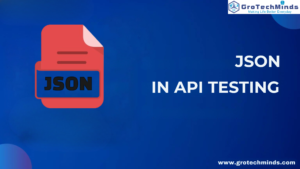
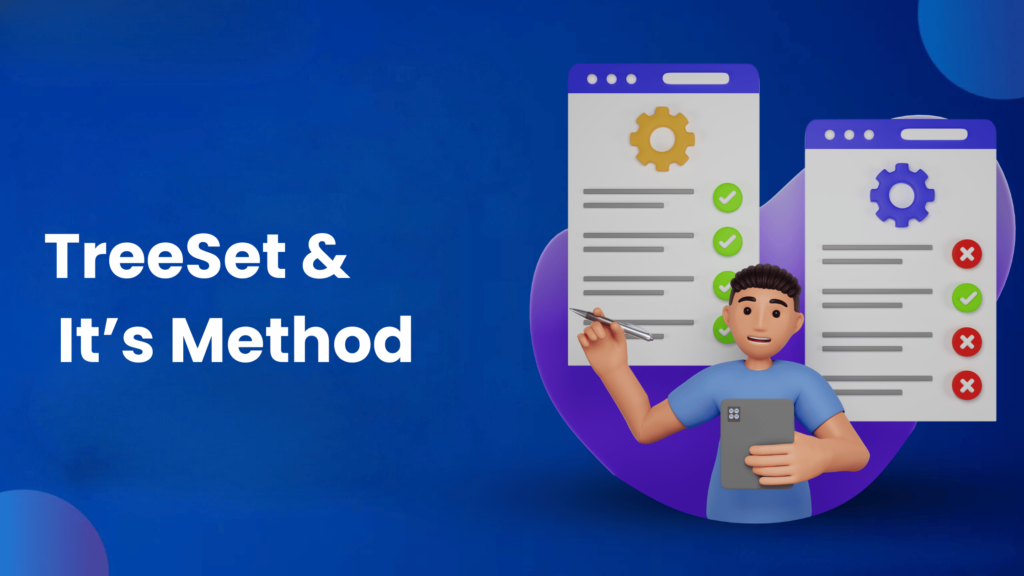
TreeSet and its Methods
Before we start with HashSet let us look at the Collection Hierarchy
Collection Hierarchy
The below diagram shows the Collection hierarchy consisting of classes and interfaces.
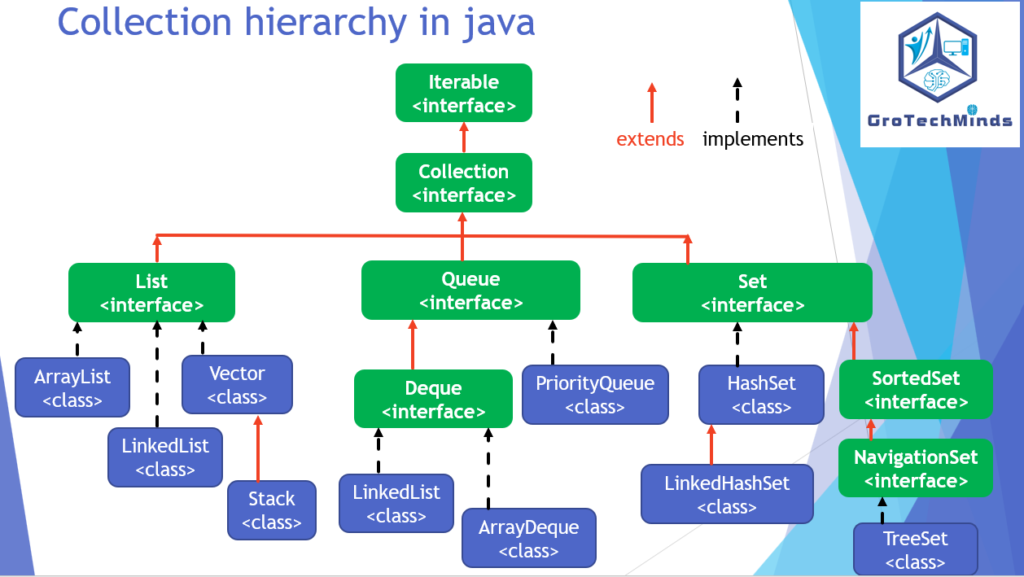
As per the scope of the blog our discussion will be limited to the TreeSet class
What is TreeSet?
TreeSet is a class which is used to store multiple elements. It is the implemented class of NavigationSet interface,SortedSet interface,Set interface,Collection interface and Iterable interface. TreeSet class comes from “java.utils” package.TreeSet is not an index based data structure and all the datas are stored in accordance with the hashcode values. Duplicate elements cannot be stored in TreeSet. TreeSet does not allow null value to be stored in it. TreeSet does not follow the order of insertion. TreeSet elements can be iterated by only Iterator method. TreeSet is nether thread safe nor synchronized. TreeSet grows dynamically and it has no threshold. Sorting happens in ascending order by default. The retrieval and access time of TreeSet class is very fast.
Methods of TreeSet class
Below are some of the methods of TreeSet class:
Method Name | Description |
add(Object e) | This method is used to add a new element of any datatype into the TreeSet class. |
addAll(Collection c) | This method is used to add all elements of different datatypes into the TreeSet class. |
clear() | It is used to clear all the elements from the Set interface but it does not clear the TreeSet class. |
contains(Object o) | This method is used to check the presence of a Collection element in the TreeSet. It returns ‘true’ value if the element is present in TreeSet and ‘false’ value if the element is not present in the TreeSet. |
containsAll(Collection c) | This method is used to check if the TreeSet contains all the collection elements. It returns ‘true’ value if all the elements are present in TreeSet and ‘false’ value if any one of the element is not present in the TreeSet. |
isEmpty() | This method checks if the given TreeSet is empty |
iterator() | This method is used to iterate sequentially all the Collection elements present in a TreeSet. |
remove(Object o) | This method is used to remove a specified collection element from the TreeSet. |
removeAll(Collection c) | This method removes all those Collection elements from the current TreeSet which are present in another TreeSet. |
retainAll(Collection c) | This method retains all those elements from the current TreeSet which are present in another TreeSet and removes those elements which are present in current TreeSet but not in another TreeSet and vice versa. |
size() | This method is used to find out and display the number of Collection elements present in the TreeSet |
toArray() | This method is used to convert all collection elements present in a TreeSet into an array. |
toString() | This method is used to convert all collection elements present in a TreeSet into a String. |
equals() | This method is used to check if two LinkedHashSets are equal. It returns ‘true’ value if elements as well as size of elements present in one TreeSet is equal to the elements and its size present in another TreeSet. If the elements and the size of elements present in one TreeSet is not equal to the elements and its size present in another TreeSet then it returns ‘false’ value. |
clone() | This method is used to create a shallow copy of the elements present in the TreeSet class. |
pollFirst() | This method is used to remove the lowest element from the TreeSet class. |
pollLast() | This method is used to remove the highest element from the TreeSet class. |
first() | It is used to display the smallest element of the TreeSet class. |
last() | It is used to display the largest element of the TreeSet class. |
descendingIterator() | This method is used to iterate all the Collection elements present in a TreeSet in descending order. |
descendingSet() | This method is used to display TreeSet elements in reverse order. |
ceiling(Object e) | It is used to display the smallest element from the TreeSet which is greater than or equal to given element. |
floor(Object e) | It is used to display the largest element from the TreeSet which is less than or equal to given element. |
1.Let us check the usage of add(Object e) method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("hello");
a1.add("Heya");
a1.add("Hey");
a1.add("Hji");
a1.add("Hiii");
System.out.println(a1);
}
}
Output: [Hey, Heya, Hiii, Hji, hello]
Screenshot:
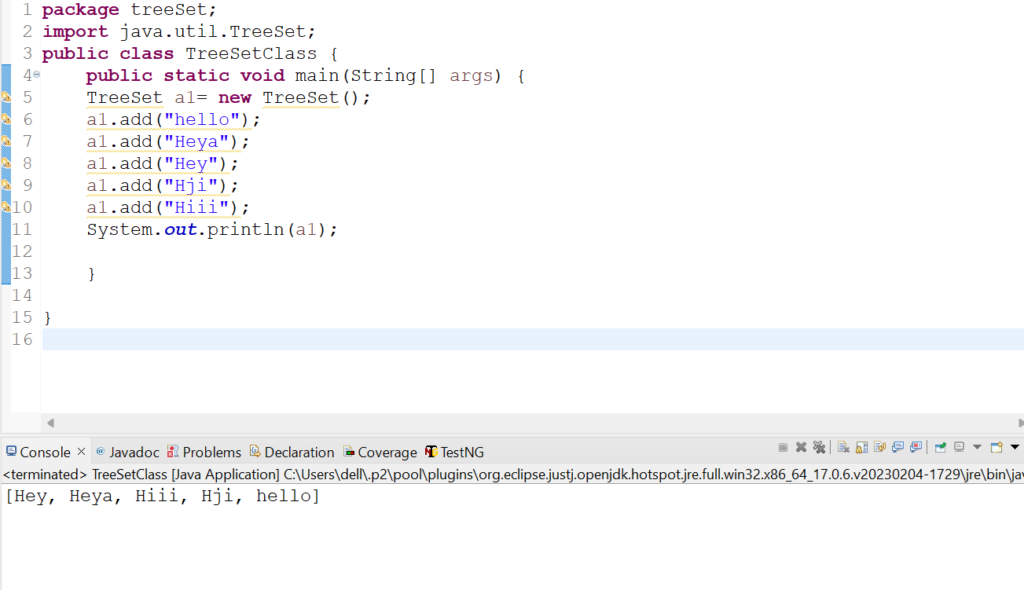
2. Let us check the usage of addAll(Collection c) method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("hello");
a1.add("Heya");
a1.add("Hey");
a1.add("Hji");
a1.add("Hiii");
TreeSet a2= new TreeSet();
a2.addAll(a1);
System.out.println(a2);
}
}
Output: [Hey, Heya, Hiii, Hji, hello]
Screenshot:
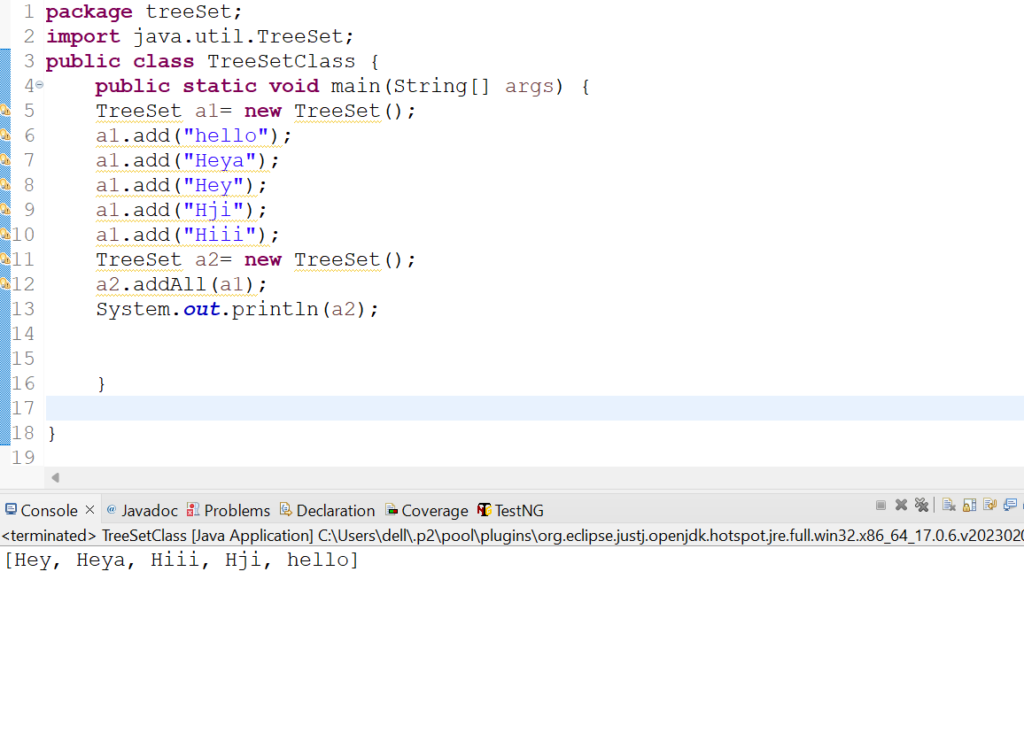
3. Let us check the usage of clear() method inTreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("hello");
a1.add("Heya");
a1.add("Hey");
a1.add("Hji");
a1.add("Hiii");
a1.clear();
System.out.println(a1);
}
}
Output: []
Screenshot:
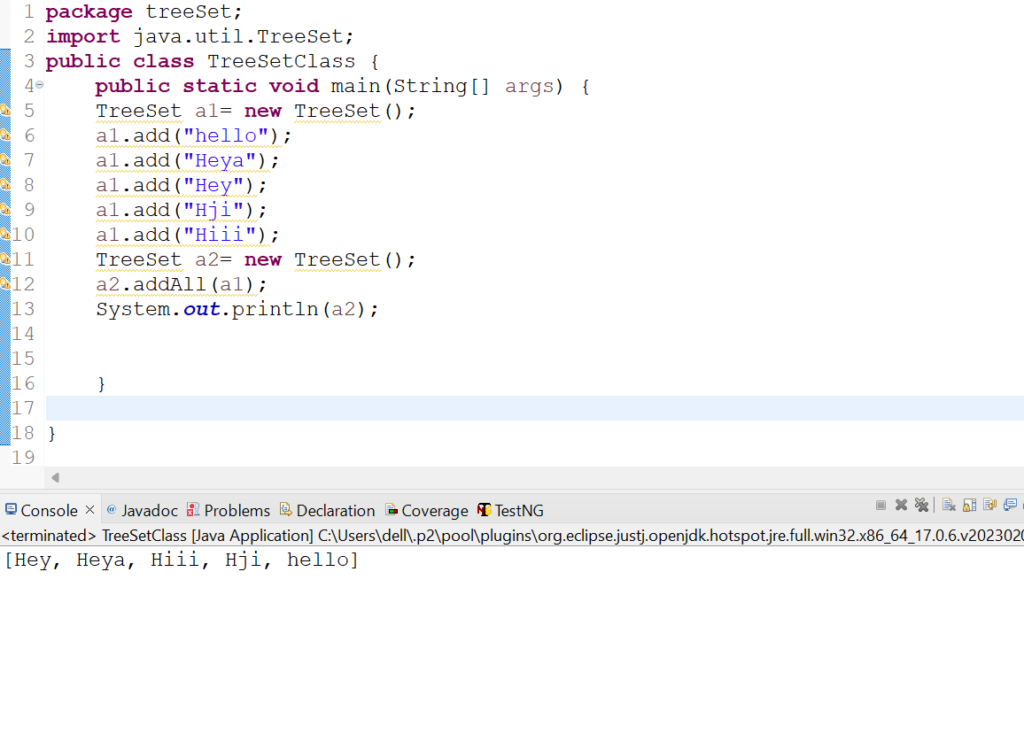
4. Let us check the usage of contains(Object o) method in TreeSet class.
Code Snippet:
package hashSetClass;
import java.util.HashSet;
public class HashSetClass {
public static void main(String[] args) {
HashSet a1= new HashSet();
a1.add("hello");
a1.add('c');
a1.add(3.4f);
a1.add(true);
a1.add(null);
a1.add(3.55);
a1.add("Hiii");
System.out.println("The given TreeSet contains: "+a1.contains("hello"));
System.out.println("The given TreeSet contains: "+a1.contains("Harish"));
}
}
Output: The given TreeSet contains: true
The given TreeSet contains: false
Screenshot:
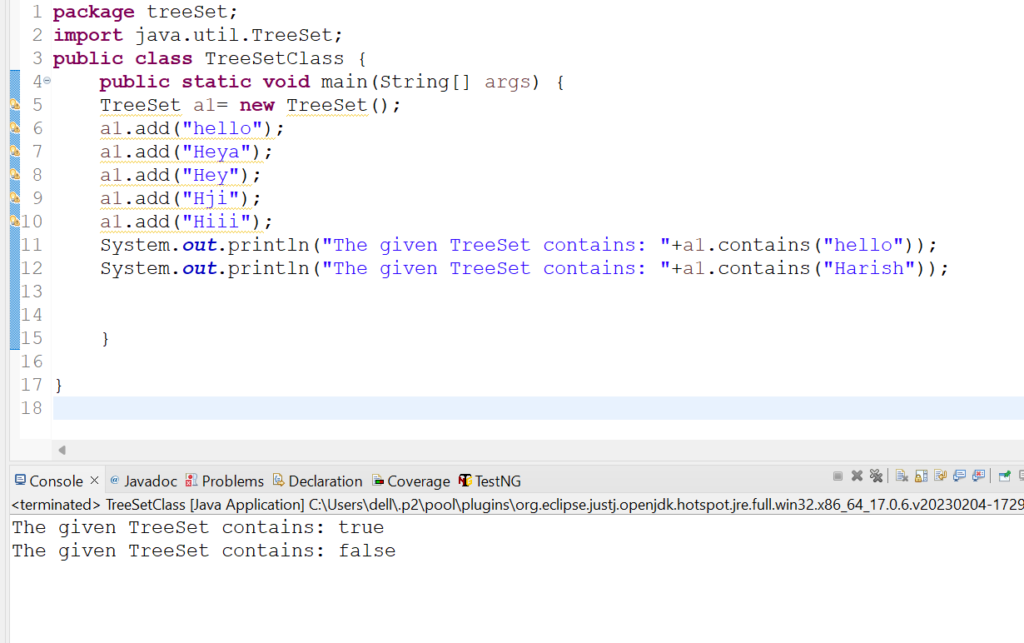
5. Let us check the usage of containsAll(Collection c) method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("hello");
a1.add("Heya");
a1.add("Hey");
a1.add("Hji");
a1.add("Hiii");
TreeSet a2= new TreeSet();
a2.add("hello");
a2.add("Heya");
a2.add("Hey");
System.out.println("Does a2 contains all elements of a1:"+a2.containsAll(a1));
System.out.println("Does a1 contains all elements of a2:"+a1.containsAll(a2));
}
}
Output:
Does a2 contains all elements of a1:false
Does a1 contains all elements of a2:true
Screenshot:
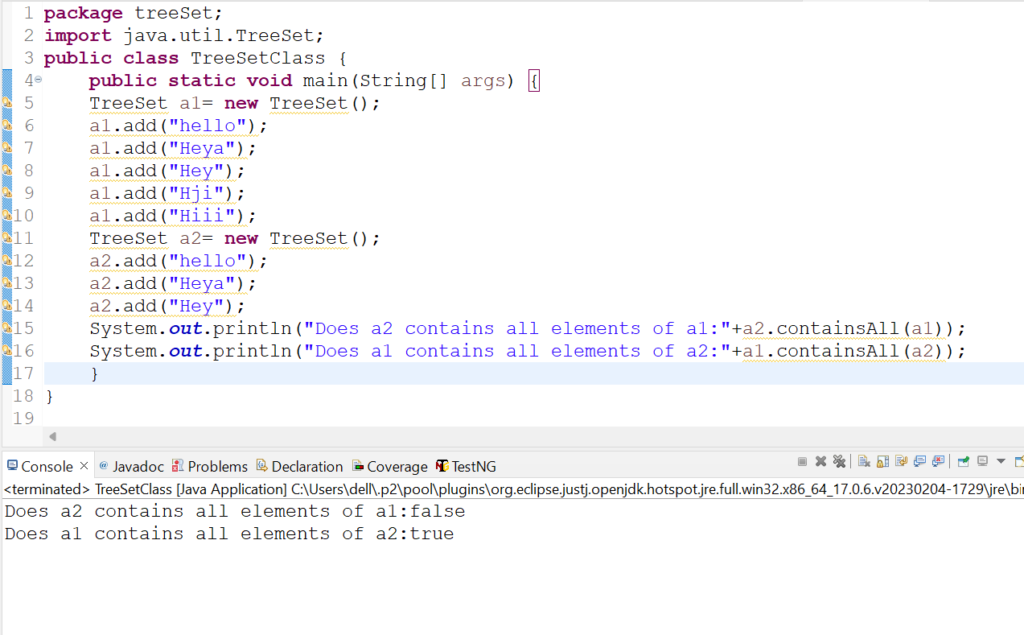
6. Let us check the usage of isEmpty() method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("hello");
a1.add("Heya");
a1.add("Hey");
a1.add("Hji");
a1.add("Hiii");
System.out.println("Is a1 TreeSet empty?: "+a1.isEmpty());
TreeSet a2= new TreeSet();
System.out.println("Is a2 TreeSet empty?: "+a2.isEmpty());
}
}
Output:
Is a1 TreeSet empty?: false
Is a2 TreeSet empty?: true
Screenshot:
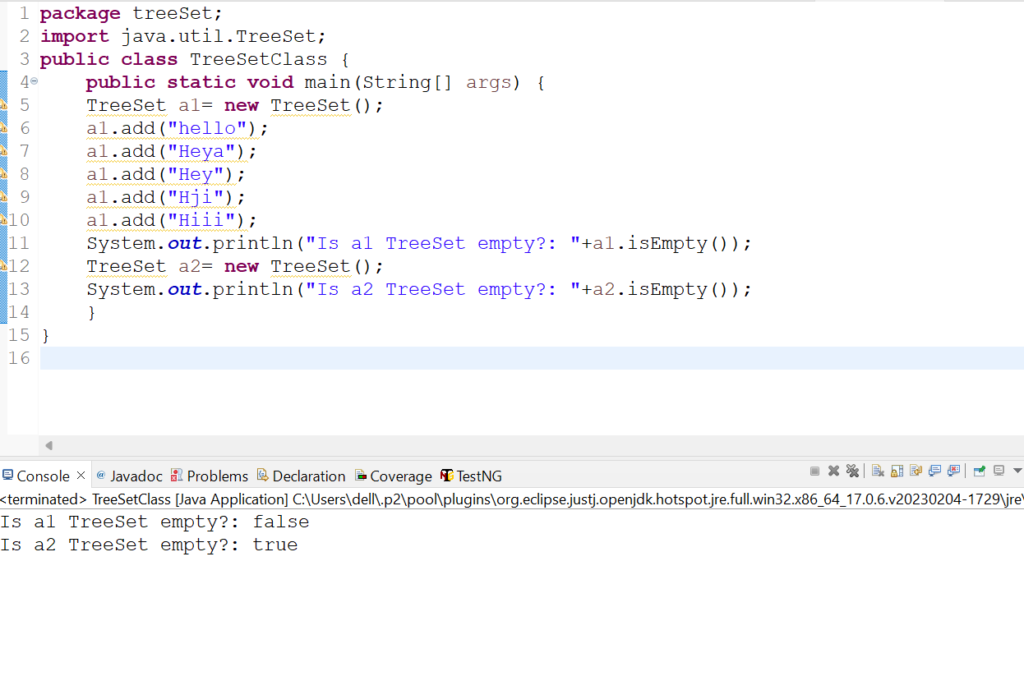
7. Let us check the usage of iterator() method in TreeSet class.
Code Snippet:
import java.util.Iterator;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("hello");
a1.add("Heya");
a1.add("Hey");
a1.add("Hji");
a1.add("Hiii");
Iterator a2= a1.iterator();
System.out.println("The collection elements values are: ");
while(a2.hasNext())
{
System.out.println(a2.next());
}
}
}
Output:
The collection elements values are:
Hey
Heya
Hiii
Hji
hello
Screenshot:
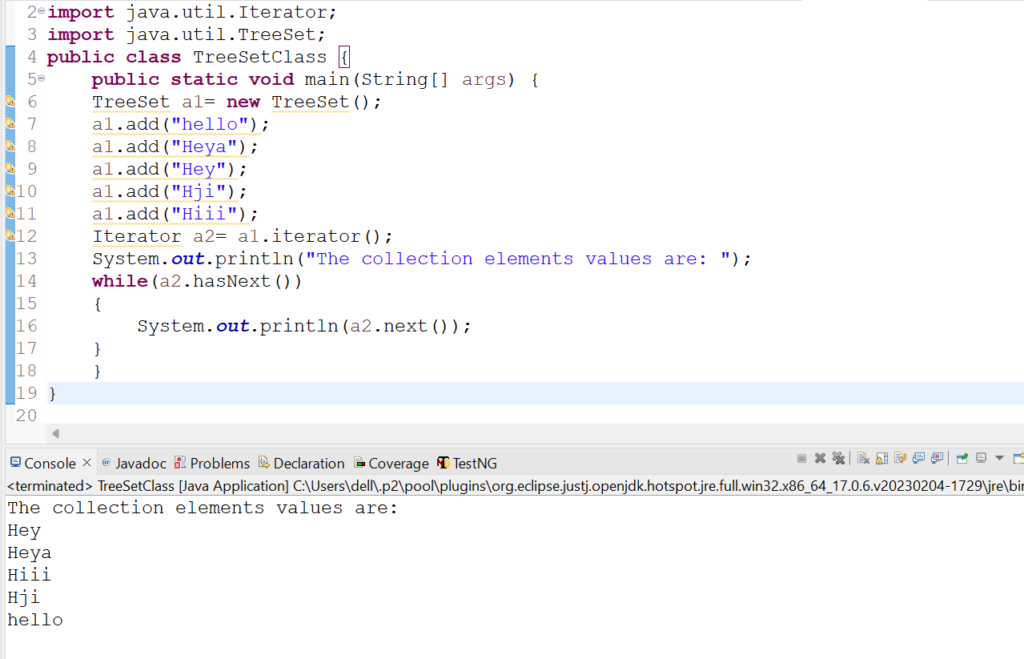
8. Let us check the usage of remove(Object o) method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("hello");
a1.add("Heya");
a1.add("Hey");
a1.add("Hji");
a1.add("Hiii");
a1.remove("hello");
System.out.println(a1);
}
}
Output: [Hey, Heya, Hiii, Hji]
Screenshot:
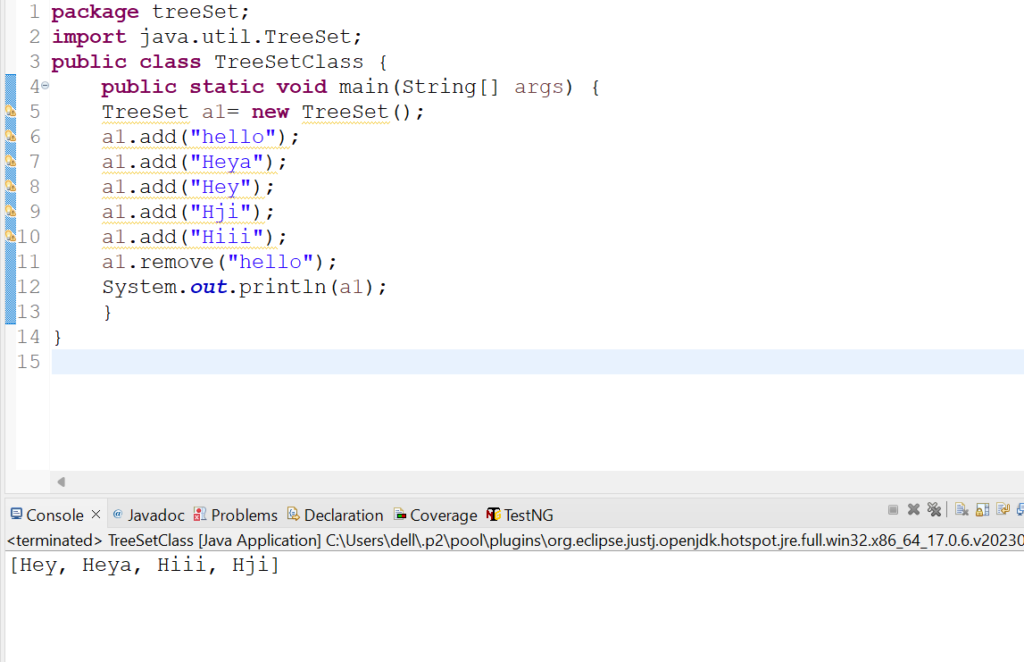
9. Let us check the usage of removeAll(Collection c) method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("hello");
a1.add("Heya");
a1.add("Hey");
a1.add("Hji");
a1.add("Hiii");
a1.add("Heiz");
TreeSet a2= new TreeSet();
a2.add("hello");
a2.add("Heya");
a2.add("Hey");
a2.add("Hiii");
a1.removeAll(a2);
System.out.println("Elements present in a1 after removing a2 elements from a1 are:"+a1);
}
}
Output:
Elements present in a1 after removing a2 elements from a1 are:[Heiz, Hji]
Screenshot:
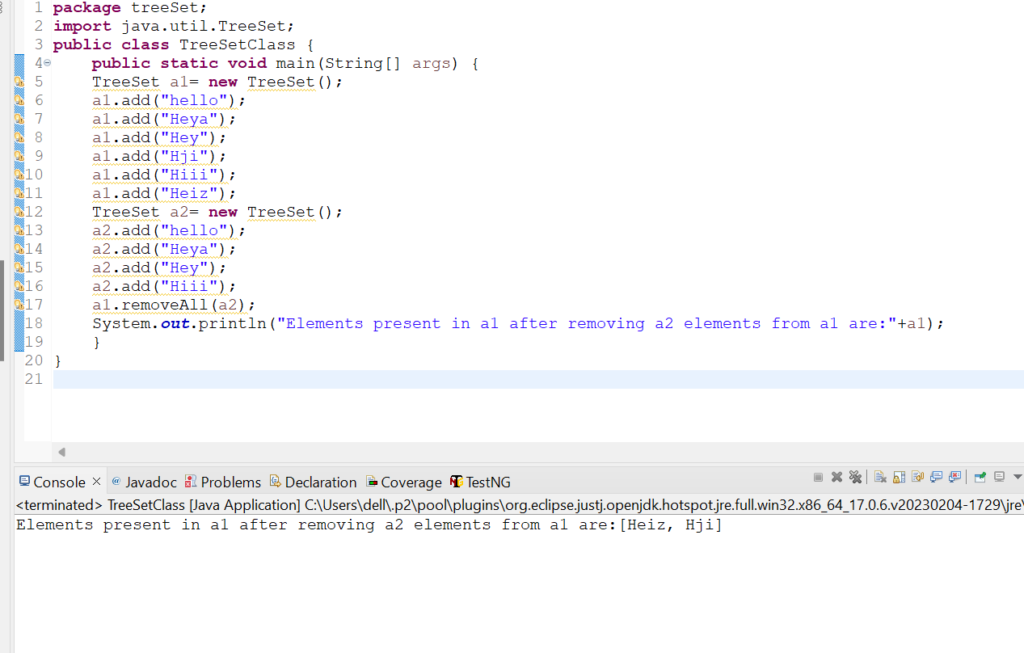
10. Let us check the usage of retainAll(Collection c) method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("hello");
a1.add("Heya");
a1.add("Hey");
a1.add("Hji");
a1.add("Hiii");
a1.add("Heiz");
TreeSet a2= new TreeSet();
a2.add("hello");
a2.add("Heya");
a2.add("Hey");
a2.add("Hiii");
a1.retainAll(a2);
System.out.println("Elements present in a1 after retaining a2 elements from a1 are:"+a1);
}
}
Output: Elements present in a1 after retaining a2 elements from a1 are:[Hey, Heya, Hiii, hello]
Screenshot:
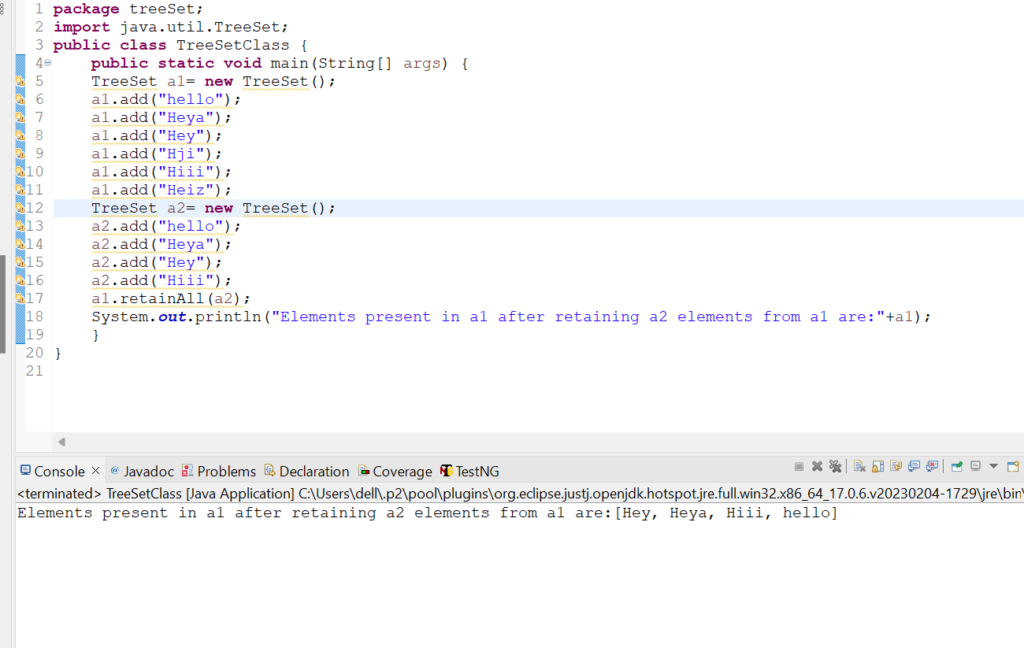
11. Let us check the usage of size() method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("hello");
a1.add("Heya");
a1.add("Hey");
a1.add("Hji");
a1.add("Hiii");
a1.add("Heiz");
System.out.println("The size of the collection elements present in the TreeSet is: "+a1.size());
}
}
Output: The size of the collection elements present in the TreeSet is: 6
Screenshot:

12. Let us check the usage of toArray() method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("hello");
a1.add("Heya");
a1.add("Hey");
a1.add("Hji");
a1.add("Hiii");
a1.add("Heiz");
Object a2[]= a1.toArray();
System.out.println("The list of array elements are:");
for(int i=0;i<=a2.length-1;i++)
{
System.out.println(a2[i]);
}
}
}
Output: The list of array elements are:
Heiz
Hey
Heya
Hiii
Hji
hello
Screenshot:
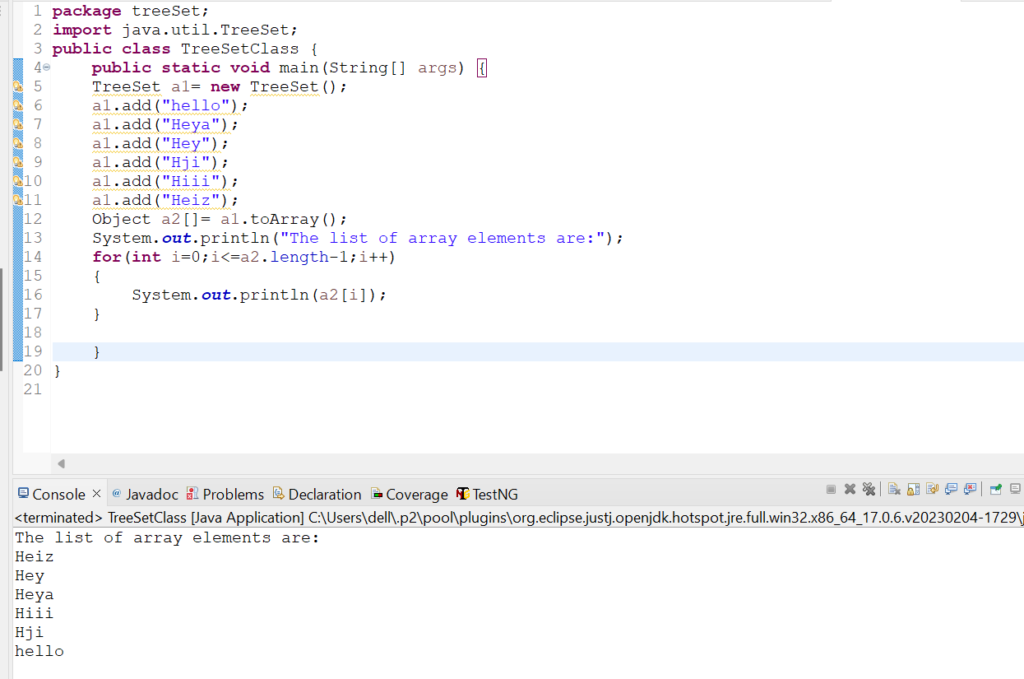
13. Let us check the usage of toString() method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("hello");
a1.add("Heya");
a1.add("Hey");
a1.add("Hji");
a1.add("Hiii");
a1.add("Heiz");
System.out.println(a1.toString());
}
}
Output: [Heiz, Hey, Heya, Hiii, Hji, hello]
Screenshot:
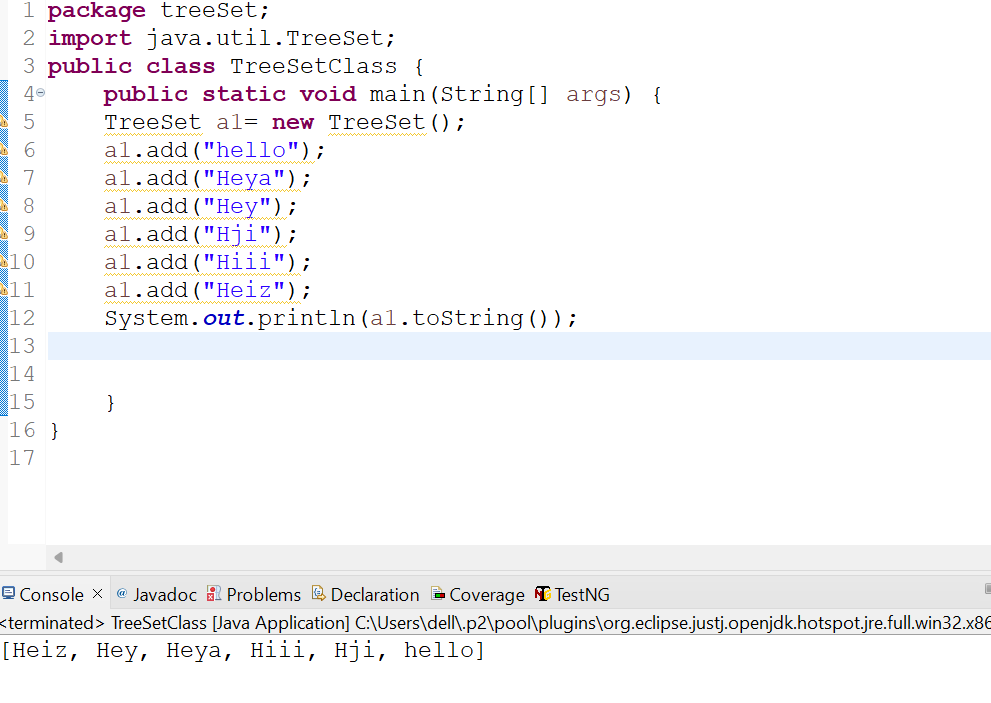
14. Let us check the usage of equals() method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("hello");
a1.add("Heya");
a1.add("Hey");
a1.add("Hji");
a1.add("Hiii");
a1.add("Heiz");
TreeSet a2= new TreeSet();
a2.add("hello");
a2.add("Heya");
a2.add("Hey");
a2.add("Hji");
a2.add("Hiii");
a2.add("Heiz");
TreeSet a3= new TreeSet();
a3.add("hello");
a3.add("Heya");
a3.add("Hey");
a3.add("Hji");
System.out.println("Is TreeSet a1 equal to a2 ?:"+ a1.equals(a2));
System.out.println("Is TreeSet a2 equal to a3 ?:"+ a2.equals(a3));
}
}
Output: Is TreeSet a1 equal to a2 ?:true
Is TreeSet a2 equal to a3 ?:false
Screenshot:
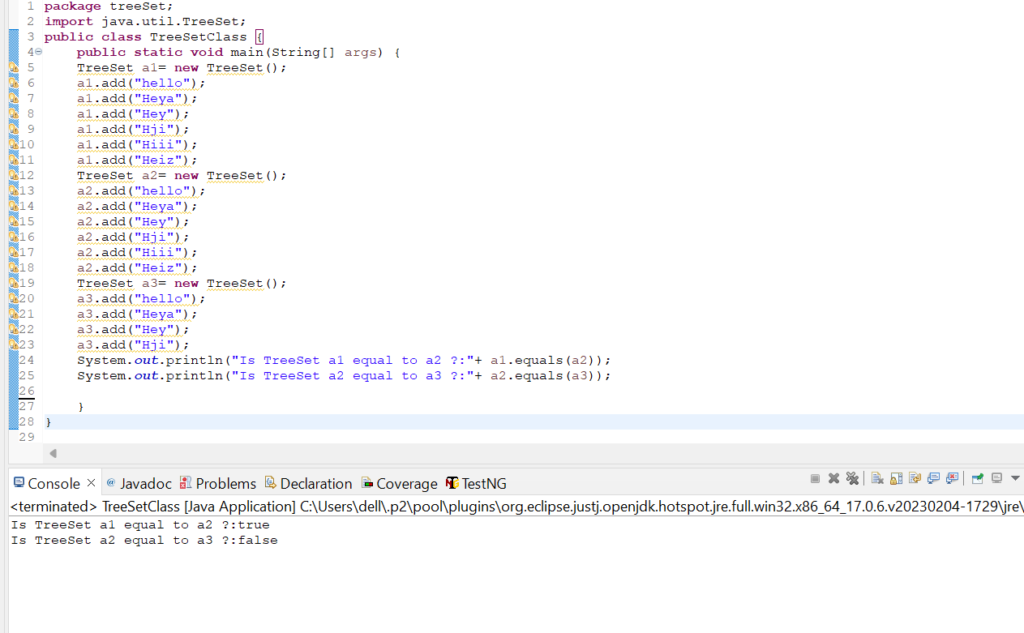
15. Let us check the usage of clone() method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("hello");
a1.add("Heya");
a1.add("Hey");
a1.add("Hji");
a1.add("Hiii");
a1.add("Heiz");
System.out.println("List of elements in TreeSet class: "+a1);
TreeSet clonea1= new TreeSet();
clonea1=(TreeSet)a1.clone();
System.out.println("List of elements after using clone method:"+ clonea1);
}
}
Output: List of elements in TreeSet class: [Heiz, Hey, Heya, Hiii, Hji, hello]
List of elements after using clone method:[Heiz, Hey, Heya, Hiii, Hji, hello]
Screenshot:
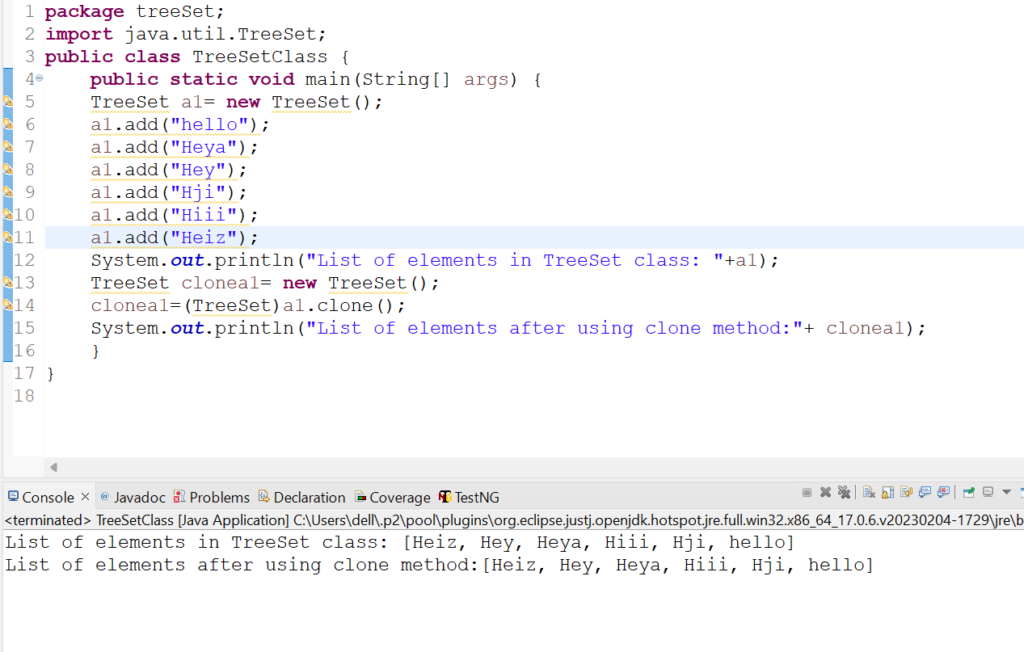
16. Let us check the usage of pollFirst() method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add(6);
a1.add(7);
a1.add(3);
a1.add(4);
a1.add(9);
a1.pollFirst();
System.out.println(a1);
}
}
Output: [4, 6, 7, 9]
Screenshot:
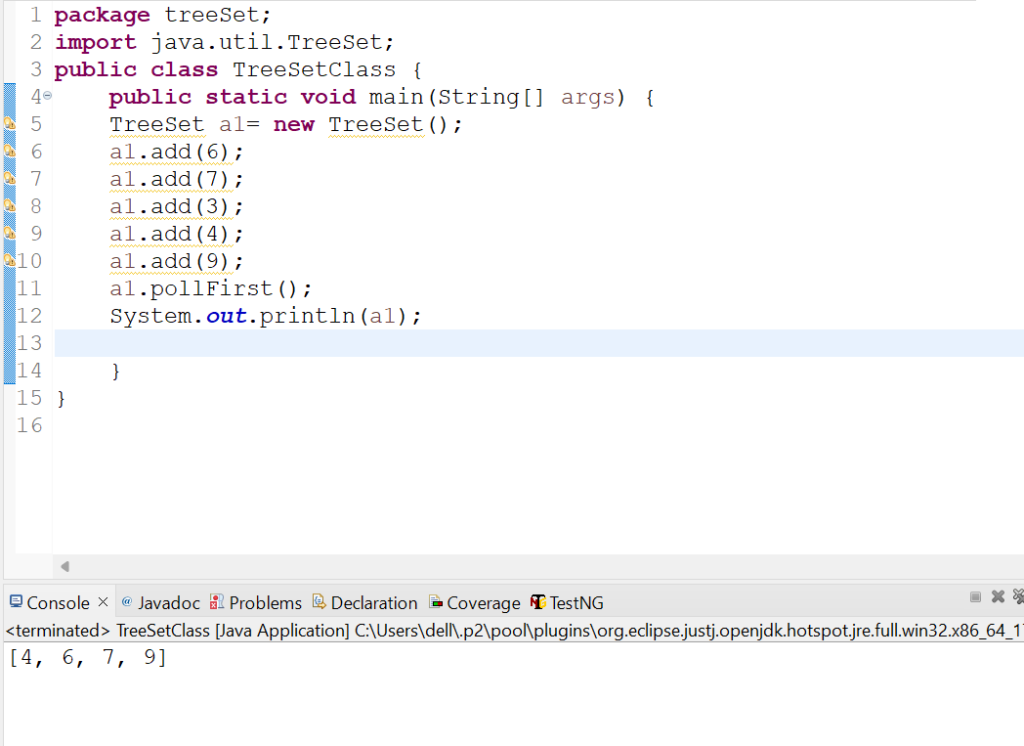
17. Let us check the usage of pollLast() method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add(6);
a1.add(7);
a1.add(3);
a1.add(4);
a1.add(9);
a1.pollLast();
System.out.println(a1);
}
}
Output: [3, 4, 6, 7]
Screenshot:
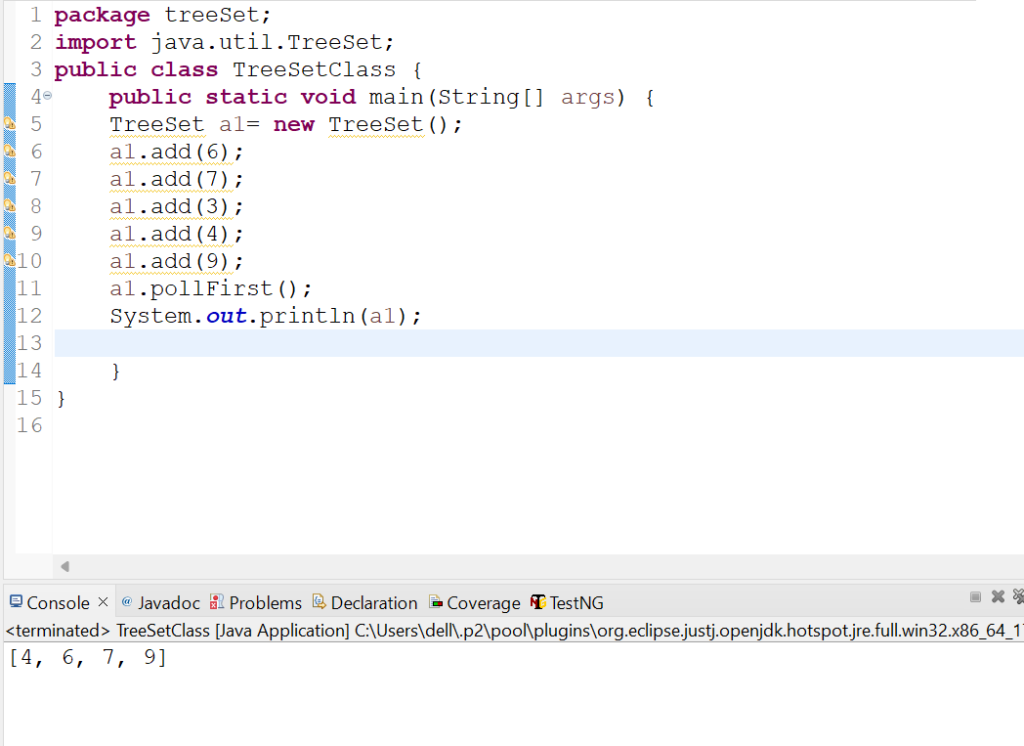
18. Let us check the usage of first() method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add(6);
a1.add(7);
a1.add(3);
a1.add(4);
a1.add(9);
System.out.println(a1.first());
}
}
Output: 3
Screenshot:
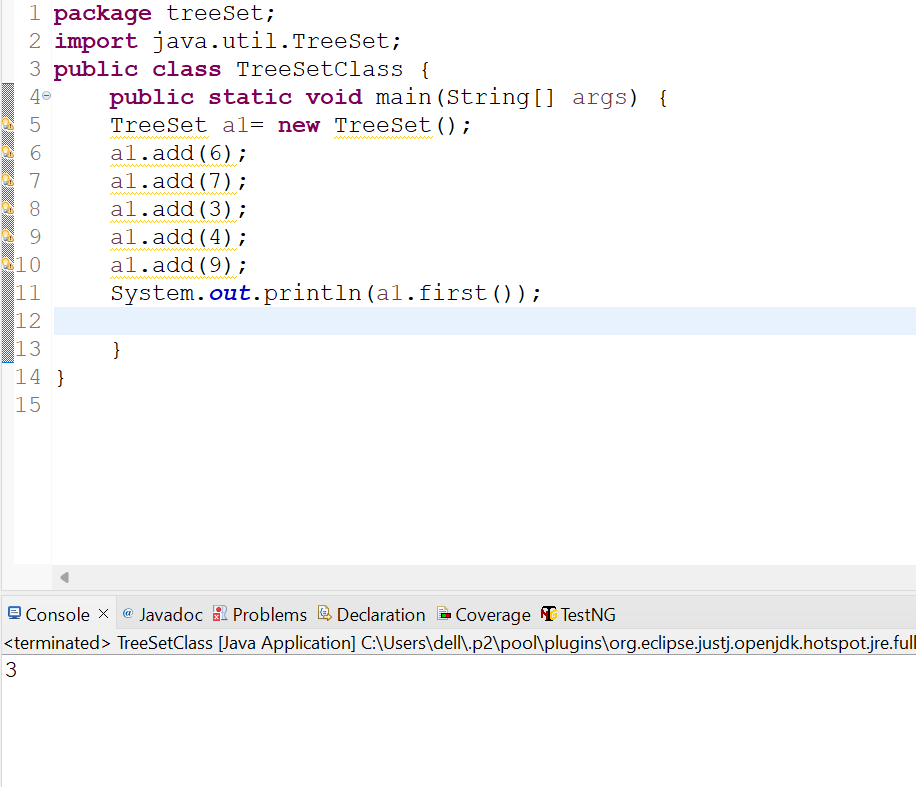
19. Let us check the usage of last() method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add(6);
a1.add(7);
a1.add(3);
a1.add(4);
a1.add(9);
System.out.println(a1.last());
}
}
Output: 9
Screenshot:
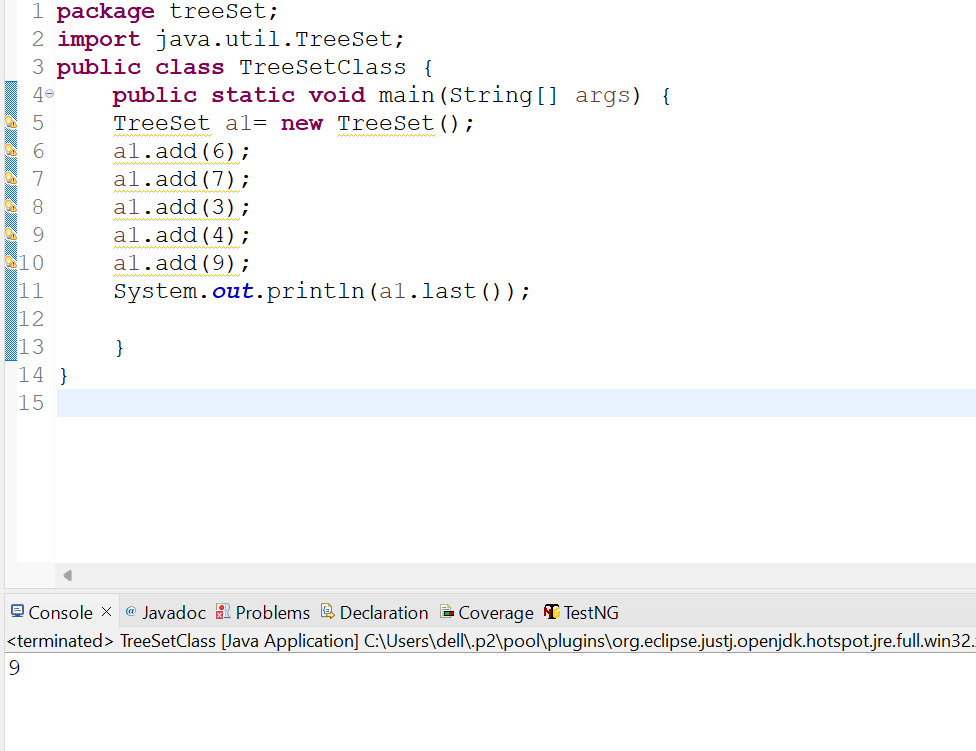
20. Let us check the usage of descendingIterator() method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.Iterator;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add(6);
a1.add(7);
a1.add(3);
a1.add(4);
a1.add(9);
Iterator a2= a1.descendingIterator();
System.out.println("The collection elements values in descending order are: ");
while(a2.hasNext())
{
System.out.println(a2.next());
}
}
}
Output: The collection elements values in descending order are:
9
7
6
4
3
Screenshot:
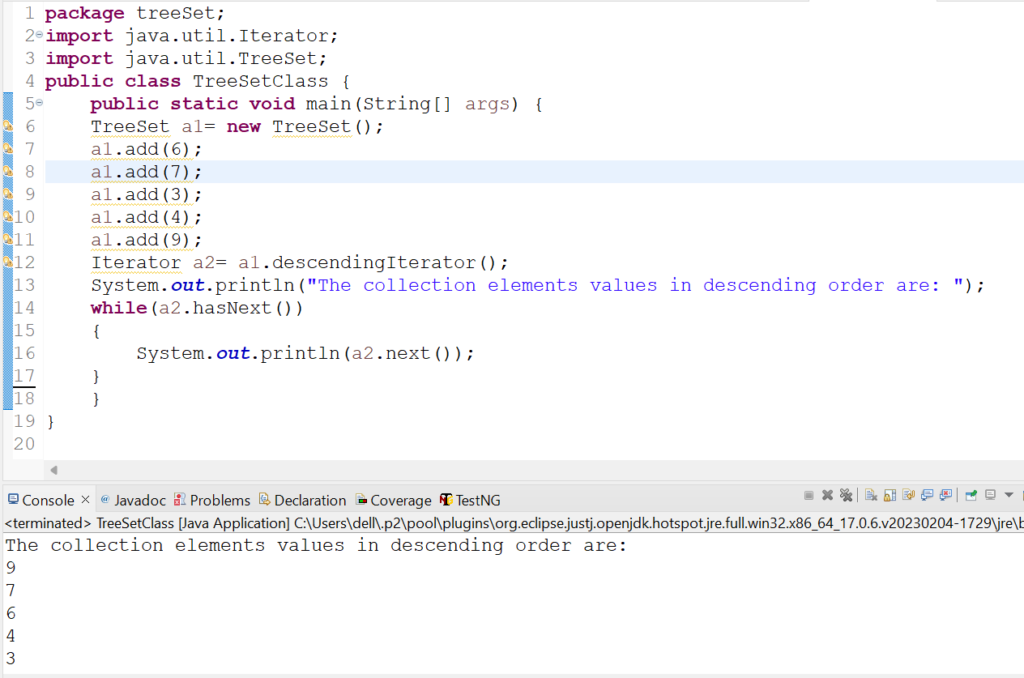
21. Let us check the usage of descendingSet() method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add("A");
a1.add("B");
a1.add("C");
a1.add("D");
a1.add("E");
System.out.println("Reverse Set: "+a1.descendingSet());
}
}
Output: Reverse Set: [E, D, C, B, A]
Screenshot:
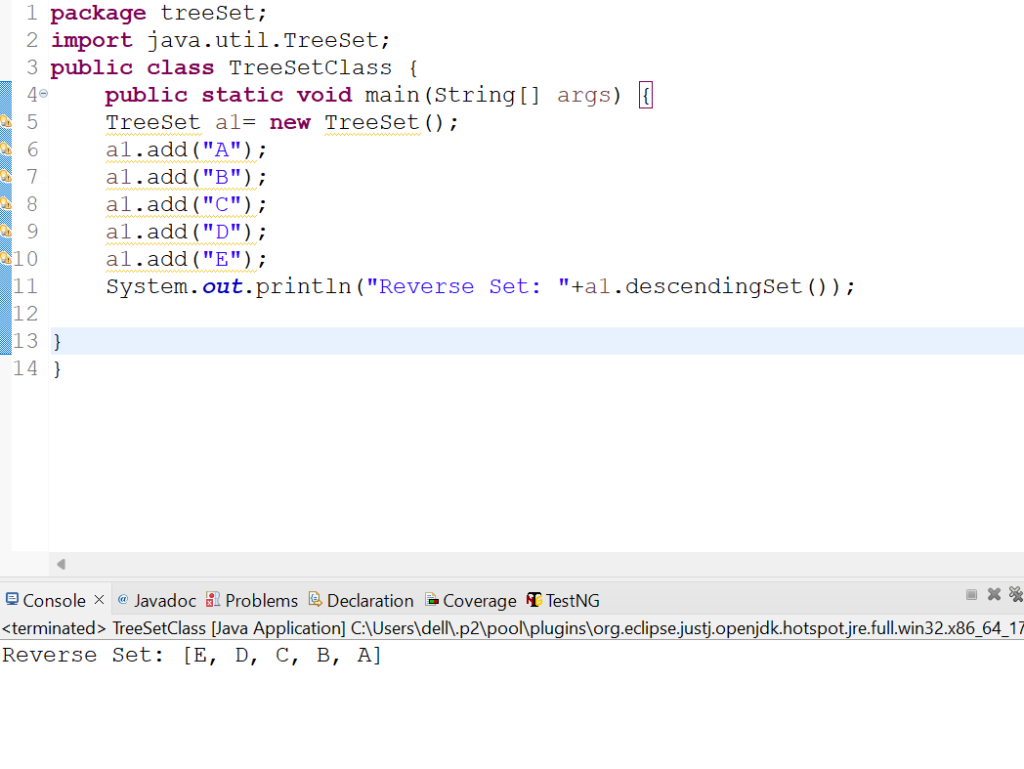
22. Let us check the usage of ceiling(Object e) method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add(10);
a1.add(20);
a1.add(30);
a1.add(40);
a1.add(50);
System.out.println("The given TreeSet elements :"+a1);
System.out.println("The ceiling value of 22 is :"+a1.ceiling(22));
}
}
Output: The given TreeSet elements :[10, 20, 30, 40, 50]
The ceiling value of 22 is :30
Screenshot:
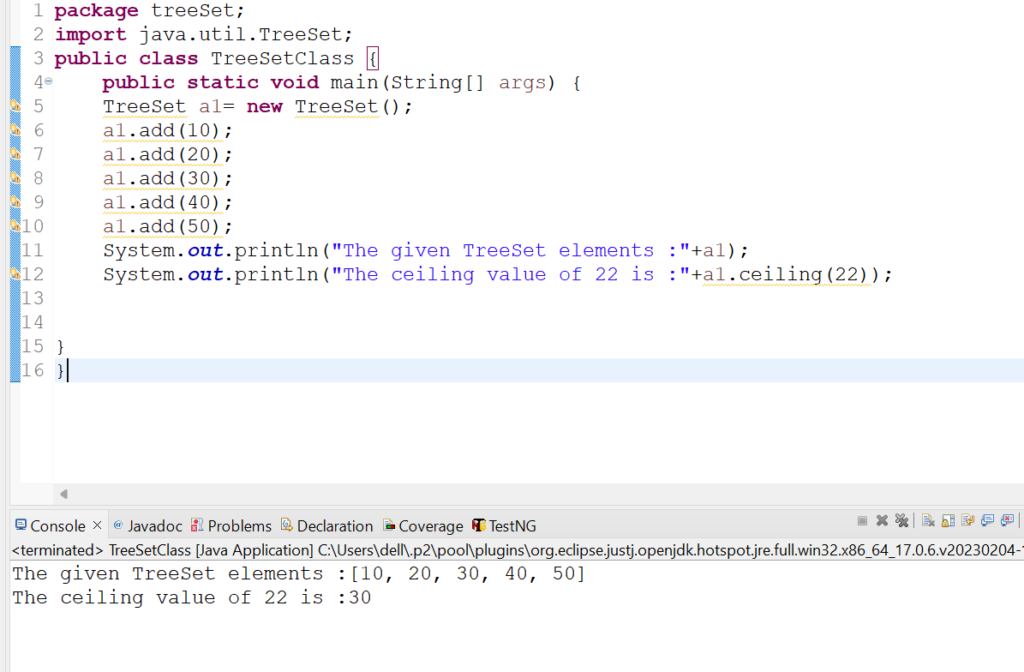
23. Let us check the usage of floor(Object e) method in TreeSet class.
Code Snippet:
package treeSet;
import java.util.TreeSet;
public class TreeSetClass {
public static void main(String[] args) {
TreeSet a1= new TreeSet();
a1.add(10);
a1.add(20);
a1.add(30);
a1.add(40);
a1.add(50);
System.out.println("The given TreeSet elements :"+a1);
System.out.println("The floor value of 42 is :"+a1.floor(40));
}
}
Output: The given TreeSet elements :[10, 20, 30, 40, 50]
The floor value of 42 is :40
Screenshot:
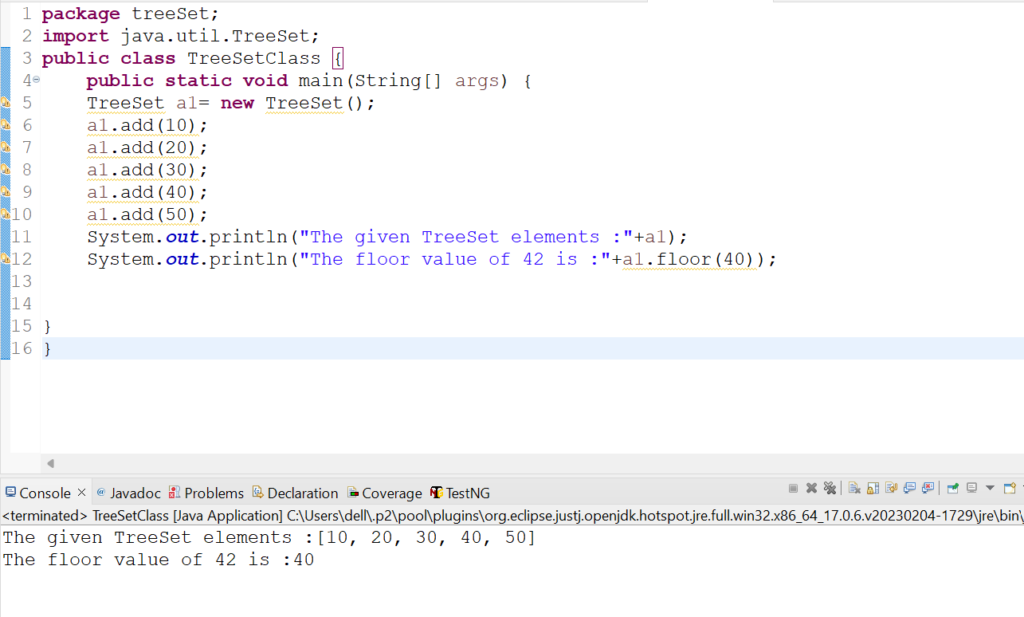
Difference between LinkedHashSet and TreeSet
SL NO: | LinkedHashSet | TreeSet |
1 | It is the subclass of HashSet and the implemented class of Set interface,Collection interface and Iterable interface | It is the implemented class of NavigationSet interface,SortedSet interface,Set interface,Collection interface and Iterable interface |
2 | It allows one null value | It does not allow any null value |
3 | Sorting happens after converting LinkedHashSet into ArrayList | By default sorting happens in ascending order |
4 | The growth strategy is that it increases its capacity when it reaches the load factor (default 0.75) | The growth strategy is that it grows dynamically |
5 | It’s threshold is 0.75 times its current capacity | It has no threshold |
Conclusion
TreeSet class is used for handling all the Collection elements in an effective way. It is necessary to understand the usage of all the methods of TreeSet for better handling of Collection elements. By thoroughly understanding the TreeSet class and its methods developers and testers can utitlise those concepts in their real life tasks.
Consult Us