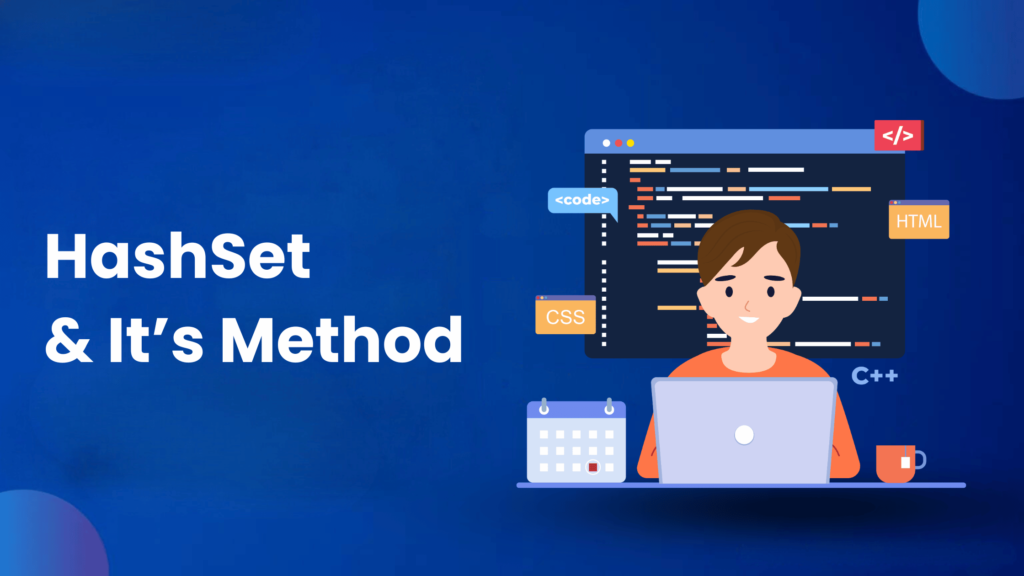
HashSet and its Methods
Before we start with HashSet let us look at the Collection Hierarchy
Collection Hierarchy
The below diagram shows the Collection hierarchy consisting of classes and interfaces.
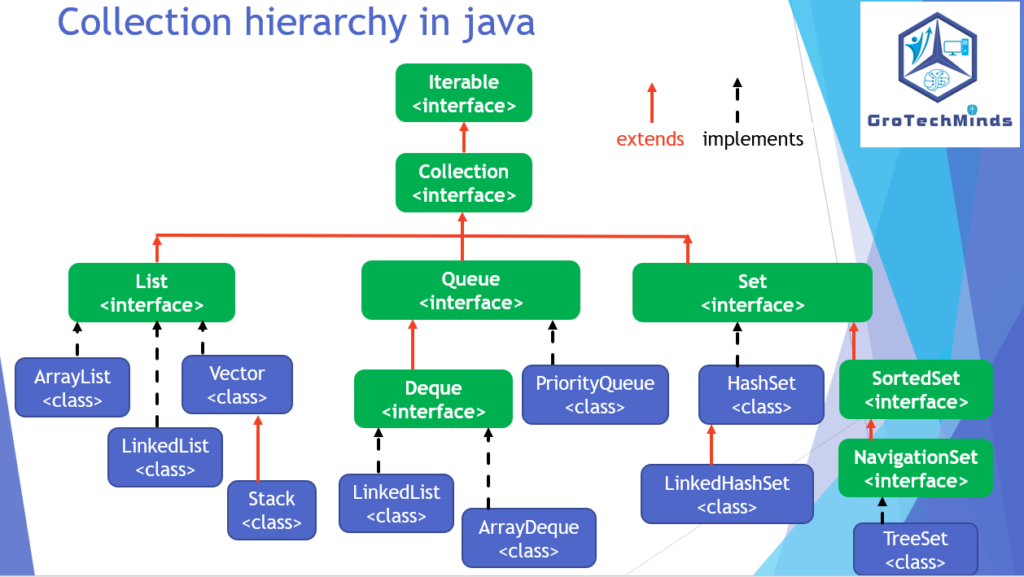
As per the scope of the blog our discussion will be limited to the HashSet class
What is HashSet
HashSet is a class which is used to store multiple elements. It is the implemented class of Set interface,Collection interface and Iterable interface. HashSet class comes from “java.utils” package.HashSet is not an index based data structure and all the datas are stored in accordance with the hashcode values. Duplicate elements cannot be stored in HashSet. HashSet allows only one null value to be stored in it. HashSet does not follow the order of insertion. HashSet elements can be iterated by only Iterator method. “HashTable” is the data structure of HashSet which means all the Collection elements of HashSet are stored using HashTable. The mechanism by which all the elements are stored in HashSet is called as Hashing. HashSet is nether thread safe nor synchronized. Its initial capacity is 16 and its capacity is increased after load factor becomes 0.75.
Methods of HashSet class
Below are some of the methods of HashSet class:
Method Name | Description |
add(Object e) | This method is used to add a new element of any datatype into the HashSet class. |
addAll(Collection c) | This method is used to add all elements of different datatypes into the HashSet class. |
clear() | It is used to clear all the elements from the Set interface but it does not clear the HashSet class. |
contains(Object o) | This method is used to check the presence of a Collection element in the HashSet. It returns ‘true’ value if the element is present in HashSet and ‘false’ value if the element is not present in the HashSet. |
containsAll(Collection c) | This method is used to check if the HashSet contains all the collection elements. It returns ‘true’ value if all the elements are present in HashSet and ‘false’ value if any one of the element is not present in the HashSet. |
isEmpty() | This method checks if the given HashSet is empty |
iterator() | This method is used to iterate sequentially all the Collection elements present in a HashSet. |
remove(Object o) | This method is used to remove a specified collection element from the HashSet. |
removeAll(Collection c) | This method removes all those Collection elements from the current HashSet which are present in another HashSet. |
retainAll(Collection c) | This method retains all those elements from the current HashSet which are present in another HashSet and removes those elements which are present in current HashSet but not in another HashSet and vice versa. |
size() | This method is used to find out and display the number of Collection elements present in the HashSet |
toArray() | This method is used to convert all collection elements present in a HashSet into an array. |
toString() | This method is used to convert all collection elements present in a HashSet into a String. |
equals() | This method is used to check if two HashSets are equal. It returns ‘true’ value if elements as well as size of elements present in one HashSet is equal to the elements and its size present in another HashSet. If the elements and the size of elements present in one HashSet is not equal to the elements and its size present in another HashSet then it returns ‘false’ value. |
clone() | This method is used to create a shallow copy of the elements present in the HashSet class. |
1.Let us check the usage of add(Object e) method in HashSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
System.out.println(a1);
}
}
Output: [null, c, 3.4, Hiii, 3.55, hello, true]
Screenshot:
2. Let us check the usage of addAll(Collection c) method in HashSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
HashSet a2= new HashSet();
a2.addAll(a1);
System.out.println(a2);
}
}
Output: [null, c, 3.4, Hiii, 3.55, hello, true]
Screenshot:
3. Let us check the usage of clear() method in HashSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
a1.clear();
System.out.println(a1);
}
}
Output: []
Screenshot:
4. Let us check the usage of contains(Object o) method in HashSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
System.out.println("The given HashSet contains: "+a1.contains("hello"));
System.out.println("The given HashSet contains: "+a1.contains("Harish"));
}
}
Output: The given HashSet contains: true
The given HashSet contains: false
Screenshot:
5.Let us check the usage of containsAll(Collection c) method in HashSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
HashSet a2= new HashSet();
a2.add("hello");
a2.add('c');
a2.add(3.4f);
a2.add(true);
System.out.println("Does a2 contains all elements of a1:"+a2.containsAll(a1));
System.out.println("Does a1 contains all elements of a2:"+a1.containsAll(a2));
}
}
Output:
Does a2 contains all elements of a1:false
Does a1 contains all elements of a2:true
Screenshot:
6.Let us check the usage of isEmpty() method in HashSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
System.out.println("Is a1 HashSet empty?: "+a1.isEmpty());
HashSet a2= new HashSet();
System.out.println("Is a2 HashSet empty?: "+a2.isEmpty());
}
}
Output:
Is a1 HashSet empty?: false
Is a2 HashSet empty?: true
Screenshot:
7.Let us check the usage of iterator() method in HashSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
import java.util.Iterator;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
Iterator a2= a1.iterator();
System.out.println("The collection elements values are: ");
while(a2.hasNext())
{
System.out.println(a2.next());
}
}
}
Output:
The collection elements values are:
null
c
3.4
Hiii
3.55
hello
true
Screenshot:
8. Let us check the usage of remove(Object o) method in HashSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
a1.remove("hello");
a1.remove(null);
System.out.println(a1);
}
}
Output: [c, 3.4, Hiii, 3.55, true]
Screenshot:
9. Let us check the usage of removeAll(Collection c) method in HashSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
HashSet a2= new HashSet();
a2.add(true);
a2.add('c');
a2.add("hello");
a2.add("hi");
a1.removeAll(a2);
System.out.println("Elements present in a1 after removing a2 elements from a1 are:"+a1);
}
}
Output:
Elements present in a1 after removing a2 elements from a1 are:[null, 3.4, Hiii, 3.55
Screenshot:
10. Let us check the usage of retainAll(Collection c) method in HashSet class.
Code Snippet:
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
HashSet a2= new HashSet();
a2.add(true);
a2.add('c');
a2.add("hello");
a2.add("hi");
a1.retainAll(a2);
System.out.println("Elements present in a1 after retaining a2 elements are:"+a1);
}
}
Output: Elements present in a1 after retaining a2 elements are:[c, hello, true]
Screenshot:
11. Let us check the usage of size() method in HashSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
System.out.println("The size of the collection elements present in the HashSet is: "+a1.size());
}
}
Output: The size of the collection elements present in the HashSet is: 7
Screenshot:
12. Let us check the usage of toArray() method in HashSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
Object a2[]= a1.toArray();
System.out.println("The list of array elements are:");
for(int i=0;i<=a2.length-1;i++)
{
System.out.println(a2[i]);
}
}
}
Output: The list of array elements are:
null
c
3.4
Hiii
3.55
hello
true
Screenshot:
13. Let us check the usage of toString() method in HashSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
System.out.println(a1.toString());
}
}
Output: [null, c, 3.4, Hiii, 3.55, hello, true]
Screenshot:
14. Let us check the usage of equals() method in HashSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
HashSet a2= new HashSet();
a2.add("hello");
a2.add('c');
a2.add(3.4f);
a2.add(true);
a2.add(null);
a2.add(3.55);
a2.add("Hiii");
HashSet a3= new HashSet();
a3.add("hello");
a3.add('c');
a3.add(3.4f);
a3.add(true);
System.out.println("Is HashSet a1 equal to a2 ?:"+ a1.equals(a2));
System.out.println("Is HashSet a2 equal to a3 ?:"+ a2.equals(a3));
}
}
Output: Is HashSet a1 equal to a2 ?:true
Is HashSet a2 equal to a3 ?:false
Screenshot:
15. Let us check the usage of clone() method in HashSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
System.out.println("List of elements in HashSet class: "+a1);
HashSet clonea1= new HashSet();
clonea1=(HashSet)a1.clone();
System.out.println("List of elements after using clone method:"+ clonea1);
}
}
Output: List of elements in HashSet class: [null, c, 3.4, Hiii, 3.55, hello, true]
List of elements after using clone method:[null, c, 3.4, Hiii, 3.55, hello, true]
Screenshot:
Conclusion
HashSet class is used for handling all the Collection elements in an effective way. It is necessary to understand the usage of all the methods of HashSet for better handling of Collection elements. By thoroughly understanding the HashSet class and its methods developers and testers can utitlise those concepts in their real life tasks.
Also Read:
Consult Us