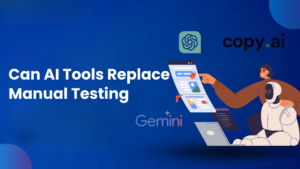

SortedSet Interface and its methods
Before discussing about SortedSet interface let us look at Collection Hierarchy
Collection Hierarchy
The below diagram shows the Collection hierarchy consisting of classes and interfaces.
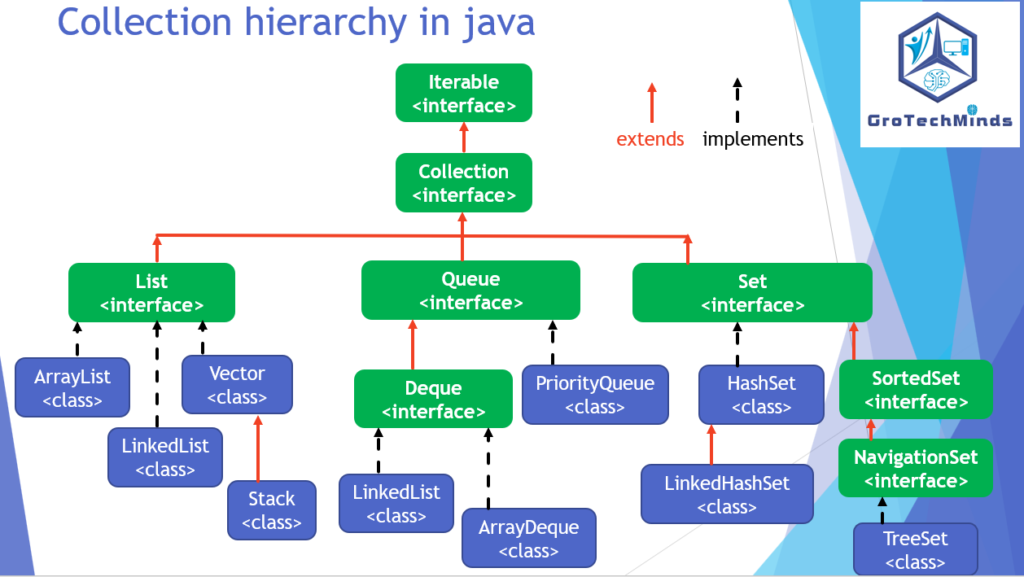
As per the scope of the blog our discussion will be limited to the SortedSet interface
What is SortedSet ?
SortedSet is an interface which is the child interface of Collection interface and grandchild of Iterable interface. SortedSet is not an index based data structure and all the datas are stored in accordance with the hashcode values. Duplicate elements cannot be stored in SortedSet. SortedSet allows only one null value to be stored in it. SortedSet does not follow the order of insertion. SortedSet elements can be iterated by only Iterator method. The various implemented classes of SortedSet interface are TreeSet,LinkedTreeSet and TreeSortedSet where TreeSet and LinkedTreeSet are child classes of SortedSet interface and TreeSortedSet is the great grand child class of SortedSet interface. The various child interfaces associated with SortedSet are SortedSet and Navigation SortedSet where SortedSet is the child interface of SortedSet, Navigation SortedSet is the grandchild interface of SortedSet. Since SortedSet is an interface we cannot create Object of SortedSet directly but instead we upcast the implemented classes of SortedSet like TreeSet, LinkedTreeSet etc. with SortedSet in order to create an instance of SortedSet.
Methods of SortedSet interface
Below are some of the methods of SortedSet interface:
Method Name | Description |
add(Object e) | This method is used to add a new element of any datatype into the SortedSet interface. |
addAll(Collection c) | This method is used to add all elements of different datatypes into the SortedSet interface. |
clear() | It is used to clear all the elements from the SortedSet interface but it does not clear the SortedSet interface. |
contains(Object o) | This method is used to check the presence of a Collection element in the SortedSet. It returns ‘true’ value if the element is present in SortedSet and ‘false’ value if the element is not present in the SortedSet. |
containsAll(Collection c) | This method is used to check if the SortedSet contains all the collection elements. It returns ‘true’ value if all the elements are present in SortedSet and ‘false’ value if any one of the element is not present in the SortedSet. |
isEmpty() | This method checks if the given SortedSet is empty |
iterator() | This method is used to iterate sequentially all the Collection elements present in a SortedSet. |
remove(Object o) | This method is used to remove a specified collection element from the SortedSet. |
removeAll(Collection c) | This method removes all those Collection elements from the current SortedSet which are present in another SortedSet. |
retainAll(Collection c) | This method retains all those elements from the current SortedSet which are present in another SortedSet and removes those elements which are present in current SortedSet but not in another SortedSet and vice versa. |
size() | This method is used to find out and display the number of Collection elements present in the SortedSet |
toArray() | This method is used to convert all collection elements present in a SortedSet into an array. |
toString() | This method is used to convert all collection elements present in a SortedSet into a String. |
equals() | This method is used to check if two SortedSets are equal. It returns ‘true’ value if elements as well as size of elements present in one SortedSet is equal to the elements and its size present in another SortedSet. If the elements and the size of elements present in one SortedSet is not equal to the elements and its size present in another SortedSet then it returns ‘false’ value. |
1. Let us check the usage of add(Object e) method in SortedSet interface.
Code Snippet:
package sortedSetInterface;
import java.util.TreeSet;
import java.util.List;
import java.util.SortedSet;
public class SortedSetInterfaceProgram {
public static void main(String[] args) {
SortedSet a1= new TreeSet();
a1.add(12);
a1.add(14);
a1.add(15);
a1.add(16);
a1.add(18);
a1.add(19);
a1.add(20);
System.out.println(a1);
}
}
Output: [12, 14, 15, 16, 18, 19, 20]
Screenshot:

2. Let us check the usage of addAll(Collection c) method in SortedSet interface.
Code Snippet :
package sortedSetInterface;
import java.util.TreeSet;
import java.util.SortedSet;
public class SortedSetInterfaceProgram {
public static void main(String[] args) {
SortedSet a1= new TreeSet();
a1.add(12);
a1.add(14);
a1.add(15);
a1.add(16);
a1.add(18);
a1.add(19);
a1.add(20);
SortedSet a2= new TreeSet();
a2.addAll(a1);
System.out.println(a2);
}
}
Output: [12, 14, 15, 16, 18, 19, 20]
Screenshot:
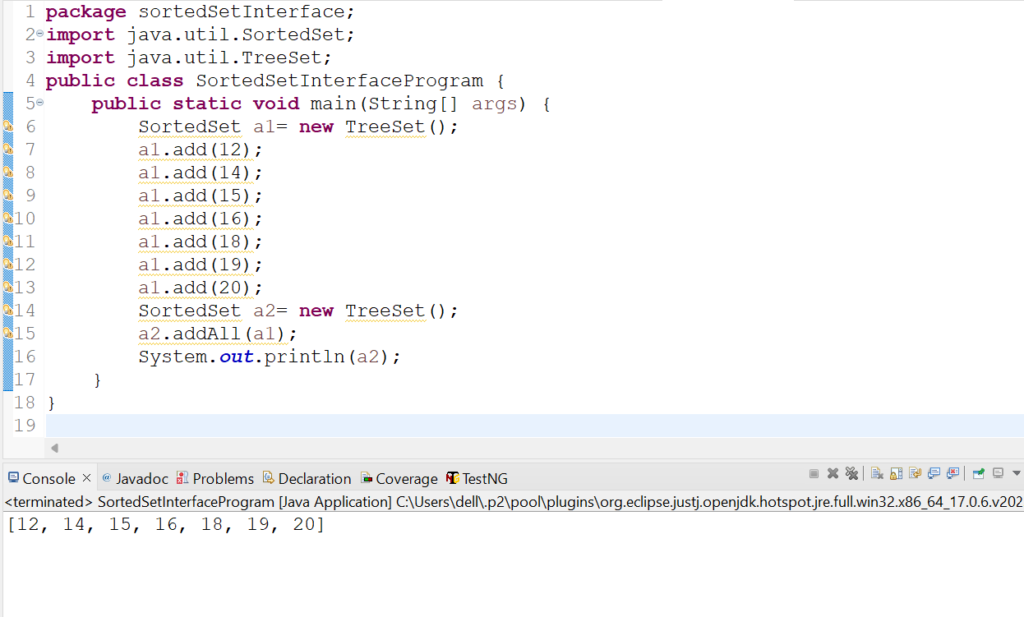
3. Let us check the usage of clear() method in SortedSet interface.
 Code Snippet :
package sortedSetInterface;
import java.util.TreeSet;
import java.util.SortedSet;
public class SortedSetInterfaceProgram {
public static void main(String[] args) {
SortedSet a1= new TreeSet();
a1.add(12);
a1.add(14);
a1.add(15);
a1.add(16);
a1.add(18);
a1.add(19);
a1.add(20);
a1.clear();
System.out.println(a1);
}
}
Output: []
Screenshot:
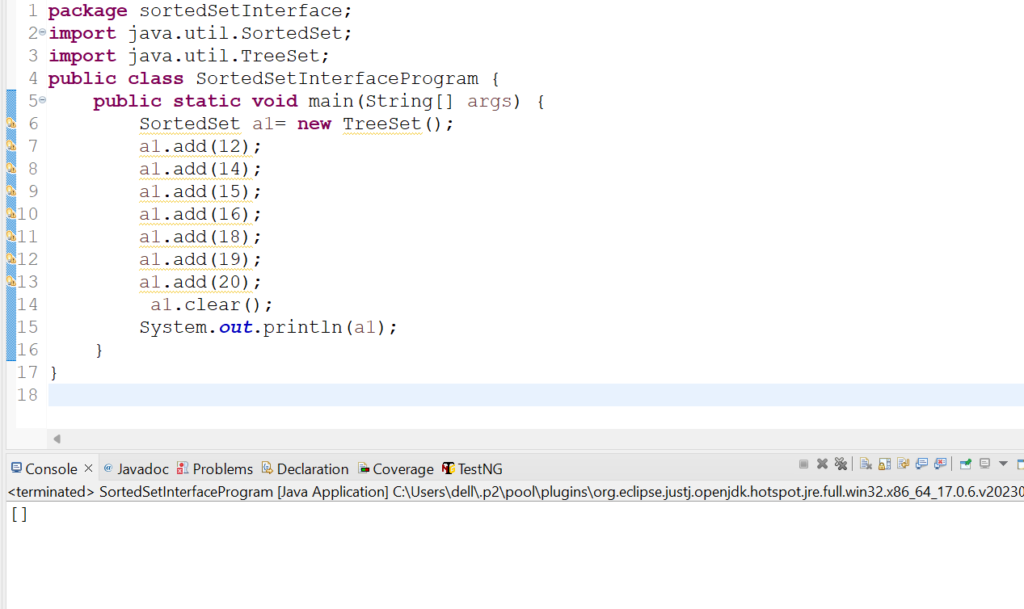
4. Let us check the usage of contains(Object o) method in SortedSet interface.
 Code Snippet:
package sortedSetInterface;
import java.util.TreeSet;
import java.util.SortedSet;
public class SortedSetInterfaceProgram {
public static void main(String[] args) {
SortedSet a1= new TreeSet();
a1.add("Hi");
a1.add("Hey");
a1.add("Hello");
a1.add("Hiji");
a1.add("Hji");
a1.add("Harish");
a1.add("Harshdeep");
System.out.println("The given SortedSet contains: "+a1.contains("hello"));
System.out.println("The given SortedSet contains: "+a1.contains("Harish"));
}
}
Output: The given SortedSet contains: false
The given SortedSet contains: true
Screenshot:Â

5. Let us check the usage of containsAll(Collection c) method in SortedSet interface
 Code Snippet:
package sortedSetInterface;
import java.util.TreeSet;
import java.util.SortedSet;
public class SortedSetInterfaceProgram {
public static void main(String[] args) {
SortedSet a1= new TreeSet();
a1.add("Hi");
a1.add("Hey");
a1.add("Hello");
a1.add("Hiji");
a1.add("Hji");
a1.add("Harish");
a1.add("Harshdeep");
SortedSet a2= new TreeSet();
a2.add("Hi");
a2.add("Hey");
a2.add("Hello");
a2.add("Hiji");
System.out.println("Does a2 contains all elements of a1:"+a2.containsAll(a1));
System.out.println("Does a1 contains all elements of a2:"+a1.containsAll(a2));
}
}
Output: Does a2 contains all elements of a1:false
Does a1 contains all elements of a2:true
Screenshot:Â

6. Let us check the usage of isEmpty() method in SortedSet interface.
 Code Snippet:
package sortedSetInterface;
import java.util.TreeSet;
import java.util.SortedSet;
public class SortedSetInterfaceProgram {
public static void main(String[] args) {
SortedSet a1= new TreeSet();
a1.add("Hi");
a1.add("Hey");
a1.add("Hello");
a1.add("Hiji");
a1.add("Hji");
a1.add("Harish");
a1.add("Harshdeep");
System.out.println("Is a1 SortedSet empty?: "+a1.isEmpty());
SortedSet a2= new TreeSet();
System.out.println("Is a2 SortedSet empty?: "+a2.isEmpty());
}
}
Output: Is a1 SortedSet empty?: false
Is a2 SortedSet empty?: true
Screenshot:

7. Let us check the usage of iterator() method in SortedSet interface.
Code Snippet:
package sortedSetInterface;
import java.util.TreeSet;
import java.util.Iterator;
import java.util.SortedSet;
public class SortedSetInterfaceProgram {
public static void main(String[] args) {
SortedSet a1= new TreeSet();
a1.add("Hi");
a1.add("Hey");
a1.add("Hello");
a1.add("Hiji");
a1.add("Hji");
a1.add("Harish");
a1.add("Harshdeep");
Iterator a2= a1.iterator();
System.out.println("The collection elements values are: ");
while(a2.hasNext())
{
System.out.println(a2.next());
}
}
}
Output: The collection elements values are:
Harish
Harshdeep
Hello
Hey
Hi
Hiji
Hji
Screenshot:
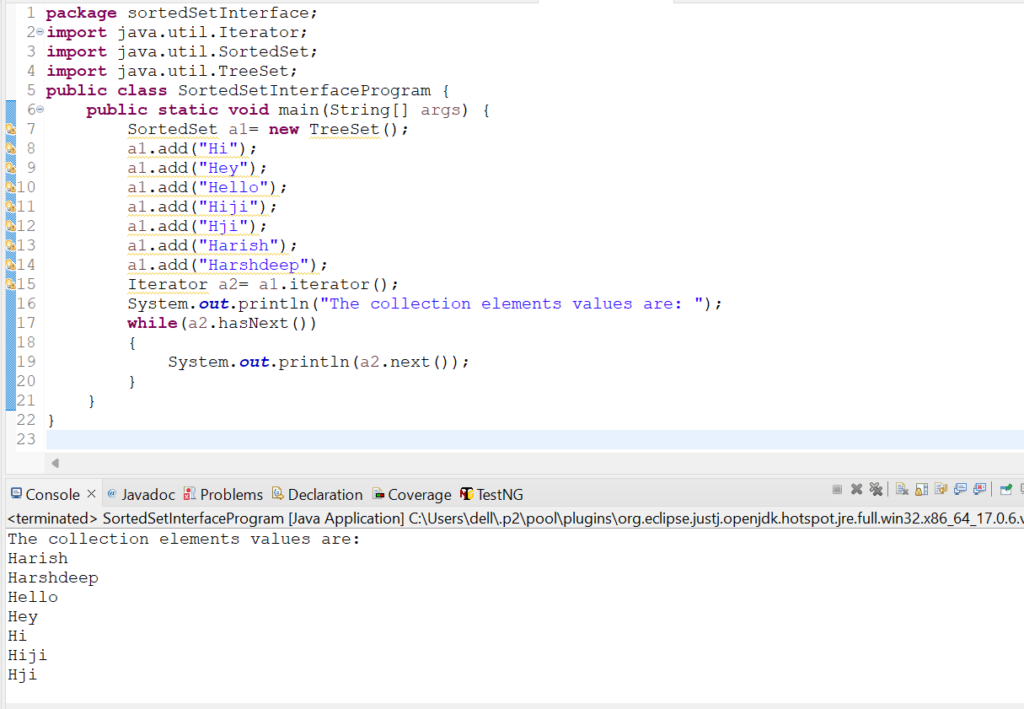
8. Let us check the usage of remove(Object o) method in SortedSet interface
 Code Snippet:
package sortedSetInterface;
import java.util.TreeSet;
import java.util.Iterator;
import java.util.SortedSet;
public class SortedSetInterfaceProgram {
public static void main(String[] args) {
SortedSet a1= new TreeSet();
a1.add("Hi");
a1.add("Hey");
a1.add("Hello");
a1.add("Hiji");
a1.add("Hji");
a1.add("Harish");
a1.add("Harshdeep");
a1.remove("Hello");
System.out.println(a1);
}
}
Output: [Harish, Harshdeep, Hey, Hi, Hiji, Hji]
Screenshot:

9. Let us check the usage of removeAll(Collection c) method in SortedSet interface
Code Snippet:
package sortedSetInterface;
import java.util.TreeSet;
import java.util.SortedSet;
public class SortedSetInterfaceProgram {
public static void main(String[] args) {
SortedSet a1= new TreeSet();
a1.add("Hi");
a1.add("Hey");
a1.add("Hello");
a1.add("Hiji");
a1.add("Hji");
a1.add("Harish");
a1.add("Harshdeep");
SortedSet a2= new TreeSet();
a2.add("Hi");
a2.add("Hey");
a2.add("Hello");
a2.add("Hiji");
a1.removeAll(a2);
System.out.println("Elements present in a1 after removing a2 elements from a1 are:"+a1);
}
}
Output: Elements present in a1 after removing a2 elements from a1 are:[Harish, Harshdeep, Hji]
System.out.println("Elements present in a1 after removing a2 elements from a1 are:"+a1);
}
}
Screenshot:
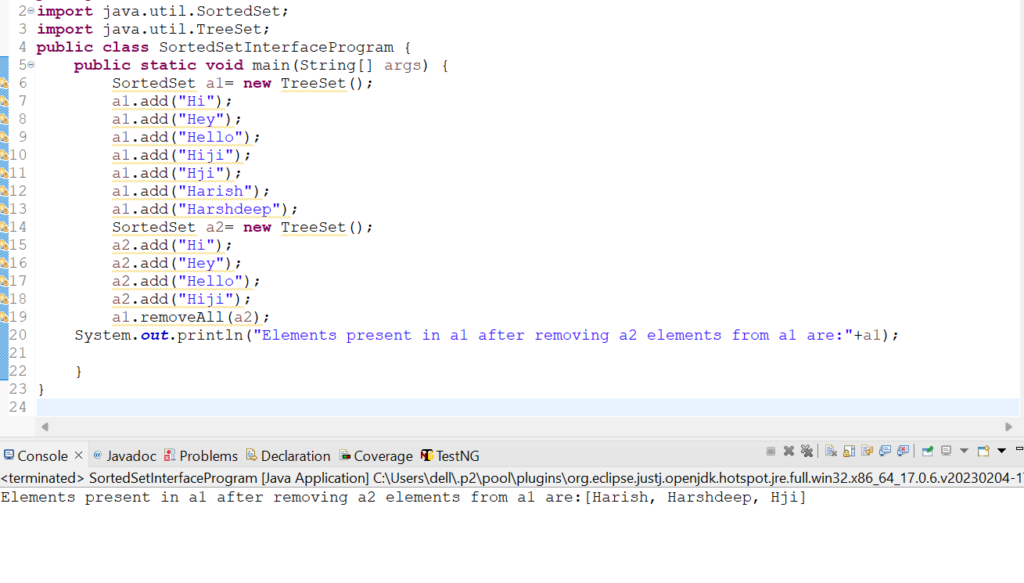
10. Let us check the usage of retainAll(Collection c) method in SortedSet interface.
Code Snippet:
package sortedSetInterface;
import java.util.TreeSet;
import java.util.SortedSet;
public class SortedSetInterfaceProgram {
public static void main(String[] args) {
SortedSet a1= new TreeSet();
a1.add("Hi");
a1.add("Hey");
a1.add("Hello");
a1.add("Hiji");
a1.add("Hji");
a1.add("Harish");
a1.add("Harshdeep");
SortedSet a2= new TreeSet();
a2.add("Hi");
a2.add("Hey");
a2.add("Hello");
a2.add("Hiji");
a1.retainAll(a2);
System.out.println("Elements present in a1 after retaining a2 elements are:"+a1);
}
}
Output:
Elements present in a1 after retaining a2 elements are:[Hello, Hey, Hi, Hiji]
Screenshot:
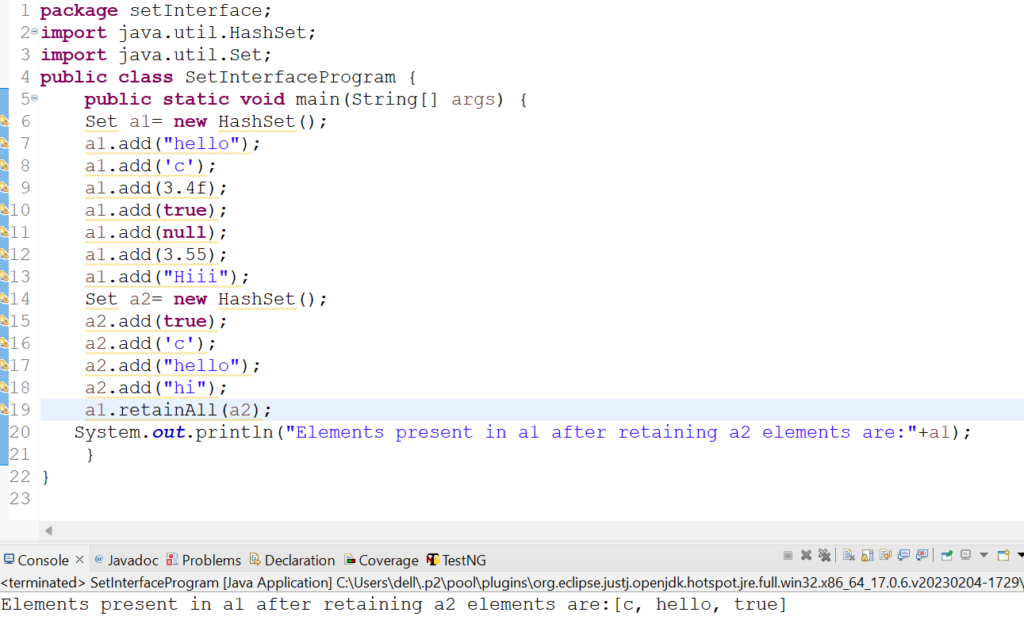
11. Let us check the usage of size() method in SortedSet interface.
Code Snippet:
package sortedSetInterface;
import java.util.TreeSet;
import java.util.SortedSet;
public class SortedSetInterfaceProgram {
public static void main(String[] args) {
SortedSet a1= new TreeSet();
a1.add("Hi");
a1.add("Hey");
a1.add("Hello");
a1.add("Hiji");
a1.add("Hji");
a1.add("Harish");
a1.add("Harshdeep");
System.out.println("The size of the collection elements present in the SortedSet is: "+a1.size());
}
}
Output: The size of the collection elements present in the SortedSet is: 7
Screenshot:

12. Let us check the usage of toArray() method in SortedSet interface
 Code Snippet :
package sortedSetInterface;
import java.util.TreeSet;
import java.util.SortedSet;
public class SortedSetInterfaceProgram {
public static void main(String[] args) {
SortedSet a1= new TreeSet();
a1.add("Hi");
a1.add("Hey");
a1.add("Hello");
a1.add("Hiji");
a1.add("Hji");
a1.add("Harish");
a1.add("Harshdeep");
Object a2[]= a1.toArray();
System.out.println("The list of array elements are:");
for(int i=0;i<=a2.length-1;i++)
{
System.out.println(a2[i]);
}
}
}
Output: The list of array elements are:
Harish
Harshdeep
Hello
Hey
Hi
Hiji
Hji
Screenshot:

13. Let us check the usage of toString() method in SortedSet interface.
Code Snippet:
package sortedSetInterface;
import java.util.TreeSet;
import java.util.SortedSet;
public class SortedSetInterfaceProgram {
public static void main(String[] args) {
SortedSet a1= new TreeSet();
a1.add("Hi");
a1.add("Hey");
a1.add("Hello");
a1.add("Hiji");
a1.add("Hji");
a1.add("Harish");
a1.add("Harshdeep");
System.out.println(a1.toString());
}
}
Output: [Harish, Harshdeep, Hello, Hey, Hi, Hiji, Hji]
 Screenshot:
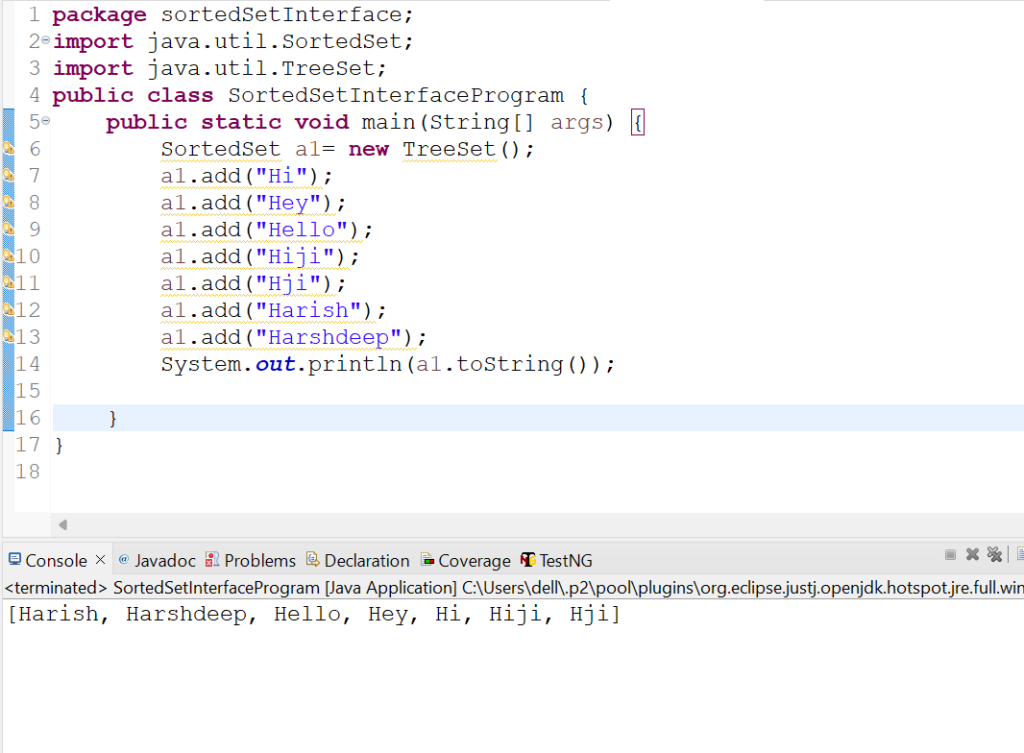
14. Let us check the usage of equals() method in SortedSet interface.
  Code Snippet:
package sortedSetInterface;
import java.util.TreeSet;
import java.util.SortedSet;
public class SortedSetInterfaceProgram {
public static void main(String[] args) {
SortedSet a1= new TreeSet();
a1.add("Hi");
a1.add("Hey");
a1.add("Hello");
a1.add("Hiji");
a1.add("Hji");
a1.add("Harish");
a1.add("Harshdeep");
SortedSet a2= new TreeSet();
a2.add("Hi");
a2.add("Hey");
a2.add("Hello");
a2.add("Hiji");
a2.add("Hji");
a2.add("Harish");
a2.add("Harshdeep");
SortedSet a3= new TreeSet();
a3.add("Hi");
a3.add("Hey");
a3.add("Hello");
a3.add("Hiji");
System.out.println("Is SortedSet a1 equal to a2 ?:"+ a1.equals(a2));
System.out.println("Is SortedSet a2 equal to a3 ?:"+ a2.equals(a3));
}
}
Output: Is SortedSet a1 equal to a2 ?:true
Is SortedSet a2 equal to a3 ?:false
Screenshot:
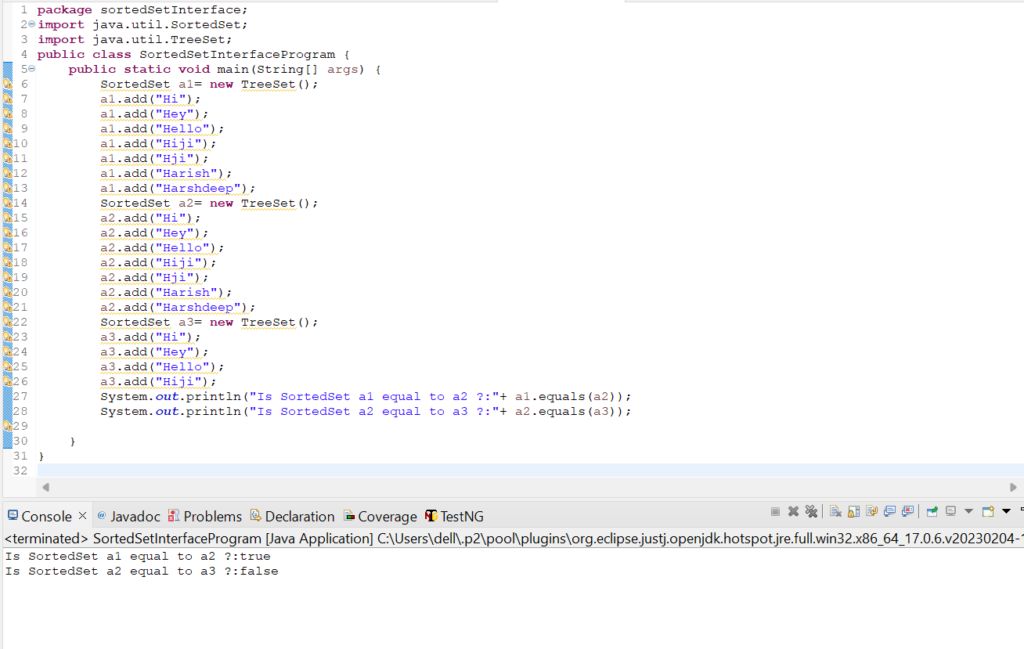
Differences between List Interface and SortedSet Interface
SL NO: | List | SortedSet |
1 | List follows indexing | SortedSet does not follow indexing |
2 | List allows duplicate elements | SortedSet does not allow duplicate elements |
3 | List allows any number of null values | SortedSet allows only one null value |
4 | List follows order of insertion | SortedSet does not follow order of insertion |
5 | We iterate List elements using Iterator and ListIterator | We iterate SortedSet elements only by using Iterator |
6 | ArrayList,LinkedList and Vector are some of the implemented classes of List interface. | TreeSortedSet,TreeSet and LinkedTreeSet are some of the implemented methods of SortedSet. |
Conclusion
SortedSet interface is used for handling all the Collection elements in an effective way. It is necessary to understand the usage of all the implemented classes and interfaces of SortedSet interfaces as well as its methods for better handling of Collection elements. Remember to practice, stay updated with the latest trends in Automation Software Testing Course,and maintain a positive attitude throughout your interview process. By thoroughly understanding the SortedSet interface and its methods developers and testers can utitlise those concepts in their real life tasks.
Also read:
Automation testing is a software testing technique that uses specialised tools and scripts to run predefined test cases, compare the results to what was expected, and report the results.
The fundamental purpose of automated testing is to improve the efficiency, effectiveness, and coverage of the testing process by automating repetitive and time-consuming processes that would otherwise be completed manually
Consult Us