
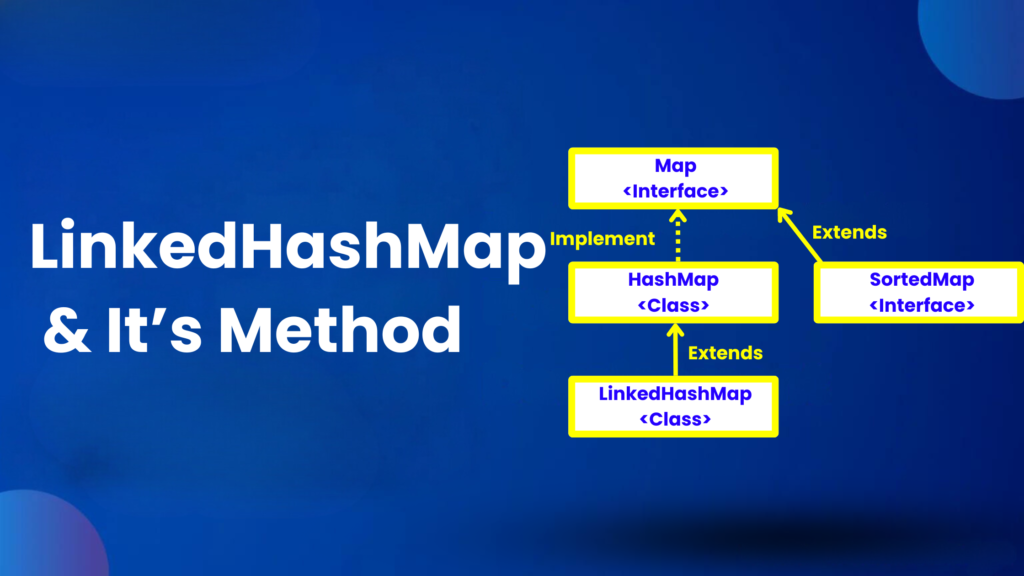
LinkedHashMap and its methods
Before going into LinkedHashMap let us discuss about Map Hierarchy
Map Hierarchy
The below diagram shows the Map hierarchy consisting of classes and interfaces.
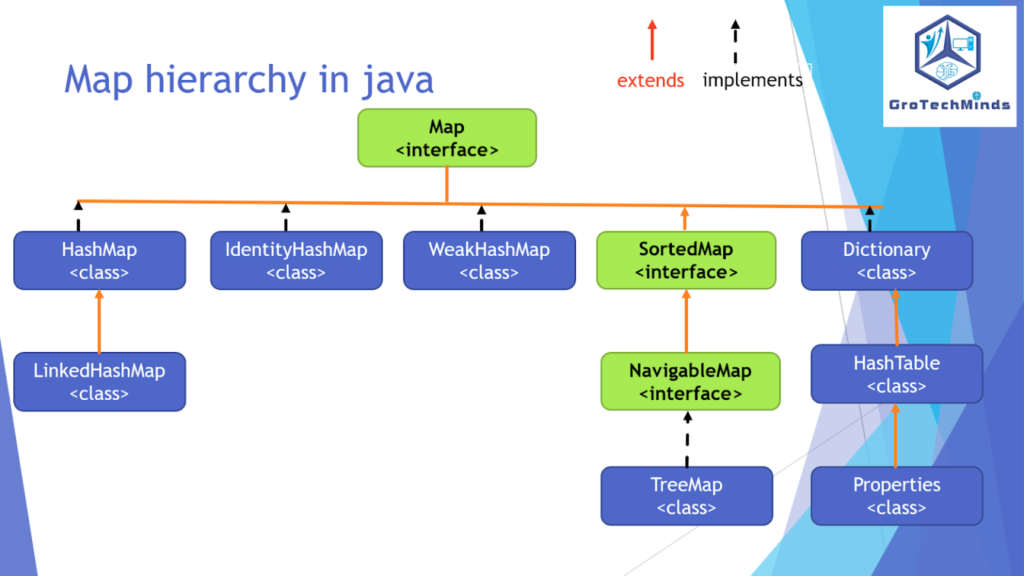
Now let us discuss about LinkedHashMap and its methods
What is LinkedHashMap?
LinkedHashMap is the implemented class of Map interface and sub class of HashMap class. It is present in java.utils package. LinkedHashMap has one null key and multiple null values. It does not accept duplicate elements. Using LinkedHashMap insertion order can be maintained easily since it uses doubly-linkedlist. It is neither threadsafe nor synchronized as a result it has faster execution. It increases its capacity when it reaches the load factor value of 0.75. Its threshold is 0.75 times the current capacity.
Methods of LinkedHashMap
Below are some of the methods of LinkedHashMap:
Method Name | Description |
put(Object key, Object value) | It is used to add a pair of key and value into the LinkedHashMap. |
putAll(Map m) | It is used to add all pairs of keys and values from one LinkedHashMap to another LinkedHashMap. |
clear() | It is used to clear all key and value pairs present in LinkedHashMap. |
size() | It is used to display the size of the key and value pairs present in LinkedHashMap. |
equals(Object o) | It is used to check if key and value pairs present in one LinkedHashMap is equal to the key and value pairs present in another LinkedHashMap. It gives ‘true’ value if key and value pairs present in two LinkedHashMaps are equal and ‘false’ value if key and value pairs present in two LinkedHashMaps are not equal. |
containsKey(Object key) | It is used to check if the specified key is present among all the key and value pairs of LinkedHashMap class. It gives ‘true’ value if the specified Key is present among all the key and value pairs and ‘false’ value if the specified key is not present among all the key and value pairs. |
containsValue(Object value) | It is used to check if the specified value of a key is present among all the key and value pairs of LinkedHashMap class. It gives ‘true’ value if the specified value of a key is present among all the key and value pairs and ‘false’ value if the specified value of a key is not present among all the key and value pairs. |
get(Object key) | It is used to display the value of a specified key among all key and value pairs of HashSet. It displays ‘null’ if the specified key is not present among all key and value pairs of LinkedHashMap. |
isEmpty() | It is used to check if the LinkedHashMap class is empty or not. It display ‘true’ value if the LinkedHashMap class is empty and ‘false’ value if the LinkedHashMap class is not empty. |
keySet() | It is used to display the keys among all the key and value pairs present in LinkedHashMap. |
remove(Object key) | It is used to remove a specified key and value pair from the LinkedHashMap. If we try to print a key which is not present in LinkedHashMap it will display null value but if we try to print a key which is present in LinkedHashMap it will display its corresponding value. |
values() | It is used to display the corresponding values of the keys present in the LinkedHashMap |
putIfAbsent(Object key, Object value) | Using this method, the specified key and value pairs get added alongwith the key and value pairs present in LinkedHashMap if that key and value pair is absent in the LinkedHashMap. If the specified key and value is present in the LinkedHashMap the specified key and value pair will not get added again in the LinkedHashMap. This method will also display the value of the specified key and value pair if that key and value pair is present in LinkedHashMap. If the specified key and value pair is not displayed in LinkedHashMap then null will be displayed. |
replace(Object key, Object oldValue, Object newValue) | It is used to replace the old value of the specified key with a new value. Here we also mention the old value of the specified key. |
replace(Object key, Object value) | It is used to replace the old value of the specified key with a new value. |
entrySet() | It is used to display all the key and value pairs present in LinkedHashMap. |
iterator() | It is used to iterate sequentially all the key and value pairs present in LinkedHashMap. |
clone() | It is used to create a shallow copy of the key and value pairs present in LinkedHashMap. |
1. Let us check how put(Object key, Object value) method works in LinkedHashMap class.
Code Snippet:
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println(m1);
}
}
Output: {Roll No=1101219512, Registration Number=1653005, Pin Number=3434, Student ID=25, Postal code=751121}
  Screenshot:

2. Let us check how putAll(Map m) method works in LinkedHashMap class.
Code Snippet:
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println(m1);
LinkedHashMap m2= new LinkedHashMap();
m2.putAll(m1);
System.out.println(m2);
}
}
Output: {Roll No=1101219512, Registration Number=1653005, Pin Number=3434, Student ID=25, Postal code=751121}
{Roll No=1101219512, Registration Number=1653005, Pin Number=3434, Student ID=25, Postal code=751121}
Screenshot:
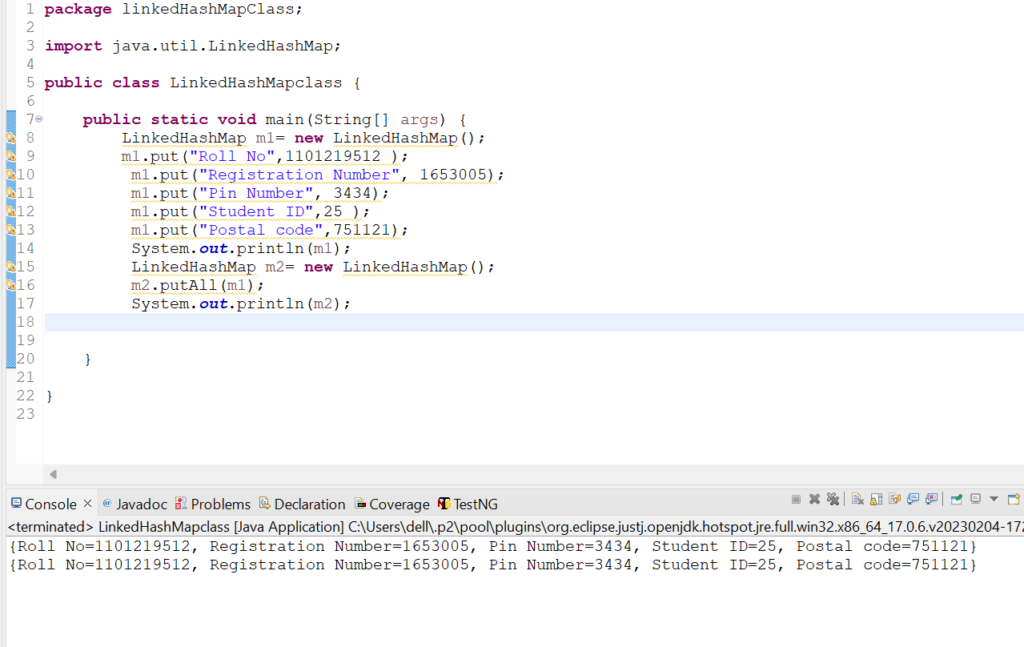
3. Let us check how clear() method works in LinkedHashMap class.
Code Snippet:
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
m1.clear();
System.out.println(m1);
}
}
Output: {}
  Screenshot:
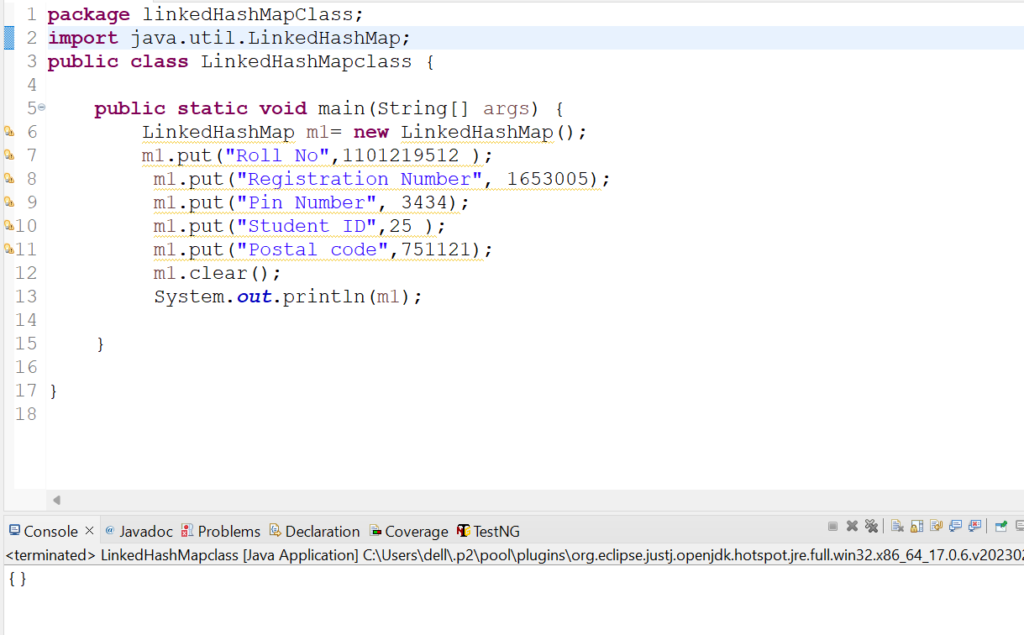
4. Let us check how size() method works in LinkedHashMap class.
Code Snippet:
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println(m1.size());
}
}
Output: 5
Screenshot:
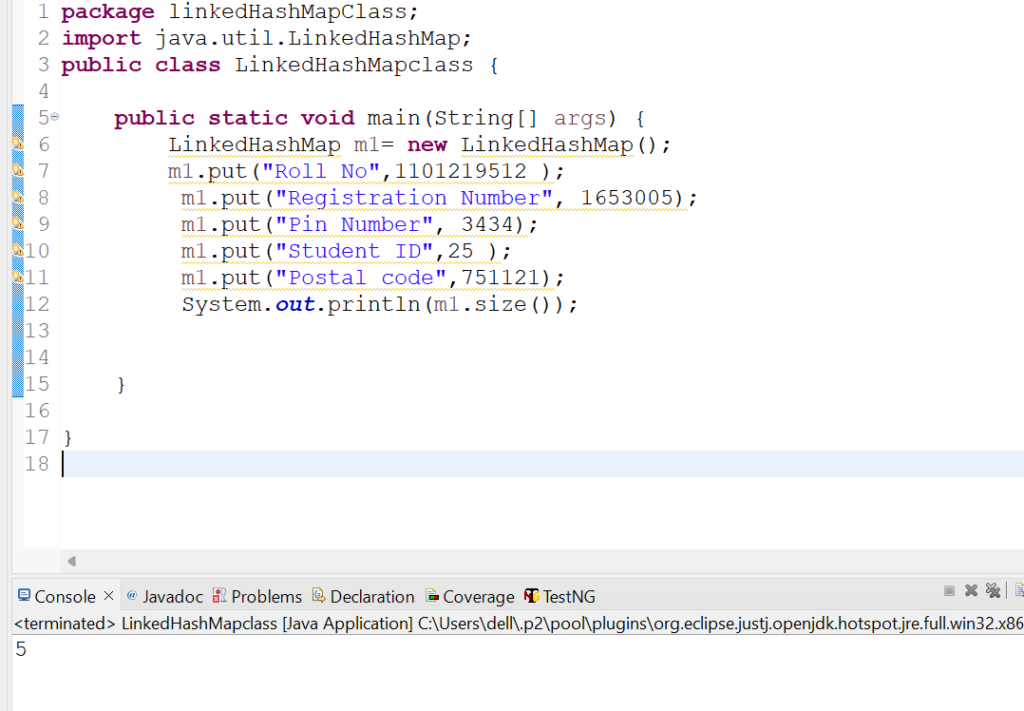
5. Let us check how equals(Object o) method works in LinkedHashMap class.
Code Snippet:
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
LinkedHashMap m2= new LinkedHashMap();
m2.put("Roll No",1101219512 );
m2.put("Registration Number", 1653005);
m2.put("Pin Number", 3434);
m2.put("Student ID",25 );
m2.put("Postal code",751121);
LinkedHashMap m3= new LinkedHashMap();
m3.put("Roll No",1101219512 );
m3.put("Registration Number", 1653005);
m3.put("Pin Number", 3434);
m3.put("Student ID",25 );
m3.put("Postal code",751121);
m3.put("Flat No",01);
System.out.println("Is LinkedHashMap m1 equals m2 ?: "+m1.equals(m2));
System.out.println("Is LinkedHashMap m1 equals m3 ?: "+m1.equals(m3));
}
}
Output: Is LinkedHashMap m1 equals m2 ?: true
Is LinkedHashMap m1 equals m3 ?: false
Screenshot:

6. Let us check how containsKey(Object key) method works in LinkedHashMap class.
Code Snippet:
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println("Does the LinkedHashMap contains Registration Number key?: "+m1.containsKey("Registration Number"));
System.out.println("Does the LinkedHashMap contains Flat No key?: "+m1.containsKey("Flat No"));
}
}
Output: Does the LinkedHashMap contains Registration Number key?: true
Does the LinkedHashMap contains Flat No key?: false
Screenshot:
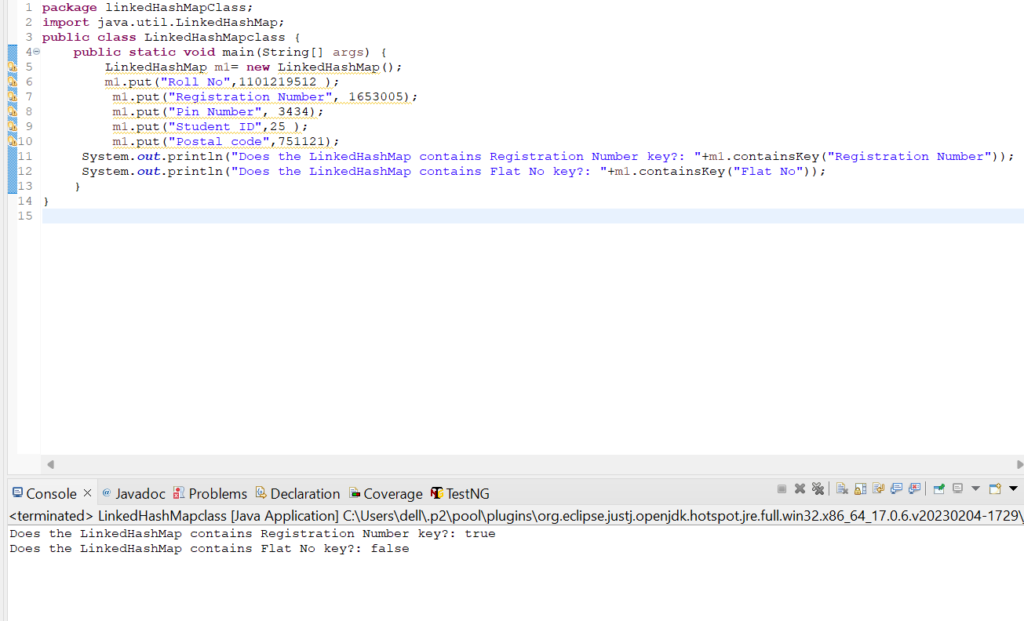
7. Let us check how containsValue(Object value) method works in LinkedHashMap class.
Code Snippet:Â Â
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println("Does the LinkedHashMap contains 1653005 value?: "+m1.containsValue(1653005));
System.out.println("Does the LinkedHashMap contains 01 value?: "+m1.containsValue(01));
}
}
Output: Does the LinkedHashMap contains 1653005 value?: true
Does the LinkedHashMap contains 01 value?: false
Screenshot:Â Â

8. Let us check how get(Object key) method works in LinkedHashMap class.
Code Snippet:
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println(m1.get("Pin Number"));
System.out.println(m1.get("Registration Number"));
}
}
Output:
3434
1653005
Screenshot:
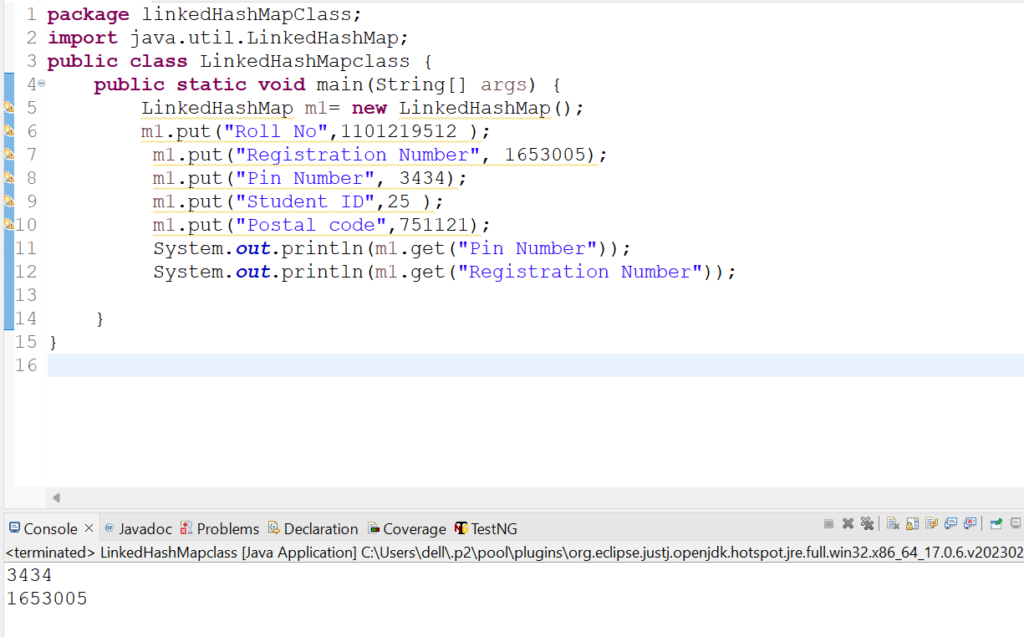
9. Let us check how isEmpty() method works in LinkedHashMap class.
Code Snippet:Â Â
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println("Is LinkedHashMap m1 empty? :" +m1.isEmpty());
LinkedHashMap m2= new LinkedHashMap();
System.out.println("Is LinkedHashMap m2 empty? :" +m2.isEmpty());
}
}
Output: Is LinkedHashMap m1 empty? :false
Is LinkedHashMap m2 empty? :true
 Screenshot:

10. Let us check how keySet() method works in LinkedHashMap class.
Code Snippet:
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println(m1.keySet());
}
}
Output: [Roll No, Registration Number, Pin Number, Student ID, Postal code]
Screenshot:
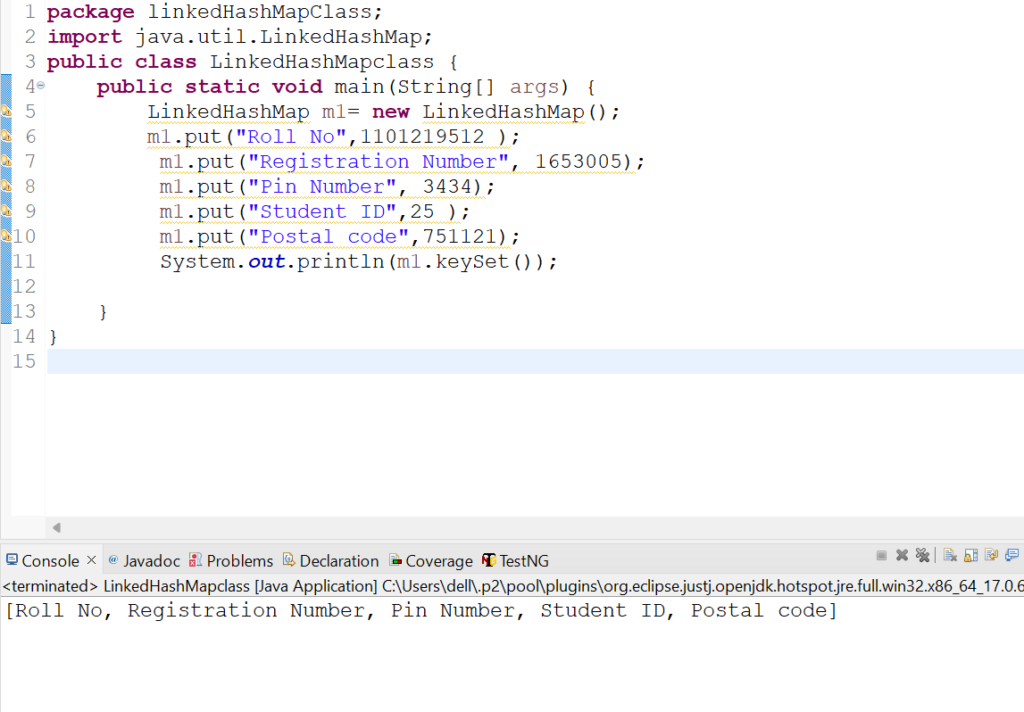
11. Let us check how remove(Object key) method works in LinkedHashMap class.
Code Snippet:
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println(m1);
m1.remove("Registration Number");
System.out.println("New key value pairs are:");
System.out.println(m1);
System.out.println(m1.remove("Flat No"));
System.out.println(m1.remove("Student ID"));
}
}
Output:
{Roll No=1101219512, Registration Number=1653005, Pin Number=3434, Student ID=25, Postal code=751121}
New key value pairs are:
{Roll No=1101219512, Pin Number=3434, Student ID=25, Postal code=751121}
null
25
Screenshot:
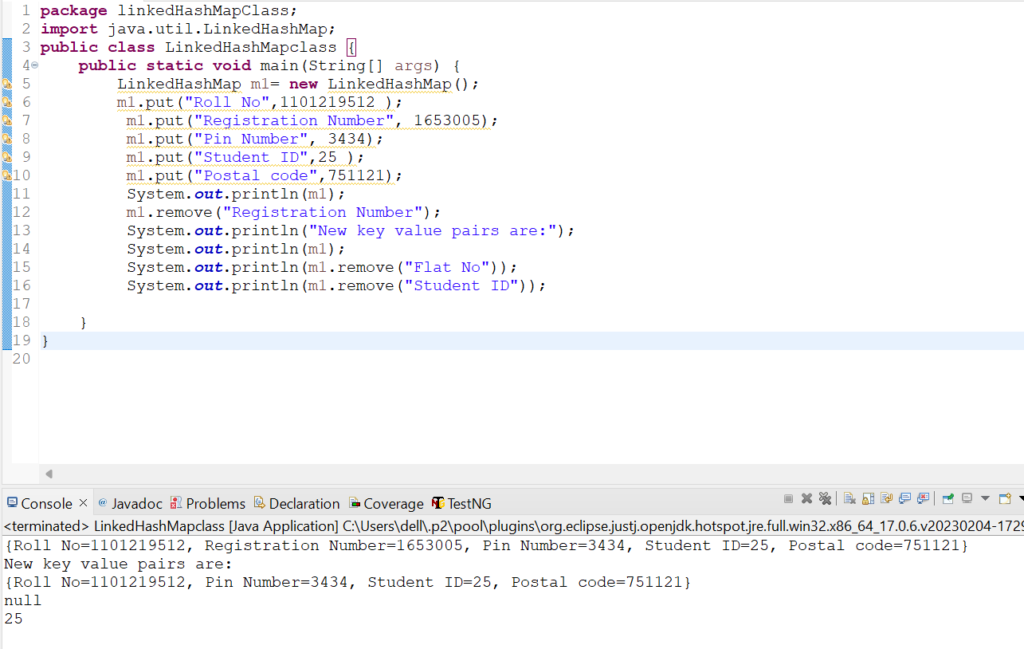
12. Let us check how values() method works in LinkedHashMap class.
Code Snippet:
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println(m1.values());
}
}
Output: [1101219512, 1653005, 3434, 25, 751121]
 Screenshot:
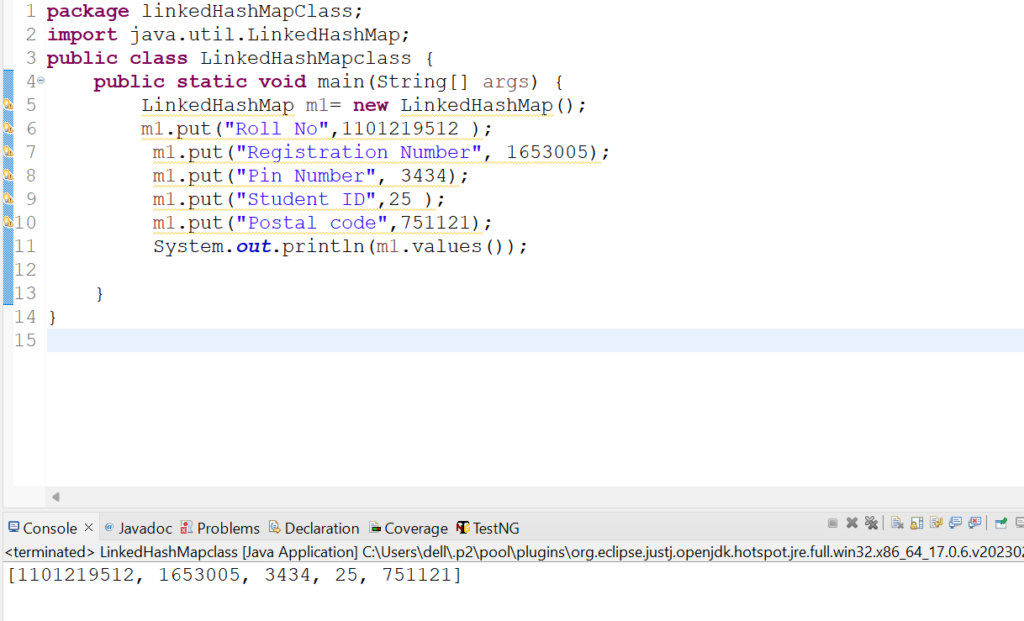
13. Let us check how putIfAbsent(Object key, Object value) method works in LinkedHashMap class.
 Code Snippet:
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println(m1);
System.out.println(m1.putIfAbsent("Registration Number", 1653005));
System.out.println(m1);
System.out.println(m1.putIfAbsent("Flat No",01));
System.out.println(m1);
}
}
Output: {Roll No=1101219512, Registration Number=1653005, Pin Number=3434, Student ID=25, Postal code=751121}
1653005
{Roll No=1101219512, Registration Number=1653005, Pin Number=3434, Student ID=25, Postal code=751121}
null
{Roll No=1101219512, Registration Number=1653005, Pin Number=3434, Student ID=25, Postal code=751121, Flat No=1}
Screenshot:
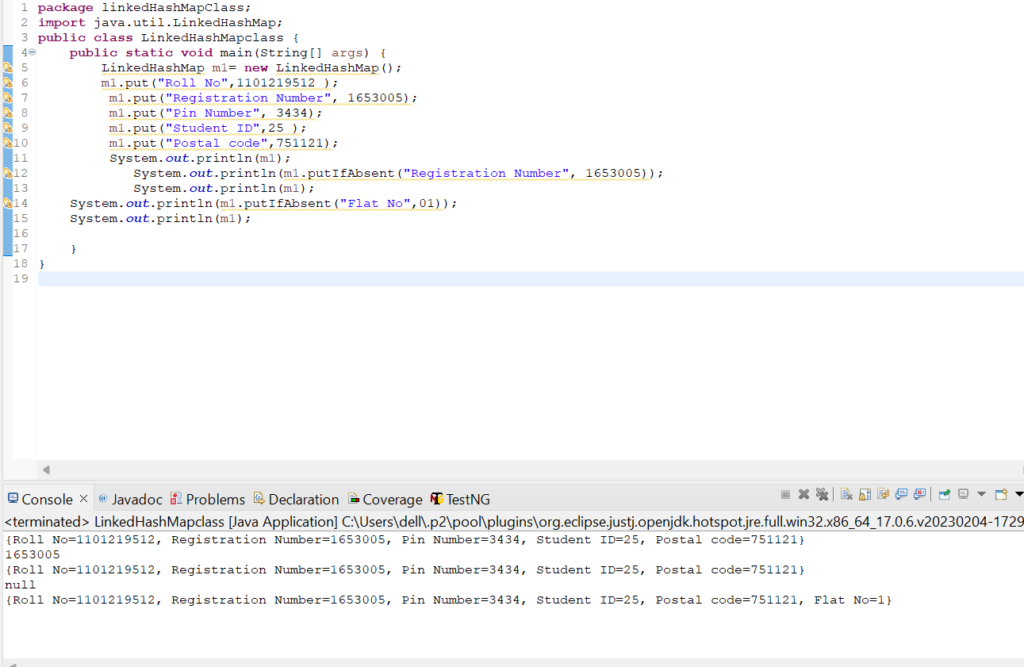
14. Let us check how replace(Object key, Object oldValue, Object newValue) method works in LinkedHashMap class.
Code Snippet:
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println(m1);
m1.replace("Registration Number", 1653005, 1653007);
System.out.println(m1);
}
}
Output: {Roll No=1101219512, Registration Number=1653005, Pin Number=3434, Student ID=25, Postal code=751121}
{Roll No=1101219512, Registration Number=1653007, Pin Number=3434, Student ID=25, Postal code=751121}
Screenshot:
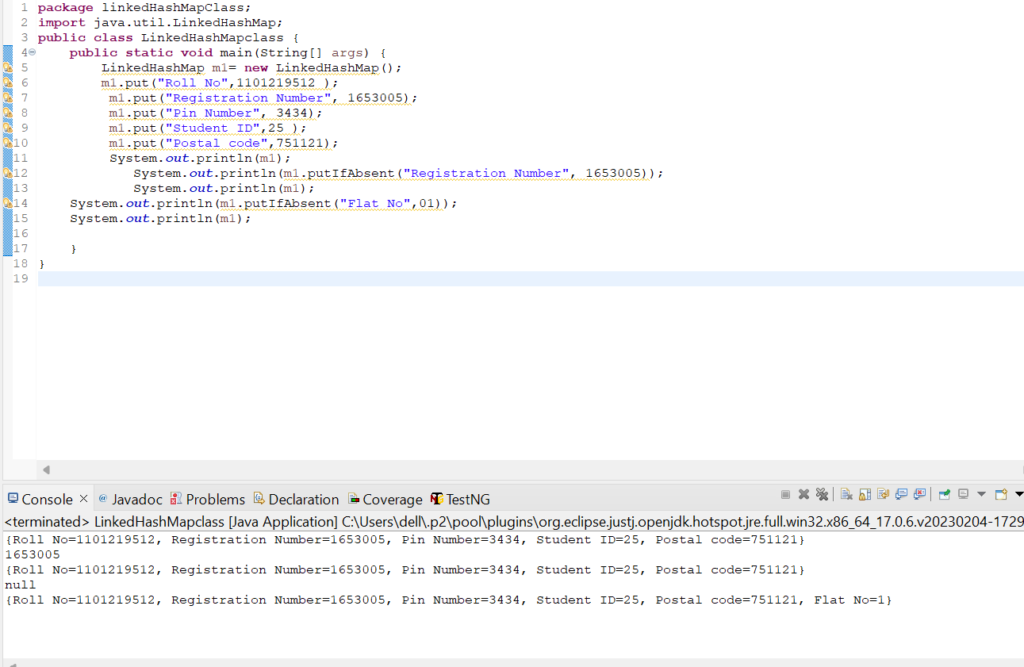
15. Let us check how replace(Object key, Object value) method works in LinkedHashMap class.
 Code Snippet:
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println(m1);
m1.replace("Registration Number", 1653005);
System.out.println(m1);
}
}
Output:{Roll No=1101219512, Registration Number=1653005, Pin Number=3434, Student ID=25, Postal code=751121}
{Roll No=1101219512, Registration Number=1653005, Pin Number=3434, Student ID=25, Postal code=751121}
Screenshot:

16. Let us check how entrySet() method works in LinkedHashMap class.
Code Snippet:Â
package linkedHashMapclass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println(m1.entrySet());
}
}
Output: [Roll No=1101219512, Registration Number=1653005, Pin Number=3434, Student ID=25, Postal code=751121]
Screenshot:
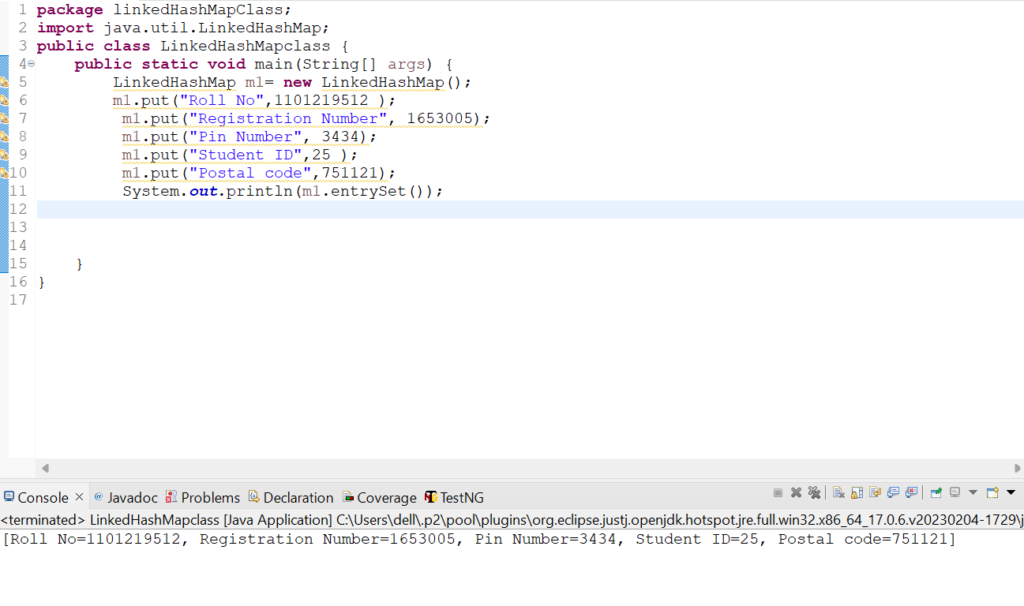
17. Let us check how iterator() method works in LinkedHashMap class.
Code Snippet:
package linkedHashMapclass;
import java.util.LinkedHashMap;
import java.util.Iterator;
import java.util.Map.Entry;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
Iterator> itr= m1.entrySet().iterator();
while(itr.hasNext())
{
System.out.println(itr.next());
}
}
}
Output: Roll No=1101219512
Registration Number=1653005
Pin Number=3434
Student ID=25
Postal code=751121
Screenshot:

18. Let us check how clone() method works in HashMap class.
Code Snippet:
package linkedHashMapClass;
import java.util.LinkedHashMap;
public class LinkedHashMapclass {
public static void main(String[] args) {
LinkedHashMap m1= new LinkedHashMap();
m1.put("Roll No",1101219512 );
m1.put("Registration Number", 1653005);
m1.put("Pin Number", 3434);
m1.put("Student ID",25 );
m1.put("Postal code",751121);
System.out.println(m1);
System.out.println(m1.clone());
}
}
Output: {Roll No=1101219512, Registration Number=1653005, Pin Number=3434, Student ID=25, Postal code=751121}
{Roll No=1101219512, Registration Number=1653005, Pin Number=3434, Student ID=25, Postal code=751121}
Screenshot:
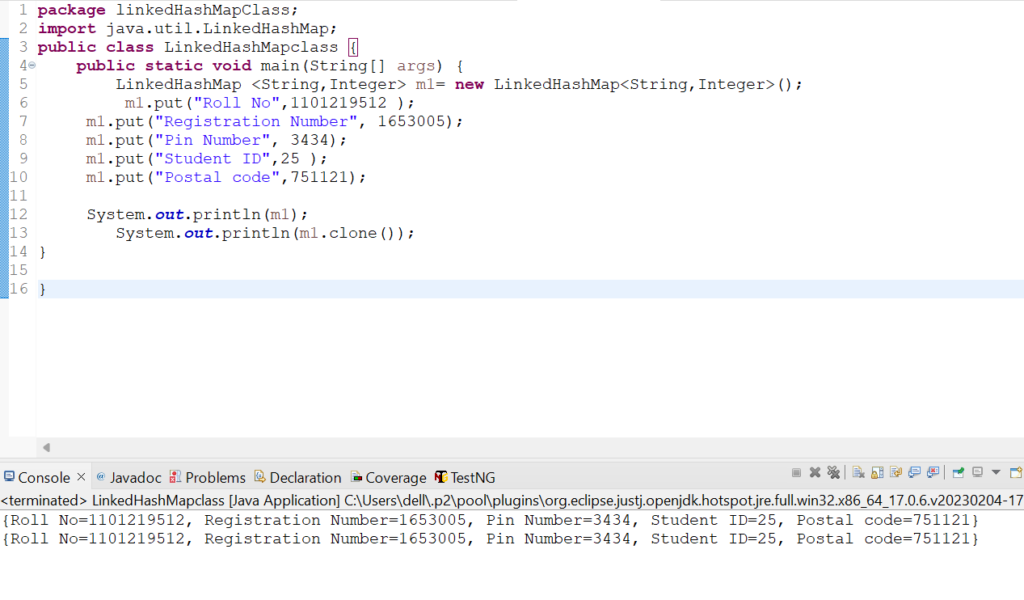
Difference between HashMap and LinkedHashMap
SL NO: | HashMap | LinkedHashMap |
1 | It is the parent class of LinkedHashMap | It is the child class of HashMap |
2 | Stores elements in hash table. | Stores elements in key-value pairs. |
3 | Provides better performance while accessing over large datasets. | Provides better performance while iterating through elements. |
4 | Does not use doubly- linkedlist to maintain order of elements | Uses doubly- linkedlist to maintain order of elements. |
5 | Insertion and Deletion of elements is slower. | Insertion and Deletion of elements is faster. |
Conclusion:
LinkedHashMap class is used for handling all the key and value pairs in an effective way. It is necessary to understand the usage of all the methods of the LinkedHashMap class for better handling of LinkedHashMap elements. By thoroughly understanding the LinkedHashMap class and its methods developers and testers can utitlise those concepts in their real life tasks.Remember to practise, stay updated with the latest trends in Automation Software Testing Course,and maintain a positive attitude throughout your interview process.Automation testers can utlize all the methods of LinkedHashMap class to create better and effective test scripts for automation testing of web applications and websites.
Also, read my blogs on:
Consult Us