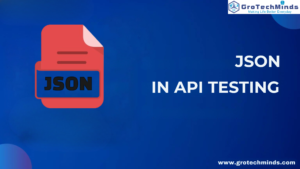

package arrayproblems;
public class IndexOfArrayElement {
public static void main(String[] args) {
int no[]=new int[5]; // we have taken an integer array and provided the fixed size of 5 to the array
no[0]=21; // here different numeric values are given for each index position of array
no[1]=23;
no[2]=98;
no[3]=98;
no[4]=765;
int notobechecked=23; // for checking the presence of ‘23’ in the different positions of array
for(int i=0;i<=no.length-1;i++) // forloop is used to check presence of ‘23’ in different positions of array
{
if(notobechecked==no[i])
{ // If ‘23’ is present in a particular index of array it will print below statement
System.out.println("This is the index of 23="+i);
}
else
{ // If ‘23’ is not present in a particular index of array it will print below statement
System.out.println("Sorry we could not find this element in array");
}
}
}} The below is the output of the above code snippet
Output: Sorry we could not find this element in array
This is the index of 23=1
Sorry we could not find this element in array
Sorry we could not find this element in array
Sorry we could not find this element in array
package arrayproblems;
public class AverageOfArrayElements {
public static void main(String[] args) {
int no[]= {10,5,10,15}; // we have taken an array of numbers and stored in int datatype
int sum=0; // before printing average finding of sum is necessary so by default sum is assumed as ‘0’ and stored in int datatype
for(int i=0;i<=no.length-1;i++) // for loop is used to print the sum of all given numbers in an array.
{
sum=sum+no[i]; // here the sum is the addition of given default sum and array indexes
}
System.out.println(sum); // it will print the sum of numbers in the array.
double average=sum/no.length; // for finding out the average of the array and since average will be in decimal so double datatype is taken
double reminder=sum%no.length; // for finding the remainder after dividing sum of array with length of array
System.out.println(average);// for printing average
System.out.println(reminder);// for printing reminder
}
}
The below is the output of the above code snippet
Output: 40
10.0
0.0
package arrayproblems;
import java.util.Arrays;
import java.util.Collections;
public class SortArrayInAscendingAndDescendingOrder {
public static void main(String[] args) {
String name[]= {"Ram","Laxman","Sita",}; // An array with string datatype is taken
Arrays.sort(name); // Before arranging the array it is necessary to sort the array
System.out.println(Arrays.toString(name)); // after sorting it is necessary to convert array to string using toString method
Arrays.sort(name, Collections.reverseOrder()); // reverse order method is used to sort array in descending order
System.out.println(Arrays.toString(name)); // after sorting it is converted to string using toString method and printed
}
} The below is the output of the code snippet
Output: [Laxman, Ram, Sita]
[Sita, Ram, Laxman]
package arrayproblems;
public class NumberPresent {
public static void main(String[] args) {
int rollno[]=new int[3]; we have taken an integer array and provided the fixed size of 3 to the array
rollno[0]=10; // here different numeric values are given for each index position of array
rollno[1]=20;
rollno[2]=30;
int numbertocheck=30; // for checking the presence of ‘30’ in the different positions of array
for(int i=0;i<=2;i++) // forloop is used to check presence of ‘30’ in different positions of array
{
if(numbertocheck==rollno[i])
{ // If ‘30’ is present in a particular index of array it will print below statement
System.out.println("number found"+rollno[i]);
}
else
{ // If ‘30’ is not present in a particular index of array it will print below statement
System.out.println("No is not available"+rollno[i]);
}
}
}} The below is the output of the code snippet
Output: No is not available10
No is not available20
number found30
package arrayproblems;
import java.util.Arrays;
public class CopyArrayByIterating {
public static void main(String[] args) {
int no[]= {543,76,98}; // An array of 3 numbers is taken and stored in int datatype and stored in ‘no’ variable
int newarray[]=new int[3]; // Array object of size 3 and int datatype is taken and stored in ‘newarray’ variable
for(int i=0;i<=no.length-1;i++) // using forloop we are iterating the array
{
newarray[i]=no[i]; // after iteration arrays are copied
}
System.out.println(Arrays.toString(newarray) );// After copying the arrays it is being converted to string using toString Method and printed
}
} The below is the output of the code snippet
Output:[543, 76, 98]
package arrayproblems;
import java.util.Arrays;
import java.util.Scanner;
public class ArrayInputScanner {
public static void main(String[] args) {
int no[]=new int[3]; // Array object of size 3 and int datatype is taken and stored in ‘no’ variable
Scanner s=new Scanner(System.in); //Creation of Scanner class to give array input at runtime
for(int i=0;i<=2;i++) // for loop is taken to take human input at runtime thrice
{
System.out.println("Enter the value");//to print the statement of entering value
no[i]= s.nextInt(); // an array value is given as input at runtime
}
System.out.println(Arrays.toString(no)); // that array value is converted to string and printed in console after entering values at runtime
}
} The below is the output of the code snippet
Output: Enter the value
4
Enter the value
7
Enter the value
8
[4, 7, 8]
package arrayproblems;
public class MinAndMaxValueInArray {
public static void main(String[] args) {
int no[]= {85,741,74,35,11,25}; // here numbers are taken in an array and stored in int datatype
int max=no[0];
int min=no[0];
// to find maximum value and minimum values in array teh default maximum and minimum value is taken as zero
for(int i=0;i<=no.length-1;i++) // for loop is taken to find maximum and minimum values in array
{
if(no[i]>max) // If the given number in each array index is greater than the default maximum value then below condition will occur
{
max=no[i];
}
if(no[i]
package arrayproblems;
public class ArrayReverse {
public static void main(String[] args) {
int no[]=new int[5]; // Array object of size 5 and int datatype is taken and stored in ‘no’ variable
// the values for each array index is printed individually
no[0]=21;
no[1]=23;
no[2]=98;
no[3]=98;
no[4]=765;
for(int i=no.length-1;i>=0;i--) // for loop is taken in reverse order to print array in reverse order
{ // Since System.out.print does not move cursor in next line so print array in reverse order System.out.print is used and square bracket and spaces are given to print each reversed array numbers in square bracket and with spaces in between them
System.out.print("["+no[i]+"]");
System.out.print(" ");
}
}
} The below is the output of the code snippet
Output: [765] [98] [98] [23] [21]
package arrayproblems;
import java.util.Arrays;
public class ArrayEquals {
public static void main(String[] args) {
// Two equal arrays are taken and stored in String datatype and ‘name’ and ‘name1’ variables respectively.
String name[] = { "Ram", "Laxman", "Sita", };
String name1[] = { "Ram", "Laxman", "Sita", };
System.out.println(name[0] + name[1] + name[2]);// here array elements of each index position of ‘name’ array is printed
boolean asnwer = Arrays.equals(name, name1); // to check two arrays are equal to each other we use an array method called as ‘equals’ method and store in boolean datatype
System.out.println(asnwer); // for printing the value of whether 2 arrays are equal or not which will be true if equal or false if not equal. In this program since two arrays are equal it will give true value.
}
} The below is the output of the code snippet
Output: RamLaxmanSita
True
In conclusion, mastering array problems in Java is key for automation engineers to streamline their workflow and create efficient scripts. By understanding the concepts and applying the solutions we’ve shared in this blog, you can enhance your Java skills and approach array challenges with confidence. We hope this blog has provided you with valuable insights and practical tips to excel in your automation projects. Keep exploring, practising, and embracing the world of Java arrays – you’re well on your way to becoming a skilled automation engineer!
Pursuing a career in Automation Software Testing Course can be a rewarding and fulfilling choice for anyone interested in the field of software testing. The Automation Software Testing Course offers valuable skills and knowledge that can help you excel in your career and stay competitive in the ever-evolving tech industry.
Consult Us