course,and maintain a positive attitude throughout your interview process. This ensures proper execution of test scripts without exceptions at run time resulting in effective automation testing of web applications and websites.
Also read:
Consult Us